


Detailed explanation of usage examples of PHP singleton mode and factory mode
Design pattern is a set of classification and cataloging summary of code design experience that is used repeatedly, known to most people. The purpose of using design patterns is to reuse code, make the code easier to understand by others, and ensure code reliability.
There is no doubt that design patterns are win-win for ourselves, others, and the system; design patterns make coding truly engineering; design patterns are the cornerstone of software engineering, just like the structure of a building.
Singleton pattern
The singleton pattern is very useful when you need to ensure that there can only be one instance of an object. It delegates control of creating objects to a single point, and only one instance of the application will exist at any time. A singleton class should not be instantiated outside the class. A singleton class should have the following elements.
Must have a constructor with access level private, effectively preventing the class from being instantiated at will.
Must have a static variable that holds an instance of the class.
There must be a public static method to access this instance, which is usually named GetInstance().
Must have a private, empty clone method to prevent the instance from being cloned.
The following is a simple example of a singleton class to illustrate
class ClassName { public static $_instance; private function construct() { # code... } private function clone() { # empty } public static function GetInstance() { if(!(self::$_instance instanceof self)) { self::$_instance = new self(); } return self::$_instance; } public function SayHi() { echo "Hi boy!"; } } $App= ClassName::GetInstance(); $App->SayHi(); /** * * Output * * Hi boy! * */
When you have a large number of When implementing classes of the same interface, instantiate the appropriate class at the appropriate time. If these new elements are scattered to every corner of the project, it will not only make the business logic confusing and make the project difficult to maintain. At this time, if the concept of factory mode is introduced, this problem can be solved well. We can also let the factory class return the appropriate instance for us through application configuration or by providing parameters.
Factory class, which puts the process of instantiating a class into each factory class, is specifically used to create objects of other classes. The factory pattern is often used in conjunction with interfaces, so that the application does not need to know the specific details of these instantiated classes. As long as the factory returns a class that supports a certain interface, it can be used conveniently. The following is a simple example to illustrate the use of factory classes.
interface ProductInterface { public function showProductInfo(); } class ProductA implements ProductInterface { function showProductInfo() { echo 'This is product A.'; } } class ProductB implements ProductInterface { function showProductInfo() { echo 'This is product B.'; } } class ProductFactory { public static function factory($ProductType) { $ProductType = 'Product' . strtoupper($ProductType); if(class_exists($ProductType)) { return new $ProductType(); } else { throw new Exception("Error Processing Request", 1); } } } //这里需要一个产品型号为 A 的对象 $x = ProductFactory::factory('A'); $x -> showProductInfo(); //这里需要一个产品型号为 B 的对象 $o = ProductFactory::factory('B'); $o -> showProductInfo(); //都可以调用showProductInfo方法,因为都实现了接口 ProductInterface.
Summary
Pattern is like the cornerstone of software engineering. Like the design drawings of a building, two patterns are exposed here: singleton pattern and engineering pattern. There is a static variable in the singleton class that stores an instance of itself, and provides a static method to obtain this static variable. Singleton classes should also mark the constructor and clone function as private to prevent the uniqueness of the instance from being violated. The factory pattern creates different types of instances based on the parameters passed in or the configuration of the program. The factory class returns objects. The factory class is crucial in the practice of polymorphic programming.
The above is the detailed content of Detailed explanation of usage examples of PHP singleton mode and factory mode. For more information, please follow other related articles on the PHP Chinese website!
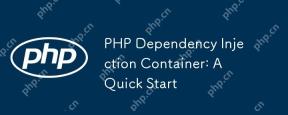
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
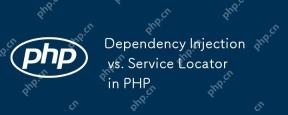
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
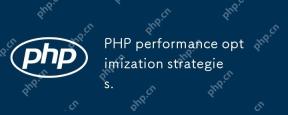
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
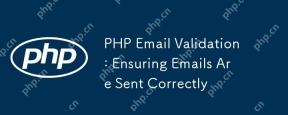
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
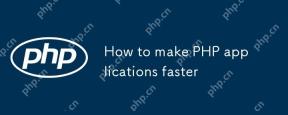
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
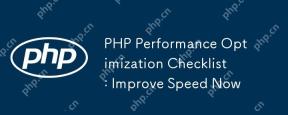
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
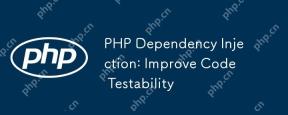
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
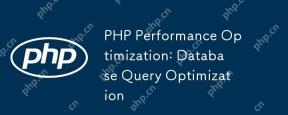
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
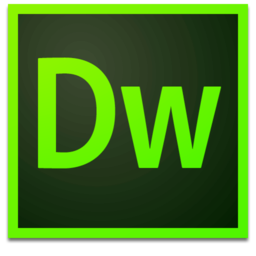
Dreamweaver Mac version
Visual web development tools
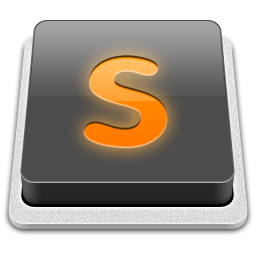
SublimeText3 Mac version
God-level code editing software (SublimeText3)
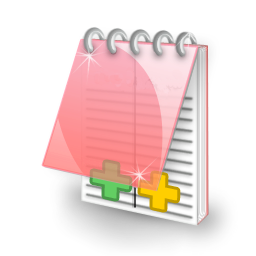
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
