This article mainly introduces the creation and access implementation methods of javascript objects in detail. Interested friends can refer to
JavaScript, which rarely reminds people of It has object-oriented features, and some people even say that it is not an object-oriented language because it has no classes. Yes, JavaScript doesn't really have classes, but JavaScript is an object-oriented language. JavaScript only has objects, and objects are objects, not instances of classes.
Because most objects in object-oriented languages are based on classes, people often confuse the concepts of class instances and objects. Objects are instances of classes, which is true in most languages, but not in JavaScript. Objects in JavaScript are prototype-based.
Creation and access
An object in JavaScript is actually an associative array composed of attributes. The attributes consist of names and values. The type of the value can be anyData type, or functions and other objects. Note that JavaScript has the characteristics of functional programming, so functions are also types of variables and most of the time do not need to be distinguished from general data types.
In JavaScript, you can create a simple object using the following method:
var foo = {}; foo.prop_1 = 'bar'; foo.prop_2 = false; foo.prop_3 = function() { return 'hello world'; } console.log(foo.prop_3());
In the above code, we create an object through var foo = {}; and assign its reference For foo,
obtain its members and assign values through foo.prop1, where {} is the representation method of object literals. You can also use var foo = new Object() to explicitly create an object.
1. Use associative array to access object members
We can also use associative array mode to create objects, the above code is modified to:
var foo = {}; foo['prop1'] = 'bar'; foo['prop2'] = false; foo['prop3'] = function() { return 'hello world'; }
In JavaScript, using the period operator and associative array references are equivalent, which means that any object (including the
this pointer) can use both modes. The advantage of using an associative array is that when we don't know the attribute name of the object, we can use variables as the index of the associative array. For example:
var some_prop = 'prop2'; foo[some_prop] = false;
2. Use object initializer to create objects
The above method is just to give you an understanding of the definition of JavaScript objects. When actually using it, we will use The following is a more compact and clear method:
var foo = { 'prop1': 'bar', prop2: 'false', prop3: function (){ return 'hello world'; } };
This defined method is called the object's initializer. Note that when using an initializer, Object property names are optional in quotes. Unless there are spaces or other characters that may cause ambiguity in the property name, there is no need to use quotes.
The above is the detailed content of Creation and access of JS objects. For more information, please follow other related articles on the PHP Chinese website!
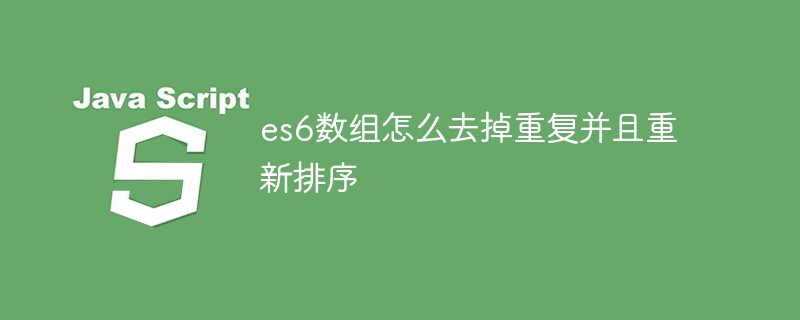
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
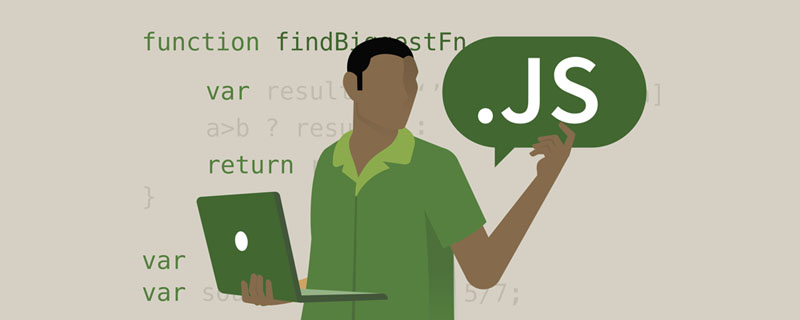
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
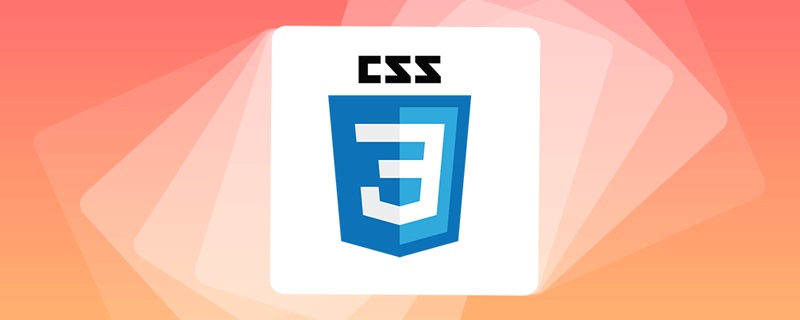
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
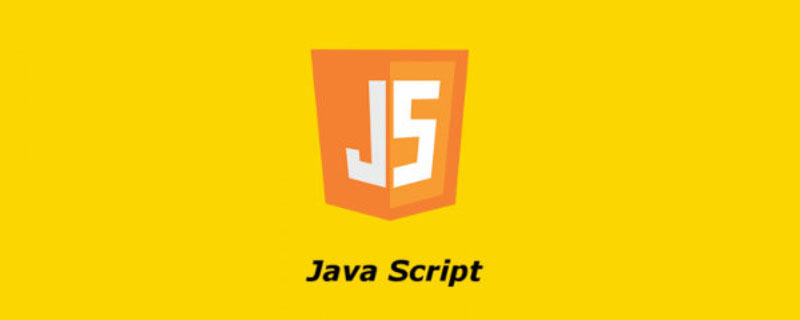
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
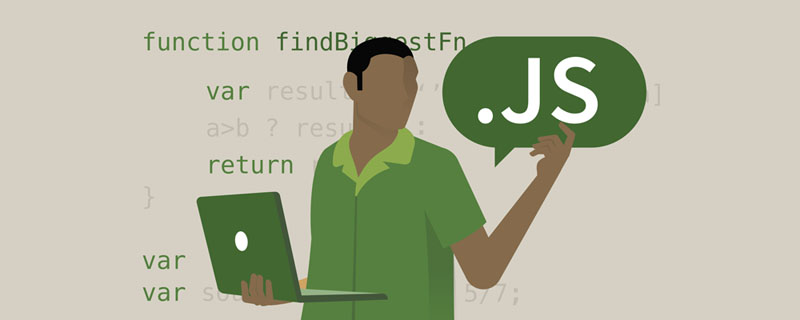
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
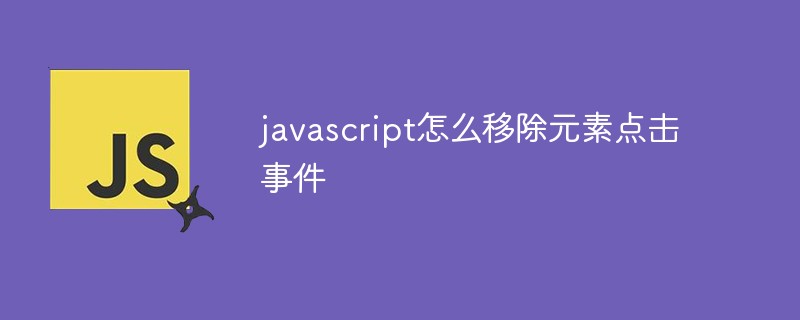
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
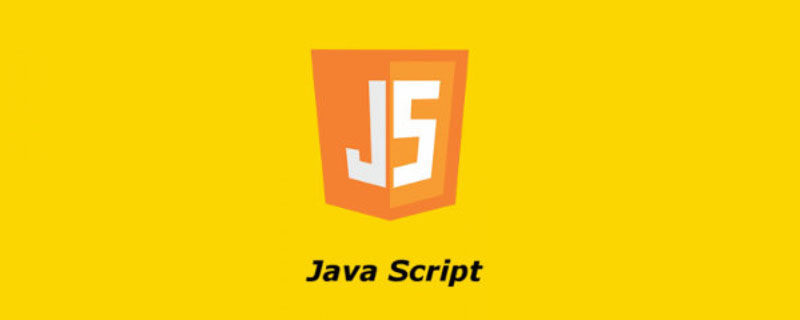
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
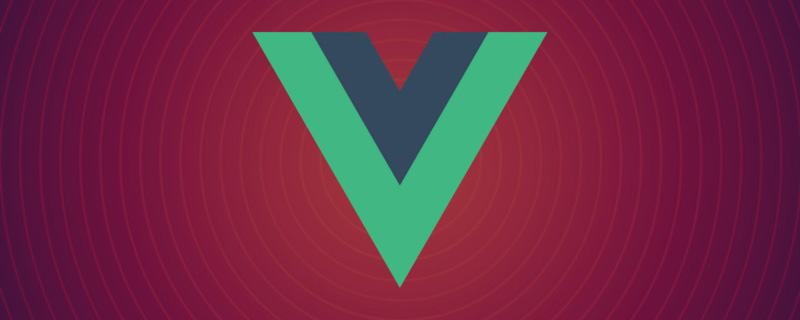
本篇文章整理了20+Vue面试题分享给大家,同时附上答案解析。有一定的参考价值,有需要的朋友可以参考一下,希望对大家有所帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
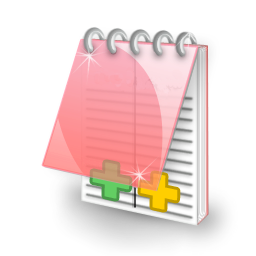
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
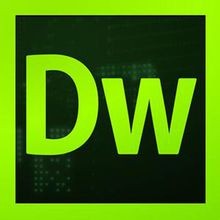
Dreamweaver CS6
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
