Initial exploration of Thread
Preface
In the past, everyone wrote single-threaded programs, all calling methods in the main function. You can clearly see that its efficiency is It is particularly low, just like using a single thread to crawl a website in Python, it can be said that it can make you vomit blood because the amount of data is too large. Today we will take a look at the learning of concurrent programming and multi-threading in Java
Create thread
There are many ways to create a thread, such as inheriting the Thread class and implementing the Runnable interface... Let's take a detailed look at the creation method.
Inherit Thread
Why inheriting
Thread
can directly call thestart()
method to start the thread, becausestart()
itself is a method of Thread, that is, it inherits the start() method of Thread, so the object of this class can call start() to start the thread
//继承Threadpublic class MyThread extends Thread { public void run() {for (int i = 0; i < 10; i++) { System.out.println(this.getName()+"正在跑"); } } }public class Test{public static void main(String[] args) { Mythread t1=new MyThread(); //创建对象t1.start(); //启动线程} }
Note: Inherit the creation method of the
Thread
class. An object can only create one thread, and multiple threads cannot share one object. Only one thread can correspond to one object, so Let's take a look at the class that implements theRunnable
interface to enable multiple threads to share the same object
Implements the Runnable interface
//实现Runnable接口public class Demo implements Runnable { @Overridepublic void run() {for(int i=0;i<10;i++) { System.out.println(Thread.currentThread().getName()+"正在跑"); } } }//测试类public class Test{public static void main(String[] args) { Demo d=new Demo(); //创建对象Thread thread1=new Thread(d); //为对象创建一个线程Thread thread2=new Thread(d); //创建另外一个线程//同时启动两个线程thread1.start(); thread2.start(); } }
It can be clearly seen from the above that an object of a class that implements the
Runnable
interface can be shared by multiple threads. It is not as simple as inheriting the Thread class and only using it for one thread.
The creation method
is created directly in the
main
method. If the object of the ordinary class created is outside, it must be final modified, which allows multiple threads to share an object at the same time. , this is the same as implementing theRunnable
interface. At this time, you need to control the synchronization conditions. If you define an object in the run method, then one thread corresponds to an object, which has the same effect as inheriting the Thread class. So you can freely choose according to the conditions
//普通的一个类public class Simple {public void display() {for(int i=0;i<10;i++) { System.out.println(Thread.currentThread().getName()+"正在跑"); } } }//线程测试类public class Test {public static void main(String[] args) { //如果在外面必须使用final,当然也可以直写在run方法中,不过写在外面可以实现多个线程共享一个对象//写在run方法中当前对象只能为一个线程使用,和继承Thread类一样的效果final Simple simple=new Simple(); //下面创建使用同一个对象创建同两个线程,实现多个线程共享一个对象,和实现Runnable接口一样的效果Thread t1=new Thread(){public void run() { simple.display(); }; }; Thread t2=new Thread(){public void run() { simple.display(); }; }; //启动这两个线程t1.start(); t2.start(); }}
Commonly used methods
static void sleep(long mils)
Make the running thread sleep for mils milliseconds, but it should be noted here that if the thread is locked, sleeping the thread will not release the lock
String getName()
Get the name of the thread. This method has been used in the above program
void setName(String name)
Set the name of the running thread to name
start()
Start the thread. The creation of the thread does not mean the starting of the thread. Only by calling the start() method can the thread really start running.
long getId()
Returns the identifier of the thread
Use
##run()
The code executed by the thread is placed in the run() method. The calls in the run method are ordered and are executed in the order in which the program runs.
Use the above method to create an instance
//线程的类,继承Threadpublic class MyThread1 extends Thread {public void run() { // 重载run方法,并且在其中写线程执行的代码块for (int i = 0; i < 10; i++) {// 获取线程的id和nameSystem.out.println("Thread-Name: " + this.getName() + " Thread-id: " + this.getId());try {this.sleep(1000); // 线程休眠1秒} catch (InterruptedException e) { e.printStackTrace(); } } } }//线程测试的类public class Test {public static void main(String[] args) { MyThread1 t1 = new MyThread1(); // 创建线程t1.setName("第一个线程"); // 设置线程的名字MyThread1 t2 = new MyThread1(); t2.setName("第二个线程"); t1.start(); // 启动线程,开始运行t2.start(); } }
- ##void join()
Wait for the thread to terminate before running other threads
- void join(long mils)
##UseThe time to wait for the thread is mils milliseconds , once this time has passed, other threads execute normally
//线程类public class MyThread1 extends Thread {public void run() { // 重载run方法,并且在其中写线程执行的代码块for (int i = 0; i < 10; i++) {// 获取线程的id和nameSystem.out.println("Thread-Name: " + this.getName() + " Thread-id: " + this.getId());try {this.sleep(1000); // 线程休眠1秒} catch (InterruptedException e) { e.printStackTrace(); } } } }//测试类public class Test {public static void main(String[] args) { MyThread1 t1 = new MyThread1(); // 创建线程t1.setName("第一个线程"); // 设置线程的名字t1.start(); // 启动线程,开始运行try { t1.join(); //阻塞其他线程,只有当这个线程运行完之后才开始运行其他的线程} catch (InterruptedException e) { e.printStackTrace(); }for (int i = 0; i < 10; i++) { System.out.println("主线程正在运行"); } } }//输出结果/*Thread-Name: 第一个线程 Thread-id: 9Thread-Name: 第一个线程 Thread-id: 9Thread-Name: 第一个线程 Thread-id: 9Thread-Name: 第一个线程 Thread-id: 9Thread-Name: 第一个线程 Thread-id: 9Thread-Name: 第一个线程 Thread-id: 9Thread-Name: 第一个线程 Thread-id: 9Thread-Name: 第一个线程 Thread-id: 9Thread-Name: 第一个线程 Thread-id: 9Thread-Name: 第一个线程 Thread-id: 9主线程正在运行主线程正在运行主线程正在运行主线程正在运行主线程正在运行主线程正在运行主线程正在运行主线程正在运行主线程正在运行主线程正在运行 */
getPriority()
- Get the current thread priority
setPriority(int num)
- Change the priority of the thread (0-10). The default is 5. The higher the priority, the higher the chance of obtaining CPU resources.
Use
//线程类public class MyThread1 extends Thread {public void run() { // 重载run方法,并且在其中写线程执行的代码块for (int i = 0; i < 10; i++) {// 获取线程的id和nameSystem.out.println("Thread-Name: " + this.getName() + " Thread-id: " + this.getId());try {this.sleep(1000); // 线程休眠1秒} catch (InterruptedException e) { e.printStackTrace(); } } } }//测试类public class Test {public static void main(String[] args) { MyThread1 t1 = new MyThread1(); // 创建线程t1.setName("第一个线程"); // 设置线程的名字MyThread1 t2 = new MyThread1(); t2.setName("第二个线程"); t2.setPriority(8); //设置第二个线程的优先级为8,第一个线程的优先级为5(是默认的)t1.start(); t2.start(); } }/* * 从上面的运行结果可以看出大部分的第二个线程都是在第一个线程之前开始执行的,也就是说优先级越高获得cpu执行的几率就越大 * /
setDaemon(boolean)
- Whether to set it as a daemon thread. If it is set to a daemon thread, the daemon thread will also be destroyed when the main thread is destroyed
isDaemon ()
- Determine whether it is a daemon thread
Use
//测试类public class MyThread1 extends Thread {public void run() { // 重载run方法,并且在其中写线程执行的代码块for (int i = 0; i < 10; i++) {// 获取线程的id和nameSystem.out.println("Thread-Name: " + this.getName() + " Thread-id: " + this.getId());try { Thread.sleep(1000); //休眠一秒,方便主线程运行结束} catch (InterruptedException e) { e.printStackTrace(); } } } }public class Test {public static void main(String[] args) { MyThread1 t1 = new MyThread1(); // 创建线程t1.setName("第一个线程"); // 设置线程的名字t1.setDaemon(true); t1.start();for (int i = 0; i < 1; i++) { System.out.println(i); } } }//结果:/* 0123456789Thread-Name: 第一个线程 Thread-id: 9*//* * 从上面的结果可以看出,一旦主线程结束,那么守护线程就会自动的结束 *
The above is the detailed content of The definition and common methods of Thread. For more information, please follow other related articles on the PHP Chinese website!
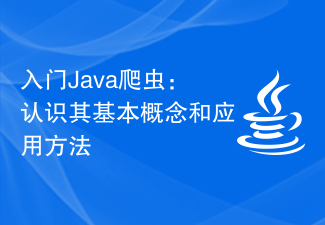
Java爬虫初探:了解它的基本概念与用途,需要具体代码示例随着互联网的快速发展,获取并处理大量的数据成为企业和个人不可或缺的一项任务。而爬虫(WebScraping)作为一种自动化的数据获取方法,不仅能够快速地收集互联网上的数据,还能够对大量的数据进行分析和处理。在许多数据挖掘和信息检索项目中,爬虫已经成为一种非常重要的工具。本文将介绍Java爬虫的基本概
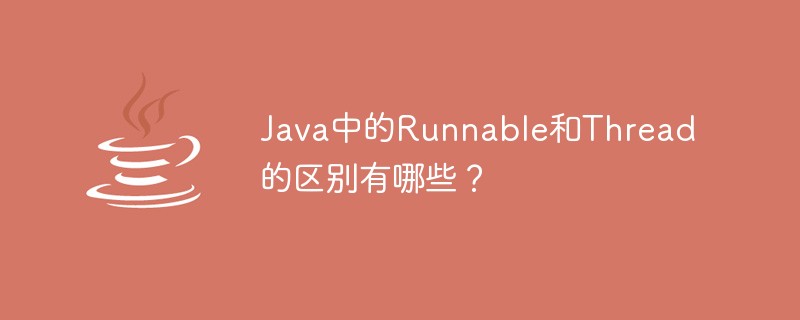
在java中可有两种方式实现多线程,一种是继承Thread类,一种是实现Runnable接口;Thread类是在java.lang包中定义的。一个类只要继承了Thread类同时覆写了本类中的run()方法就可以实现多线程操作了,但是一个类只能继承一个父类,这是此方法的局限。下面看例子:packageorg.thread.demo;classMyThreadextendsThread{privateStringname;publicMyThread(Stringname){super();this
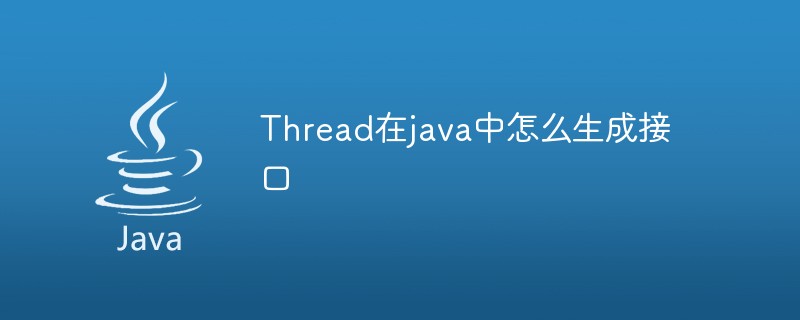
在java中,说到线程,Thread是必不可少的。线程是一个比过程更轻的调度执行器。为什么要使用线程?通过使用线程,可以将操作系统过程中的资源分配和执行调度分开。每个线程不仅可以共享过程资源(内存地址、文件I/O等),还可以独立调度(线程是CPU调度的基本单位)。说明1、Thread是制作线程最重要的类,这个词本身也代表线程。2、Thread类实现了Runnable接口。实例publicclassThreadDemoextendsThread{publicvoidrun(){for(inti=0
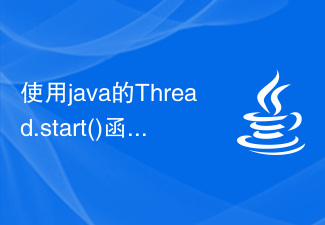
使用Java的Thread.start()函数启动新线程在Java中,我们可以使用多线程来实现并发执行多个任务。Java提供了Thread类来创建和管理线程。Thread类中的start()函数用于启动一个新线程,并执行该线程的run()方法中的代码。代码示例:publicclassMyThreadextendsThread{@Overr
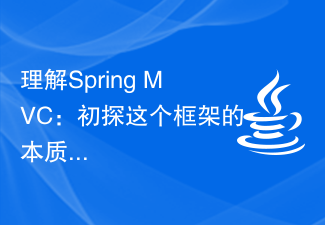
理解SpringMVC:初探这个框架的本质,需要具体代码示例引言:SpringMVC是一种基于Java的Web应用开发框架,它采用了MVC(Model-View-Controller)的设计模式,提供了一种灵活、可扩展的方式来构建Web应用程序。本文将介绍SpringMVC框架的基本工作原理和核心组件,并结合实际代码示例来帮助读者更好地理解这个框架的本
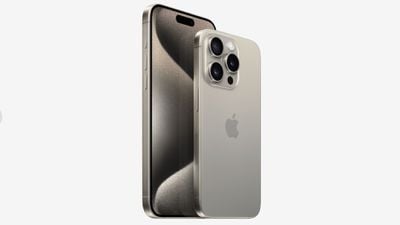
iPhone15Pro和iPhone15ProMax支持Thread网状网络协议。Thread网络技术被列为Pro型号的新功能,但不包括在iPhone15和iPhone15Plus中。苹果表示,iPhone15Pro是第一款带有Thread收音机的智能手机,可用于直接控制支持Thread的智能家居产品。Thread之前已被添加到HomePodmini和AppleTV中,但没有其他Apple设备具有Thread连接功能。在iPhone15Pro型号的新闻稿中,Apple解释说,Thread为“家庭
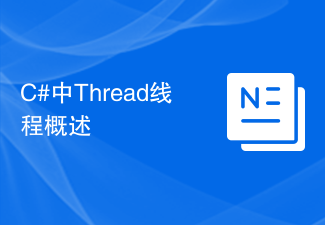
C#中Thread线程介绍,需要具体代码示例在C#中,Thread(线程)是一种用于执行代码的独立执行路径。通过使用线程,我们可以实现并行执行多个任务,提高程序的性能和响应能力。本文将介绍C#中Thread线程的基本概念、使用方法和相关代码示例。一、线程的基本概念线程是操作系统中的基本执行单位。在C#中,Thread类是用于创建和操作线程的主要工具。线程可以
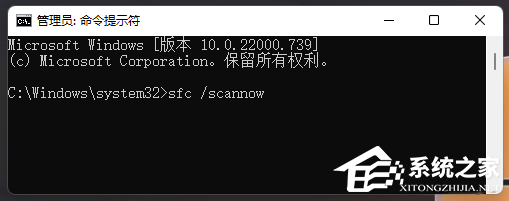
有用户反映,在安装了微软3月份的Win11更新补丁KB5035853后,出现了蓝屏死机错误,其中系统页面显示“ThreadStuckinDeviceDriver”。据了解,这种错误可能是由硬件或驱动程序问题引起的。以下是五种修复方法,希望能够快速解决电脑蓝屏问题。方法一:运行系统文件检查在命令提示符中运行【sfc/scannow】命令,可用于检测和修复系统文件的完整性问题。这个命令的作用是扫描并修复任何缺失或受损的系统文件,有助于确保系统的稳定性和正常运行。方法二:1.下载并打开“蓝屏修复工具”


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
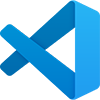
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
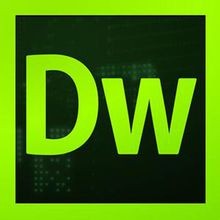
Dreamweaver CS6
Visual web development tools
