Class refers to: a definition that describes a thing, which is an abstract concept
Instance refers to: a specific individual of this kind of thing, which is a specific thing
For example:
"Person" is a class. "Zhang San" is a specific example of human beings
The same is true in programming. You first define a "class" yourself. When you need to use it, use the definition of "class" to create a specific example. .
Using the definition of a class to create an instance is called instantiation of the class.
The instantiation process is to call the constructor of the class to complete the data allocation of the instance
The following is an example of a washing machine to demonstrate:
This is a simple manual washing machine w instantiation Washer class:
1 #class Washer: 2 class Washer:#定义一个Washer类 3 4 def __init__(self): #这是定义类的构造函数,也是一个特殊的实例方法 5 self.water=0 #实例的属性(变量) 6 self.scour=0 7 8 def add_water(self,water):#实例的方法(函数),可以接受实例化后传过来的参数给类内实例变量,又因为实例继承了类的属性和方法,所以 9 #相当于传递给了类的实例化对象的实例变量10 print('Add water:',water)11 self.water=water#在类的方法内引用实例变量用 self.属性名12 13 def add_scour(self,scour):14 self.scour=scour15 print('Add scour:',scour)16 17 def start_wash(self):18 print('Start wash...')19 20 if __name__=='__main__':21 w=Washer()#类的实例化22 w.add_water(10)#传递参数到实例变量23 w.add_scour(2)24 w.start_wash()
Execution result:
w.add_scour(2),必要的时候加上参数。
Let’s change the program to make a semi-automatic washing machine , that is, within the class, methods call each other. washa.py:
1 class Washer: 2 3 def __init__(self): 4 self.water=0 5 self.scour=0 6 7 def set_water(self,water): 8 self.water=water 9 self.add_water()10 11 def set_scour(self,scour):12 self.scour=scour13 self.add_scour()#在类内调用函数,用 self.方法名14 15 def add_water(self):16 print('Add water:',self.water)17 18 def add_scour(self):19 print('Add scour:',self.scour)20 21 def start_wash(self):22 print('Start wash...')23 24 if __name__=='__main__':25 w=Washer()26 w.set_water(10)27 w.set_scour(2)28 w.start_wash()29can also be changed to:
1 class Washer: 2 3 def __init__(self): 4 self.water=0 5 self.scour=0 6 7 def set_water(self,water): 8 self.water=water 9 10 def set_scour(self,scour):11 self.scour=scour 12 13 def add_water(self):14 print('Add water:',self.water)15 16 def add_scour(self):17 print('Add scour:',self.scour)18 19 def start_wash(self):20 self.add_water()21 self.add_scour()22 print('Start wash...')23 24 if __name__=='__main__':25 w=Washer()26 w.set_water(10)27 w.set_scour(2)28 w.start_wash()Running result:
Make the following changes, the user can wash clothes without setting the water and detergent amount of the washing machine:
1 class Washer: 2 3 def __init__(self): 4 self.water=10#将实例属性修改为默认的标准洗衣程序需要的量 5 self.scour=2 6 7 def set_water(self,water): 8 self.water=water 9 10 def set_scour(self,scour):11 self.scour=scour 12 13 def add_water(self):14 print('Add water:',self.water)15 16 def add_scour(self):17 print('Add scour:',self.scour)18 19 def start_wash(self):20 self.add_water()21 self.add_scour()22 print('Start wash...')23 24 if __name__=='__main__':25 w=Washer()26 ## w.set_water(10) #这两行代码注释掉,代表用户不必设置水和洗涤剂量27 ## w.set_scour(2)28 w.start_wash()29But In this way, only 10L of water and 2L of detergent can be added, which is not good and needs to be improved. Use the constructor.
1 class Washer: 2 3 def __init__(self,water=10,scour=2): 4 self.water=water 5 self.scour=scour 6 7 def set_water(self,water): 8 self.water=water 9 10 def set_scour(self,scour):11 self.scour=scour 12 13 def add_water(self):14 print('Add water:',self.water)15 16 def add_scour(self):17 print('Add scour:',self.scour)18 19 def start_wash(self):20 self.add_water()21 self.add_scour()22 print('Start wash...')23 24 if __name__=='__main__':25 w=Washer()26 w.start_wash()27 28 wb=Washer(100,10)29 wb.start_wash()30 31 wc=Washer(100,10)32 wc.set_water(50)33 wc.set_scour(5)34 wc.start_wash()35 36 37 38 39
Run result:
The constructor can make the instance object more abundant when the class is instantiated, and has the function of instantiating different types of instances.
The above is the detailed content of Introduction to how to use the constructor. For more information, please follow other related articles on the PHP Chinese website!
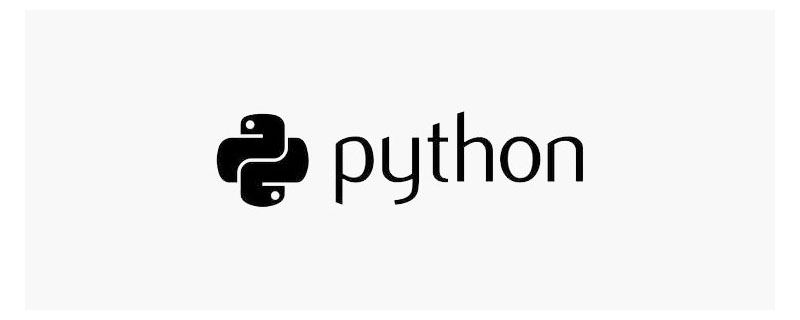
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
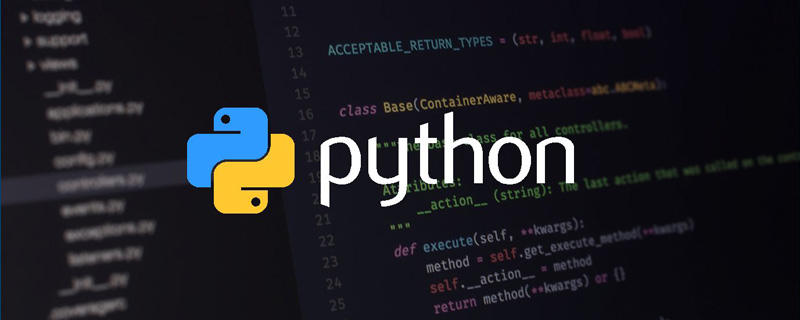
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
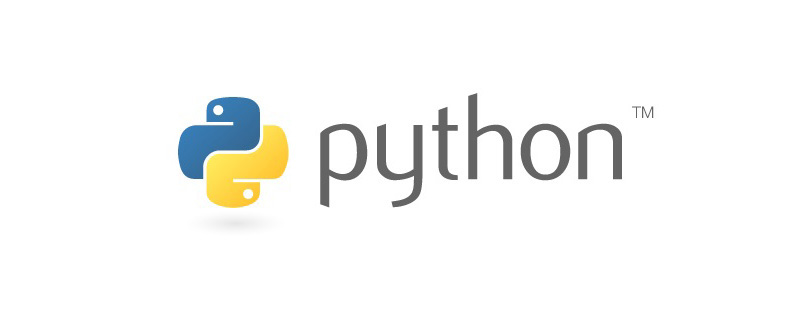
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
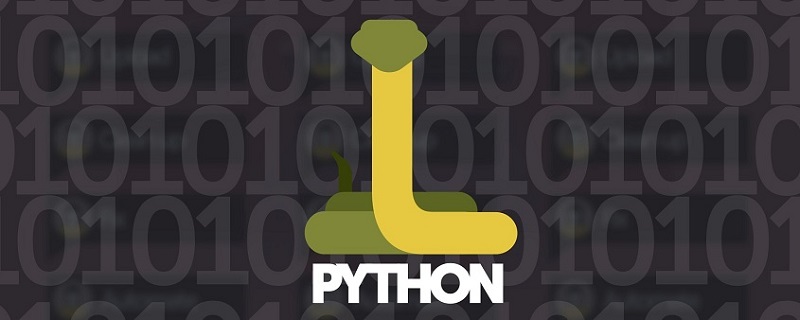
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
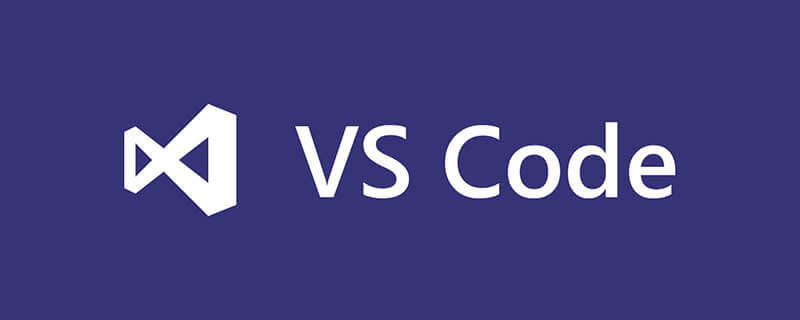
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
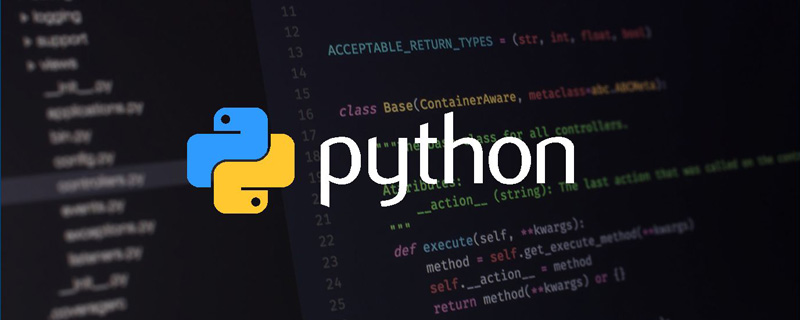
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。
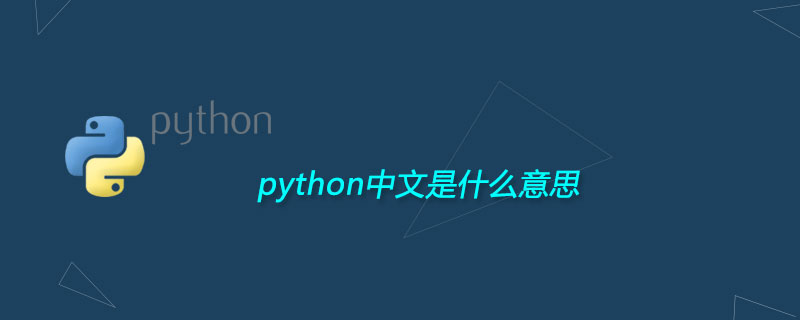
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
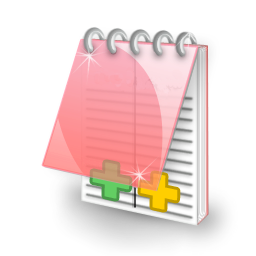
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
