The final keyword in Java is very important, it can be applied to classes, methods and variables. In this article I will take you to see what is the final keyword? What does it mean to declare variables, methods and classes as final? What are the benefits of using final? Finally, there are some examples of using the final keyword. final is often used together with static to declare constants. You will also see how final can improve application performance.
What is the meaning of the final keyword?
Final is a reserved keyword in Java that can declare member variables, methods, classes and local variables. Once you make the reference declaration final, you cannot change the reference. The compiler will check the code and if you try to initialize the variable again, the compiler will report a compilation error.
What is a final variable?
Any member variables or local variables (variables in methods or code blocks are called local variables) that are declared final are called final variables. Final variables are often used with the static keyword as constants. The following is an example of a final variable:
public static final String LOAN = "loan"; LOAN = new String("loan") //invalid compilation error
Final variables are read-only.
What is a final method?
Final can also declare methods. Adding the final keyword in front of a method means that this method cannot be overridden by methods of subclasses. If you think the function of a method is complete enough and does not need to be changed in subclasses, you can declare the method as final. Final methods are faster than non-final methods because they are statically bound at compile time and do not need to be dynamically bound at runtime. The following is an example of a final method:
class PersonalLoan{ public final String getName(){ return "personal loan"; } } class CheapPersonalLoan extends PersonalLoan{ @Override public final String getName(){ return "cheap personal loan"; //compilation error: overridden method is final } }
What is a final class?
A class modified with final is called a final class. Final classes are usually functionally complete and they cannot be inherited. There are many classes in Java that are final, such as String, Interger and other wrapper classes. The following is an example of the final class:
final class PersonalLoan{ } class CheapPersonalLoan extends PersonalLoan{ //compilation error: cannot inherit from final class }
The benefits of the final keyword
The following summarizes some of the benefits of using the final keyword
The final keyword improves performance. Both JVM and Java applications cache final variables.
Final variables can be safely shared in a multi-threaded environment without additional synchronization overhead.
Using the final keyword, the JVM will optimize methods, variables and classes.
Immutable class
To create an immutable class, use the final keyword. An immutable class means that its objects cannot be changed once they are created. String is a representative of immutable classes. Immutable classes have many benefits. For example, their objects are read-only and can be safely shared in a multi-threaded environment without additional synchronization overhead.
The above is the detailed content of What is the use of final keyword in Java?. For more information, please follow other related articles on the PHP Chinese website!
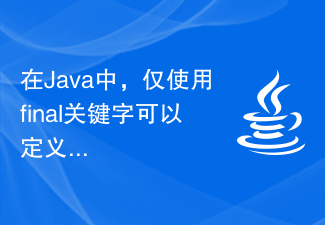
常量变量是其值固定且程序中只存在一个副本的变量。一旦你声明了一个常量变量并给它赋值,你就不能在整个程序中再次改变它的值。与其他语言不同,Java不直接支持常量。但是,你仍然可以通过声明一个变量为静态和final来创建一个常量。静态-一旦你声明了一个静态变量,它们将在编译时加载到内存中,即只有一个副本可用。Final-一旦你声明了一个final变量,就不能再修改它的值。因此,你可以通过将实例变量声明为静态和final来在Java中创建一个常量。示例 演示classData{&am
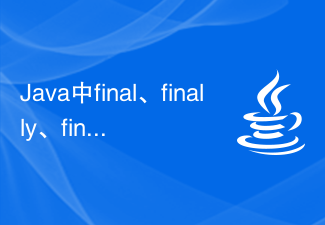
Java中final、finally、finalize的区别,需要具体代码示例在Java编程中,经常会遇到final、finally、finalize这三个关键词,它们虽然拼写相似,但却有不同的含义和用法。本文将详细解释这三个关键词的区别,同时给出代码示例以帮助读者更好地理解。一、final关键字final关键字可以用于类、方法和变量。它的作用是使被修饰的类
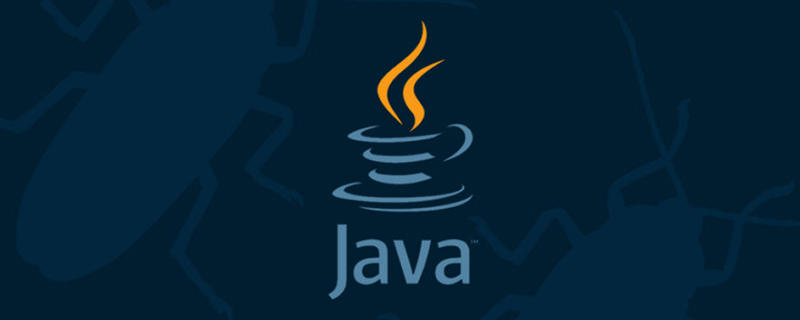
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
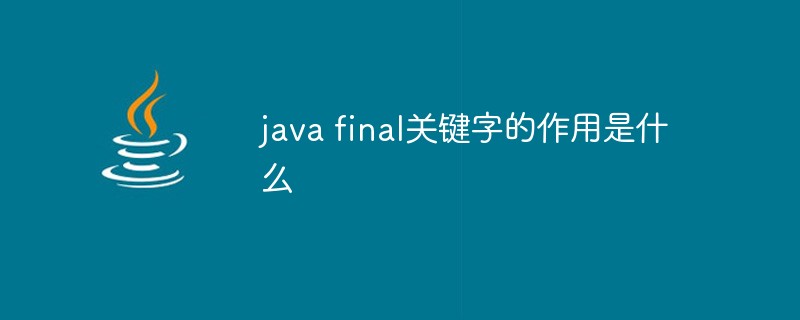
在java中,final可以用来修饰类、方法和变量。final修饰类,表示该类是无法被任何其他类继承的,意味着此类在一个继承树中是一个叶子类,并且此类的设计已被认为很完美而不需要进行修改或扩展。final修饰类中的方法,表示该类是无法被任何其他类继承的,不可以被重写;也就是把该方法锁定了,以防止继承类对其进行更改。final修饰类中的变量,表示该变量一旦被初始化便不可改变。
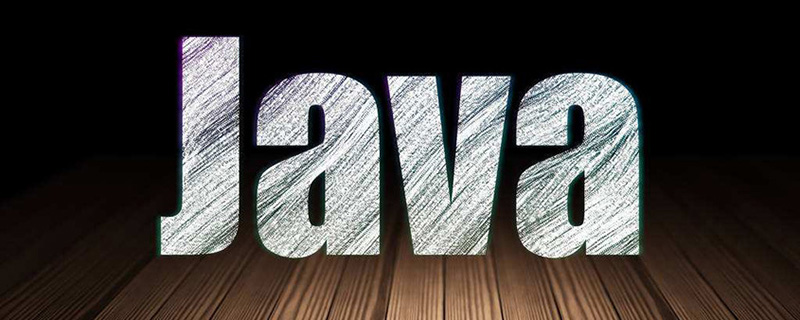
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
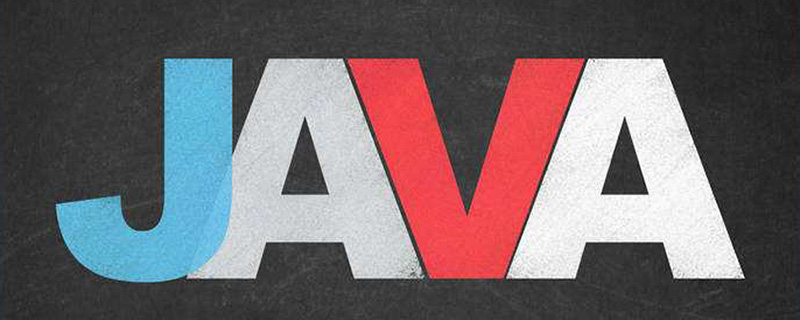
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
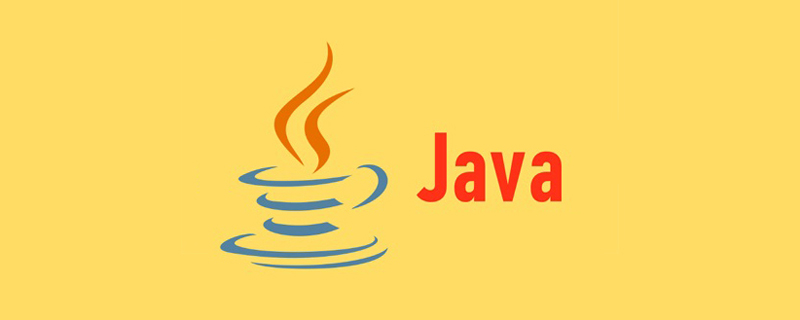
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
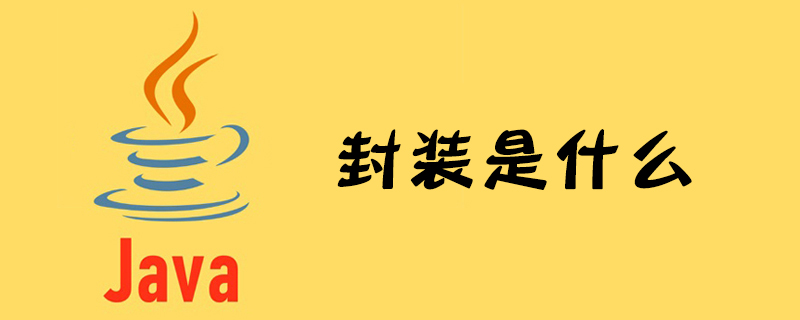
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
