


This article mainly introduces the relevant information of native JS to send asynchronous data requests in detail. It has certain reference value. Interested friends can refer to it
When working on projects, there are Sometimes you need to use asynchronous data requests, but if there is no framework dependency at this time, you need to use native JS for asynchronous data requests. There are only two request methods at this time, one is AJAX and the other is JSONP. Simple encapsulation of asynchronous requests through native JS.
AJAX
AJAX is a data request method that can update the data of a partial page without refreshing the entire page. The technical core of AJAX is the XMLHttpRequest object. The main request process is as follows:
Create XMLHttpRequest object (new)
Connect to the server (open)
Send request(send)
Receive response data(onreadystatechange)
Don’t speak Directly paste the code
/** * 通过JSON的方式请求 * @param {[type]} params [description] * @return {[type]} [description] */ ajaxJSON(params) { params.type = (params.type || 'GET').toUpperCase(); params.data = params.data || {}; var formatedParams = this.formateParams(params.data, params.cache); var xhr; //创建XMLHttpRequest对象 if (window.XMLHttpRequest) { //非IE6 xhr = new XMLHttpRequest(); } else { xhr = new ActiveXObject('Microsoft.XMLHTTP'); } //异步状态发生改变,接收响应数据 xhr.onreadystatechange = function() { if (xhr.readyState == 4 && xhr.status == 200) { if (!!params.success) { if (typeof xhr.responseText == 'string') { params.success(JSON.parse(xhr.responseText)); } else { params.success(xhr.responseText); } } } else { params.error && params.error(status); } } if (params.type == 'GET') { //连接服务器 xhr.open('GET', (!!formatedParams ? params.url + '?' + formatedParams : params.url), true); //发送请求 xhr.send(); } else { //连接服务器 xhr.open('POST', params.url, true); xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded'); //发送请求 xhr.send(formatedParams); } }, /** * 格式化数据 * @param {Obj} data 需要格式化的数据 * @param {Boolean} isCache 是否加入随机参数 * @return {String} 返回的字符串 */ formateParams: function(data, isCache) { var arr = []; for (var name in data) { arr.push(encodeURIComponent(name) + '=' + encodeURIComponent(data[name])); } if (isCache) { arr.push('v=' + (new Date()).getTime()); } return arr.join('&'); }
IE7 and above support native XHR objects, so you can use it directly: var oAjax = new XMLHttpRequest();. In IE6 and previous versions, the XHR object is implemented through an ActiveXObject object in the MSXML library.
Connect to the server through the open function of xhr, which mainly receives three parameters: request method, request address and whether to request asynchronously (usually asynchronous request). There are two request methods, GET and POST. GET submits data to the server through the URL, and POST sends the data to the server as a parameter of the send method.
Bind the status change function onreadystatechange to xhr, which is mainly used to detect changes in the readyState of xhr. When the asynchronous transmission is successful, the value of readyState will change from 0 to 4, and the onreadystatechange event will be triggered at the same time. The attributes and corresponding states of readyState are as follows:
0 (Uninitialized) The object has been created, but it has not yet been initialized (the open method has not been called)
1 (Initialized) The object has been created, but the send method has not been called yet
2 (Send data) The send method has been called, but the current status and http header are unknown
3 (Data is being transmitted) Part of the data has been received, because the response and http header are incomplete. At this time, obtaining part of the data through responseBody and responseText will appear. Error
4 (Complete) Data reception is completed. At this time, the complete response data can be obtained through responseBody and responseText
In the readystatechange event, first determine whether the response has been received, and then determine whether the server The request is processed successfully, xhr.status is status code, status codes starting with 2 are successful, 304 means obtained from the cache, the above code adds a random number to each request, so The value will not be retrieved from the cache, so this status does not need to be judged.
JSONP
If you still use the above XMLHttpRequest object to send cross-domain requests, although the send function is called, the status of xhr is always 0, and The onreadystatechange event will not be triggered. At this time, the JSONP request method will be used.
JSONP (JSON with Padding) is a cross-domain request method. The main principle is to take advantage of the cross-domain request feature of the script tag, send the request to the server through its src attribute, the server returns the js code, the web page accepts the response, and then executes it directly. This is the same as the principle of referencing external files through the script tag. the same.
JSONP consists of two parts: Callback function and data. The callback function is generally controlled by the web page and sent to the server as a parameter. The server combines the function and data. Return as a string.
For example, the web page creates a script tag and assigns its src value to http://www.test.com/json/?callback=process. At this time, the web page Just make a request. The data to be returned by the server is passed in as the parameter of the function. The data format returned by the server is similar to "process({'name:'xieyufei'})". The web page received the response value because the request The one is script, so it is equivalent to calling the process method directly and passing in a parameter.
Post the code directly without saying anything.
/** * 通过JSONP的方式请求 * @param {[type]} params [description] * @return {[type]} [description] */ ajaxJSONP(params) { params.data = params.data || {}; params.jsonp = params.jsonp || 'callback'; // 设置传递给后台的回调参数名和参数值 var callbackName = 'jsonp_' + (new Date()).getTime(); params.data[params.jsonp] = callbackName; var formatedParams = this.formateParams(params.data, params.cache); //创建script标签并插入到页面中 var head = document.getElementsByTagName('head')[0]; var script = document.createElement('script'); head.appendChild(script); //创建jsonp回调函数 window[callbackName] = function(json) { head.removeChild(script); clearTimeout(script.timer); window[callbackName] = null; params.success && params.success(json); }; //发送请求 script.src = (!!formatedParams ? params.url + '?' + formatedParams : params.url); //为了得知此次请求是否成功,设置超时处理 if (params.time) { script.timer = setTimeout(function() { window[callbackName] = null; head.removeChild(script); params.error && params.error({ message: '超时' }); }, params.time); } }
When the src attribute is set to the script tag, the browser will send a request, but it can only send the request once, causing the script tag to not be reused, so each operation is completed You need to remove the script tag. Before the browser sends a request, bind a callback function globally. This callback function will be called when the data request is successful.
Summary
Integrate the two methods of sending asynchronous data and determine which method to use based on dataType. Post the complete code
var xyfAjax = { ajax: function(params) { params = params || {}; params.cache = params.cache || false; if (!params.url) { throw new Error('参数不合法'); } params.dataType = (params.dataType || 'json').toLowerCase(); if (params.dataType == 'jsonp') { this.ajaxJSONP(params); } else if (params.dataType == 'json') { this.ajaxJSON(params); } }, /** * 通过JSONP的方式请求 * @param {[type]} params [description] * @return {[type]} [description] */ ajaxJSONP(params) { params.data = params.data || {}; params.jsonp = params.jsonp || 'callback'; // 设置传递给后台的回调参数名和参数值 var callbackName = 'jsonp_' + (new Date()).getTime(); params.data[params.jsonp] = callbackName; var formatedParams = this.formateParams(params.data, params.cache); //创建script标签并插入到页面中 var head = document.getElementsByTagName('head')[0]; var script = document.createElement('script'); head.appendChild(script); //创建jsonp回调函数 window[callbackName] = function(json) { head.removeChild(script); clearTimeout(script.timer); window[callbackName] = null; params.success && params.success(json); }; //发送请求 script.src = (!!formatedParams ? params.url + '?' + formatedParams : params.url); //为了得知此次请求是否成功,设置超时处理 if (params.time) { script.timer = setTimeout(function() { window[callbackName] = null; head.removeChild(script); params.error && params.error({ message: '超时' }); }, params.time); } }, /** * 通过JSON的方式请求 * @param {[type]} params [description] * @return {[type]} [description] */ ajaxJSON(params) { params.type = (params.type || 'GET').toUpperCase(); params.data = params.data || {}; var formatedParams = this.formateParams(params.data, params.cache); var xhr; //创建XMLHttpRequest对象 if (window.XMLHttpRequest) { //非IE6 xhr = new XMLHttpRequest(); } else { xhr = new ActiveXObject('Microsoft.XMLHTTP'); } //异步状态发生改变,接收响应数据 xhr.onreadystatechange = function() { if (xhr.readyState == 4 && xhr.status == 200) { if (!!params.success) { if (typeof xhr.responseText == 'string') { params.success(JSON.parse(xhr.responseText)); } else { params.success(xhr.responseText); } } } else { params.error && params.error(status); } } if (params.type == 'GET') { //连接服务器 xhr.open('GET', (!!formatedParams ? params.url + '?' + formatedParams : params.url), true); //发送请求 xhr.send(null); } else { //连接服务器 xhr.open('POST', params.url, true); xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded'); //发送请求 xhr.send(formatedParams); } }, /** * 格式化数据 * @param {Obj} data 需要格式化的数据 * @param {Boolean} isCache 是否加入随机参数 * @return {String} 返回的字符串 */ formateParams: function(data, isCache) { var arr = []; for (var name in data) { arr.push(encodeURIComponent(name) + '=' + encodeURIComponent(data[name])); } if (isCache) { arr.push('v=' + (new Date()).getTime()); } return arr.join('&'); } } xyfAjax.ajax({ url:'http://www.xieyufei.com', type:'get', //or post dataType:'json', //or jsonp data:{ name:'xyf' }, success: function(data){ console.log(data) } })
The above is the detailed content of Detailed explanation of native JS sending asynchronous data request examples. For more information, please follow other related articles on the PHP Chinese website!
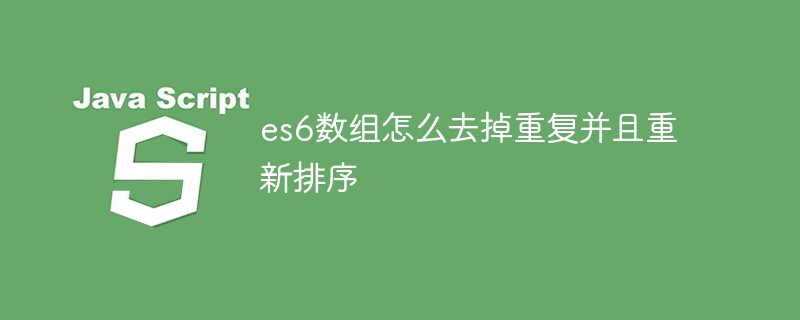
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
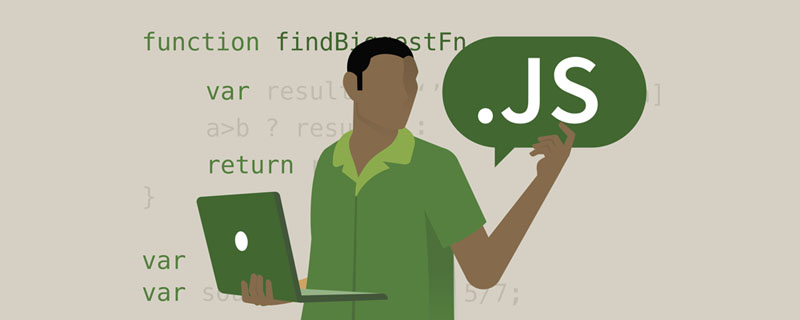
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
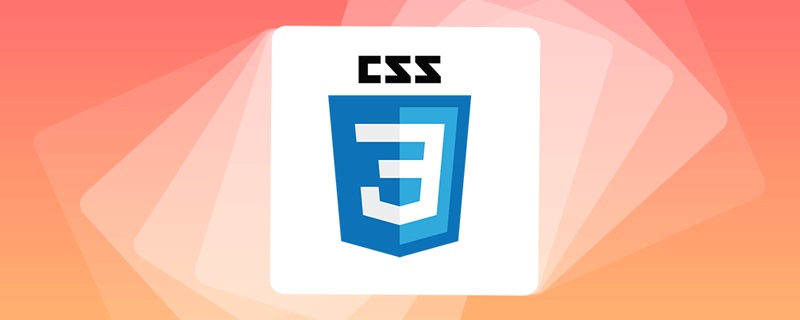
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
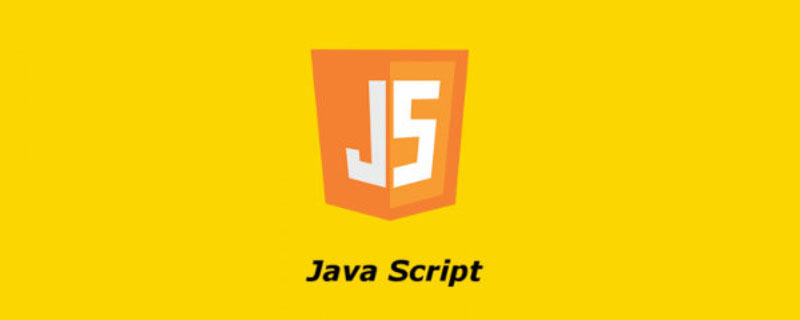
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
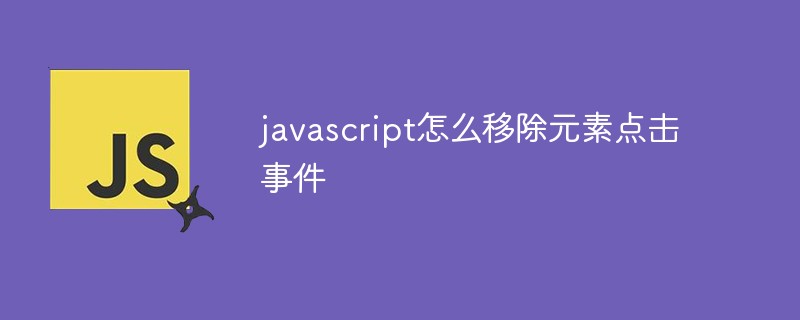
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
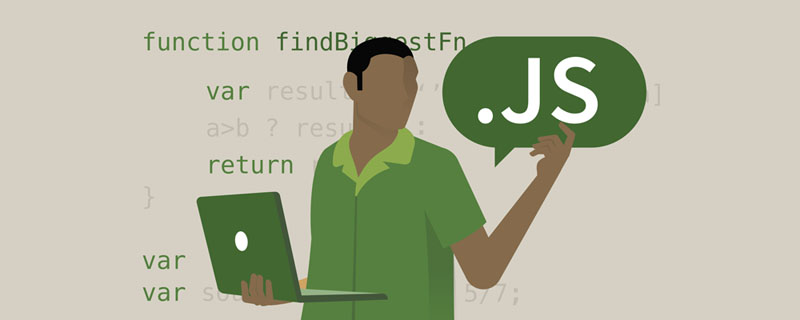
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
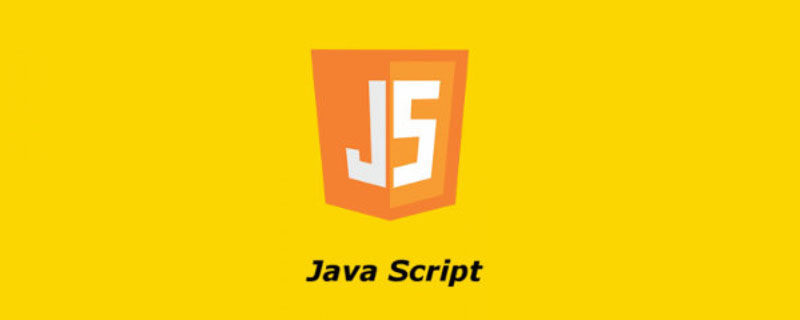
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
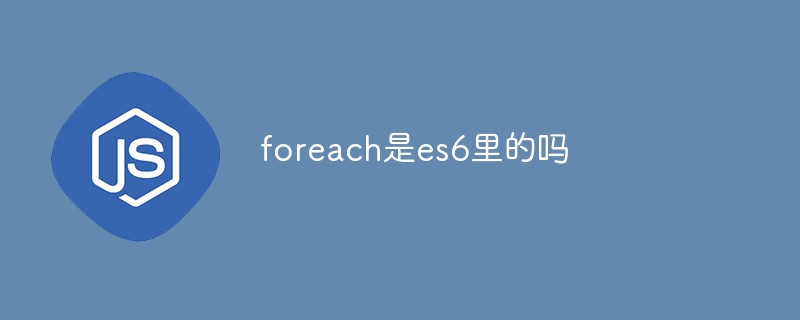
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
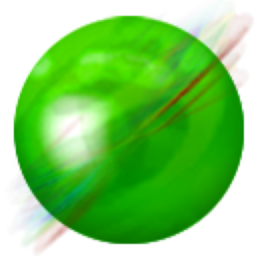
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
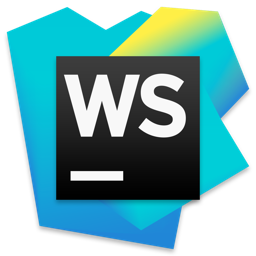
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
