


In the previously introduced attachment management modules, "Winform Development Framework's Universal Attachment Management Module" and "Winform Development Framework's Attachment Management Application" introduce the management functions of attachments. Through the processing of database records and file management, It realizes the integrated management of attachment files and records, and can be used in the stand-alone version of the WInform framework or in the distributed hybrid development framework. As some development scenarios become richer, we need to upload files via FTP, so for This attachment management module is expanded to suit more practical project needs.
1. Implementation ideas for FTP upload and HTTP file preview
The bottom layer of attachment management we envision needs to be reused in Winform, Web and other development projects, so the bottom layer design needs to be considered Corresponding processing can be integrated later using WInform's HTML editing control or Web's HTML editing control. Attachments are unified and implemented in one component.
With the help of FTP file upload, our stand-alone version or the LAN-based Winform interface program can also build a separate FTP server to achieve file sharing; in the distributed hybrid development framework, for file upload , you can choose service-based file system writing, and you can also upload based on FTP.
Upload files based on the FTP method of the hybrid framework. The logical relationship is as follows.
After the file is uploaded to the file system through FTP, we build an HTTP service in the file system, so that the corresponding HTTP address can be used to download the file, and View images and other operations (can be implemented in the HTML editor).
2. Introducing FTP components to implement file upload
Using FTP to upload, although there is an FTPHelper class available in your own public class library, but relatively speaking, I We are more willing to introduce more complete and powerful FTP open source components for related processing. Here we use the FluentFTP component (GitHub address: ), which is a widely used and powerful FTP component.
FluentFTP is an FTP class library based on .Net developed by foreigners that supports FTP and FTPS. FluentFTP is a fully managed FTP client that is designed to be easy to use and easy to expand. It supports file and directory listing, uploading and downloading files and SSL/TLS connections. It can connect to Unix and Windows IIS to establish FTP servers. This project is developed entirely in managed C#.
The usage code of this component is pasted here so that you can have an intuitive understanding of it.
// create an FTP clientFtpClient client = new FtpClient("123.123.123.123");// if you don't specify login credentials, we use the "anonymous" user accountclient.Credentials = new NetworkCredential("david", "pass123");// begin connecting to the serverclient.Connect();// get a list of files and directories in the "/htdocs" folderforeach (FtpListItem item in client.GetListing("/htdocs")) { // if this is a fileif (item.Type == FtpFileSystemObjectType.File){ // get the file sizelong size = client.GetFileSize(item.FullName); } // get modified date/time of the file or folderDateTime time = client.GetModifiedTime(item.FullName); // calculate a hash for the file on the server side (default algorithm)FtpHash hash = client.GetHash(item.FullName); }// upload a fileclient.UploadFile(@"C:\MyVideo.mp4", "/htdocs/big.txt");// rename the uploaded fileclient.Rename("/htdocs/big.txt", "/htdocs/big2.txt");// download the file againclient.DownloadFile(@"C:\MyVideo_2.mp4", "/htdocs/big2.txt");// delete the fileclient.DeleteFile("/htdocs/big2.txt");// delete a folder recursivelyclient.DeleteDirectory("/htdocs/extras/");// check if a file existsif (client.FileExists("/htdocs/big2.txt")){ }// check if a folder existsif (client.DirectoryExists("/htdocs/extras/")){ }// upload a file and retry 3 times before giving upclient.RetryAttempts = 3; client.UploadFile(@"C:\MyVideo.mp4", "/htdocs/big.txt", FtpExists.Overwrite, false, FtpVerify.Retry);// disconnect! good bye!client.Disconnect();
With this understanding, in ordinary Winform programs or hybrid framework programs, we can load this information in the code by configuring the specified FTP related information. Perform FTP login, file upload, download and other operations.
3. Implementation of Attachment Management Module
With the above ideas and the assistance of components, we can implement FTP by performing relevant upgrades on the original attachment management module. Upload mode is handled.
First of all, for convenience, we first define a configuration entity class to obtain FTP server, user name, password and other parameters, as shown below.
/// <summary>/// FTP配置信息/// </summary> [DataContract] [Serializable]public class FTPInfo {/// <summary>/// 默认构造函数/// </summary>public FTPInfo() { }/// <summary>/// 参数化构造函数/// </summary>/// <param>/// <param>/// <param>public FTPInfo(string server, string user, string password, string baseUrl) {this.Server = server;this.User = user;this.Password = password;this.BaseUrl = baseUrl; }/// <summary>/// FTP服务地址/// </summary> [DataMember]public string Server { get; set; }/// <summary>/// FTP用户名/// </summary> [DataMember]public string User { get; set; }/// <summary>/// FTP密码/// </summary> [DataMember]public string Password { get; set; }/// <summary>/// FTP的基础路径,如可以指定为IIS的路径::8000 ,方便下载打开/// </summary> [DataMember]public string BaseUrl { get; set; } }
Define a function specifically used to extract the relevant FTP parameters in the configuration file, as shown below.
/// <summary>/// 获取配置的FTP配置参数/// </summary>/// <returns></returns>private FTPInfo GetFTPConfig() {var ftp_server = config.AppConfigGet("ftp_server");var ftp_user = config.AppConfigGet("ftp_user");var ftp_pass = config.AppConfigGet("ftp_password");var ftp_baseurl = config.AppConfigGet("ftp_baseurl");return new FTPInfo(ftp_server, ftp_user, ftp_pass, ftp_baseurl); }
Our configuration file is as follows.
The component code using FluentFTP is as follows.
//使用FluentFTP操作FTP文件FtpClient client = new FtpClient(ftpInfo.Server, ftpInfo.User, ftpInfo.Password);
Then call the FTP component to determine the directory, if not, just create one.
//确定日期时间目录(格式:yyyy-MM),不存在则创建string savePath = string.Format("/{0}-{1:D2}/{2}", DateTime.Now.Year, DateTime.Now.Month, category);bool isExistDir = client.DirectoryExists(savePath);if(!isExistDir) { client.CreateDirectory(savePath); }
Finally, use the component to upload the file. Upload the file here. Since the byte array is stored in the previous FileUploadInfo entity class, you can also use the FTP component to directly upload the byte array. .
//使用FTP上传文件//避免文件重复,使用GUID命名var ext = FileUtil.GetExtension(info.FileName);var newFileName = string.Format("{0}{1}", Guid.NewGuid().ToString(), ext);//FileUtil.GetFileName(file);savePath = savePath.UriCombine(newFileName);bool uploaded = client.Upload(info.FileData, savePath, FtpExists.Overwrite, true);
After the file is uploaded to the file server, all that is left is to store the relevant information in the data table of the attachment management module. In this way, the information in the database can be directly used when using it. If you need to view pictures or Download the file and then splice the relevant HTTP addresses. Let’s take a look at the corresponding database record screenshot as shown below.
With this basic information, we can also transform the Winform HTML editing control I introduced before: ZetaHtmlEditControl (share an HTML editing control Zeta HTML Edit in Winform Control (Chinese version with source code), I translated all the English menus, toolbars, dialog boxes, prompt content and other resources of this control into Chinese, and added the functions of inserting pictures and printing in the toolbar, and the interface is as follows.
By default, the way we add pictures is definitely based on local files; but after we modified it to use FTP to upload files, we got HTTP on the control Address, you can preview and display the image file.
The image address constructed by this method is a standard URL address and can be viewed in various places, as shown in the following interface.
This is the ZetaHtmlEditControl control. It integrates the attachment management module that we have completed in the FTP upload mode earlier to realize the function of editing online HTML. Such HTML content can also be suitable for It is displayed in the HTML editor under the web interface.
The above are the project components I constructed for the entire WInform development framework. I added the FTP upload method and improved the corresponding scene requirements. I implemented the function of editing online HTML on the ZetaHtmlEditControl control. I hope the development ideas will be useful to you. Some gain.
The above is the detailed content of How to add support for FTP upload and preview in the attachment management module?. For more information, please follow other related articles on the PHP Chinese website!
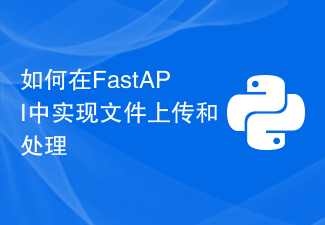
如何在FastAPI中实现文件上传和处理FastAPI是一个现代化的高性能Web框架,简单易用且功能强大,它提供了原生支持文件上传和处理的功能。在本文中,我们将学习如何在FastAPI框架中实现文件上传和处理的功能,并提供代码示例来说明具体的实现步骤。首先,我们需要导入需要的库和模块:fromfastapiimportFastAPI,UploadF
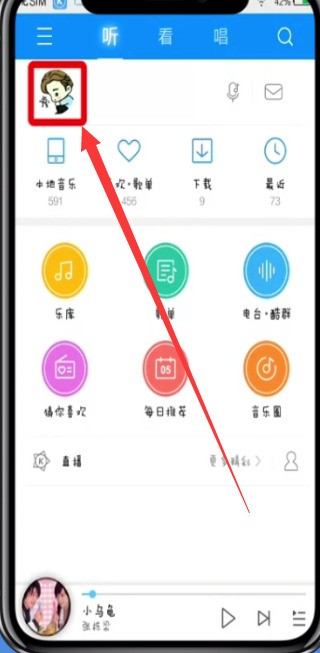
1、打开酷狗音乐,点击个人头像。2、点击右上角设置的图标。3、点击【上传音乐作品】。4、点击【上传作品】。5、选择歌曲,然后点击【下一步】。6、最后点击【上传】即可。
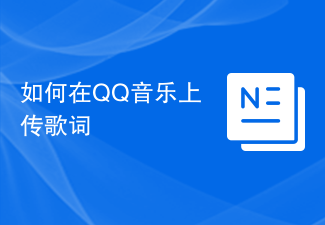
随着数字化时代的到来,音乐平台成为人们获取音乐的主要途径之一。然而,有时候我们在听歌的时候,发现没有歌词是一件十分困扰的事情。很多人都希望在听歌的时候能够显示歌词,以便更好地理解歌曲的内容和情感。而QQ音乐作为国内最大的音乐平台之一,也为用户提供了上传歌词的功能,使得用户可以更好地享受音乐的同时,感受到歌曲的内涵。下面将介绍一下在QQ音乐上如何上传歌词。首先
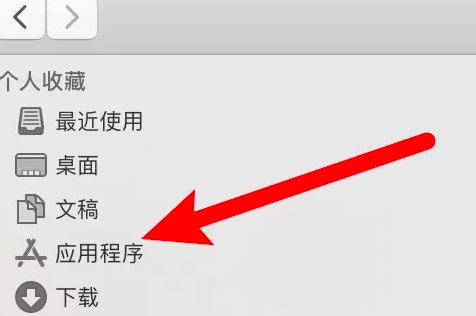
电脑只要安装了摄像头就可以进行拍照,但是有些用户还不知道该怎么拍照上传,现在就给大家具体介绍一下电脑拍照的方法,这样用户得到图片之后想上传到哪里都可以了。电脑怎么拍照上传一、Mac电脑1、打开访达,再点击左边的应用程序。2、打开后点击相机应用。3、点击下方的拍照按钮就可以了。二、Windows电脑1、打开下方搜索框,输入相机。2、接着打开搜索到的应用。3、再点击旁边的拍照按钮就可以了。
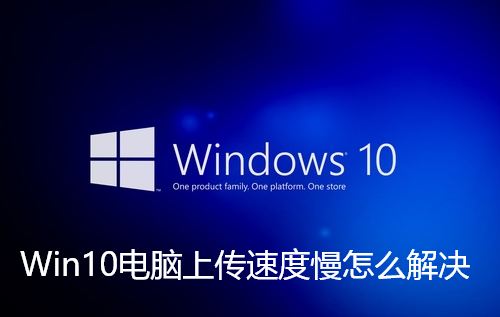
Win10电脑上传速度慢怎么解决?我们在使用电脑的时候可能会觉得自己电脑上传文件的速度非常的慢,那么这是什么情况呢?其实这是因为电脑默认的上传速度为20%,所以才导致上传速度非常慢,很多小伙伴不知道怎么详细操作,小编下面整理了win11格式化c盘操作步骤,如果你感兴趣的话,跟着小编一起往下看看吧! Win10上传速度慢的解决方法 1、按下win+R调出运行,输入gpedit.msc,回车。 2、选择管理模板,点击网络--Qos数据包计划程序,双击限制可保留带宽。 3、选择已启用,将带
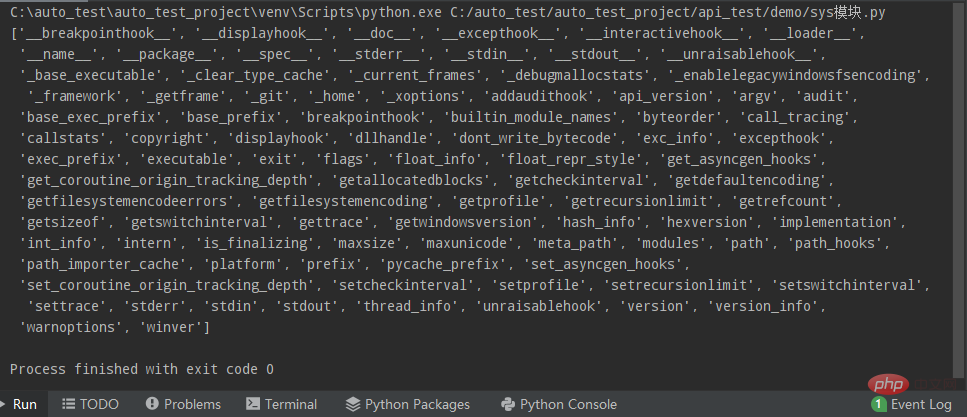
一、sys模块简介前面介绍的os模块主要面向操作系统,而本篇的sys模块则主要针对的是Python解释器。sys模块是Python自带的模块,它是与Python解释器交互的一个接口。sys 模块提供了许多函数和变量来处理 Python 运行时环境的不同部分。二、sys模块常用方法通过dir()方法可以查看sys模块中带有哪些方法:import sys print(dir(sys))1.sys.argv-获取命令行参数sys.argv作用是实现从程序外部向程序传递参数,它能够获取命令行参数列
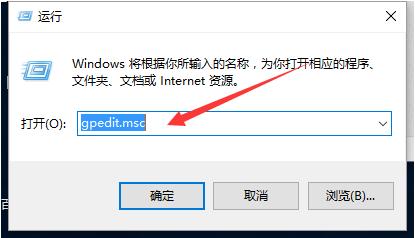
上传速度变得非常慢?相信这是很多朋友用电脑上传东西时候都会遇到的一个问题,在使用电脑传送文件的时候如果遇到网络不稳定,上传的速度就会很慢,那么应该怎么提高网络上传速度呢?下面,小编将电脑上传速度慢的处理方法告诉大家。说到网络速度,我们都知道打开网页的速度,下载速度,其实还有一个上传速度也非常关键,特别是一些用户经常需要上传文件到网盘的,那么上传速度快无疑会给你省下不少时间,那么上传速度慢怎么办?下面,小编给大伙带来了电脑上传速度慢的处理图文。电脑上传速度慢怎么解决点击“开始--运行”或者“窗口键
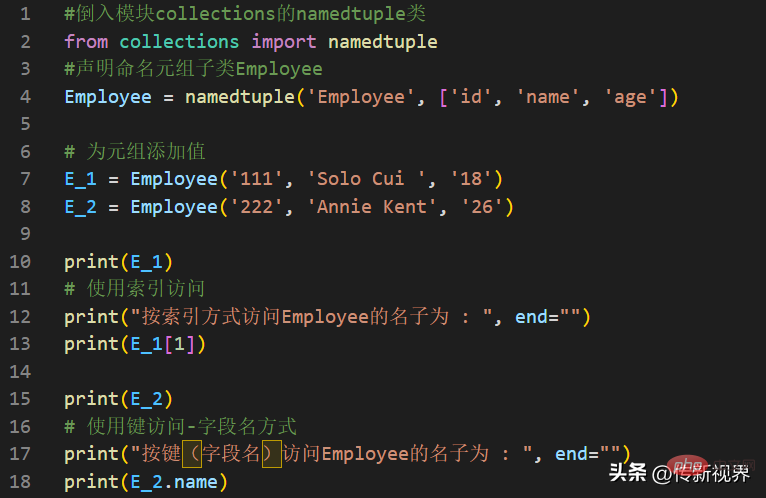
前言本文继续来介绍Python集合模块,这次主要简明扼要的介绍其内的命名元组,即namedtuple的使用。闲话少叙,我们开始——记得点赞、关注和转发哦~ ^_^创建命名元组Python集合中的命名元组类namedTuples为元组中的每个位置赋予意义,并增强代码的可读性和描述性。它们可以在任何使用常规元组的地方使用,且增加了通过名称而不是位置索引方式访问字段的能力。其来自Python内置模块collections。其使用的常规语法方式为:import collections XxNamedT


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
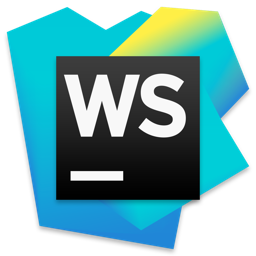
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
