The following editor will bring you a detailed explanation of log rotate in golang. The editor thinks it is quite good, so I will share it with you now and give it as a reference for everyone. Let’s follow the editor and take a look
Operating system: CentOS 6.9_x64
go language version: 1.8.3
Problem description
The log module of golang provides the log writing function. The sample code is as follows:
/* golang log example */ package main import ( "log" "os" ) func main() { logFile,err := os.Create("test1.log") defer logFile.Close() if err != nil { log.Fatalln("open file error!") } logger := log.New(logFile,"[Debug]",log.Ldate | log.Ltime | log.Lshortfile) logger.Println("test debug message") logger.SetPrefix("[Info]") logger.Println("test info message") }
Operating effect:
[root@local t2]# go build logTest1.go [root@local t2]# ./logTest1 [root@local t2]# cat test1.log [Debug]2017/06/13 23:18:36 logTest1.go:19: test debug message [Info]2017/06/13 23:18:36 logTest1.go:21: test info message [root@local t2]#
The log module of the go language does not provide log rotate interface, but in actual development we need this function:
We don’t want a single log to be too large, otherwise the text editor cannot open it and it will be difficult to view;
We also don’t want to occupy too much storage Space, you can specify the maximum number of log files to store.
Solution
Achieved with the help of buffered channel.
The sample code is as follows:
/* golang log rotate example */ package main import ( "fmt" "log" "os" "time" ) const ( BACKUP_COUNT = 5 MAX_FILE_BYTES = 2 * 1024 ) func doRotate(fPrefix string) { for j := BACKUP_COUNT; j >= 1; j-- { curFileName := fmt.Sprintf("%s_%d.log",fPrefix,j) k := j-1 preFileName := fmt.Sprintf("%s_%d.log",fPrefix,k) if k == 0 { preFileName = fmt.Sprintf("%s.log", fPrefix) } _,err := os.Stat(curFileName) if err == nil { os.Remove(curFileName) fmt.Println("remove : ", curFileName) } _,err = os.Stat(preFileName) if err == nil { fmt.Println("rename : ", preFileName, " => ", curFileName) err = os.Rename(preFileName, curFileName) if err != nil { fmt.Println(err) } } } } func NewLogger(fPrefix string) (*log.Logger, *os.File) { var logger *log.Logger fileName := fmt.Sprintf("%s.log", fPrefix) fmt.Println("fileName :", fileName) logFile, err := os.OpenFile(fileName, os.O_RDWR|os.O_CREATE|os.O_APPEND, 0666) if err != nil { fmt.Println("open file error!") } else { logger = log.New(logFile, "[Debug]", log.Ldate|log.Ltime|log.Lshortfile) } return logger, logFile } func logWorker(msgQueue <-chan string) { fPrefix := "msg" logger, logFile := NewLogger(fPrefix) for msg := range msgQueue { logger.Println(msg) fi, err2 := logFile.Stat() if err2 == nil { if fi.Size() > MAX_FILE_BYTES { logFile.Close() doRotate(fPrefix) logger,logFile = NewLogger(fPrefix) } } } logFile.Close() } func main() { msgQueue := make(chan string, 1000) go logWorker(msgQueue) for j := 1; j <= 1000; j++ { msgQueue <- fmt.Sprintf("msg_%d", j) time.Sleep(1 * time.Second) } close(msgQueue) return }
The running effect is as follows:
[root@local t2]# ./logRotateTest1 fileName : msg.log rename : msg.log => msg_1.log fileName : msg.log rename : msg_1.log => msg_2.log rename : msg.log => msg_1.log fileName : msg.log rename : msg_2.log => msg_3.log rename : msg_1.log => msg_2.log rename : msg.log => msg_1.log fileName : msg.log ^C
Discussion
This is just a simple sample code that implements log rotate. More functions need to be developed by yourself.
Okay, that’s all, I hope it helps.
The above is the detailed content of Detailed introduction to the log module in golang. For more information, please follow other related articles on the PHP Chinese website!
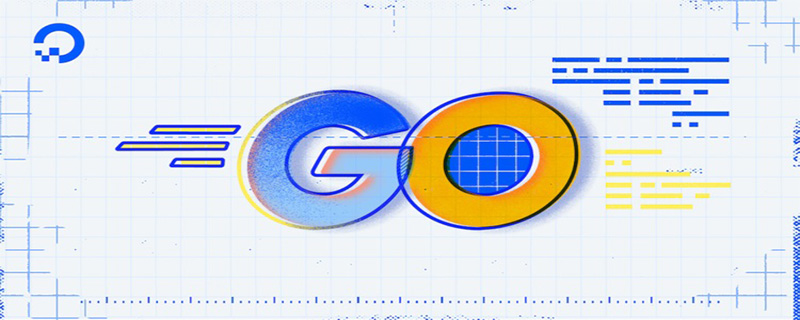
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
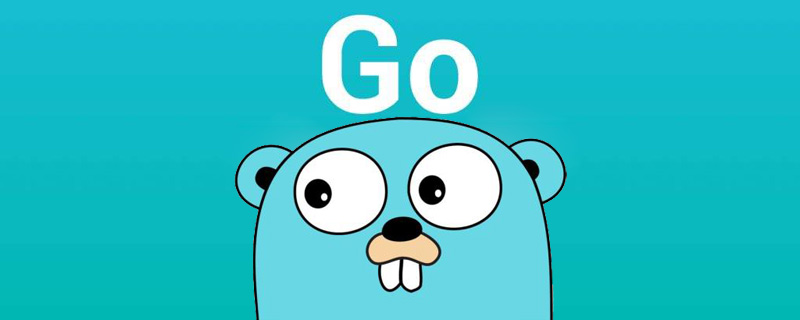
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
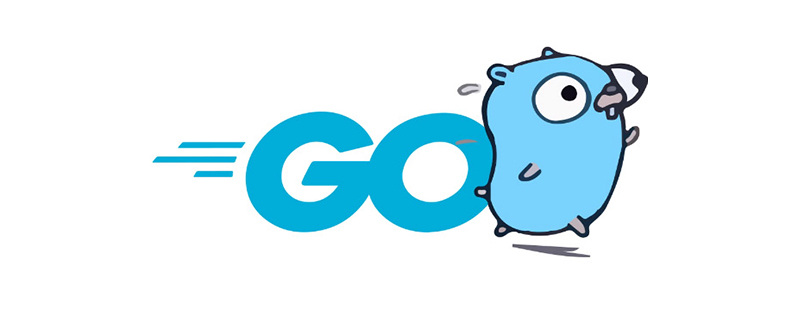
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
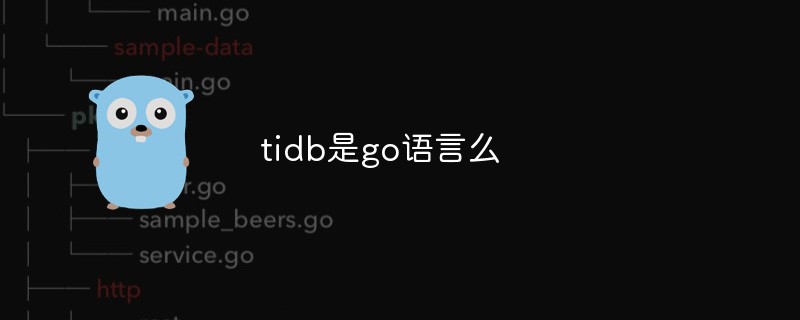
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
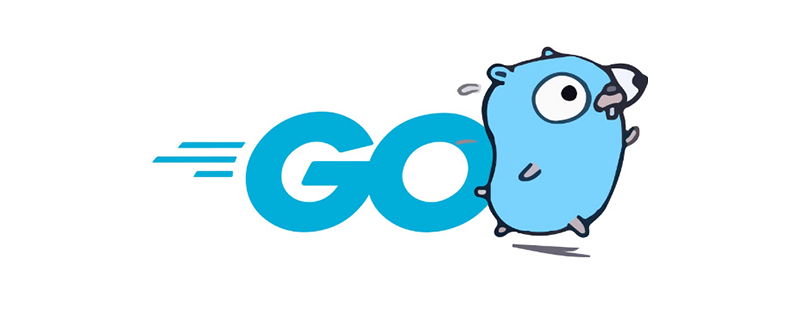
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
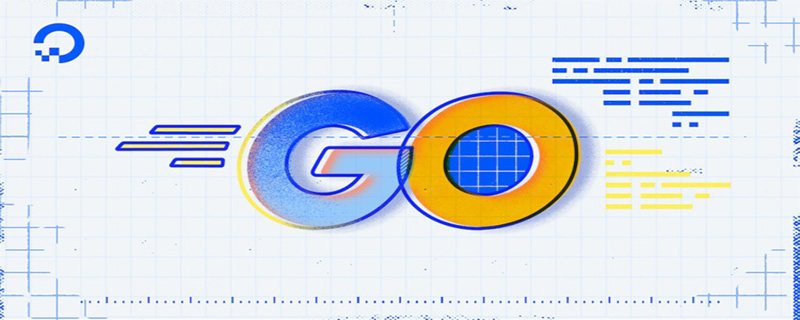
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
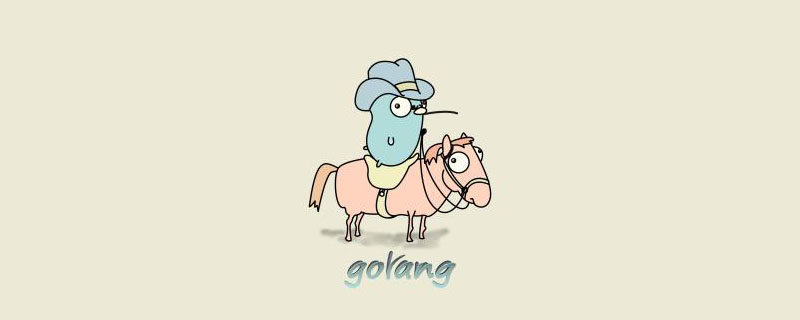
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
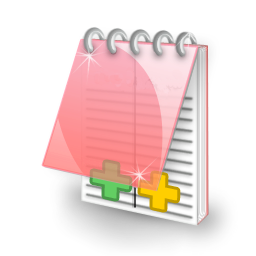
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
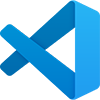
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
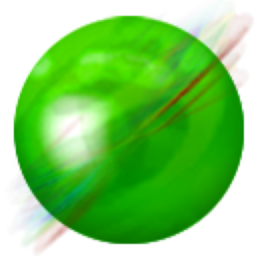
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
