


This article mainly introduces the relevant information of PHP Dependency Injection (DI) and Inversion of Control (IoC), which has certain reference value. Interested friends can refer to it
First Dependency Injection It talks about the same thing as inversion of control. It is a design pattern. This design pattern is used to reduce the coupling between programs. I studied it for a while and saw that there is no related article on the TP official website, so I wrote this introduction. Let’s take a look at this design pattern, hoping to contribute some strength to the TP community.
First of all, don’t pursue the definition of this design pattern, otherwise you will definitely be confused. The author is deeply affected by it. There are many articles on Baidu, all of which are described from a theoretical perspective. There are a lot of unfamiliar vocabulary, or it is described by java code, which is also unfamiliar.
No matter what, I finally have some clarity. Let’s describe the concept of dependency injection from the perspective of PHP.
Let’s first assume that we have a class here, which needs to use a database connection. According to the most primitive method, we may write this class like this:
class example { private $_db; function __construct(){ include "./Lib/Db.php"; $this->_db = new Db("localhost","root","123456","test"); } function getList(){ $this->_db->query("......");//这里具体sql语句就省略不写了 } }
Process:
Include the database class file in the constructor first;
Then pass in new Db and pass in the database connection information Instantiate the db class;
After that, the getList method can call the database class through $this->_db to implement database operations.
It seems that we have achieved the desired function, but this is the beginning of a nightmare. In the future, example1, example2, example3... more and more classes will need to use the db component. If they are all written like this If so, if one day the database password is changed or the db class changes, wouldn't it be necessary to go back and modify all class files?
ok, in order to solve this problem, the factory mode appeared. We created a Factory method and obtained the instance of the db component through the Factory::getDb() method:
class Factory { public static function getDb(){ include "./Lib/Db.php"; return new Db("localhost","root","123456","test"); } }
The sample class becomes:
class example { private $_db; function __construct(){ $this->_db = Factory::getDb(); } function getList(){ $this->_db->query("......");//这里具体sql语句就省略不写了 } }
Is this perfect? Think again about all classes in the future, example1, example2, example3..., you need to obtain a Db instance through Factory::getDb(); in the constructor. In fact, you directly interact with the Db class from the original The coupling becomes coupling with the Factory class. The factory class just helps you package the database connection information. Although when the database information changes, you only need to modify the Factory::getDb() method, but suddenly one day the factory method The name needs to be changed, or the getDb method needs to be renamed, what should you do? Of course, this kind of demand is actually very messed up, but sometimes this situation does exist. One solution is:
We don’t instantiate the Db component from within the example class. We rely on injection from the outside. What? What does it mean? Look at the following example:
class example { private $_db; function getList(){ $this->_db->query("......");//这里具体sql语句就省略不写了 } //从外部注入db连接 function setDb($connection){ $this->_db = $connection; } } //调用 $example = new example(); $example->setDb(Factory::getDb());//注入db连接 $example->getList();
In this way, the example class is completely decoupled from the external class. You can see that there are no factory methods or methods in the Db class. Db class figure. We inject the connection instance directly into it by calling the setDb method of the example class from the outside. In this way, the example does not need to worry about how the db connection is generated.
This is called dependency injection. The implementation does not create a dependency relationship within the code, but passes it as a parameter. This makes our program easier to maintain, reduces the coupling of the program code, and achieves a loose coupling.
This is not over yet. Let us assume that in addition to db, other external classes are used in the example class. We pass:
$example->setDb(Factory::getDb());//注入db连接 $example->setFile(Factory::getFile());//注入文件处理类 $example->setImage(Factory::getImage());//注入Image处理类 ...
We are not done yet. No need to write so many sets? Are you tired?
ok, in order to not have to write so many lines of code every time, we have another factory method:
class Factory { public static function getExample(){ $example = new example(); $example->setDb(Factory::getDb());//注入db连接 $example->setFile(Factory::getFile());//注入文件处理类 $example->setImage(Factory::getImage());//注入Image处理类 return $expample; } }
Instantiate example When it becomes:
$example=Factory::getExample(); $example->getList();
It seems perfect, but why does it feel like it’s back to the scene when the factory method was used for the first time? This is indeed not a good solution, so another concept is proposed: containers, also called IoC containers and DI containers.
We originally injected various classes through the setXXX method. The code is very long and there are many methods. Although it can be packaged through a factory method, it is not so cool. Well, we don’t use the setXXX method, so that’s it. Without secondary packaging with factory methods, how can we implement dependency injection?
Here we introduce a convention: pass in a parameter named Di $di in the constructor of the example class, as follows:
class example { private $_di; function __construct(Di &$di){ $this->_di = $di; } //通过di容器获取db实例 function getList(){ $this->_di->get('db')->query("......");//这里具体sql语句就省略不写了 } } $di = new Di(); $di->set("db",function(){ return new Db("localhost","root","root","test"); }); $example = new example($di); $example->getList();
Di is IoC container, the so-called container is to store instances of various classes that we may use. We set an instance named db through $di->set(). Because it is passed in through a callback function, set The db class will not be instantiated immediately, but will be instantiated when $di->get('db'). Similarly, the singleton mode can also be integrated into the design of the di class.
In this way, we only need to declare a Di class in the global scope, put all the classes that need to be injected into the container, and then pass the container into example as a parameter of the constructor, and then in the example class Get the instance from the container. Of course, it doesn’t have to be a constructor. You can also use a setDi(Di $di) method to pass in the Di container. In short, the agreement is made by you, so you just need to know it yourself.
In this way, dependency injection and key container concepts have been introduced, and the rest is to use and understand it in practice!
The above is the detailed content of Share practical tutorials on PHP Dependency Injection (DI) and Inversion of Control (IoC). For more information, please follow other related articles on the PHP Chinese website!
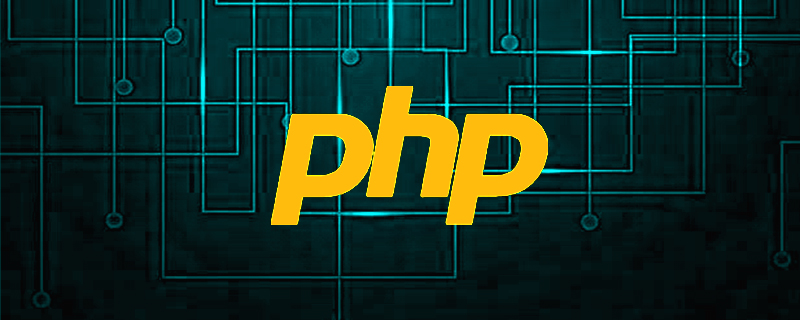
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
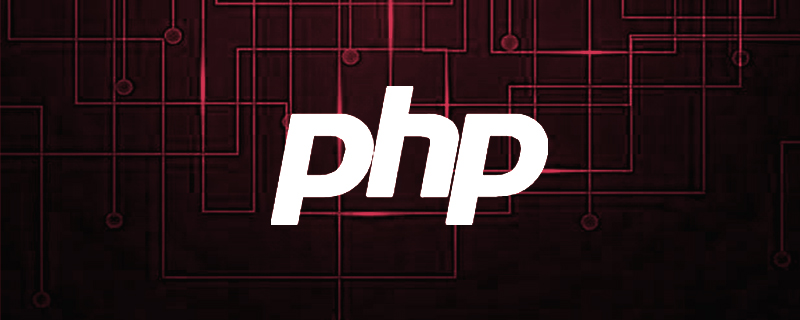
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
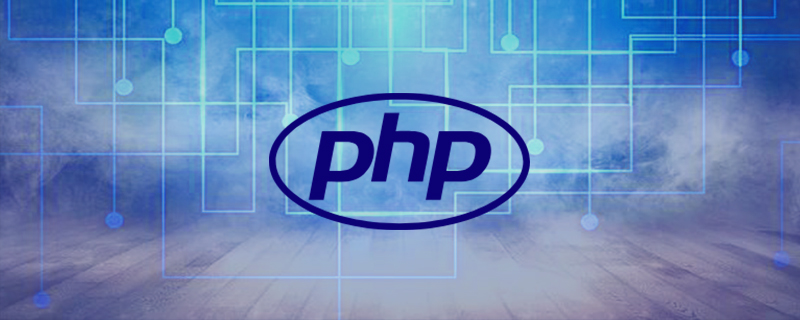
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
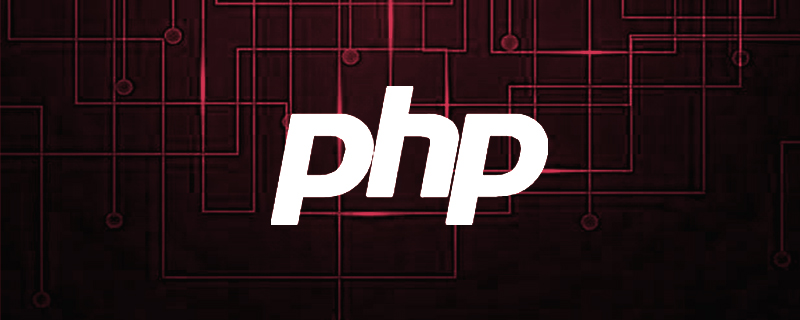
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
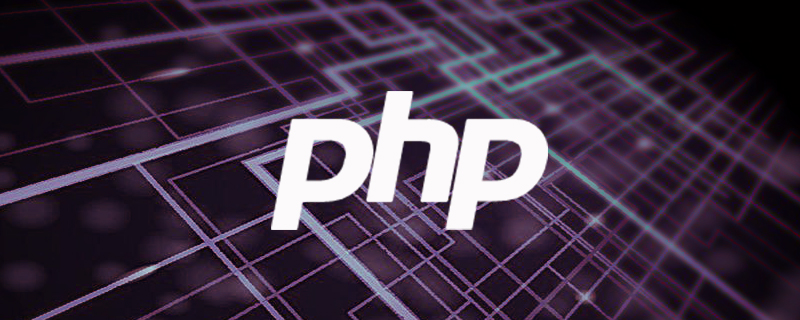
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
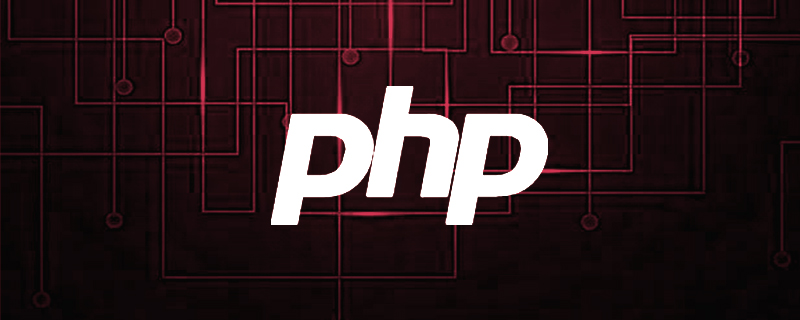
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
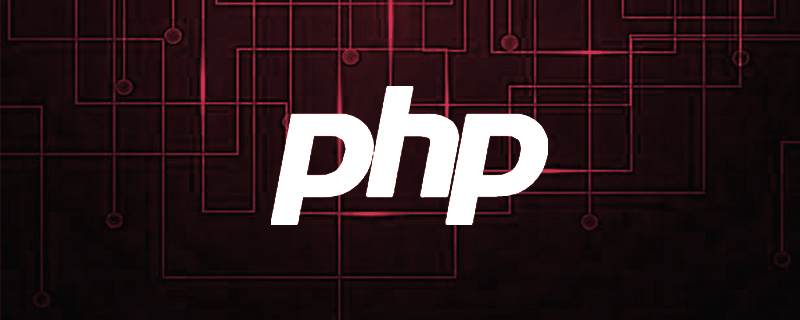
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
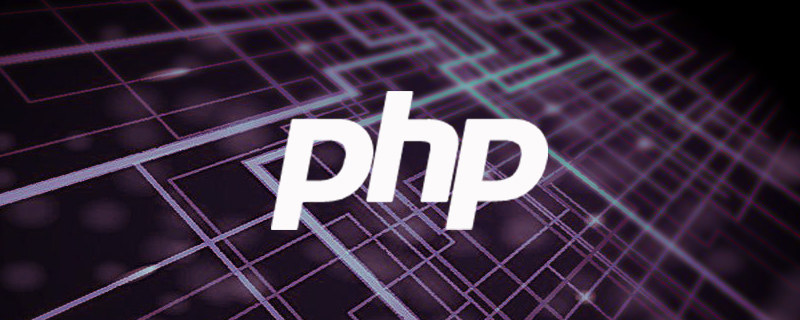
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
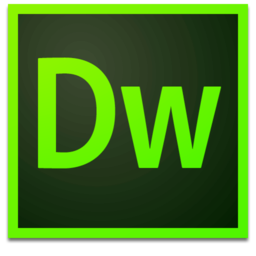
Dreamweaver Mac version
Visual web development tools
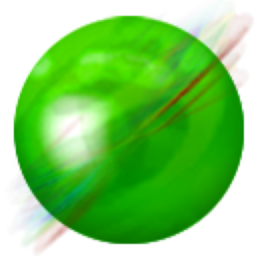
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
