


This article mainly introduces the example code of Javascript to implement anonymous recursion, and uses arguments.callee to implement anonymous recursion. Those who are interested can learn about
Recursion is a common programming technique. I believe everyone is familiar with real-name recursion, but what if you want to implement anonymous recursion? For example, if you want to return an anonymous recursive function, or define an anonymous recursive function and call it directly, how should you do it? This article will explore its implementation.
Real-name recursion
Let’s start with real-name recursion, or use the simplest example of finding factorial:
function fact(n) { if (n < 2) { return n; } else { return n * fact(n - 1); } } console.log(fact(5));
Recursion requirements Call yourself, which is too easy if the function has a name.
Use variables to achieve recursion
The function can also be assigned to a variable, but to achieve recursion, the function body still depends on thisVariable name:
var f = function(n) { if (n < 2) { return n; } else { return n * f(n - 1); } } console.log(f(5));
It should be said that this method is not essentially different from the previous one.
Anonymous Recursion
Now let’s discuss the implementation of anonymous recursion.
Initial idea
If you want to return an anonymous recursive function, or define an anonymous recursive function and call it directly:
(function (n) { if (n < 2) { return n; } else { return n * ?(n - 1); } })(5);
If Without a name, we don't know what to fill in the question mark in the code, and we can't form recursion. What should we do at this time? At this time, it is necessary to ask for arguments object.
arguments object
In a javascript function, the arguments object represents the parameter object when actually called. In our recursive function, we actually don’t need to define the “formal parameters” n at all:
function factNoParam() { if (arguments[0] < 2) { return arguments[0]; } else { return arguments[0] * factNoParam(arguments[0] - 1); } } console.log(factNoParam(5));
As long as we pass in the actual parameters when calling, we can use arguments[0] to get the actual parameters The value of this parameter passed in.
If there are more parameters, they can also be obtained by arguments[1], arguments[2], etc.
arguments.callee Attributes
arguments can be used to obtain parameters. I believe you may already know this, but the arguments object actually has There is a property called callee. arguments.callee represents the function itself. What does it mean? In fact, we can write fact like this:
function fact(n) { if (n < 2) { return n; } else { return n * arguments.callee(n - 1); } } console.log(fact(5));
Then it is still recursive. Because arguments.callee is actually equal to fact.
So, here we are, with the help of this attribute, it is not difficult to implement anonymous recursion, just change ? to arguments.callee:
(function (n) { if (n < 2) { return n; } else { return n * arguments.callee(n - 1); } })(5);
If necessary, also This can be returned as anonymous recursion.
The above is the detailed content of Detailed explanation of code cases for anonymous recursive implementation in Javascript. For more information, please follow other related articles on the PHP Chinese website!
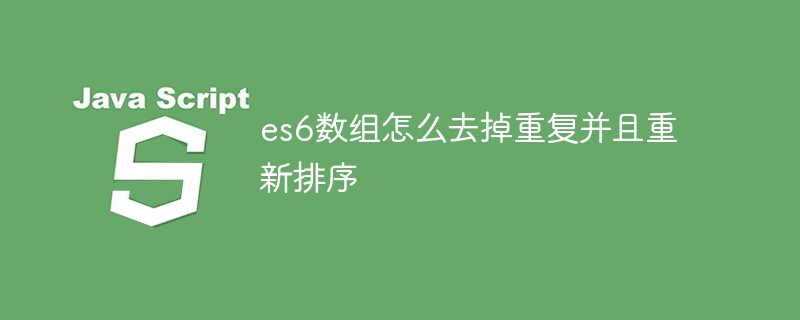
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
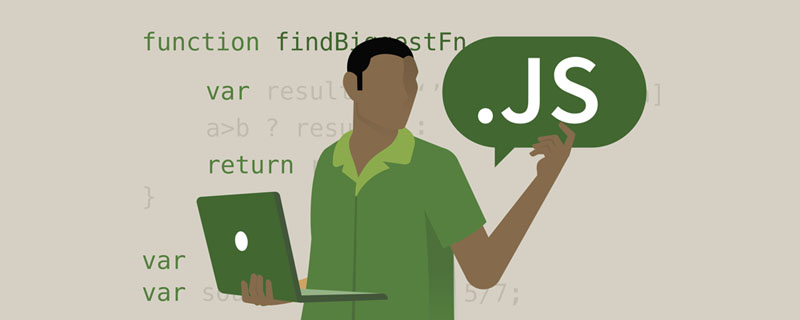
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
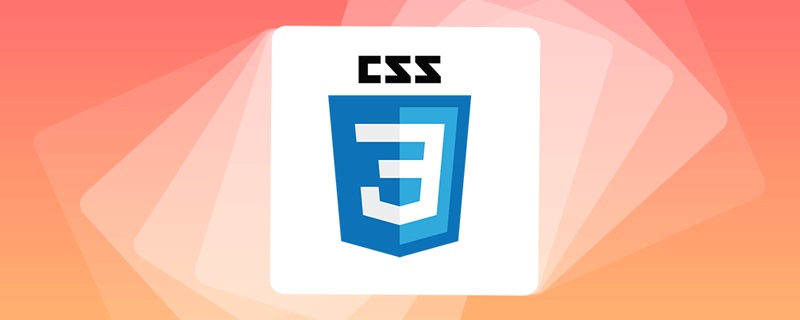
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
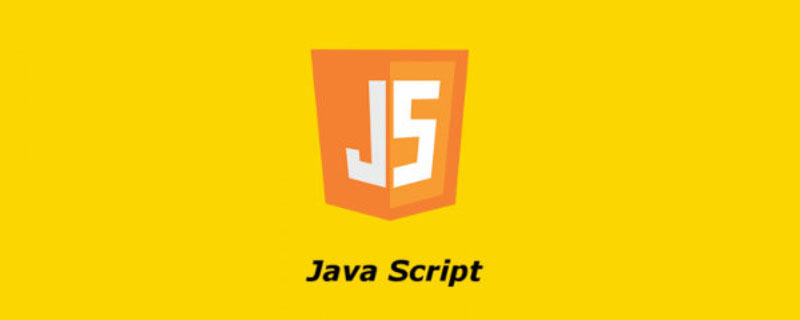
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
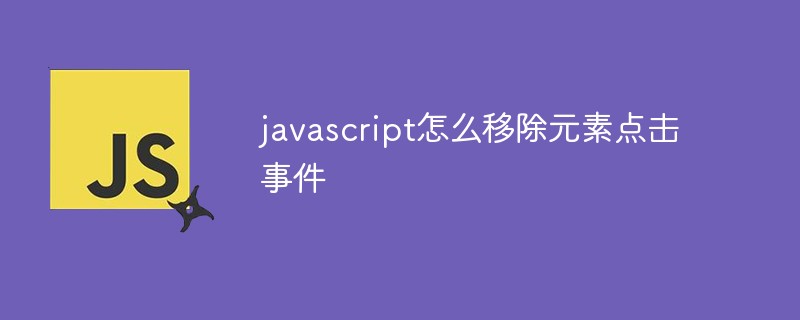
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
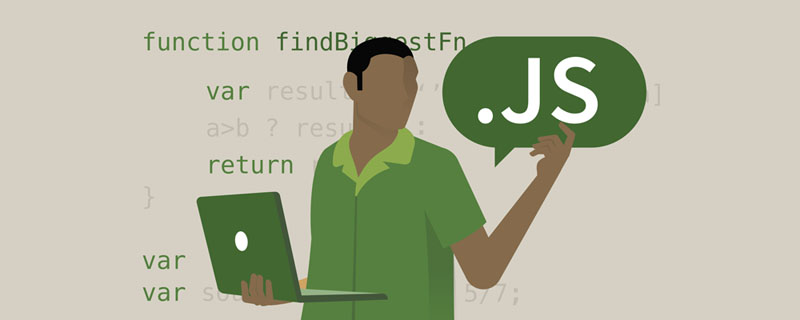
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
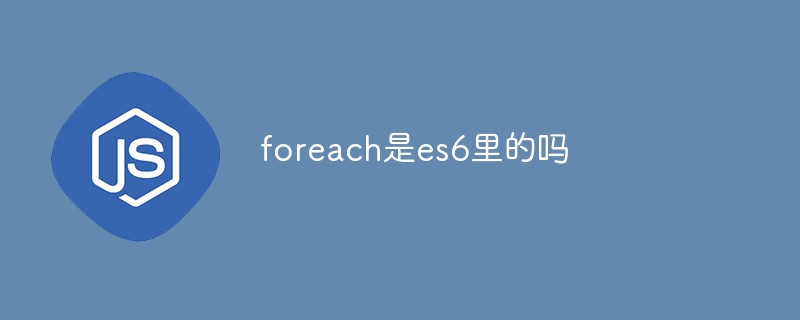
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。
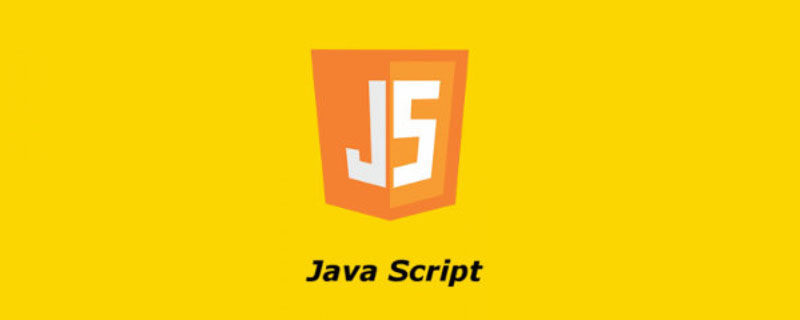
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
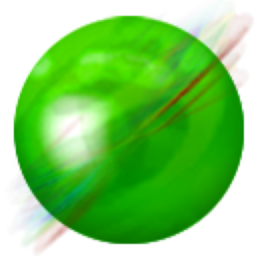
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor
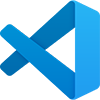
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.