This article mainly introduces some commonly used Spring Bean extension interfaces and their simple usage, which has a good reference value. Let’s take a look at it with the editor
Preface
Spring is a very powerful framework. It can be said that almost all enterprise-level Java projects use Spring. , and Bean is the core of the Spring framework.
Spring framework uses a lot of design patterns. On the whole, its design strictly follows the OCP----opening and closing principle, that is:
1. Ensure that it is closed to modifications, that is, the entire operation process of Spring cannot be modified externally
2. It is open to extensions, that is, you can inherit the many abstract classes provided by Spring through inheritance Use interfaces to change the behavior of class loading
It is helpful to read the Spring source code (you don’t need to look at each class in detail, the general process can be sorted out) For individuals The improvement of the level is very helpful, and at the same time, some uncommon Spring problems can be discovered and solved at work.
However, the purpose of this article is not to organize the Spring process, but to introduce some commonly used Spring Bean tool classes so that we can better use the various features that Spring provides to developers. Let us let's start.
InitialingBean and DisposableBean
InitialingBean is an interface that provides a unique method afterPropertiesSet().
DisposableBean is also an interface, providing a unique method destroy().
These two interfaces are a set with similar functions, so they are put together: the former, as the name implies, calls the afterPropertiesSet() method to do some initialization work after the Bean properties are set. The destroy() method is called to do some finishing work before the end of the Beanlife cycle. Let's take a look at an example. In order to clearly know when to call the afterPropertiesSet() method, add an attribute, give the attribute set method, and print some content in the set method:
/** * @author 五月的仓颉 http://www.cnblogs.com/xrq730/p/5721366.html */ public class LifecycleBean implements InitializingBean, DisposableBean { @SuppressWarnings("unused") private String lifeCycleBeanName; public void setLifeCycleBeanName(String lifeCycleBeanName) { System.out.println("Enter LifecycleBean.setLifeCycleBeanName(), lifeCycleBeanName = " + lifeCycleBeanName); this.lifeCycleBeanName = lifeCycleBeanName; } public void destroy() throws Exception { System.out.println("Enter LifecycleBean.destroy()"); } public void afterPropertiesSet() throws Exception { System.out.println("Enter LifecycleBean.afterPropertiesSet()"); } public void beanStart() { System.out.println("Enter LifecycleBean.beanStart()"); } public void beanEnd() { System.out.println("Enter LifecycleBean.beanEnd()"); } }
Configure a spring.xml:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.1.xsd"> <bean id="lifeCycleBean" class="org.xrq.bean.lifecycle.LifecycleBean"> <property name="lifeCycleBeanName" value="lifeCycleBean" /> </bean> </beans>
Start the Spring container, and the result of LifecycleBean execution is:
Enter LifecycleBean.setLifeCycleBeanName(), lifeCycleBeanName = lifeCycleBean Enter LifecycleBean.afterPropertiesSet() Enter LifecycleBean.beanStart() Enter LifecycleBean.destroy() Enter LifecycleBean.beanEnd()
The execution result is the same as we thought, the afterPropertiesSet() method is just as its name indicates, it is will not be called until all properties of the Bean are set.
Regarding these two interfaces, I summarize a few points:
1. InitializingBean interface and Disposable interface can be used in conjunction with init-method and destroy-method. The execution order of the interface takes precedence over the configuration
2. The bottom layer of InitializingBean interface and Disposable interface uses type force transfer. Method name () to make direct method calls, and the bottom layer of init-method and destroy-methodUse reflection, the former is more coupled with Spring but more efficient, the latter decouples with Spring but is less efficient, which one to use depends on personal preference
3, afterPropertiesSet () method is called after the properties of the Bean are set. The afterPropertiesSet() method of a certain Bean will not be executed until the afterPropertiesSet() method of the next Bean is executed. Therefore, it is not recommended to write in the afterPropertiesSet() method that takes too long to process. Long method
BeanNameAware, ApplicationContextAware and BeanFactoryAware
The three interfaces are written together because they are a group and have similar functions.
"Aware" means "perceived", so the meaning of these three interfaces is not difficult to understand:
1. Beans that implement the BeanNameAware interface, during the Bean loading process You can get the Bean's id
2. For Beans that implement the ApplicationContextAware interface, you can get Spring's ApplicationContext during the Bean loading process. This is especially important. ApplicationContext is the Spring application context. You can get it from the ApplicationContext including A large amount of Spring container content and information including any Bean
3. Beans that implement the BeanFactoryAware interface can obtain the BeanFactory that loads the Bean during the Bean loading process
Look at the example:
/** * @author 五月的仓颉 http://www.cnblogs.com/xrq730/p/5721366.html */ public class AwareBean implements BeanNameAware, BeanFactoryAware, ApplicationContextAware { private String beanName; private ApplicationContext applicationContext; private BeanFactory beanFactory; public void setBeanName(String beanName) { System.out.println("Enter AwareBean.setBeanName(), beanName = " + beanName + "\n"); this.beanName = beanName; } public void setApplicationContext(ApplicationContext applicationContext) throws BeansException { System.out.println("Enter AwareBean.setApplicationContext(), applicationContext = " + applicationContext + "\n"); this.applicationContext = applicationContext; } public void setBeanFactory(BeanFactory beanFactory) throws BeansException { System.out.println("Enter AwareBean.setBeanFactory(), beanfactory = " + beanFactory + "\n"); this.beanFactory = beanFactory; } }
Configure a Spring.xml:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.1.xsd"> <bean id="AwareBean" class="org.xrq.bean.aware.AwareBean" /> </beans>
The execution result after starting the Spring container is:
Enter AwareBean.setBeanName(), beanName = AwareBean Enter AwareBean.setBeanFactory(), beanfactory = org.springframework.beans.factory.support.DefaultListableBeanFactory@2747fda0: defining beans [AwareBean,org.springframework.context.annotation.internalConfigurationAnnotationProcessor,org.springframework.context.annotation.internalAutowiredAnnotationProcessor,org.springframework.context.annotation.internalRequiredAnnotationProcessor,org.springframework.context.annotation.internalCommonAnnotationProcessor,org.springframework.context.annotation.ConfigurationClassPostProcessor.importAwareProcessor,org.springframework.context.annotation.ConfigurationClassPostProcessor.enhancedConfigurationProcessor]; root of factory hierarchy Enter AwareBean.setApplicationContext(), applicationContext = org.springframework.context.support.GenericApplicationContext@5514cd80: startup date [Mon Aug 08 19:23:30 CST 2016]; root of context hierarchy
Regarding these three interfaces and the above printing information, summarize a few points :
1. If your BeanName, ApplicationContext, and BeanFactory are useful, then define a variable to save them. If they are not useful, then you only need to implement the setXXX() method, using Just click on the parameters injected by Spring
2. If the Bean also implements InitializingBean,The container will ensure that BeanName, ApplicationContext and BeanFactory are injected after calling the afterPropertiesSet() method
FactoryBean
FactoryBean在Spring中是非常有用的,使用Eclipse/MyEclipse的朋友可以对FactoryBean使用ctrl+t查看一下,FactoryBean这个接口在Spring容器中有大量的子实现。
传统的Spring容器加载一个Bean的整个过程,都是由Spring控制的,换句话说,开发者除了设置Bean相关属性之外,是没有太多的自主权的。FactoryBean改变了这一点,开发者可以个性化地定制自己想要实例化出来的Bean,方法就是实现FactoryBean接口。
看一下代码例子,为了讲清楚FactoryBean,内容相对多一些,首先定义一个接口Animal:
public interface Animal { public void move(); }
定义两个实现类Monkey和Tiger:
public class Monkey implements Animal { public void move() { System.out.println("Monkey move!"); } }
public class Tiger implements Animal { public void move() { System.out.println("Tiger move!"); } }
写一个实现类,实现FactoryBean接口:
/** * @author 五月的仓颉 http://www.cnblogs.com/xrq730/p/5721366.html */ public class AnimalFactoryBean implements FactoryBean<Animal> { private String animal; public Animal getObject() throws Exception { if ("Monkey".equals(animal)) { return new Monkey(); } else if ("Tiger".equals(animal)) { return new Tiger(); } else { return null; } } public Class<?> getObjectType() { return Animal.class; } public boolean isSingleton() { return true; } public void setAnimal(String animal) { this.animal = animal; } }
配置一个spring.xml,注入属性Tiger:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.1.xsd"> <bean id="animal" class="org.xrq.bean.factory.AnimalFactoryBean"> <property name="animal" value="Tiger"/> </bean> </beans>
写一个JUnit的测试类:
@RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(locations = { "classpath*:spring.xml", }) public class BaseTest { @Resource private Animal animal; @Test public void aa() { animal.move(); } }
查看一下运行结果:
Tiger move!
看到最后得到的并不是FactoryBean本身,而是FactoryBean的泛型对象,这就是FactoryBean的作用。FactoryBean的几个方法:
1、getObject()方法是最重要的,控制Bean的实例化过程
2、getObjectType()方法获取接口返回的实例的class
3、isSingleton()方法获取该Bean是否为一个单例的Bean
像我这段代码的功能就是传入一个String类型的参数,可以动态控制生成出来的是接口的哪种子类。有了FactoryBean,同样的我们也可以灵活地操控Bean的生成。
BeanPostProcessor
之前的InitializingBean、DisposableBean、FactoryBean包括init-method和destory-method,针对的都是某个Bean控制其初始化的操作,而似乎没有一种办法可以针对每个Bean的生成前后做一些逻辑操作,PostProcessor则帮助我们做到了这一点,先看一个简单的BeanPostProcessor。
网上有一张图画了Bean生命周期的过程,画得挺好,原图出处:
BeanPostProcess接口有两个方法,都可以见名知意:
1、postProcessBeforeInitialization:在初始化Bean之前
2、postProcessAfterInitialization:在初始化Bean之后
值得注意的是,这两个方法是有返回值的,不要返回null,否则getBean的时候拿不到对象。
写一段测试代码,首先定义一个普通的Bean,为了后面能区分,给Bean加一个属性:
public class CommonBean { private String commonName; public void setCommonName(String commonName) { this.commonName = commonName; } public void initMethod() { System.out.println("Enter CommonBean.initMethod(), commonName = " + commonName); } }
定义一个PostProcess,实现BeanPostProcess接口:
/** * @author 五月的仓颉 http://www.cnblogs.com/xrq730/p/5721366.html */ public class PostProcessorBean implements BeanPostProcessor { public Object postProcessAfterInitialization(Object bean, String beanName) throws BeansException { System.out.println("Enter ProcessorBean.postProcessAfterInitialization()\n"); return bean; } public Object postProcessBeforeInitialization(Object bean, String beanName) throws BeansException { System.out.println("Enter ProcessorBean.postProcessBeforeInitialization()"); return bean; } }
配置一个spring.xml,给CommonBean的commonName赋予不同的值以区分:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.1.xsd"> <bean id="common0" class="org.xrq.bean.common.CommonBean" init-method="initMethod"> <property name="commonName" value="common0"/> </bean> <bean id="common1" class="org.xrq.bean.common.CommonBean" init-method="initMethod"> <property name="commonName" value="common1"/> </bean> <bean id="postProcessorBean" class="org.xrq.bean.processor.PostProcessorBean" /> </beans>
运行一个Spring容器, 初始化结果为:
Enter ProcessorBean.postProcessBeforeInitialization() Enter CommonBean.initMethod(), commonName = common0 Enter ProcessorBean.postProcessAfterInitialization() Enter ProcessorBean.postProcessBeforeInitialization() Enter CommonBean.initMethod(), commonName = common1 Enter ProcessorBean.postProcessAfterInitialization() Enter ProcessorBean.postProcessBeforeInitialization() Enter ProcessorBean.postProcessAfterInitialization()
看到每个Bean初始化前后都会分别执行postProcessorBeforeInitiallization()方法与postProcessorAfterInitialization()方法,最后两行出现原因是,PostProcessorBean本身也是一个Bean。
BeanFactoryPostProcessor
接下来看另外一个PostProcessor----BeanFactoryPostProcessor。
Spring允许在Bean创建之前,读取Bean的元属性,并根据自己的需求对元属性进行改变,比如将Bean的scope从singleton改变为prototype,最典型的应用应当是PropertyPlaceholderConfigurer,替换xml文件中的占位符,替换为properties文件中相应的key对应的value,这将会在下篇文章中专门讲解PropertyPlaceholderConfigurer的作用及其原理。
BeanFactoryPostProcessor就可以帮助我们实现上述的功能,下面来看一下BeanFactoryPostProcessor的使用,定义一个BeanFactoryPostProcessor的实现类:
/** * @author 五月的仓颉 http://www.cnblogs.com/xrq730/p/5721366.html */ public class FactoryPostProcessorBean implements BeanFactoryPostProcessor { public void postProcessBeanFactory(ConfigurableListableBeanFactory configurablelistablebeanfactory) throws BeansException { System.out.println("Enter FactoryPostProcessorBean.postProcessBeanFactory()\n"); } }
spring.xml里面配置一下这个Bean,就不写了,运行一下Spring容器,结果为:
Enter FactoryPostProcessorBean.postProcessBeanFactory() Enter ProcessorBean.postProcessBeforeInitialization() Enter CommonBean.initMethod(), commonName = common0 Enter ProcessorBean.postProcessAfterInitialization() Enter ProcessorBean.postProcessBeforeInitialization() Enter CommonBean.initMethod(), commonName = common1 Enter ProcessorBean.postProcessAfterInitialization() Enter ProcessorBean.postProcessBeforeInitialization() Enter ProcessorBean.postProcessAfterInitialization()
从执行结果中可以看出两点:
1、BeanFactoryPostProcessor的执行优先级高于BeanPostProcessor
2、BeanFactoryPostProcessor的postProcessBeanFactory()方法只会执行一次
注意到postProcessBeanFactory方法是带了参数ConfigurableListableBeanFactory的,这就和我之前说的可以使用BeanFactoryPostProcessor来改变Bean的属性相对应起来了。ConfigurableListableBeanFactory功能非常丰富,最基本的,它携带了每个Bean的基本信息,比如我简单写一段代码:
/** * @author 五月的仓颉 http://www.cnblogs.com/xrq730/p/5721366.html */ public void postProcessBeanFactory(ConfigurableListableBeanFactory configurablelistablebeanfactory) throws BeansException { BeanDefinition beanDefinition = configurablelistablebeanfactory.getBeanDefinition("common0"); MutablePropertyValues beanProperty = beanDefinition.getPropertyValues(); System.out.println("scope before change:" + beanDefinition.getScope()); beanDefinition.setScope("singleton"); System.out.println("scope after change:" + beanDefinition.getScope()); System.out.println("beanProperty:" + beanProperty); }
看一下执行结果:
scope before change: scope after change:singleton beanProperty:PropertyValues: length=1; bean property 'commonName'
这样就获取了Bean的生命周期以及重新设置了Bean的生命周期。ConfigurableListableBeanFactory还有很多的功能,比如添加BeanPostProcessor,可以自己去查看。
InstantiationAwareBeanPostProcessor
最后写一个叫做InstantiationAwareBeanPostProcessor的PostProcessor。
InstantiationAwareBeanPostProcessor又代表了Spring的另外一段生命周期:实例化。先区别一下Spring Bean的实例化和初始化两个阶段的主要作用:
1、实例化----实例化的过程是一个创建Bean的过程,即调用Bean的构造函数,单例的Bean放入单例池中
2、初始化----初始化的过程是一个赋值的过程,即调用Bean的setter,设置Bean的属性
之前的BeanPostProcessor作用于过程(2)前后,现在的InstantiationAwareBeanPostProcessor则作用于过程(1)前后,看一下代码,给前面的CommonBean加上构造函数:
public class CommonBean { public CommonBean() { System.out.println("Enter CommonBean's constructor"); } private String commonName; public void setCommonName(String commonName) { System.out.println("Enter CommonBean.setCommonName(), commonName = " + commonName); this.commonName = commonName; } public void initMethod() { System.out.println("Enter CommonBean.initMethod(), commonName = " + commonName); } }
实现InstantiationAwareBeanPostProcessor接口:
/** * @author 五月的仓颉 http://www.cnblogs.com/xrq730/p/5721366.html */ public class InstantiationAwareBeanPostProcessorBean implements InstantiationAwareBeanPostProcessor { public Object postProcessAfterInitialization(Object bean, String beanName) throws BeansException { System.out.println("Enter InstantiationAwareBeanPostProcessorBean.postProcessAfterInitialization()"); return bean; } public Object postProcessBeforeInitialization(Object bean, String beanName) throws BeansException { System.out.println("Enter InstantiationAwareBeanPostProcessorBean.postProcessBeforeInitialization()"); return bean; } public boolean postProcessAfterInstantiation(Object bean, String beanName) throws BeansException { System.out.println("Enter InstantiationAwareBeanPostProcessorBean.postProcessAfterInstantiation()"); return true; } public Object postProcessBeforeInstantiation(Class<?> bean, String beanName) throws BeansException { System.out.println("Enter InstantiationAwareBeanPostProcessorBean.postProcessBeforeInstantiation()"); return null; } public PropertyValues postProcessPropertyValues(PropertyValues pvs, PropertyDescriptor[] pd, Object bean, String beanName) throws BeansException { return pvs; } }
配置一下spring.xml:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.1.xsd"> <bean id="common" class="org.xrq.bean.common.CommonBean"> <property name="commonName" value="common"/> </bean> <bean class="org.xrq.bean.processor.InstantiationAwareBeanPostProcessorBean" /> </beans>
启动容器,观察一下运行结果为:
Enter InstantiationAwareBeanPostProcessorBean.postProcessBeforeInstantiation() Enter CommonBean's constructor Enter InstantiationAwareBeanPostProcessorBean.postProcessAfterInstantiation() Enter CommonBean.setCommonName(), commonName = common Enter InstantiationAwareBeanPostProcessorBean.postProcessBeforeInitialization() Enter InstantiationAwareBeanPostProcessorBean.postProcessAfterInitialization() Enter InstantiationAwareBeanPostProcessorBean.postProcessAfterInstantiation() Enter InstantiationAwareBeanPostProcessorBean.postProcessBeforeInitialization() Enter InstantiationAwareBeanPostProcessorBean.postProcessAfterInitialization()
最后三行的运行结果不去关注,看到很明显的,InstantiationAwareBeanPostProcessor作用的是Bean实例化前后,即:
1、Bean构造出来之前调用postProcessBeforeInstantiation()方法
2、Bean构造出来之后调用postProcessAfterInstantiation()方法
不过通常来讲,我们不会直接实现InstantiationAwareBeanPostProcessor接口,而是会采用继承InstantiationAwareBeanPostProcessorAdapter这个抽象类的方式来使用。
后记
如果只会写个Bean,配置在xml文件里面,注入一下,那是最最基础的Spring开发者。一个中级、高级的Spring开发者,必然会对Spring中的多个扩展点有所了解,并利用这些扩展点更好地为项目服务,使得整个代码结构更加地优雅,并且可读性、可维护性更好。
【相关推荐】
1. Java免费视频教程
2. JAVA教程手册
3. 全面解析Java注解
The above is the detailed content of Introduction to methods of Spring Bean extension interface. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
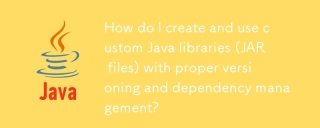
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
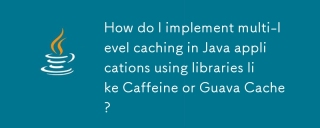
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
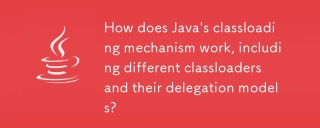
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
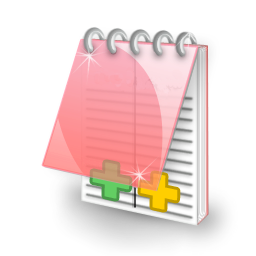
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use