This article mainly introduces the simple implementation of springMVC sending emails. It mainly uses javax.mail to send emails. Pictures and attachments can be sent. If you are interested, you can learn about it
Send using javax.mail Emails, pictures and attachments can all be sent
1, Controller class
package com.web.controller.api; import javax.annotation.Resource; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import com.service.EmailService; @Controller @RequestMapping("api") public class EmailTaskController { private static final Logger logger = LoggerFactory.getLogger(EmailTaskController.class); @Resource EmailService emailService; @RequestMapping("sendEmailTask") public void sendEmailTask() { logger.info("-------------执行发送邮件START---------------"); //写入excel //insuranceService.excelManage(); //发邮件 emailService.emailManage(); logger.info("-------------执行发送邮件END---------------"); } }
2, service class
package com.service.impl; import java.io.File; import java.util.ArrayList; import java.util.Date; import java.util.HashMap; import java.util.Iterator; import java.util.List; import java.util.Map; import javax.annotation.Resource; import javax.mail.MessagingException; import javax.mail.internet.MimeMessage; import org.apache.log4j.Logger; import org.springframework.beans.factory.annotation.Value; import org.springframework.core.io.FileSystemResource; import org.springframework.mail.SimpleMailMessage; import org.springframework.mail.javamail.JavaMailSender; import org.springframework.mail.javamail.MimeMessageHelper; import org.springframework.stereotype.Service; import com.entity.MailModel; import com.service.EmailService; import com.SimpleException; @Service public class EmailServiceImpl implements EmailService { private static Logger logger = Logger.getLogger(EmailServiceImpl.class); private String excelPath = "d://"; @Resource private JavaMailSender javaMailSender; @Resource private SimpleMailMessage simpleMailMessage; @Override public void emailManage(){ MailModel mail = new MailModel(); //主题 mail.setSubject("清单"); //附件 Map<String, String> attachments = new HashMap<String, String>(); attachments.put("清单.xlsx",excelPath+"清单.xlsx"); mail.setAttachments(attachments); //内容 StringBuilder builder = new StringBuilder(); builder.append("<html><body>你好!<br />"); builder.append("    附件是个人清单。<br />"); builder.append("    其中人信息;<br />"); builder.append("</body></html>"); String content = builder.toString(); mail.setContent(content); sendEmail(mail); } /** * 发送邮件 * * @author chenyq * @date 2016-5-9 上午11:18:21 * @throws Exception */ @Override public void sendEmail(MailModel mail) { // 建立邮件消息 MimeMessage message = javaMailSender.createMimeMessage(); MimeMessageHelper messageHelper; try { messageHelper = new MimeMessageHelper(message, true, "UTF-8"); // 设置发件人邮箱 if (mail.getEmailFrom()!=null) { messageHelper.setFrom(mail.getEmailFrom()); } else { messageHelper.setFrom(simpleMailMessage.getFrom()); } // 设置收件人邮箱 if (mail.getToEmails()!=null) { String[] toEmailArray = mail.getToEmails().split(";"); List<String> toEmailList = new ArrayList<String>(); if (null == toEmailArray || toEmailArray.length <= 0) { throw new SimpleException("收件人邮箱不得为空!"); } else { for (String s : toEmailArray) { if (s!=null&&!s.equals("")) { toEmailList.add(s); } } if (null == toEmailList || toEmailList.size() <= 0) { throw new SimpleException("收件人邮箱不得为空!"); } else { toEmailArray = new String[toEmailList.size()]; for (int i = 0; i < toEmailList.size(); i++) { toEmailArray[i] = toEmailList.get(i); } } } messageHelper.setTo(toEmailArray); } else { messageHelper.setTo(simpleMailMessage.getTo()); } // 邮件主题 if (mail.getSubject()!=null) { messageHelper.setSubject(mail.getSubject()); } else { messageHelper.setSubject(simpleMailMessage.getSubject()); } // true 表示启动HTML格式的邮件 messageHelper.setText(mail.getContent(), true); // 添加图片 if (null != mail.getPictures()) { for (Iterator<Map.Entry<String, String>> it = mail.getPictures().entrySet() .iterator(); it.hasNext();) { Map.Entry<String, String> entry = it.next(); String cid = entry.getKey(); String filePath = entry.getValue(); if (null == cid || null == filePath) { throw new RuntimeException("请确认每张图片的ID和图片地址是否齐全!"); } File file = new File(filePath); if (!file.exists()) { throw new RuntimeException("图片" + filePath + "不存在!"); } FileSystemResource img = new FileSystemResource(file); messageHelper.addInline(cid, img); } } // 添加附件 if (null != mail.getAttachments()) { for (Iterator<Map.Entry<String, String>> it = mail.getAttachments() .entrySet().iterator(); it.hasNext();) { Map.Entry<String, String> entry = it.next(); String cid = entry.getKey(); String filePath = entry.getValue(); if (null == cid || null == filePath) { throw new RuntimeException("请确认每个附件的ID和地址是否齐全!"); } File file = new File(filePath); if (!file.exists()) { throw new RuntimeException("附件" + filePath + "不存在!"); } FileSystemResource fileResource = new FileSystemResource(file); messageHelper.addAttachment(cid, fileResource); } } messageHelper.setSentDate(new Date()); // 发送邮件 javaMailSender.send(message); logger.info("------------发送邮件完成----------"); } catch (MessagingException e) { e.printStackTrace(); } } }
MailModel entity class
package com.support.entity; import java.util.Map; public class MailModel { /** * 发件人邮箱服务器 */ private String emailHost; /** * 发件人邮箱 */ private String emailFrom; /** * 发件人用户名 */ private String emailUserName; /** * 发件人密码 */ private String emailPassword; /** * 收件人邮箱,多个邮箱以“;”分隔 */ private String toEmails; /** * 邮件主题 */ private String subject; /** * 邮件内容 */ private String content; /** * 邮件中的图片,为空时无图片。map中的key为图片ID,value为图片地址 */ private Map<String, String> pictures; /** * 邮件中的附件,为空时无附件。map中的key为附件ID,value为附件地址 */ private Map<String, String> attachments; private String fromAddress;//发送人地址1个 private String toAddresses;//接收人地址,可以为很多个,每个地址之间用";"分隔,比方说450065208@qq.com;lpf@sina.com private String[] attachFileNames;//附件 public String getFromAddress() { return fromAddress; } public void setFromAddress(String fromAddress) { this.fromAddress = fromAddress; } public String getToAddresses() { return toAddresses; } public void setToAddresses(String toAddresses) { this.toAddresses = toAddresses; } public String getSubject() { return subject; } public void setSubject(String subject) { this.subject = subject; } public String getContent() { return content; } public void setContent(String content) { this.content = content; } public String[] getAttachFileNames() { return attachFileNames; } public void setAttachFileNames(String[] attachFileNames) { this.attachFileNames = attachFileNames; } public String getEmailHost() { return emailHost; } public void setEmailHost(String emailHost) { this.emailHost = emailHost; } public String getEmailFrom() { return emailFrom; } public void setEmailFrom(String emailFrom) { this.emailFrom = emailFrom; } public String getEmailUserName() { return emailUserName; } public void setEmailUserName(String emailUserName) { this.emailUserName = emailUserName; } public String getEmailPassword() { return emailPassword; } public void setEmailPassword(String emailPassword) { this.emailPassword = emailPassword; } public String getToEmails() { return toEmails; } public void setToEmails(String toEmails) { this.toEmails = toEmails; } public Map<String, String> getPictures() { return pictures; } public void setPictures(Map<String, String> pictures) { this.pictures = pictures; } public Map<String, String> getAttachments() { return attachments; } public void setAttachments(Map<String, String> attachments) { this.attachments = attachments; } }
spring.xml added Configuration information
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd"> <!-- 发送邮件 --> <bean id="javaMailSender" class="org.springframework.mail.javamail.JavaMailSenderImpl"> <property name="host"> <value>${mail.host}</value> </property> <property name="javaMailProperties"> <props> <prop key="mail.smtp.auth">true</prop> <prop key="mail.smtp.timeout">25000</prop> </props> </property> <property name="username"> <value>${mail.username}</value> </property> <property name="password"> <value>${mail.password}</value> </property> <property name="defaultEncoding"> <value>UTF-8</value> </property> </bean> <bean id="simpleMailMessage" class="org.springframework.mail.SimpleMailMessage"> <property name="from" value="${mail.from}" /> <property name="subject" value="${mail.subject}" /> <property name="to" value="${mail.to}" /> <!-- <property name="text" value="邮件内容" /> --> </bean> </beans>
dev.properties configuration
# email configuration mail.host=smtp.163.com mail.username=chenyanqing5945 mail.password=123456 mail.from=chenyanqing5945@163.com#发件人 mail.to=164792930@qq.com#收件人(多个用,隔开) mail.subject=testEmail #主题
[Related recommendations]
2. Java Basic Introduction Video Tutorial
The above is the detailed content of Java code example for sending emails. For more information, please follow other related articles on the PHP Chinese website!
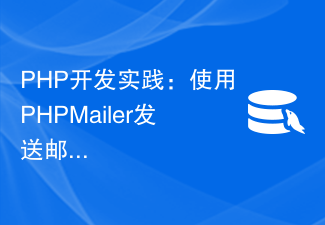
PHP开发实践:使用PHPMailer发送邮件到MySQL数据库中的用户引言:在现代互联网建设中,邮件是一种重要的沟通工具。无论是用户注册、密码重置,还是电子商务中的订单确认,发送电子邮件都是必不可少的功能。本文将介绍如何使用PHPMailer来发送电子邮件,并将邮件信息保存到MySQL数据库中的用户信息表中。一、安装PHPMailer库PHPMailer是
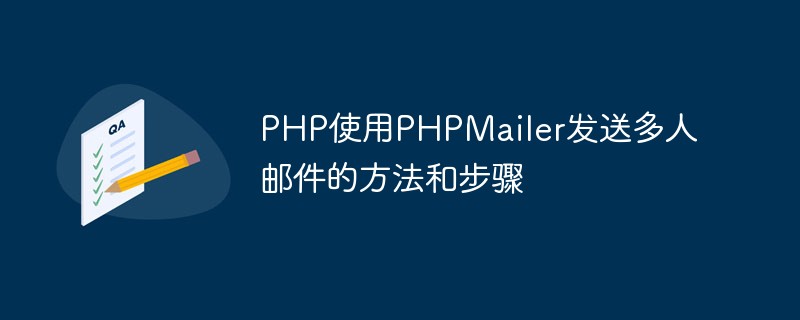
在Web应用程序中,往往需要将邮件一次性发送给多个收件人。PHP是一种很流行的Web开发语言,而PHPMailer是一种常见的发送邮件的PHP类库。PHPMailer提供了丰富的接口,使得在PHP应用程序中发送邮件变得更加方便和易于使用。在本篇文章中,我们将介绍如何使用PHPMailer向多个收件人发送邮件的方法和步骤。下载PHPMailer首先需要在官网(
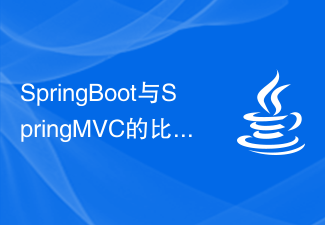
SpringBoot和SpringMVC都是Java开发中常用的框架,但它们之间有一些明显的差异。本文将探究这两个框架的特点和用途,并对它们的差异进行比较。首先,我们来了解一下SpringBoot。SpringBoot是由Pivotal团队开发的,它旨在简化基于Spring框架的应用程序的创建和部署。它提供了一种快速、轻量级的方式来构建独立的、可执行
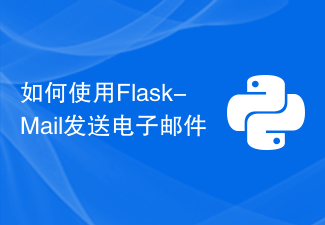
如何使用Flask-Mail发送电子邮件随着互联网的发展,电子邮件已经成为了人们沟通的重要工具。在开发Web应用中,有时候我们需要在特定的场景下发送电子邮件,比如用户注册成功后发送欢迎邮件,或者用户忘记密码时发送重置密码邮件等。Flask是一款简单而又灵活的PythonWeb框架,而Flask-Mail是Flask框架下用于发送邮件的扩展库,本文将介绍如何
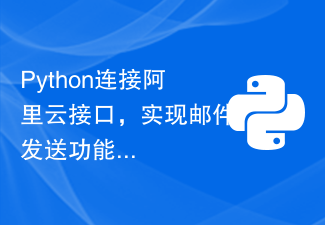
Python连接阿里云接口,实现邮件发送功能阿里云提供了一系列的服务接口,其中包括了邮件发送服务。通过Python脚本连接阿里云接口,我们可以实现邮件的快速发送。本篇文章将向您展示如何使用Python脚本连接阿里云接口,并实现邮件发送功能。首先,我们需要在阿里云上申请邮件发送服务,获取相应的接口信息。在阿里云管理控制台中,选择邮件推送服务,然后创建一个新的邮
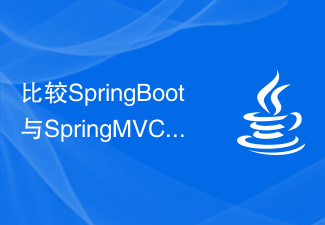
SpringBoot与SpringMVC的不同之处在哪里?SpringBoot和SpringMVC是两个非常流行的Java开发框架,用于构建Web应用程序。尽管它们经常分别被使用,但它们之间的不同之处也是很明显的。首先,SpringBoot可以被看作是一个Spring框架的扩展或者增强版。它旨在简化Spring应用程序的初始化和配置过程,以帮助开发人
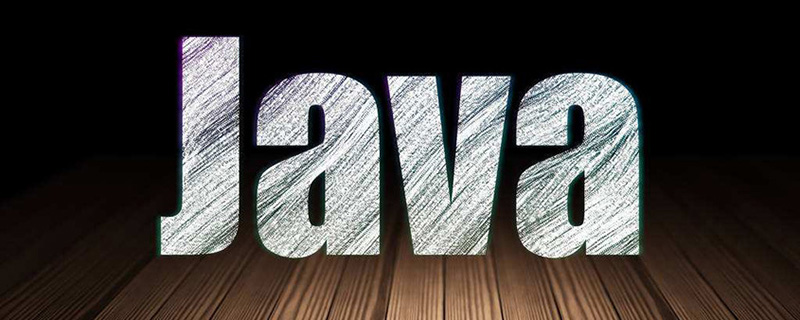
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
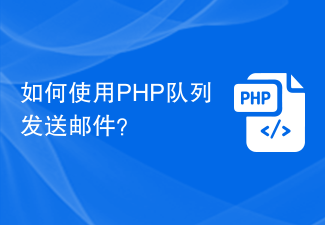
如何使用PHP队列发送邮件?在现代的Web开发中,我们经常需要发送大量的电子邮件。无论是批量发送电子邮件给大量用户,还是根据用户行为发送个性化的电子邮件,使用队列来发送邮件是一个非常好的实践。队列可以帮助我们提高邮件发送的效率和稳定性,避免因为发送太多邮件而导致服务器负载过高,同时还可以处理发送失败的场景。在PHP开发中,我们可以使用常见的队列工具,如Rab


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version
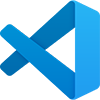
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor
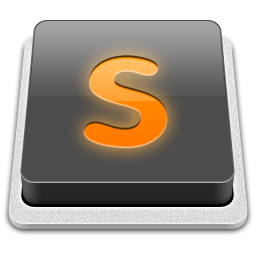
SublimeText3 Mac version
God-level code editing software (SublimeText3)
