


Java code examples for WeChat classification to receive messages and create entities
This article mainly introduces the third step of Java WeChat public platform development in detail, the classification of received messages and the creation of entities. It has certain reference value. Interested friends can refer to it
The previous article said that the application server and Tencent server communicate through messages, and briefly introduced the message types of WeChat posts. Here we will establish a message entity to facilitate our subsequent use!
(1) Message entity basic class
package com.cuiyongzhi.wechat.message.req; /** * ClassName: BaseMessage * @Description: 微信请求消息基本类 * @author dapengniao * @date 2016年3月7日 下午3:03:59 */ public class BaseMessage { // 开发者微信号 private String ToUserName; // 发送方帐号(一个OpenID) private String FromUserName; // 消息创建时间 (整型) private long CreateTime; // 消息类型(text/image/location/link/video/shortvideo) private String MsgType; // 消息id,64位整型 private long MsgId; public String getToUserName() { return ToUserName; } public void setToUserName(String toUserName) { ToUserName = toUserName; } public String getFromUserName() { return FromUserName; } public void setFromUserName(String fromUserName) { FromUserName = fromUserName; } public long getCreateTime() { return CreateTime; } public void setCreateTime(long createTime) { CreateTime = createTime; } public String getMsgType() { return MsgType; } public void setMsgType(String msgType) { MsgType = msgType; } public long getMsgId() { return MsgId; } public void setMsgId(long msgId) { MsgId = msgId; } }
(2) Ordinary message pojo entity
① Picture Message
package com.cuiyongzhi.wechat.message.req; /** * ClassName: ImageMessage * @Description: 图片消息 * @author dapengniao * @date 2016年3月7日 下午3:04:52 */ public class ImageMessage extends BaseMessage { // 图片链接 private String PicUrl; public String getPicUrl() { return PicUrl; } public void setPicUrl(String picUrl) { PicUrl = picUrl; } }
②Connection message
package com.cuiyongzhi.wechat.message.req; /** * ClassName: LinkMessage * @Description: 连接消息 * @author dapengniao * @date 2016年3月7日 下午3:05:48 */ public class LinkMessage extends BaseMessage { // 消息标题 private String Title; // 消息描述 private String Description; // 消息链接 private String Url; public String getTitle() { return Title; } public void setTitle(String title) { Title = title; } public String getDescription() { return Description; } public void setDescription(String description) { Description = description; } public String getUrl() { return Url; } public void setUrl(String url) { Url = url; } }
③Geolocation message
package com.cuiyongzhi.wechat.message.req; /** * ClassName: LocationMessage * @Description: 地理位置消息 * @author dapengniao * @date 2016年3月7日 下午3:06:10 */ public class LocationMessage extends BaseMessage { // 地理位置维度 private String Location_X; // 地理位置经度 private String Location_Y; // 地图缩放大小 private String Scale; // 地理位置信息 private String Label; public String getLocation_X() { return Location_X; } public void setLocation_X(String location_X) { Location_X = location_X; } public String getLocation_Y() { return Location_Y; } public void setLocation_Y(String location_Y) { Location_Y = location_Y; } public String getScale() { return Scale; } public void setScale(String scale) { Scale = scale; } public String getLabel() { return Label; } public void setLabel(String label) { Label = label; } }
④Text message
package com.cuiyongzhi.wechat.message.req; /** * ClassName: TextMessage * @Description: 文本消息 * @author dapengniao * @date 2016年3月7日 下午3:06:40 */ public class TextMessage extends BaseMessage { // 消息内容 private String Content; public String getContent() { return Content; } public void setContent(String content) { Content = content; } }
⑤Video/small video message
package com.cuiyongzhi.wechat.message.req; /** * ClassName: VideoMessage * @Description: 视频/小视屏消息 * @author dapengniao * @date 2016年3月7日 下午3:12:51 */ public class VideoMessage extends BaseMessage { private String MediaId; // 视频消息媒体id,可以调用多媒体文件下载接口拉取数据 private String ThumbMediaId; // 视频消息缩略图的媒体id,可以调用多媒体文件下载接口拉取数据 public String getMediaId() { return MediaId; } public void setMediaId(String mediaId) { MediaId = mediaId; } public String getThumbMediaId() { return ThumbMediaId; } public void setThumbMediaId(String thumbMediaId) { ThumbMediaId = thumbMediaId; } }
⑥Voice message
package com.cuiyongzhi.wechat.message.req; /** * ClassName: VoiceMessage * @Description: 语音消息 * @author dapengniao * @date 2016年3月7日 下午3:07:10 */ public class VoiceMessage extends BaseMessage { // 媒体ID private String MediaId; // 语音格式 private String Format; public String getMediaId() { return MediaId; } public void setMediaId(String mediaId) { MediaId = mediaId; } public String getFormat() { return Format; } public void setFormat(String format) { Format = format; } }
(3) Message classification processing
Do different distribution processing according to the message categories received above. Here we have established our own Business distributors (EventDispatcher, MsgDispatcher) perform ordinary message processing and event message processing respectively!
①MsgDispatcher.java——For business distribution processing of ordinary messages
package com.cuiyongzhi.wechat.dispatcher; import java.util.Map; import com.cuiyongzhi.wechat.util.MessageUtil; /** * ClassName: MsgDispatcher * @Description: 消息业务处理分发器 * @author dapengniao * @date 2016年3月7日 下午4:04:21 */ public class MsgDispatcher { public static String processMessage(Map<String, String> map) { if (map.get("MsgType").equals(MessageUtil.REQ_MESSAGE_TYPE_TEXT)) { // 文本消息 System.out.println("==============这是文本消息!"); } if (map.get("MsgType").equals(MessageUtil.REQ_MESSAGE_TYPE_IMAGE)) { // 图片消息 System.out.println("==============这是图片消息!"); } if (map.get("MsgType").equals(MessageUtil.REQ_MESSAGE_TYPE_LINK)) { // 链接消息 System.out.println("==============这是链接消息!"); } if (map.get("MsgType").equals(MessageUtil.REQ_MESSAGE_TYPE_LOCATION)) { // 位置消息 System.out.println("==============这是位置消息!"); } if (map.get("MsgType").equals(MessageUtil.REQ_MESSAGE_TYPE_VIDEO)) { // 视频消息 System.out.println("==============这是视频消息!"); } if (map.get("MsgType").equals(MessageUtil.REQ_MESSAGE_TYPE_VOICE)) { // 语音消息 System.out.println("==============这是语音消息!"); } return null; } }
②EventDispatcher.java——Business distribution processing of event messages
package com.cuiyongzhi.wechat.dispatcher; import java.util.Map; import com.cuiyongzhi.wechat.util.MessageUtil; /** * ClassName: EventDispatcher * @Description: 事件消息业务分发器 * @author dapengniao * @date 2016年3月7日 下午4:04:41 */ public class EventDispatcher { public static String processEvent(Map<String, String> map) { if (map.get("Event").equals(MessageUtil.EVENT_TYPE_SUBSCRIBE)) { //关注事件 System.out.println("==============这是关注事件!"); } if (map.get("Event").equals(MessageUtil.EVENT_TYPE_UNSUBSCRIBE)) { //取消关注事件 System.out.println("==============这是取消关注事件!"); } if (map.get("Event").equals(MessageUtil.EVENT_TYPE_SCAN)) { //扫描二维码事件 System.out.println("==============这是扫描二维码事件!"); } if (map.get("Event").equals(MessageUtil.EVENT_TYPE_LOCATION)) { //位置上报事件 System.out.println("==============这是位置上报事件!"); } if (map.get("Event").equals(MessageUtil.EVENT_TYPE_CLICK)) { //自定义菜单点击事件 System.out.println("==============这是自定义菜单点击事件!"); } if (map.get("Event").equals(MessageUtil.EVENT_TYPE_VIEW)) { //自定义菜单View事件 System.out.println("==============这是自定义菜单View事件!"); } return null; } }
At this time we need to The post method in the message entrance [WechatSecurity.java] has been modified. The final result is as follows:
package com.cuiyongzhi.wechat.controller; import java.io.PrintWriter; import java.util.Map; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.apache.log4j.Logger; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RequestParam; import com.cuiyongzhi.wechat.dispatcher.EventDispatcher; import com.cuiyongzhi.wechat.dispatcher.MsgDispatcher; import com.cuiyongzhi.wechat.util.MessageUtil; import com.cuiyongzhi.wechat.util.SignUtil; @Controller @RequestMapping("/wechat") public class WechatSecurity { private static Logger logger = Logger.getLogger(WechatSecurity.class); /** * * @Description: 用于接收get参数,返回验证参数 * @param @param request * @param @param response * @param @param signature * @param @param timestamp * @param @param nonce * @param @param echostr * @author dapengniao * @date 2016年3月4日 下午6:20:00 */ @RequestMapping(value = "security", method = RequestMethod.GET) public void doGet( HttpServletRequest request, HttpServletResponse response, @RequestParam(value = "signature", required = true) String signature, @RequestParam(value = "timestamp", required = true) String timestamp, @RequestParam(value = "nonce", required = true) String nonce, @RequestParam(value = "echostr", required = true) String echostr) { try { if (SignUtil.checkSignature(signature, timestamp, nonce)) { PrintWriter out = response.getWriter(); out.print(echostr); out.close(); } else { logger.info("这里存在非法请求!"); } } catch (Exception e) { logger.error(e, e); } } /** * @Description: 接收微信端消息处理并做分发 * @param @param request * @param @param response * @author dapengniao * @date 2016年3月7日 下午4:06:47 */ @RequestMapping(value = "security", method = RequestMethod.POST) public void DoPost(HttpServletRequest request,HttpServletResponse response) { try{ Map<String, String> map=MessageUtil.parseXml(request); String msgtype=map.get("MsgType"); if(MessageUtil.REQ_MESSAGE_TYPE_EVENT.equals(msgtype)){ EventDispatcher.processEvent(map); //进入事件处理 }else{ MsgDispatcher.processMessage(map); //进入消息处理 } }catch(Exception e){ logger.error(e,e); } } }
Finally, after we run the project successfully, we can verify the correctness of our message classification by sending different types of messages. , as shown in the figure below:
So many new files have been created. Finally, let’s take a look at the directory structure of our entire project:
The above is the detailed content of Java code examples for WeChat classification to receive messages and create entities. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
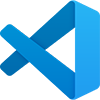
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
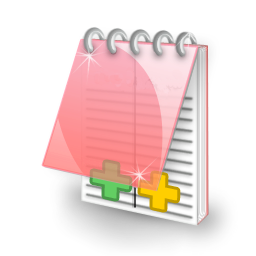
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use