This article mainly introduces the method of implementing FTP server in python. The editor thinks it is quite good. Now I will share it with you and give it as a reference. Let’s follow the editor to take a look.
Active mode and passive mode of FTP service
Before we start, let’s talk about the active mode and passive mode of FTP. The difference between them may be more clear by using two pictures:
Active mode:
Active mode working process:
1. The client initiates a connection to the server port 21 using a random non-privileged port N, which is a port greater than 1024.
2. The client starts listening to port N+1;
3. The server will actively connect to the client’s N+1 port through port 20.
Advantages of active mode:
The server configuration is simple, which is conducive to server security management. The server only needs to open port 21
Active mode Disadvantages:
If the client has a firewall turned on, or the client is on the intranet (behind a NAT gateway), the connection initiated by the server to the client port may fail
Passive Mode:
Passive mode working process:
1. The client connects to port 21 of the server through a random unprivileged port
2. The server opens an unprivileged port as a passive port and returns it to the client
3. The client actively connects to the server's passive port using the unprivileged port + 1 port
Disadvantages of passive mode:
Server configuration management is slightly complicated, which is not conducive to security. The server needs to open random high-level ports so that clients can connect, so most FTP service software can manually configure the passive port range
Passive Advantages of the mode: There are no requirements for the client network environment
After understanding FTP, start using python to implement FTP services
Preparation work
This The python version used for the first time: python 3.4.3
Install the module pyftpdlib
##
pip3 install pyftpdlibCreate the code file FtpServer.pyCode
Implement simple local verification
from pyftpdlib.authorizers import DummyAuthorizer from pyftpdlib.handlers import FTPHandler from pyftpdlib.servers import FTPServer #实例化虚拟用户,这是FTP验证首要条件 authorizer = DummyAuthorizer() #添加用户权限和路径,括号内的参数是(用户名, 密码, 用户目录, 权限) authorizer.add_user('user', '12345', '/home/', perm='elradfmw') #添加匿名用户 只需要路径 authorizer.add_anonymous('/home/huangxm') #初始化ftp句柄 handler = FTPHandler handler.authorizer = authorizer #监听ip 和 端口,因为linux里非root用户无法使用21端口,所以我使用了2121端口 server = FTPServer(('192.168.0.108', 2121), handler) #开始服务 server.serve_forever()
Start the service
$python FtpServer.pyTest Try:Add passive ports through the following code:
handler.passive_ports = range(2000, 2333)Full code:from pyftpdlib.authorizers import DummyAuthorizer from pyftpdlib.handlers import FTPHandler from pyftpdlib.servers import FTPServer #实例化虚拟用户,这是FTP验证首要条件 authorizer = DummyAuthorizer() #添加用户权限和路径,括号内的参数是(用户名, 密码, 用户目录, 权限) authorizer.add_user('user', '12345', '/home/', perm='elradfmw') #添加匿名用户 只需要路径 authorizer.add_anonymous('/home/huangxm') #初始化ftp句柄 handler = FTPHandler handler.authorizer = authorizer #添加被动端口范围 handler.passive_ports = range(2000, 2333) #监听ip 和 端口 server = FTPServer(('192.168.0.108', 2121), handler) #开始服务 server.serve_forever()Start the service and you can see the passive port information:
$ python FtpServer.py [I 2017-01-11 15:18:37] >>> starting FTP server on 192.168.0.108:2121, pid=46296 <<< [I 2017-01-11 15:18:37] concurrency model: async [I 2017-01-11 15:18:37] masquerade (NAT) address: None [I 2017-01-11 15:18:37] passive ports: 2000->2332
FTP user management:
Through the above practice, the FTP server can already work normally, but what if many FTP users are needed? Should each user write it once? In fact, we can define a user file user.py#用户名 密码 权限 目录 # root 12345 elradfmwM /home huangxm 12345 elradfmwM /homeand then traverse the file and add lines that do not start with # to the user_list listComplete code:
from pyftpdlib.authorizers import DummyAuthorizer from pyftpdlib.handlers import FTPHandler from pyftpdlib.servers import FTPServer def get_user(userfile): #定义一个用户列表 user_list = [] with open(userfile) as f: for line in f: print(len(line.split())) if not line.startswith('#') and line: if len(line.split()) == 4: user_list.append(line.split()) else: print("user.conf配置错误") return user_list #实例化虚拟用户,这是FTP验证首要条件 authorizer = DummyAuthorizer() #添加用户权限和路径,括号内的参数是(用户名, 密码, 用户目录, 权限) #authorizer.add_user('user', '12345', '/home/', perm='elradfmw') user_list = get_user('/home/huangxm/test_py/FtpServer/user.conf') for user in user_list: name, passwd, permit, homedir = user try: authorizer.add_user(name, passwd, homedir, perm=permit) except Exception as e: print(e) #添加匿名用户 只需要路径 authorizer.add_anonymous('/home/huangxm') #初始化ftp句柄 handler = FTPHandler handler.authorizer = authorizer #添加被动端口范围 handler.passive_ports = range(2000, 2333) #监听ip 和 端口 server = FTPServer(('192.168.0.108', 2121), handler) #开始服务 server.serve_forever()At this point, the FTP service has been completed.
Standardize the code
First create the conf directory to store settings.py and user.pyDirectory structure (don’t worry about the cache):ip = '0.0.0.0' port = '2121' #上传速度 300kb/s max_upload = 300 * 1024 #下载速度 300kb/s max_download = 300 * 1024 #最大连接数 max_cons = 150 #最多IP数 max_per_ip = 10 #被动端口范围,注意被动端口数量要比最大IP数多,否则可能出现无法连接的情况 passive_ports = (2000, 2200) #是否开启匿名访问 on|off enable_anonymous = 'off' #匿名用户目录 anonymous_path = '/home/huangxm' #是否开启日志 on|off enable_logging = 'off' #日志文件 loging_name = 'pyftp.log' #欢迎信息 welcome_msg = 'Welcome to my ftp'user.py
#用户名 密码 权限 目录 #root 12345 elradfmwM /home/ huangxm 12345 elradfmwM /home/ test 12345 elradfmwM /home/huangxmFtpServer.py
##
from pyftpdlib.authorizers import DummyAuthorizer from pyftpdlib.handlers import FTPHandler, ThrottledDTPHandler from pyftpdlib.servers import FTPServer from conf import settings import logging def get_user(userfile): #定义一个用户列表 user_list = [] with open(userfile) as f: for line in f: if not line.startswith('#') and line: if len(line.split()) == 4: user_list.append(line.split()) else: print("user.conf配置错误") return user_list def ftp_server(): #实例化虚拟用户,这是FTP验证首要条件 authorizer = DummyAuthorizer() #添加用户权限和路径,括号内的参数是(用户名, 密码, 用户目录, 权限) #authorizer.add_user('user', '12345', '/home/', perm='elradfmw') user_list = get_user('conf/user.py') for user in user_list: name, passwd, permit, homedir = user try: authorizer.add_user(name, passwd, homedir, perm=permit) except Exception as e: print(e) #添加匿名用户 只需要路径 if settings.enable_anonymous == 'on': authorizer.add_anonymous(settings.anonymous_path) #下载上传速度设置 dtp_handler = ThrottledDTPHandler dtp_handler.read_limit = settings.max_download dtp_handler.write_limit = settings.max_upload #初始化ftp句柄 handler = FTPHandler handler.authorizer = authorizer #日志记录 if settings.enable_logging == 'on': logging.basicConfig(filename=settings.loging_name, level=logging.INFO) #欢迎信息 handler.banner = settings.welcome_msg #添加被动端口范围 handler.passive_ports = range(settings.passive_ports[0], settings.passive_ports[1]) #监听ip 和 端口 server = FTPServer((settings.ip, settings.port), handler) #最大连接数 server.max_cons = settings.max_cons server.max_cons_per_ip = settings.max_per_ip #开始服务 print('开始服务') server.serve_forever() if __name__ == "__main__": ftp_server()
Finally, let’s talk about the permission issue
Read permission:
Change file directory | |
List files | |
Receive files from server |
d | |
f | |
m | |
w | |
M | |
The above is the detailed content of How to implement FTP server service in Python (Collection). For more information, please follow other related articles on the PHP Chinese website!
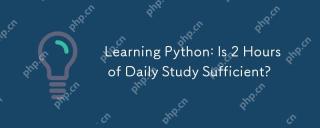
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
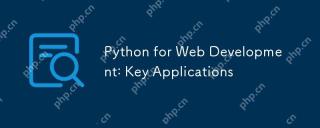
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
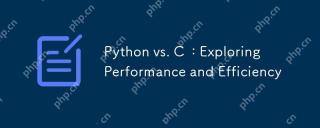
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
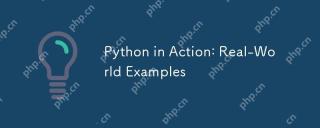
Python's real-world applications include data analytics, web development, artificial intelligence and automation. 1) In data analysis, Python uses Pandas and Matplotlib to process and visualize data. 2) In web development, Django and Flask frameworks simplify the creation of web applications. 3) In the field of artificial intelligence, TensorFlow and PyTorch are used to build and train models. 4) In terms of automation, Python scripts can be used for tasks such as copying files.
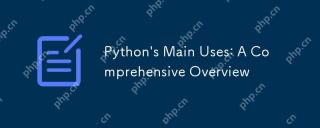
Python is widely used in data science, web development and automation scripting fields. 1) In data science, Python simplifies data processing and analysis through libraries such as NumPy and Pandas. 2) In web development, the Django and Flask frameworks enable developers to quickly build applications. 3) In automated scripts, Python's simplicity and standard library make it ideal.
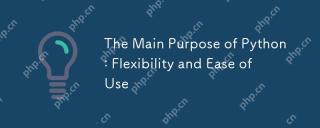
Python's flexibility is reflected in multi-paradigm support and dynamic type systems, while ease of use comes from a simple syntax and rich standard library. 1. Flexibility: Supports object-oriented, functional and procedural programming, and dynamic type systems improve development efficiency. 2. Ease of use: The grammar is close to natural language, the standard library covers a wide range of functions, and simplifies the development process.
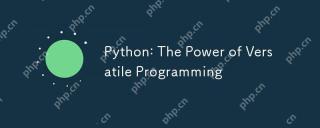
Python is highly favored for its simplicity and power, suitable for all needs from beginners to advanced developers. Its versatility is reflected in: 1) Easy to learn and use, simple syntax; 2) Rich libraries and frameworks, such as NumPy, Pandas, etc.; 3) Cross-platform support, which can be run on a variety of operating systems; 4) Suitable for scripting and automation tasks to improve work efficiency.
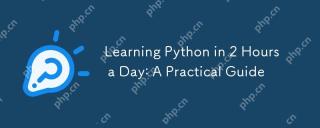
Yes, learn Python in two hours a day. 1. Develop a reasonable study plan, 2. Select the right learning resources, 3. Consolidate the knowledge learned through practice. These steps can help you master Python in a short time.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
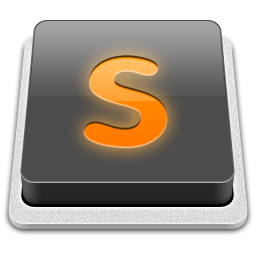
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Notepad++7.3.1
Easy-to-use and free code editor