DOM解析的基本思路:
1、将整个XML文件一次性读入内存
2、将整个XML看做一棵树
3、XML中的每一个标签,属性,文本都看做是树上的一个结点
4、然后可以对结点进行增删改查的操作
话不多说,上代码。
1、首先我在D:\ABC中新建了一个文本文件,重命名为stus.xml,以下是文件中的内容
<?xml version = "1.0" encoding = "GBK" ?> <stus class = "S160401A"> <stu num = "001" > <name>张三</name> <age>20</age> <sex>男</sex> </stu> <stu num = "002"> <name>李四</name> <age>21</age> <sex>女</sex> </stu> <stu num = "003"> <name>王五</name> <age>22</age> <sex>男</sex> </stu> </stus>
在第一行是XML声明,version表示版本号,encoding表示编码方式,微软的记事本用的是国标的编码方式,如果要用UTF-8,则要在另存为窗口中修改编码方式为UTF-8。
必须且只能有一对根标签,我写的根标签是
2、这是一个学生类,定义了一些属性和get、set方法
<span style="font-size: 16px;">public class Student { public static String Class; private String name; private int num; private int age; private char sex; public String getName() { return name; } public void setName(String name) { this.name = name; } public int getNum() { return num; } public void setNum(int num) { this.num = num; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public char getSex() { return sex; } public void setSex(char sex) { this.sex = sex; } }</span>
3、这是用DOM解析的类,看这个类之前还要了解一下。
DocumentBuilderFactory DOM解析器工厂
DocumentBuilder DOM解析器
Document 文档对象
Node 结点【接口】
Element 元素结点【标签结点】
Attr 属性结点
Text 文本结点
Node 是Document,Element,Attr,Text的父接口
NodeList 结点列表
NamedNodeMap 一个结点的所有属性
<span style="font-size: 16px;">import javax.xml.parsers.DocumentBuilder; import javax.xml.parsers.DocumentBuilderFactory; import org.w3c.dom.Attr; import org.w3c.dom.Document; import org.w3c.dom.Element; import org.w3c.dom.NamedNodeMap; import org.w3c.dom.Node; import org.w3c.dom.NodeList; import bean.Student; public class DOMParser { public static void main(String[] args) throws Exception { // 得到解析器工厂对象 DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); // 生产一个解析器对象 DocumentBuilder builder = factory.newDocumentBuilder(); // 开始解析XML文件,得到解析的结果,是一个Document对象 // Document对象叫做文档树对象 Document dom = builder.parse("D:\\ABC\\stus.xml"); // 通过Document对象提取数据 // Document对象的第一个子节点是根节点[根标签] Node root = dom.getFirstChild(); // 获得标签的名字 String str = root.getNodeName(); // 获得根节点的属性 NamedNodeMap attrs = root.getAttributes(); // 强转成Attr类型 属性类 Attr attr = (Attr) attrs.getNamedItem("class"); // 获得属性里的值 String v = attr.getValue(); System.out.println(v); // 获得所有的学生------------------------------------- NodeList list = root.getChildNodes(); for (int i = 0; i < list.getLength(); i++) { Node node = list.item(i); // 判断是否是标签结点 if (node instanceof Element) { Element e = (Element) node; // 获得标签结点里属性的值 String num = e.getAttribute("num"); System.out.println(num); // 输出标签中的文本 // System.out.println(e.getTextContent()); // 继续获得stu的子节点 NodeList nodeList = e.getChildNodes(); for (int j = 0; j < nodeList.getLength(); j++) { Node n = nodeList.item(j); if (n instanceof Element) { Element ele = (Element) n; // 获得元素结点的标签名字 String nodeName = ele.getNodeName(); // 获得元素结点标签中的文本 String value = ele.getTextContent(); if (nodeName.equals("name")) { System.out.println("姓名:" + value); } else if (nodeName.equals("age")) { System.out.println("年龄:" + value); } else if (nodeName.equals("sex")) { System.out.println("性别:" + value); } } } } } } }</span>
自己在其中总结了一些方法:
DocumentBuilderFactory类:
public static DocumentBuilderFactory newInstance(); //得到解析器工厂对象 public abstract DocumentBuilder newDocumentBuilder(); //生产一个解析器对象
DocumentBuilder类:
public Document parse(String uri); //解析路径为uri的XML文件,得到解析的结果是一个Document对象
Node类:
public Node getFirstChild(); //得到Document对象的第一个子结点,也就是根结点、或者叫根标签,在上面的代码中得到的是stus,看上面的第1点中的XML文件的内容。 public NamedNodeMap getAttributes();//获得结点的属性 public NodeList getChildNodes();//获得所有子结点 public String getNodeName();//获得标签的名字 public String getTextContent() throws DOMException;//获得标签结点中的文本
NamedNodeMap类:
public Node getNamedItem(String name);//返回所有名字为name的结点
Attr类:
public String getValue();//获得属性里的值
NodeList类:
public Node item(int index);//返回第index个结点
Element类:
public String getAttribute(String name);//获得标签结点里属性name的值
The above is the detailed content of Detailed explanation of XML-JAXP technology-DOM parsing. For more information, please follow other related articles on the PHP Chinese website!

RSS is an XML-based format used to publish frequently updated data. As a web developer, understanding RSS can improve content aggregation and automation update capabilities. By learning RSS structure, parsing and generation methods, you will be able to handle RSSfeeds confidently and optimize your web development skills.

RSS chose XML instead of JSON because: 1) XML's structure and verification capabilities are better than JSON, which is suitable for the needs of RSS complex data structures; 2) XML was supported extensively at that time; 3) Early versions of RSS were based on XML and have become a standard.

RSS is an XML-based format used to subscribe and read frequently updated content. Its working principle includes two parts: generation and consumption, and using an RSS reader can efficiently obtain information.

The core structure of RSS documents includes XML tags and attributes. The specific parsing and generation steps are as follows: 1. Read XML files, process and tags. 2. Extract,,, etc. tag information. 3. Handle custom tags and attributes to ensure version compatibility. 4. Use cache and asynchronous processing to optimize performance to ensure code readability.

The main differences between JSON, XML and RSS are structure and uses: 1. JSON is suitable for simple data exchange, with a simple structure and easy to parse; 2. XML is suitable for complex data structures, with a rigorous structure but complex parsing; 3. RSS is based on XML and is used for content release, standardized but limited use.

The processing of XML/RSS feeds involves parsing and optimization, and common problems include format errors, encoding issues, and missing elements. Solutions include: 1. Use XML verification tools to check for format errors; 2. Ensure encoding consistency and use the chardet library to detect encoding; 3. Use default values or skip the element when missing elements; 4. Use efficient parsers such as lxml and cache parsing results to optimize performance; 5. Pay attention to data consistency and security to prevent XML injection attacks.

The steps to parse RSS documents include: 1. Read the XML file, 2. Use DOM or SAX to parse XML, 3. Extract headings, links and other information, and 4. Process data. RSS documents are XML-based formats used to publish updated content, structures containing, and elements, suitable for building RSS readers or data processing tools.

RSS and XML are the core technologies in network content distribution and data exchange. RSS is used to publish frequently updated content, and XML is used to store and transfer data. Development efficiency and performance can be improved through usage examples and best practices in real projects.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Linux new version
SublimeText3 Linux latest version
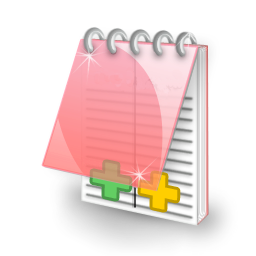
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Notepad++7.3.1
Easy-to-use and free code editor
