


This article mainly introduces the use of JavaScript to achieve image cutting effects. This article introduces you to it in great detail and has reference value. Friends who need it can refer to
to learn how to do it. Obtain the coordinate position of the mouse and monitor the mouse's action events such as pressing, dragging, and releasing, so as to drag the mouse to change the image size.
You can also learn the clip attribute in css.
1. Use CSS to achieve image opacity and cropping effects.
Three-layer structure of picture cutting
1. First layer opacity, set transparency to the layer
2. Second layer clip, clip attribute: Crop the picture to display part of the image and hide other parts
3. The third layer selection box absolute (overlapping with the second layer), including The effect of eight contacts
html code:
<p id="box"> <img src="/static/imghwm/default1.png" data-src="img/1.jpg" class="lazy" id="img1" / alt="Sample code to implement image cutting effect based on JavaScript (picture)" > <img src="/static/imghwm/default1.png" data-src="img/1.jpg" class="lazy" id="img2" / alt="Sample code to implement image cutting effect based on JavaScript (picture)" > <p id="main"> <p class="pmin up-left"></p> <p class="pmin up"></p> <p class="pmin up-right"></p> <p class="pmin right"></p> <p class="pmin right-down"></p> <p class="pmin down"></p> <p class="pmin left-down"></p> <p class="pmin left"></p> </p> </p>
css code:
body{ background: #333; } #box{ width: 500px; height: 380px; position: absolute; top: 100px; left: 200px; } #img1,#img2{ position: absolute; top: 0; left: 0; } #img1{ opacity: 0.3; } #img2{ clip: rect(0,200px,200px,0); } #main{ position: absolute;/*第三层需用绝对定位浮在上面*/ width: 200px; height: 200px; border: 1px solid #fff; } .pmin{ position: absolute; width: 8px; height: 8px; background: #fff; } .up-left{margin-top: -4px;margin-left: -4px;cursor: nw-resize;} .up{ left: 50%;/*父元素盒子main宽度的一半,注意要有绝对定位*/ margin-left:-4px; top: -4px; cursor: n-resize; } .up-right{top: -4px;right: -4px;cursor: ne-resize;} .right{top: 50%;margin-top: -4px;right: -4px;cursor: e-resize;} .right-down{right: -4px;bottom: -4px;cursor: se-resize;} .down{bottom: -4px;left: 50%;margin-left: -4px;cursor: s-resize;} .left-down{left: -4px;bottom: -4px;cursor: sw-resize;} .left{left: -4px;top: 50%;margin-top: -4px;cursor: w-resize;}
2. javascript Get the offset of the selection box
Detailed explanation of the mouse drag position of the selection box:
offsetLeft: the distance of the element relative to the left border of its parent element;
clientX: the abscissa of the mouse position;
clientWidth: the width of the element;
offsetXY: is the coordinate in the box model where the event occurs, regardless of the scroll bar.
clientXY: It is the coordinate in the available part of the entire browser, and has nothing to do with the scroll bar, that is, the area that requires dragging the scroll bar to see is not considered.
pageXY: It is the coordinate in the entire web page, related to the scroll bar.
Construct a getPosition() function to obtain the distance of an element relative to the left and top of the screen
The js code is as follows:
//获取元素相对于屏幕左边及上边的距离,利用offsetLeft function getPosition(el){ var left = el.offsetLeft; var top = el.offsetTop; var parent = el.offsetParent; while(parent != null){ left += parent.offsetLeft; top += parent.offsetTop; parent = parent.offsetParent; } return {"left":left,"top":top}; }
3. Javascript implements control contacts
Listen to the events of mouse pressing, dragging, and releasing to control the size of the selection box.
Note the difference:
The Element.clientWidth property represents the internal width of the element, in pixels. This property includes padding, but does not include vertical scrollbars (if any), borders, and margins.
That is, clientWidth does not include the border, offsetWidth includes the border
1) Click on the contact on the right
js code:
var mainp = $('main'); var rightp = $('right'); var isDraging = false; var contact = "";//表示被按下的触点 //鼠标按下时 rightp.onmousedown = function(){ isDraging = true; contact = "right"; } //鼠标松开时 window.onmouseup = function(){ isDraging = false; } //鼠标移动时 window.onmousemove = function(e){ if(isDraging == true){ if(contact == "right"){ var e = e||window.event; var x = e.clientX;//鼠标位置的横坐标 var widthBefore = mainp.offsetWidth - 2;//选取框变化前的宽度 //var widthBefore = mainp.clientWidth; var addWidth = x - getPosition(mainp).left - widthBefore;//鼠标移动后选取框增加的宽度 mainp.style.width = widthBefore + addWidth + 'px';//选取框变化后的宽度 } } } //获取id的函数 function $(id){ return document.getElementById(id); }
2) Click on the contact above
When you click on the middle contact point above to move, the height of the selection box and the position of the upper left corner need to change.
Increased height = distance of the selection box relative to the top of the screen - ordinate of the mouse position
The top value of the upper left corner of the selection box needs to be subtracted from the increased height, because the height when the mouse moves upward As it increases, the top value decreases. When moving downward, the height decreases and top increases.
Change diagram:
##
else if(contact == "up"){ var y = e.clientY;//鼠标位置的纵坐标 var heightBefore = mainp.offsetHeight - 2;//选取框变化前的高度 var addHeight = getPosition(mainp).top - y;//增加的高度 mainp.style.height = heightBefore + addHeight + 'px';//选取框变化后的宽度 mainp.style.top = mainp.offsetTop - addHeight + 'px';//相当于变化后左上角的纵坐标,鼠标向上移纵坐标减小,下移增大 }
3) Click on the left contact
The principle is the same as clicking on the contact point above, the width and left position will change
Change diagram:
js code:
else if(contact == "left"){ var widthBefore = mainp.offsetWidth - 2; var addWidth = getPosition(mainp).left - e.clientX;//增加的宽度等于距离屏幕左边的距离减去鼠标位置横坐标 mainp.style.width = widthBefore + addWidth + 'px'; mainp.style.left = mainp.offsetLeft - addWidth + 'px';//左边的距离(相当于左边位置横坐标)等于选取框距父级元素的距离减去增加的宽度 }
4) Click the contact below
Increased width = ordinate of mouse position - distance from the top of the screen - original width
Upper left corner The position does not need to be changed
js code:
else if(contact = "down"){ var heightBefore = mainp.offsetHeight - 2; var addHeight = e.clientY - getPosition(mainp).top - mainp.offsetHeight; mainp.style.height = heightBefore + addHeight + 'px'; }
5) The change when clicking the four corners is the superposition of the height and width changes, so it is best Encapsulate the above four changing processes into functions to facilitate maintenance and code reuse.
If you use if else, you need to judge 8 times, so change it to
switch statementto simplify the code The modified js code is as follows:
window.onmousemove = function(e){ var e = e||window.event; if(isDraging == true){ switch (contact){ case "up" : upMove(e);break; case "right" : rightMove(e);break; case "down" : downMove(e);break; case "left" : leftMove(e);break; case "up-right" : upMove(e);rightMove(e);break; case "down-right" : downMove(e);rightMove(e);break; case "down-left" : downMove(e);leftMove(e);break; case "up-left" : upMove(e);leftMove(e);break; } } } //获取id的函数 function $(id){ return document.getElementById(id); } //获取元素相对于屏幕左边及上边的距离,利用offsetLeft function getPosition(el){ var left = el.offsetLeft; var top = el.offsetTop; var parent = el.offsetParent; while(parent != null){ left += parent.offsetLeft; top += parent.offsetTop; parent = parent.offsetParent; } return {"left":left,"top":top}; } //up移动 function upMove(e){ var y = e.clientY;//鼠标位置的纵坐标 var heightBefore = mainp.offsetHeight - 2;//选取框变化前的高度 var addHeight = getPosition(mainp).top - y;//增加的高度 mainp.style.height = heightBefore + addHeight + 'px';//选取框变化后的宽度 mainp.style.top = mainp.offsetTop - addHeight + 'px';//相当于变化后左上角的纵坐标,鼠标向上移纵坐标减小,下移增大 } //right移动 function rightMove(e){ var x = e.clientX;//鼠标位置的横坐标 var widthBefore = mainp.offsetWidth - 2;//选取框变化前的宽度 //var widthBefore = mainp.clientWidth; var addWidth = x - getPosition(mainp).left - widthBefore;//鼠标移动后选取框增加的宽度 mainp.style.width = widthBefore + addWidth + 'px';//选取框变化后的宽度 } //down移动 function downMove(e){ var heightBefore = mainp.offsetHeight - 2; var addHeight = e.clientY - getPosition(mainp).top - mainp.offsetHeight; mainp.style.height = heightBefore + addHeight + 'px'; } //left移动 function leftMove(e){ var widthBefore = mainp.offsetWidth - 2; var addWidth = getPosition(mainp).left - e.clientX;//增加的宽度等于距离屏幕左边的距离减去鼠标位置横坐标 mainp.style.width = widthBefore + addWidth + 'px'; mainp.style.left = mainp.offsetLeft - addWidth + 'px';//左边的距离(相当于左边位置横坐标)等于选取框距父级元素的距离减去增加的宽度 }
4. Achieve bright display of the selection box area1) The clip attribute of the second layer picture in the selection box needs to be reset
Illustrations of four aspects:
##js code:
//设置选取框图片区域明亮显示 function setChoice(){ var top = mainp.offsetTop; var right = mainp.offsetLeft + mainp.offsetWidth; var bottom = mainp.offsetTop + mainp.offsetHeight; var left = mainp.offsetLeft; img2.style.clip = "rect("+top+"px,"+right+"px,"+bottom+"px,"+left+"px)"; }2) When the mouse moves, the picture will be selected. You can Add a line of code to the js code to prevent the image from being selected
//禁止图片被选中 document.onselectstart = new Function('event.returnValue = false;');You can also add *{user-select:none} in the css style, which means that the text cannot be selected, and the image is followed by p Has the same effect. 5. Implement the draggable selection box position
First of all, we must prevent the event from bubbling
The js code is as follows://鼠标按下触点时 rightp.onmousedown = function(e){ e.stopPropagation(); isDraging = true; contact = "right"; }
6. Implement preview of picture cutting area
Add a p
<p id="preview"> <img src="/static/imghwm/default1.png" data-src="img/1.jpg" class="lazy" id="img3" / alt="Sample code to implement image cutting effect based on JavaScript (picture)" > </p>
css代码:
#preview{ position: absolute; width: 500px; height: 380px; top: 100px; left:710px ; } #preview #img3{position: absolute;}
注意:要让clip:rect(top,right,bottom,left) 起作用,必须让作用元素为相对/绝对定位。
js部分同样是利用clip属性,和setChoice()函数同时被调用
同时为了让右边预览区的左上角位置固定,需要设置其top和left的值
//右边图片预览函数 function setPreview(){ var top = mainp.offsetTop; var right = mainp.offsetLeft + mainp.offsetWidth; var bottom = mainp.offsetTop + mainp.offsetHeight; var left = mainp.offsetLeft; var img3 = $('img3'); img3.style.top = -top + 'px'; img3.style.left = -left + 'px'; img3.style.clip = "rect("+top+"px,"+right+"px,"+bottom+"px,"+left+"px)"; }
The above is the detailed content of Sample code to implement image cutting effect based on JavaScript (picture). For more information, please follow other related articles on the PHP Chinese website!
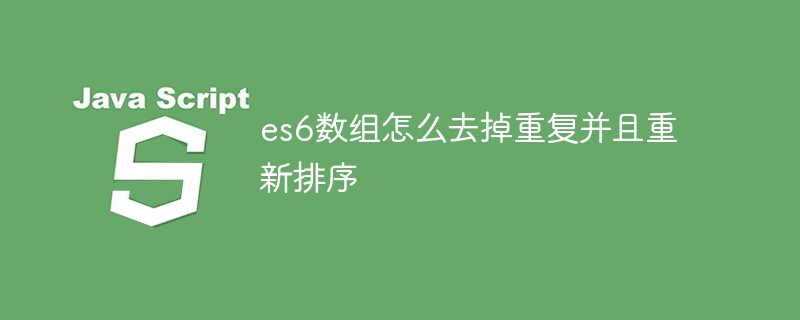
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
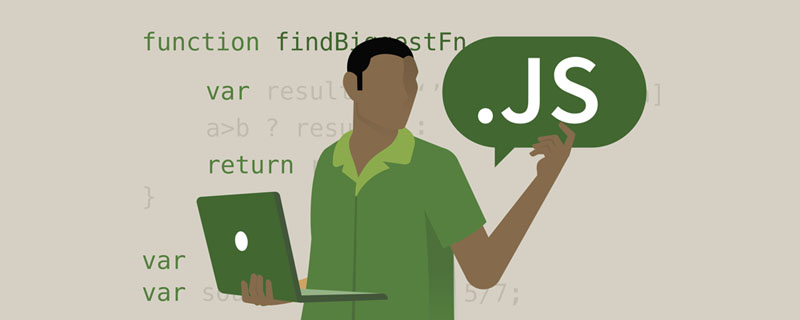
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
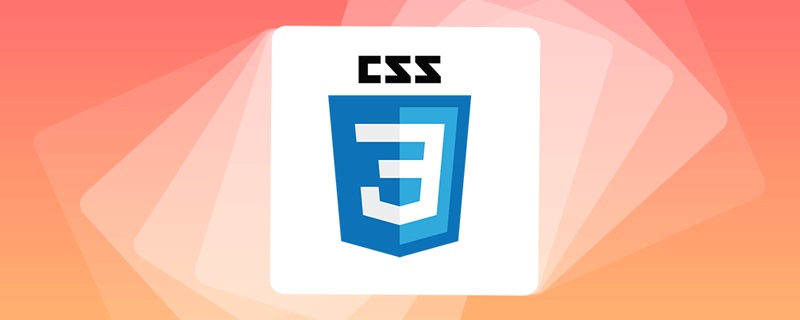
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
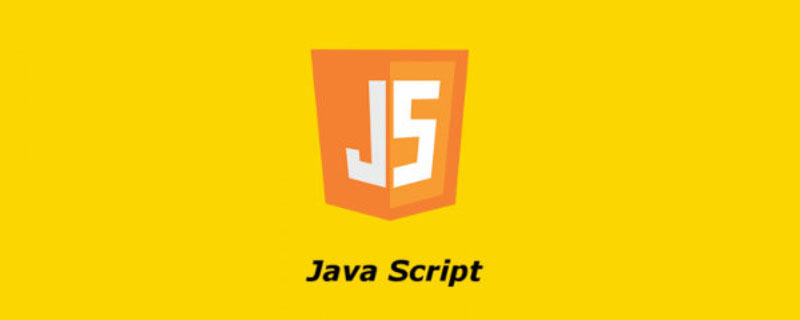
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
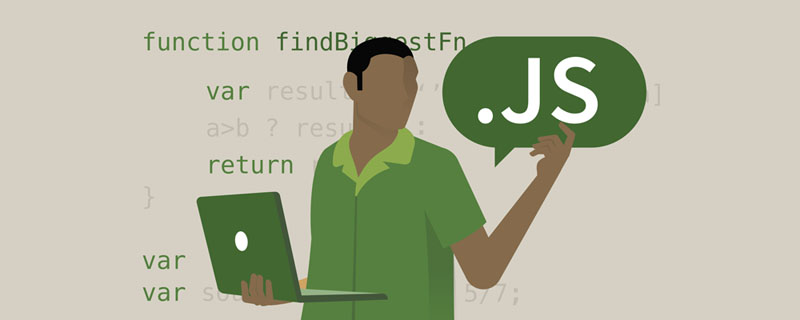
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
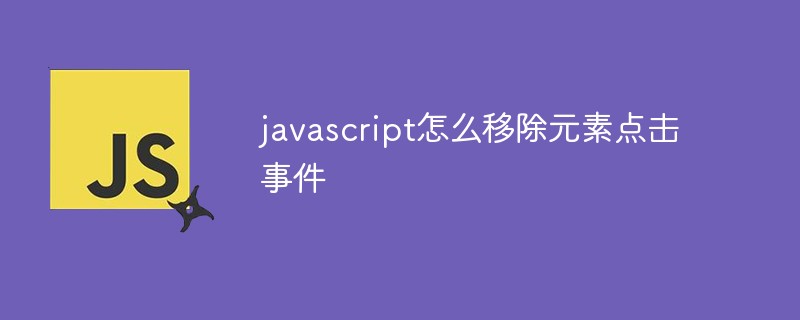
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
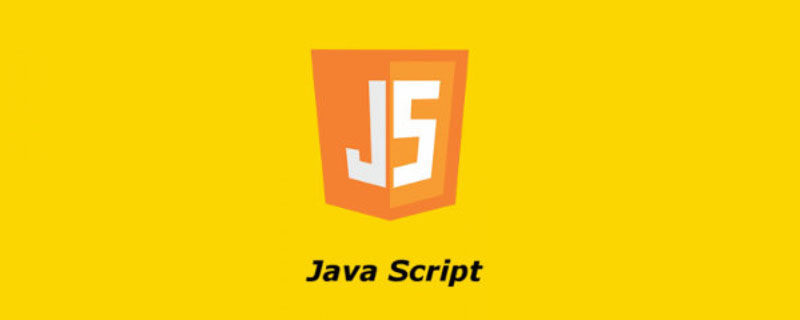
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
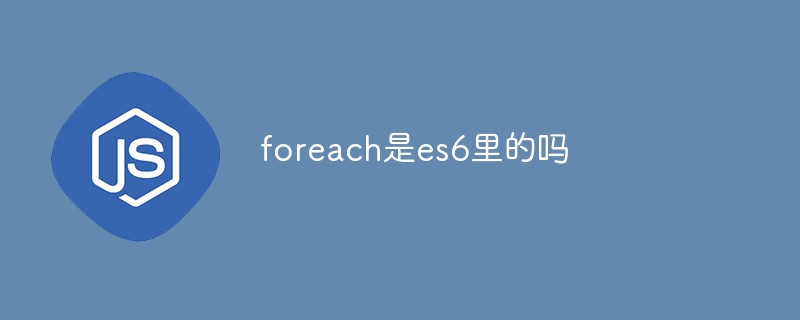
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
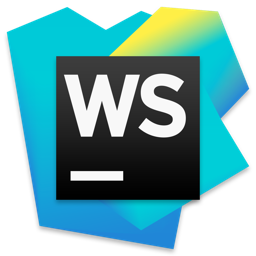
WebStorm Mac version
Useful JavaScript development tools
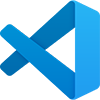
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
