This article mainly introduces the relevant knowledge of AJAX in JavaScript, which has a very good reference value. Let’s take a look at it with the editor
Abstract
AJAX technology is one of the necessary skills for web page construction. This article hopes to help everyone learn this technology easily
1. What is ajax?
ajax (asynchronous javascript xml) can refresh partial web page data instead of reloading the entire web page.
2. How to use ajax?
Step one: Create xmlhttprequest object
Create xmlhttprequest object, XMLHttpRequest object is used to exchange data with the server.
var xmlhttp =new XMLHttpRequest();
Step 2: Register the callback function
onreadystatechange function. When the server responds to the request and returns data, we want the client to process the data. We need to use the onreadystatechange function. The onreadystatechange function is triggered every time the readyState of the xmlhttprequest object changes. ReadyState will be introduced in detail in the next chapter.
xmlHttp.onreadystatechange= callback; function callback(){}
Step 3: Configure and send the request
Use the open() and send() methods of the xmlhttprequest object to configure and send the resource request to the server.
xmlhttp.open(method,url,async) method includes get and post, url is mainly the path of a file or resource, and the async parameter is true (representing asynchronous) or false (representing synchronization)
xmlhttp.send(); Use the get method to send a request to the server.
xmlhttp.send(string); Use the post method to send a request to the server.
Post form data needs to use the setRequestHeader method of the xmlhttprequest object to add an HTTP header.
When can post request be used?
(1)When updating a file or database.
(2) Send a large amount of data to the server because post request has no character limit.
(3) Send the encrypted data entered by the user.
xhttp.open("POST", "ajax_test.aspx", true); xhttp.setRequestHeader("Content-type", "application/x-www-form-urlencoded"); xhttp.send("fname=Henry&lname=Ford");
Step 4: Process the response data
Use the responseText or responseXML attribute of the xmlhttprequest object to obtain the server's response.
Use the responseText attribute to get the string data of the server response, and use the responseXML attribute to get the XML data of the server response.
Use readyState==4 and status==200 in the callback function to determine whether the interaction is over and whether the response is correct, and obtain the data returned by the server as needed to update the page content.
function callback(){ if(xmlHttp.readyState == 4){ //判断交互是否成功 if(xmlHttp.status == 200){ //获取服务器返回的数据 //获取纯文本数据 var responseText =xmlHttp.responseText; document.getElementById("info").innerHTML = responseText; } } }
3. Five states (readyState) during AJAX operation
In the actual operation of AJAX, accessing XMLHttpRequest (XHR) is not completed all at once. It is the result obtained after going through multiple states respectively. There are 5 states in AJAX. These five states are switched and set by the AJAX engine, respectively.
0: XHR is defined but has not been initialized
1: The send() method is called and the request is being sent. After the request is sent, it starts waiting to receive the response
2 : Response reception completed
3: Response content is being parsed
4: Response content parsing completed, returned to client call
For the above status, the "0" status It is a status value that is automatically acquired after definition. For the status of successful access (information obtained), we mostly use "4" to judge.
It is worth noting that every time the state is switched, the onreadystatechange event will be triggered, so the onreadystatechange event is triggered 5 times in the entire process
4. Advantages of AJAX Disadvantages
Advantages
1. The biggest point is that the page does not refresh and communicates with the server within the page without interruption. The user's operation has a more rapid response capability, giving the user a very good experience.
2. Reduce the burden on the server. The principle of ajax is to "fetch data on demand", which can minimize the burden on the server caused by redundant requests and responses.
Disadvantages
1. Ajax kills the back button, which destroys the browser's back mechanism.
2. The support for search engines is relatively weak.
The above is the detailed content of A simple understanding of JavaScript AJAX. For more information, please follow other related articles on the PHP Chinese website!
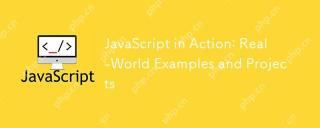
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
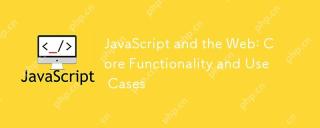
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
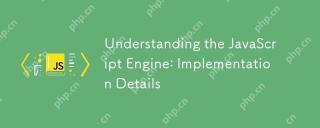
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
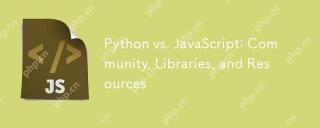
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
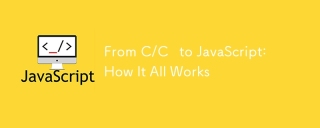
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
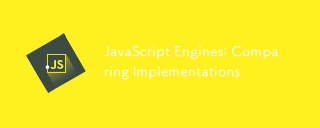
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
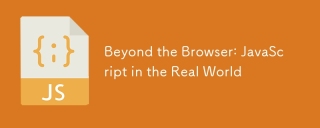
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Atom editor mac version download
The most popular open source editor
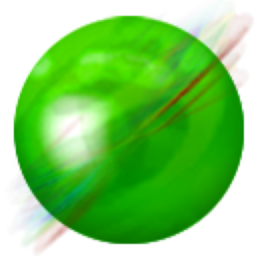
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment