


This article is the first article in the functional programming series. Here I will briefly introduce the programming paradigm and then jump straight into the concept of functional programming using Javascript, since JavsScript is one of the most recognized functional programming languages. Readers are encouraged to learn more about this fascinating concept through the References section.
Programming Paradigm
Programming paradigm is a framework composed of tools for thinking about problems and realizing the vision of the problem. Many modern languages are polyparadigm (or multi-paradigm): they support many different programming paradigms, such as object-oriented, metaprogramming, functional, procedural, etc.
Functional programming paradigm
Functional programming is like a hydrogen-powered car - advanced and futuristic, but not yet widely adopted . In contrast to imperative programming, it consists of a sequence of statements that update the global state at execution time. Functional programming turns calculations into expression evaluations. These expressions are all composed of pure mathematical functions, which are first-class (can be used and processed as normal values) and have no side effects.
Functional programming values the following values:
Functions are a first-class priority
We should treat functions with other elements in a programming language Class objects are treated similarly. In other words, you can store functions in variables, create functions dynamically, and return functions or pass functions to other functions. Let's look at an example...
A string can be saved as a variable, and functions can also be used, for example:
var sayHello = function() { return “Hello” };
A string can be saved as Object fields and functions can also be used, for example:
var person = {message: “Hello”, sayHello: function() { return “Hello” }};
A string can be created when it is used again, and functions can also be used, for example:
“Hello ” + (function() { return “World” })(); //=> Hello World
A string can be passed to the function as an input parameter , then the function can also be used:
function hellloWorld(hello, world) { return hello + world() }
A string can be used as the function return value, and the function can also be used, for example:
return “Hello”; return function() { return “Hello”};
Advanced case
If a function takes other functions as input parameters or as return values, it is called a higher-order function. We have just seen an example of a higher-order function. Next, let's look at a more complex situation.
Example 1:
[1, 2, 3].forEach(alert); // alert 弹窗显示“1" // alert 弹窗显示 "2" // alert 弹窗显示 "3”
Example 2:
function splat(fun) { return function(array) { return fun.apply(null, array); }; } var addArrayElements = splat(function(x, y) { return x + y }); addArrayElements([1, 2]); //=> 3
FavoritePure function
Pure function will not have other side effects. The so-called side effects refer to the modification of the external state of the function caused by the function. . For example:
Modify a certain variable
Modify the data structure
For an external variable Set a field
Throw an exception or pop up an error message
The simplest example is a mathematical function. The Math.sqrt(4) function always returns 2. It will not use any other chilling information, such as status or setting parameters. Mathematical functions never cause any side effects.
Avoid modifying state
Functional programming supports pure functions. Such functions cannot change data, so they are mostly used to create immutable data. In this way, there is no need to modify an existing data structure, and it can efficiently create a new one.
You may wonder, if a pure function produces an immutable return value by changing some local data, Is it allowed? The answer is yes.
Very few data types in JavaScript are immutable by default. String is an example of a data type that cannot be changed:
var s = "HelloWorld"; s.toUpperCase(); //=> "HELLOWORLD" s; //=> "HelloWorld"
Benefits of immutable state
• Avoid confusion and increase program accuracy: In complex systems, most of the Bugs are caused when state is modified by external client code within the program.
• Establish "fast and concise" multi-threaded programming: If multiple threads can modify the same shared value, you have to obtain the value synchronously. This is a tedious and error-prone programming challenge for experts.
Software transactional memory and the Actor model provide direct processing of modifications in a thread-safe manner.
Use recursion instead of loop calls
递归是最有名的函数式编程技术。如果您还不知道它的话,那么可以理解为递归函数就是一个可以调用自己的函数。
替代反复循环的最经典方式就是使用递归,即每次完成函数体操作之后,再继续执行集合里的下一项,直到满足结束条件。递归还天生符合某些算法实现,比如遍历树形结构(每个树枝都是一颗小树)。
在任何语言里,递归都是一项重要的函数式编程方式。很多函数语言甚至要求的更加严格:只支持递归遍历,而不支持显式的循环遍历。这需要语言必须保证消除了尾端调用,这是 JavasSrip 不支持的。
惰性求值优于激进计算
数学定义了很多无穷集合,比如自然数(所有的正整数)。他们都是符号表示。任意特定有限的子集都在需要时求值。我们将其称之为惰性求值(也叫做非严格求值,或者按需调用,延迟执行)。及早求值会强迫我们表示出所有无穷数据,而这显然是不可能的。
很多语言都默认是惰性的,有些也提供了惰性数据结构以表达无穷集合,并在需要时对自己进行精确计算。
很明显一行代码 result = compute() 所表达的是将 compute() 的返回结果赋值给 result。但是 result 的值究竟是多少只有其被用到的时候才有意义。
可见策略的选择会在很大程度上提高性能,特别是当用在链式处理或者数组处理的时候。这些都是函数式程序员所喜爱的编程技术。
这就开创可很多可能性,包括并发执行,并行技术以及合成。
但是,有一个问题,JavaScrip 并不对自身进行惰性求值。话虽如此,Javascript 里的函数库可以有效地模拟惰性求值。
闭包的全部好处
所有的函数式语言都有闭包,然而这个语言特性经常被讨论得很神秘。闭包是一个函数,这个函数有着对内部引用的所有变量的隐式绑定。换句话说,该函数对它引用的变量封闭了一个上下文。JavaScript 中的闭包是能够访问父级作用域的函数,即使父级函数已经调用完毕。
function multiplier(factor) { return function(number) { return number * factor; }; } var twiceOf = multiplier(2); console.log(twiceOf(6)); //=> 12
声明式优于命令式编程
函数式编程是声明式的,就像数学运算,属性和关系是定义好的。运行时知道怎么计算最终结果。阶乘函数的定义提供了一个例子:
factorial(n) = 1 if n = 1
n * factorial(n-1) if n > 1
该定义将 factorial(n) 的值关联到 factorial(n-1),是递归定义。特殊情况下的 factorial(1) 终止了递归。
var imperativeFactorial = function(n) { if(n == 1) { return 1 } else { product = 1; for(i = 1; i <= n; i++) { product *= i; } return product; } } var declarativeFactorial = function(n) { if(n == 1) { return 1 } else { return n * factorial(n - 1); } }
从它实现阶乘计算来看,声明式的阶乘可能看起来像“命令式”的,但它的结构更像声明式的。
命令式阶乘使用可变值、循环计数器和结果来累加计算后的结果。这个方法显式地实现了特定的算法。不像声明式版本,这种方法有许多可变步骤,导致它更难理解,也更难避免 bug 。
函数式JavaScript库
有很多函数式库:underscore.js, lodash,Fantasy Land, Functional.js, Bilby.js, fn.js, Wu.js, Lazy.js, Bacon.js, sloth.js, stream.js, Sugar, Folktale, RxJs 等等。
函数式程序员工具包
map(), filter(), 和 reduce()函数 构成了函数式程序员工具包的核心。 纯高阶函数成了函数式方法的主力。事实上,它们是纯函数和高阶函数应该仿效的典型。它们用一个函数作为输入,返回没有副作用的输出。
The above is the detailed content of Detailed introduction to functional programming using JavaScript (1) (picture). For more information, please follow other related articles on the PHP Chinese website!
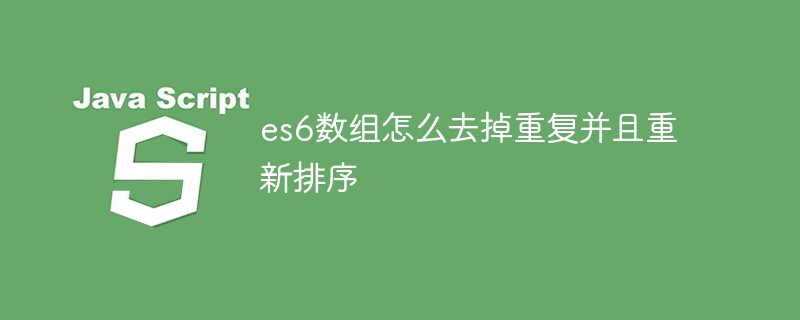
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
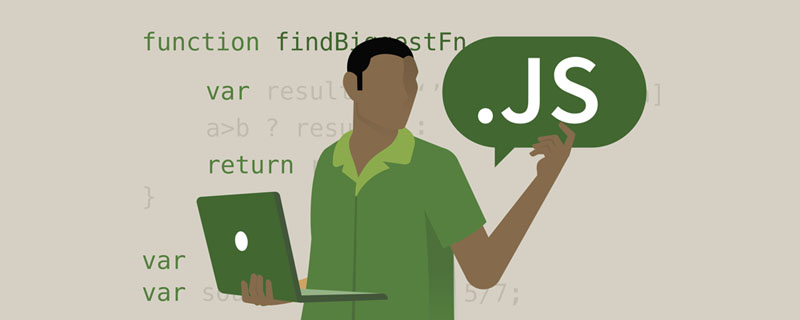
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
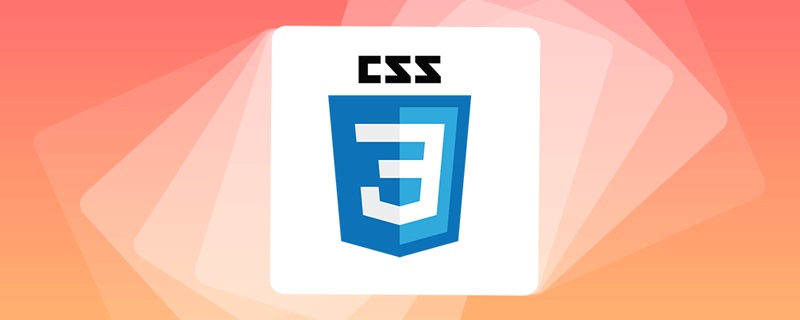
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
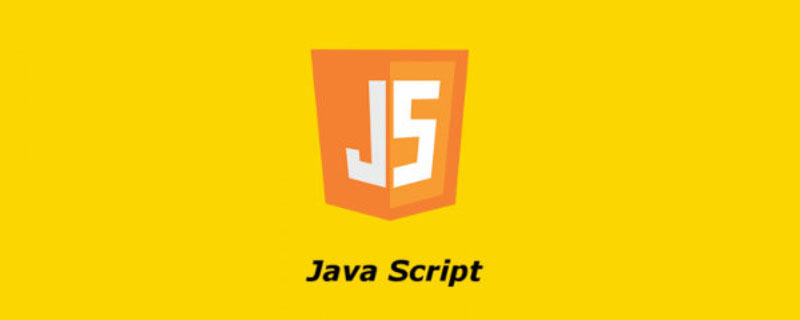
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
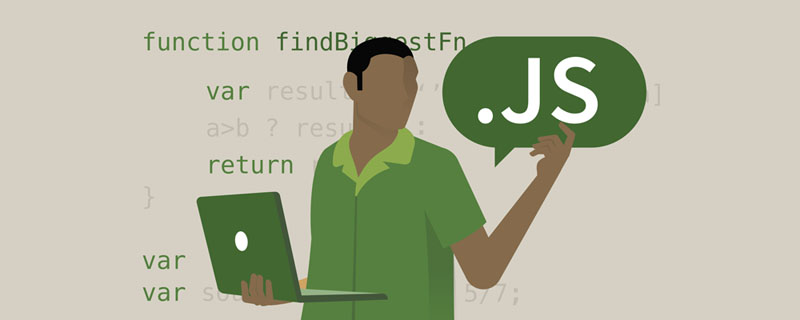
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
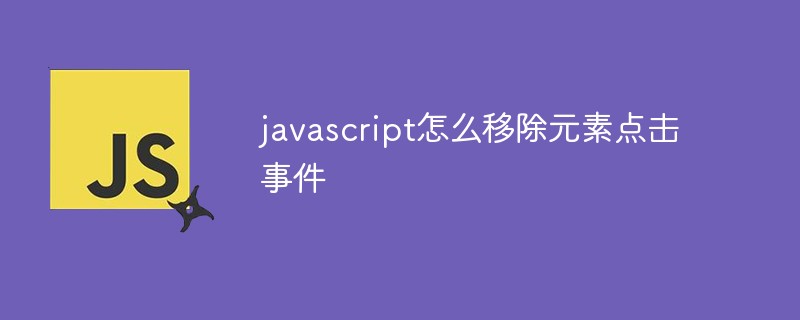
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
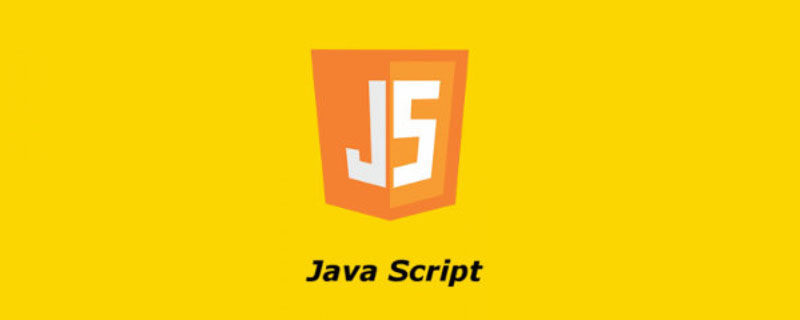
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
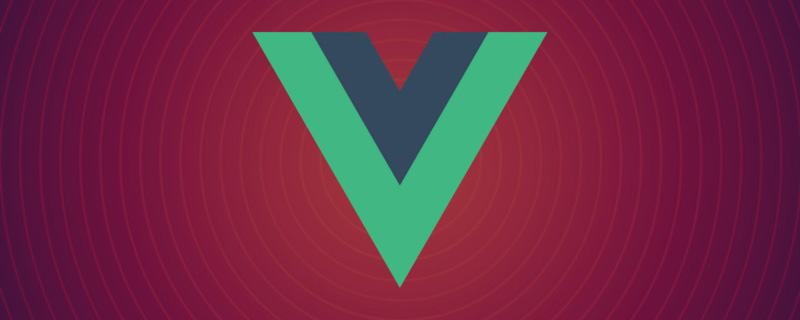
本篇文章整理了20+Vue面试题分享给大家,同时附上答案解析。有一定的参考价值,有需要的朋友可以参考一下,希望对大家有所帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
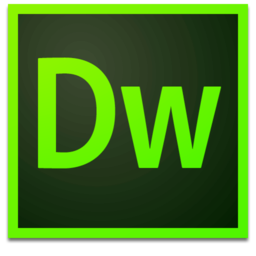
Dreamweaver Mac version
Visual web development tools
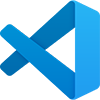
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
