1. Java’s own implementation
Class diagram
##
/** * 观察目标 继承自 java.util.Observable * @author stone * */ public class UpdateObservable extends Observable { private int data; public UpdateObservable(Observer observer) { addObserver(observer); /* * add other observer */ } public int getData() { return data; } public void setData(int data) { if (data != this.data) { this.data = data; setChanged(); //标记 改变, 只有标记后才能通知到 notifyObservers(); //通知 } } @Override public synchronized void addObserver(Observer o) { super.addObserver(o); } @Override public synchronized void deleteObserver(Observer o) { super.deleteObserver(o); } @Override public void notifyObservers() { super.notifyObservers(); } @Override public void notifyObservers(Object arg) { super.notifyObservers(arg); } @Override public synchronized void deleteObservers() { super.deleteObservers(); } @Override protected synchronized void setChanged() { super.setChanged(); } @Override protected synchronized void clearChanged() { super.clearChanged(); } @Override public synchronized boolean hasChanged() { return super.hasChanged(); } @Override public synchronized int countObservers() { return super.countObservers(); } }
/** * 观察者 实现 java.util.Observer接口 * @author stone * */ public class UpdateObserver implements Observer { @Override public void update(Observable o, Object arg) { System.out.println("接收到数据变化的通知:"); if (o instanceof UpdateObservable) { UpdateObservable uo = (UpdateObservable) o; System.out.print("数据变更为:" + uo.getData()); } } }
2. Customized observation model
/** * 抽象观察者 Observer * 观察 更新 * @author stone * */ public interface IWatcher { /* * 通知接口: * 1. 简单通知 * 2. 观察者需要目标的变化的数据,那么可以将目标用作参数, 见Java的Observer和Observable */ // void update(IWatched watched); void update(); }
/** * 抽象目标 Subject * 提供注册和删除观察者对象的接口, 及通知观察者进行观察的接口 * 及目标 自身被观察的业务的接口 * @author stone * */ public interface IWatchedSubject { public void add(IWatcher watch); public void remove(IWatcher watch); public void notifyWhatchers(); public void update();//被观察业务变化的接口 }
/** * 具体观察者 Concrete Observer * * @author stone * */ public class UpdateWatcher implements IWatcher { @Override public void update() { System.out.println(this + "观察到:目标已经更新了"); } }
/** * 具体目标角色 Concrete Subject * @author stone * */ public class UpdateWatchedSubject implements IWatchedSubject { private List<IWatcher> list; public UpdateWatchedSubject() { this.list = new ArrayList<IWatcher>(); } @Override public void add(IWatcher watch) { this.list.add(watch); } @Override public void remove(IWatcher watch) { this.list.remove(watch); } @Override public void notifyWhatchers() { for (IWatcher watcher : list) { watcher.update(); } } @Override public void update() { System.out.println("目标更新中...."); notifyWhatchers(); } }The listener is an implementation of the observerClass diagram
/** * 监听 用户在注册后 * @author stone * */ public interface IRegisterListener { void onRegistered(); }
/** * 监听 当用户登录后 * @author stone * */ public interface ILoginListener { void onLogined(); }
/* * 监听器 是观察者模式的一种实现 * 一些需要监听的业务接口上添加 监听器,调用监听器的相应方法,实现监听 */ public class User { public void register(IRegisterListener register) { /* * do ... register */ System.out.println("正在注册中..."); //注册后 register.onRegistered(); } public void login(ILoginListener login) { /* * do ... login */ System.out.println("正在登录中..."); //登录后 login.onLogined(); } }
/** * 观察者(Observer)模式 行为型模式 * 观察者模式定义了一种一对多的依赖关系,让多个观察者对象同时观察某一个目标对象。 * 这个目标对象在状态上发生变化时,会通知所有观察者对象,让它们能够自动更新自己 * 目标对象中需要有添加、移除、通知 观察者的接口 * * @author stone */ public class Test { public static void main(String[] args) { /* * 使用Java自带的Observer接口和Observable类 */ UpdateObservable observable = new UpdateObservable(new UpdateObserver()); observable.setData(99); System.out.println(""); System.out.println(""); /* * 自定义的观察者模型 */ IWatchedSubject watched = new UpdateWatchedSubject(); watched.add(new UpdateWatcher()); watched.add(new UpdateWatcher()); watched.update(); System.out.println(""); /* * 子模式-监听器 */ User user = new User(); user.register(new IRegisterListener() { @Override public void onRegistered() { System.out.println("监听到注册后。。。"); } }); user.login(new ILoginListener() { @Override public void onLogined() { System.out.println("监听到登录后。。。"); } }); } }Print
接收到数据变化的通知: 数据变更为:99 目标更新中.... observer.UpdateWatcher@457471e0观察到:目标已经更新了 observer.UpdateWatcher@5fe04cbf观察到:目标已经更新了 正在注册中... 监听到注册后。。。 正在登录中... 监听到登录后。。。
The above is the detailed content of Java implementation of Observer pattern instance details (picture). For more information, please follow other related articles on the PHP Chinese website!
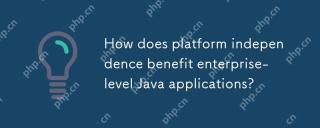
Java is widely used in enterprise-level applications because of its platform independence. 1) Platform independence is implemented through Java virtual machine (JVM), so that the code can run on any platform that supports Java. 2) It simplifies cross-platform deployment and development processes, providing greater flexibility and scalability. 3) However, it is necessary to pay attention to performance differences and third-party library compatibility and adopt best practices such as using pure Java code and cross-platform testing.
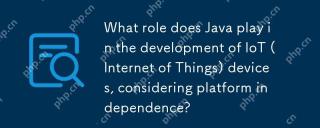
JavaplaysasignificantroleinIoTduetoitsplatformindependence.1)Itallowscodetobewrittenonceandrunonvariousdevices.2)Java'secosystemprovidesusefullibrariesforIoT.3)ItssecurityfeaturesenhanceIoTsystemsafety.However,developersmustaddressmemoryandstartuptim
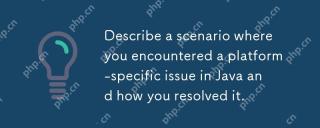
ThesolutiontohandlefilepathsacrossWindowsandLinuxinJavaistousePaths.get()fromthejava.nio.filepackage.1)UsePaths.get()withSystem.getProperty("user.dir")andtherelativepathtoconstructthefilepath.2)ConverttheresultingPathobjecttoaFileobjectifne
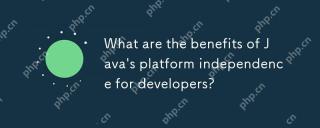
Java'splatformindependenceissignificantbecauseitallowsdeveloperstowritecodeonceandrunitonanyplatformwithaJVM.This"writeonce,runanywhere"(WORA)approachoffers:1)Cross-platformcompatibility,enablingdeploymentacrossdifferentOSwithoutissues;2)Re
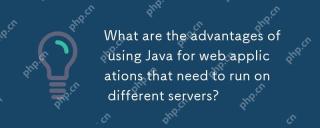
Java is suitable for developing cross-server web applications. 1) Java's "write once, run everywhere" philosophy makes its code run on any platform that supports JVM. 2) Java has a rich ecosystem, including tools such as Spring and Hibernate, to simplify the development process. 3) Java performs excellently in performance and security, providing efficient memory management and strong security guarantees.
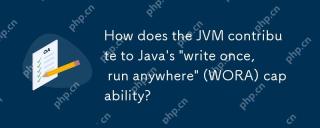
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
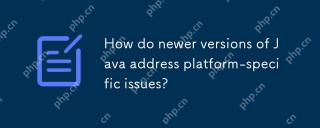
The latest version of Java effectively solves platform-specific problems through JVM optimization, standard library improvements and third-party library support. 1) JVM optimization, such as Java11's ZGC improves garbage collection performance. 2) Standard library improvements, such as Java9's module system reducing platform-related problems. 3) Third-party libraries provide platform-optimized versions, such as OpenCV.
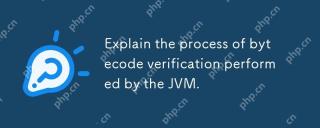
The JVM's bytecode verification process includes four key steps: 1) Check whether the class file format complies with the specifications, 2) Verify the validity and correctness of the bytecode instructions, 3) Perform data flow analysis to ensure type safety, and 4) Balancing the thoroughness and performance of verification. Through these steps, the JVM ensures that only secure, correct bytecode is executed, thereby protecting the integrity and security of the program.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
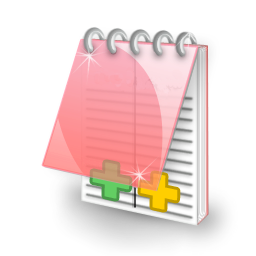
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
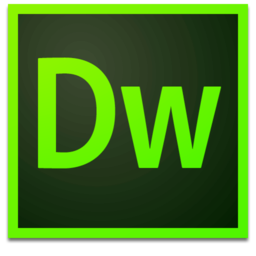
Dreamweaver Mac version
Visual web development tools
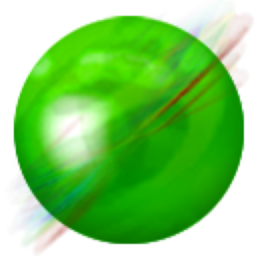
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
