


Time module method:
time.time(): Get the timestamp of the current time
time.localtime(): Accept a timestamp , and convert it into a tuple of the current time. If no parameters are given, time.time() will be passed in as a parameter by default
Attribute | Meaning | |
tm_year | 年 | |
tm_mon | 月 | |
tm_mday | 日 | |
tm_hour | hour | |
tm_min | minutes | |
tm_sec | seconds | |
tm_wday | Day of the week | |
tm_yday | Day of the year | |
tm_isdst | Daylight Saving Time |
- time.mktime(): Contrary to time.localtime(), it converts a time tuple into a timestamp (this must be given a parameter)
- time.asctime(): Represents a time tuple in the format of "Sun Jul 28 03:35:26 2013". If no parameters are given, time.localtime() will be passed in as a parameter by default
- time.ctime(): Convert a timestamp to the expression format of time.asctime(). If no parameters are given, time.time() will be passed in as a parameter by default
- time.gmtime(): Convert a timestamp to a time tuple in UTC+0 time zone (China should be +8 time zone, a difference of 8 hours). If no parameters are given, it will default to time.time() is passed in as a parameter
- time.strftime(format, time.localtime()): Convert a time tuple into a formatted time character, without giving a time For tuple parameters, time.localtime() will be passed in as a parameter by default
Sun Jul 28 04:37:38 2013format:
Format | Meaning | Value range (format) | |
%y | The year minus the century | 00-99 | |
Complete year | |||
Day of the year | 001-366 | ||
%m | Month | January 12th | |
Local simplified month name | Abbreviated English month | ##%B | |
Full English month | Date | ||
Day of the month | 1 month 31st | ##hour | |
The hour of the day (24-hour clock) | 00-23 | %l | |
“01-12” | Minutes | ||
Minutes | 00-59 | Seconds | |
Seconds | 00-59 | Week | |
The number of weeks in the year (from Starting from Sunday) | 00-53 | %W | |
%w |
|||
0-6 | Time Zone | ||
China: It should be GMT+8 (China Standard Time) | Please educate yourself | Others | |
Local corresponding date | 日/month/year | %X | |
Hour:Minute:Second | %c | ||
Day/Month/Year Hour:Minute:Second | %% | ||
'%' character | %p | ||
AM or PM |
time.strptime('28/Jul/2013:04:33:29', '%d/%b/%Y:%X') 返回结果: 复制代码 代码如下: time.struct_time(tm_year=2013, tm_mon=7, tm_mday=28, tm_hour=4, tm_min=33, tm_sec=29, tm_wday=6, tm_yday=209, tm_isdst=-1) time.clock():返回处理器时钟时间,一般用于性能测试和基准测试等,因为他们反映了程序使用的实际时间,平常用不到这个。 time.sleep():推迟指定的时间运行,单位为秒 import time print time.time() #打印时间戳 print time.localtime()#打印本地时间元组 print time.gmtime()#答应UTC+0时区的时间元组 print time.ctime()#打印asctime格式化时间 print time.mktime(time.localtime())#将时间元组转换为时间戳 print time.asctime()#打印格式化时间 print time.strftime('%d/%b/%Y:%X')#打印指定格式的时间格式 #把时间字符串和它的格式翻译成时间元组 print time.strptime('28/Jul/2013:04:33:29', '%d/%b/%Y:%X') print '%0.5f'%time.clock() #打印处理器时间 for i in range(100000): pass print '%0.5f'%time.clock()#打印处理器时间 来看一下结果: [root@localhost ~]# python time1.py 1364028568.55 time.struct_time(tm_year=2013, tm_mon=3, tm_mday=23, tm_hour=4, tm_min=49, tm_sec=28, tm_wday=5, tm_yday=82, tm_isdst=1) time.struct_time(tm_year=2013, tm_mon=3, tm_mday=23, tm_hour=8, tm_min=49, tm_sec=28, tm_wday=5, tm_yday=82, tm_isdst=0) Sat Mar 23 04:49:28 2013 1364028568.0 Sat Mar 23 04:49:28 2013 23/Mar/2013:04:49:28 time.struct_time(tm_year=2013, tm_mon=7, tm_mday=28, tm_hour=4, tm_min=33, tm_sec=29, tm_wday=6, tm_yday=209, tm_isdst=-1) 0.02000 0.03000 datetime模块 #coding:utf-8 import datetime print datetime.time() t = datetime.time(1, 3, 5, 25) print t print t.hour #时 print t.minute #分 print t.second #秒 print t.microsecond #毫秒 print datetime.time.max #一天的结束时间 print datetime.time.min #一天的开始时间 执行一下: 00:00:00 01:03:05.000025 23:59:59.999999 00:00:00 datetime.date():生成一个日期对象。这个日期要由我们来设置,(这个类只针对日期) #coding:utf-8 import datetime #设置日期 t = datetime.date(2013, 2, 3) #打印设置日期的和元组 print t.timetuple()#日期元组 print t print t.year #年 print t.month #月 print t.day #日 #获取今天的日期 today = datetime.date.today() print today print datetime.datetime.now()#这个打印到毫秒级别 #获取今天日期的元组 t1 = today.timetuple() print t1 #打印成ctime格式(time.ctime()格式) #'%a %b %d %H:%M:%S %Y' print t.ctime() print today.ctime() 运行结果 time.struct_time(tm_year=2013, tm_mon=2, tm_mday=3, tm_hour=0, tm_min=0, tm_sec=0, tm_wday=6, tm_yday=34, tm_isdst=-1) 2013-02-03 2013 2 3 2013-07-28 2013-07-28 20:13:25.942000 time.struct_time(tm_year=2013, tm_mon=7, tm_mday=28, tm_hour=0, tm_min=0, tm_sec=0, tm_wday=6, tm_yday=209, tm_isdst=-1) Sun Feb 3 00:00:00 2013 Sun Jul 28 00:00:00 2013 datetime.timedelta():这个类用来做时间的算数运算 #coding:utf-8 import datetime #打印:从毫秒到周的表示格式 = 转换成秒 (total_seconds()) for i in [datetime.timedelta(milliseconds=1), #1毫秒 datetime.timedelta(seconds=1), #1秒 datetime.timedelta(minutes=1), #1分钟 datetime.timedelta(hours=1), #1小时 datetime.timedelta(days=1), #1天 datetime.timedelta(weeks=1)]:#11周 #print i + ':' + i.total_seconds() print '%s = %s seconds'%(i,i.total_seconds()) print print '~' * 20 + '我是分割线' + '~' * 20 print '计算时间的加减。。。。。。。。。' a = datetime.datetime.now() print '现在时间是:' print a print '加5小时之后变成:' b = a + datetime.timedelta(hours=5) print b print '加一周之后变成:' c = a + datetime.timedelta(weeks=1) print c print '减去一周后变成:' d = a - datetime.timedelta(weeks=1) print d print '计算2个时间相差多久' print '%s减去%s'%(b, a) print '等于:%s'%(b - a) print '%s减去%s'%(a, d) print '等于:%s'%(a - d) print print '~' * 20 + '我是分割线' + '~' * 20 print '比较2个时间:' print '比较当天和一周前的' print a > d print '如果比较d > a 的话就返回False' print print '~' * 20 + '我是分割线' + '~' * 20 print '上面的列子都是把日期和时间分开的,现在我们来把他们自由结合' print '假设我们想要的时间是:2014-01-05 13:14:25' t = datetime.time(13, 14, 25) d = datetime.date(2014, 01, 05) print datetime.datetime.combine(d, t) 打印为: 0:00:00.001000 = 0.001 seconds 0:00:01 = 1.0 seconds 0:01:00 = 60.0 seconds 1:00:00 = 3600.0 seconds 1 day, 0:00:00 = 86400.0 seconds 7 days, 0:00:00 = 604800.0 seconds 计算时间的加减。。。。。。。。。 现在时间是: 2013-07-28 21:34:33.531000 加5小时之后变成: 2013-07-29 02:34:33.531000 加一周之后变成: 2013-08-04 21:34:33.531000 减去一周后变成: 2013-07-21 21:34:33.531000 计算2个时间相差多久 2013-07-29 02:34:33.531000减去2013-07-28 21:34:33.531000 等于:5:00:00 2013-07-28 21:34:33.531000减去2013-07-21 21:34:33.531000 等于:7 days, 0:00:00 比较2个时间: 比较当天和一周前的 True 如果比较d > a 的话就返回False 上面的列子都是把日期和时间分开的,现在我们来把他们自由结合 假设我们想要的时间是:2014-01-05 13:14:25 2014-01-05 13:14:25 更多Python中time模块和datetime模块的用法示例分析相关文章请关注PHP中文网! |
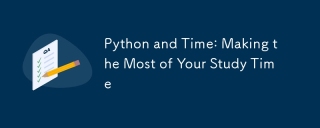
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
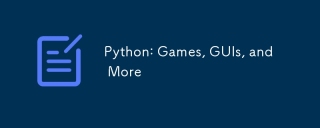
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
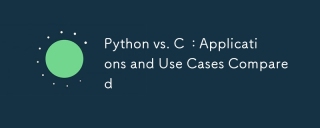
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
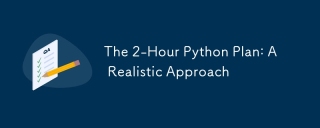
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
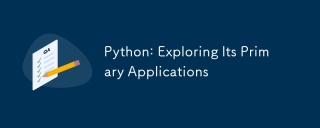
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
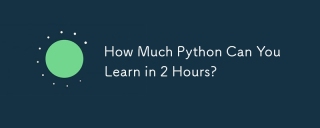
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
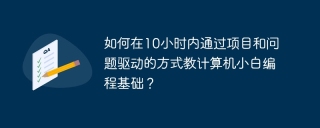
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
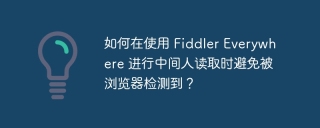
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
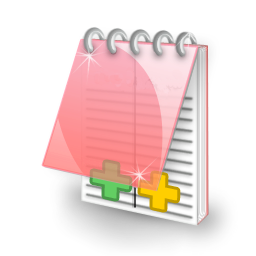
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
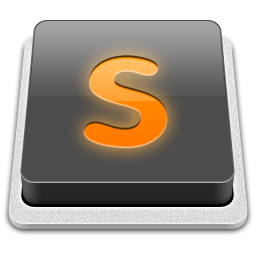
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.