


Python’s magic methods are those predefined functions in Python like __XXX__
.
The biggest advantage of using Python's magic methods is that python provides simple methods to allow objects to behave like built-in types.
__str__ function
__str__
The function is used to process the output content when printing the instance itself. If this function is not overridden, an object name and memory address are output by default.
For example:
>>> class Student(object): ... def __init__(self,name): ... self._name = name ... >>> print Student()
Output: <__main__.student object at></__main__.student>
.
So how do we make the output results more readable? We can override the __str__
function. For example, the output result of
>>> class Student(object): ... def __init__(self, name): ... self._name = name ... def __str__(self): ... return "I'm a student, named %s" % self._name ... >>> print Student("Charlie")
is: I'm a student, named Charlie
.
When we apply the str()
function to the object, in fact It is the __str__
function that calls the object.
_repr_ Function
__repr__
also serializes objects, but __repr__
is more for the python compiler watch. __str__
It’s more about readability.
When we use the repr()
function to touch an object, what we call is actually the __repr__
function of the function.
Paired with repr()
is the eval()
function. eval()
The function is to convert the serialized object back into an object. The premise is that the object implements the __repr__
function.
The above paragraph is based on my own understanding, I don’t know whether it is right or wrong.
>>> item = [1,2,3] >>> repr(item) '[1, 2, 3]' >>> other_item = eval(repr(item)) >>> other_item[1] 2
__iter__ function
We often use for...in... to iterate on lists or tuples. That is list inherits from Iterable. Iterable implements the __iter__ function.
If you want to turn a custom object into an iterable object, you must implement two methods: __iter__
and next
.
__iter__
The function returns an object. When iterating, the next
function will be continuously called to get the next value until StopIteration
is captured.
Teacher Liao Xuefeng’s tutorial writes the __next__
method, I don’t know why.
class Fib(object): def __init__(self): self.a, self.b = 0, 1 def __iter__(self): return self def next(self): self.a, self.b = self.b, self.a + self.b if self.a > 10000: raise StopIteration return self.a for i in Fib(): print i
__getitem__ function
The above implements the iteration of objects by implementing the __iter__
function.
So how to implement the object to extract elements by subscript.
This is achieved by implementing the __getitem__
method of the object.
Let’s give one
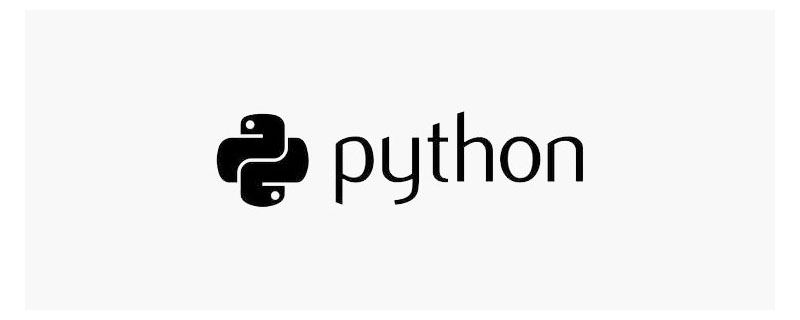
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
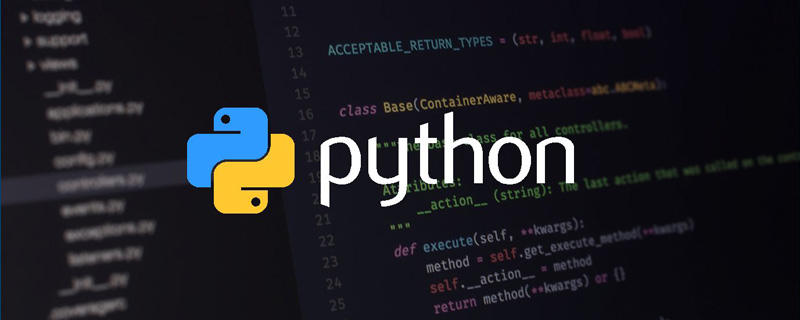
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
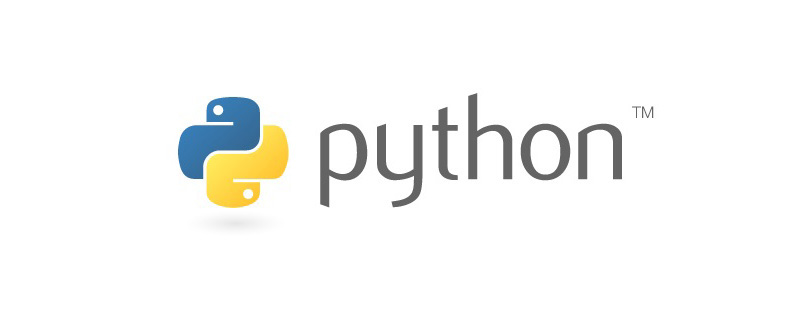
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
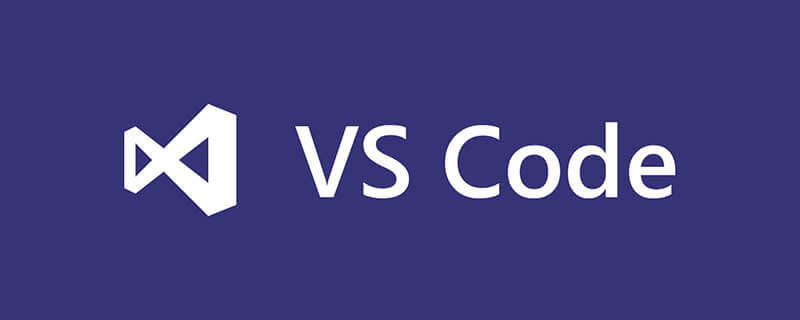
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
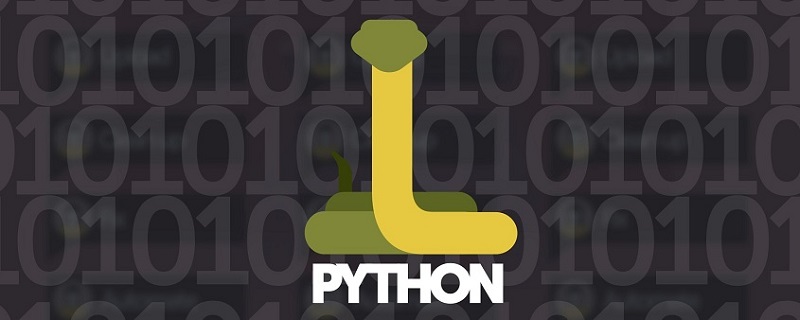
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
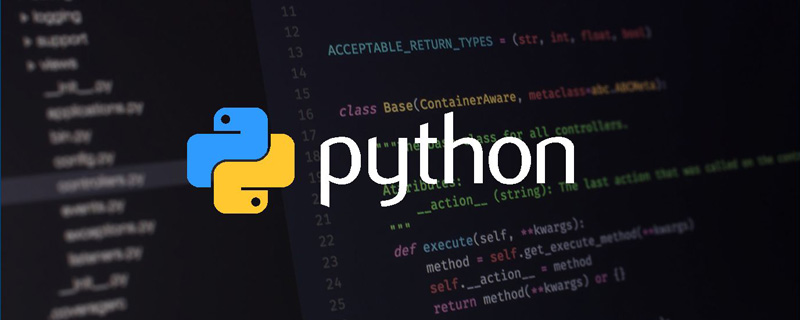
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。
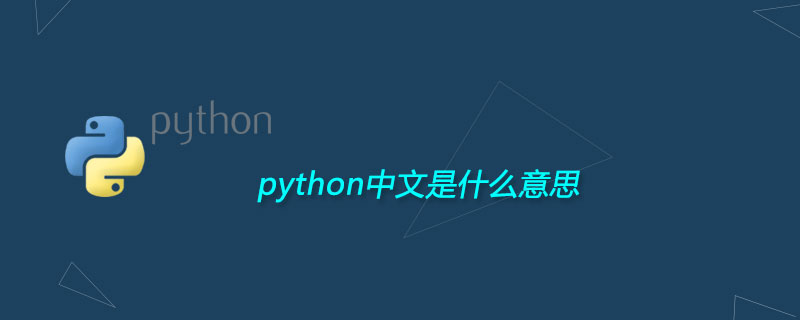
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version
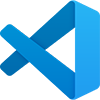
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor
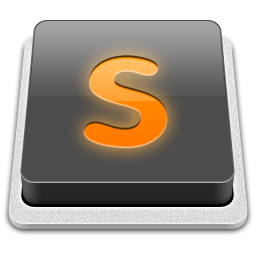
SublimeText3 Mac version
God-level code editing software (SublimeText3)
