The only difference between a tuple and a list is that the list can be changed, but the tuple cannot be changed. Other functions are the same as the list
Two ways to create a tuple
One
ages = (11, 22, 33, 44, 55)
The second
ages = tuple((11, 22, 33, 44, 55))
If there is only one element in the ancestor, then a comma needs to be added, otherwise it will become a string.
In [1]: t = (1) In [2]: t Out[2]: 1 In [3]: type(t) Out[3]: int In [4]: t = (1,) In [5]: t Out[5]: (1,) In [6]: type(t) Out[6]: tuple
Methods possessed by tuples
View the number of occurrences of elements in the list
count(self, value):
Attribute | Description |
---|---|
value | The value of the element |
>>> ages = tuple((11, 22, 33, 44, 55)) >>> ages (11, 22, 33, 44, 55) >>> ages.count(11) 1
Find the position of the element in the tuple
index(self, value, start=None, stop=None):
Attribute | Description |
---|---|
The value of the element | |
Starting position | |
Ending position |
>>> T = (1,2,3,4,5) >>> (x * 2 for x in T) <generator> at 0x102a3e360> >>> T1 = (x * 2 for x in T) >>> T1 <generator> at 0x102a3e410> >>> for t in T1: print(t) ... 2 4 6 8 10</generator></generator>Tuple nesting modificationThe elements of the tuple are unchangeable, but the elements of the tuple elements may be changeable The
>>> tup=("tup",["list",{"name":"ansheng"}]) >>> tup ('tup', ['list', {'name': 'ansheng'}]) >>> tup[1] ['list', {'name': 'ansheng'}] >>> tup[1].append("list_a") >>> tup[1] ['list', {'name': 'ansheng'}, 'list_a']The element of the tuple itself cannot be modified, but if the element of the tuple is a list or dictionary, it can be modifiedSlices modify immutable types in place
>>> T = (1,2,3)
>>> T = T[:2] + (4,)
>>> T
(1, 2, 4)
For more related articles on the Tuple Data Type of the Python Full Stack Road Series, please pay attention to the PHP Chinese website!
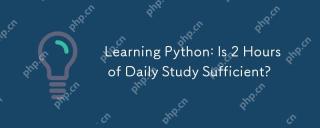
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
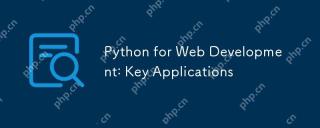
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
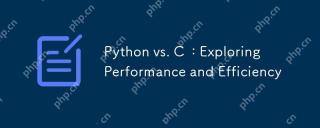
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
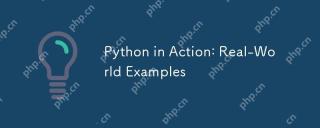
Python's real-world applications include data analytics, web development, artificial intelligence and automation. 1) In data analysis, Python uses Pandas and Matplotlib to process and visualize data. 2) In web development, Django and Flask frameworks simplify the creation of web applications. 3) In the field of artificial intelligence, TensorFlow and PyTorch are used to build and train models. 4) In terms of automation, Python scripts can be used for tasks such as copying files.
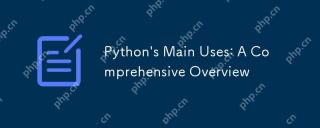
Python is widely used in data science, web development and automation scripting fields. 1) In data science, Python simplifies data processing and analysis through libraries such as NumPy and Pandas. 2) In web development, the Django and Flask frameworks enable developers to quickly build applications. 3) In automated scripts, Python's simplicity and standard library make it ideal.
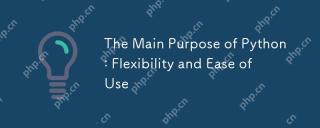
Python's flexibility is reflected in multi-paradigm support and dynamic type systems, while ease of use comes from a simple syntax and rich standard library. 1. Flexibility: Supports object-oriented, functional and procedural programming, and dynamic type systems improve development efficiency. 2. Ease of use: The grammar is close to natural language, the standard library covers a wide range of functions, and simplifies the development process.
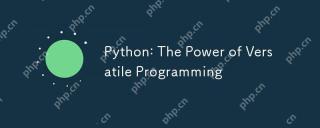
Python is highly favored for its simplicity and power, suitable for all needs from beginners to advanced developers. Its versatility is reflected in: 1) Easy to learn and use, simple syntax; 2) Rich libraries and frameworks, such as NumPy, Pandas, etc.; 3) Cross-platform support, which can be run on a variety of operating systems; 4) Suitable for scripting and automation tasks to improve work efficiency.
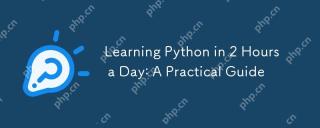
Yes, learn Python in two hours a day. 1. Develop a reasonable study plan, 2. Select the right learning resources, 3. Consolidate the knowledge learned through practice. These steps can help you master Python in a short time.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor
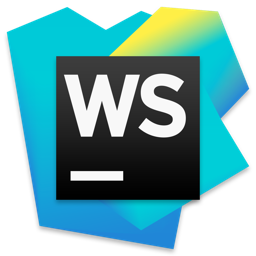
WebStorm Mac version
Useful JavaScript development tools
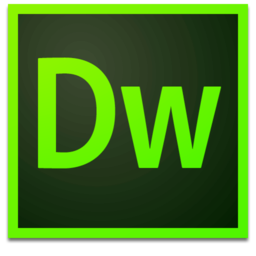
Dreamweaver Mac version
Visual web development tools
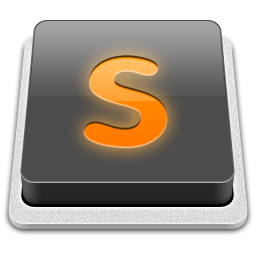
SublimeText3 Mac version
God-level code editing software (SublimeText3)