


As mentioned in inheritance and abstract classes, there are these relationships between the methods of subclasses and parent classes:
Subclasses directly use parent class methods (but the parent class methods must be public or protected types);
The method of the subclass overrides the method of the parent class (override);
The method of the subclass overrides the method of the parent class (overload);
Look at the following situation:
public class YSchool { private int id = 0; private string name = string.Empty; public int ID { get { return this.id; } } public string Name { get { return name; } } public YSchool() { this.id = 0; this.name = @"清华大学附中"; } public YSchool(int id, string name) { this.id = id; this.name = name; } /// <summary> /// 构造器 /// </summary> public YSchool(int id) { this.id = id; this.name = @"陕师大附中"; } } public class YTeacher { private int id = 0; private string name = string.Empty; private YSchool school = null; private string introDuction = string.Empty; private string imagePath = string.Empty; public int ID { get { return id; } } public string Name { get { return name; } } public YSchool School { get { if (school == null) { school = new YSchool(); } return school; } set { school = value; } } public string IntroDuction { get { return introDuction; } set { introDuction = value; } } public string ImagePath { get { return imagePath; } set { imagePath = value; } } /// <summary> /// 构造器 /// </summary> public YTeacher(int id, string name) { this.id = id; this.name = name; } /// <summary> /// 构造器 /// </summary> public YTeacher(int id, string name, YSchool school) { this.id = id; this.name = name; this.school = school; } /// <summary> /// 给学生讲课的方法 /// </summary> public void ToTeachStudents() { Console.WriteLine(string.Format(@"{0} 老师教育同学们: Good Good Study,Day Day Up!", this.Name)); } /// <summary> /// 惩罚犯错误学生的方法 /// 加virtual关键字,表示该方法可以被覆盖重写 /// </summary> /// <param name="punishmentContent"></param> public virtual void PunishmentStudents(string punishmentContent) { Console.WriteLine(string.Format(@"{0} 的{1} 老师让犯错误的学生 {2}。", this.School.Name, this.name, punishmentContent)); } } public class UniversityTeacher : YTeacher { public UniversityTeacher(int id, string name,YSchool school) : base(id, name, school) { } /// <summary> /// 隐藏父类的同名方法,隐藏后该类只能访问隐藏后的方法,不能访问到父类的该方法了。 /// </summary> public new void ToTeachStudents() { Console.WriteLine(string.Format(@"{0} 老师教育同学们:认真学习.net!", this.Name)); } /// <summary> /// 覆盖 /// </summary> public override void PunishmentStudents(string punishmentContent) { base.PunishmentStudents(punishmentContent);//也可以不执行父类方法。 //自己的代码 } }
using System; namespace YYS.CSharpStudy.MainConsole { class Program { static void Main(string[] args) { UniversityTeacher uTeacher = new UniversityTeacher(3, @"董边成", new YSchool(3, @"清华大学")); //访问的是子类方法 uTeacher.ToTeachStudents(); //可以访问覆盖后的方法 uTeacher.PunishmentStudents(@"让你挂科。"); YTeacher teacher = new UniversityTeacher(3, @"董边成", new YSchool(3, @"清华大学")); //访问不到隐藏的那个方法了 teacher.ToTeachStudents(); //可以访问覆盖后的方法 teacher.PunishmentStudents(@"跑10000米。"); Console.ReadKey(); } } }
Result:
The process of using new to modify a new method with the same name and parameter list as the parent class method is called hiding. That is, the subclass hides this method of the parent class. However, hiding is different from overwriting. A hidden method can only be accessed through the class in which the method is located. If you use variables of the parent class, you will still access the hidden method.
As you can see from the above code, the difference between covering and hiding. After the parent class variable refers to the subclass instance, only the hidden method can be accessed, but the hidden method cannot be accessed. However, the overridden methods can be accessed.
Another point is that if you want this method to be overridden by the subclass, then the parent class must add virtual to the method. You don’t need to add the new keyword to hide the parent class’s method. Hide is generally used less and solves some problems in some special situations.
The above is the summary of C# basic knowledge: the hidden content of basic knowledge (7) method. For more related content, please pay attention to the PHP Chinese website (www.php.cn)!
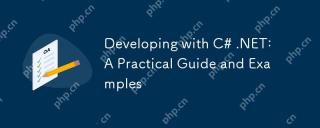
C# and .NET provide powerful features and an efficient development environment. 1) C# is a modern, object-oriented programming language that combines the power of C and the simplicity of Java. 2) The .NET framework is a platform for building and running applications, supporting multiple programming languages. 3) Classes and objects in C# are the core of object-oriented programming. Classes define data and behaviors, and objects are instances of classes. 4) The garbage collection mechanism of .NET automatically manages memory to simplify the work of developers. 5) C# and .NET provide powerful file operation functions, supporting synchronous and asynchronous programming. 6) Common errors can be solved through debugger, logging and exception handling. 7) Performance optimization and best practices include using StringBuild
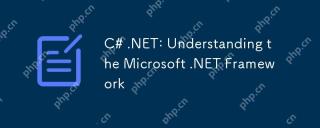
.NETFramework is a cross-language, cross-platform development platform that provides a consistent programming model and a powerful runtime environment. 1) It consists of CLR and FCL, which manages memory and threads, and FCL provides pre-built functions. 2) Examples of usage include reading files and LINQ queries. 3) Common errors involve unhandled exceptions and memory leaks, and need to be resolved using debugging tools. 4) Performance optimization can be achieved through asynchronous programming and caching, and maintaining code readability and maintainability is the key.
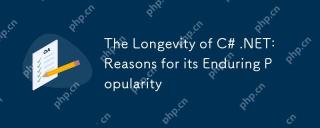
Reasons for C#.NET to remain lasting attractive include its excellent performance, rich ecosystem, strong community support and cross-platform development capabilities. 1) Excellent performance and is suitable for enterprise-level application and game development; 2) The .NET framework provides a wide range of class libraries and tools to support a variety of development fields; 3) It has an active developer community and rich learning resources; 4) .NETCore realizes cross-platform development and expands application scenarios.
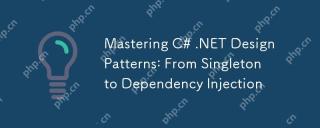
Design patterns in C#.NET include Singleton patterns and dependency injection. 1.Singleton mode ensures that there is only one instance of the class, which is suitable for scenarios where global access points are required, but attention should be paid to thread safety and abuse issues. 2. Dependency injection improves code flexibility and testability by injecting dependencies. It is often used for constructor injection, but it is necessary to avoid excessive use to increase complexity.
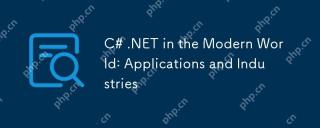
C#.NET is widely used in the modern world in the fields of game development, financial services, the Internet of Things and cloud computing. 1) In game development, use C# to program through the Unity engine. 2) In the field of financial services, C#.NET is used to develop high-performance trading systems and data analysis tools. 3) In terms of IoT and cloud computing, C#.NET provides support through Azure services to develop device control logic and data processing.
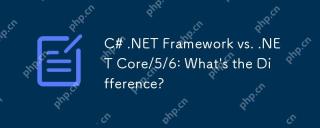
.NETFrameworkisWindows-centric,while.NETCore/5/6supportscross-platformdevelopment.1).NETFramework,since2002,isidealforWindowsapplicationsbutlimitedincross-platformcapabilities.2).NETCore,from2016,anditsevolutions(.NET5/6)offerbetterperformance,cross-
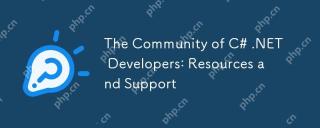
The C#.NET developer community provides rich resources and support, including: 1. Microsoft's official documents, 2. Community forums such as StackOverflow and Reddit, and 3. Open source projects on GitHub. These resources help developers improve their programming skills from basic learning to advanced applications.
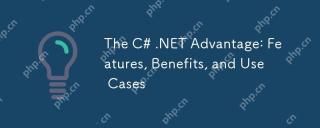
The advantages of C#.NET include: 1) Language features, such as asynchronous programming simplifies development; 2) Performance and reliability, improving efficiency through JIT compilation and garbage collection mechanisms; 3) Cross-platform support, .NETCore expands application scenarios; 4) A wide range of practical applications, with outstanding performance from the Web to desktop and game development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
