Requirements: This is the case. To develop a template for sending text messages, different customers may use different templates, and the variable parameters used by different customers are also different.
For emergency purposes, a text message template function for sending text messages has been completed online. The text message template table has also been created, and a new record has been added to the table. I only need to make an interface for adding, deleting, modifying and checking text message templates. It seems that my task is quite simple. An experienced driver should know that he has made a mess.
The picture below shows the originally created table
The SQL creation script is as follows:
Before this, an API interface for sending text messages has been developed for customers to call. In other words, the caller (customer) will not modify the code, only me. to modify. Although I am extremely reluctant to take on half-finished tasks, I have no choice but to start all development tasks from scratch.
The entity class code is as follows:
DOT class:
public class MessageModuleBusiness : GenericRepository<Model.MessageModule> { private UnitOfWork.UnitOfWork unitOfWork = new UnitOfWork.UnitOfWork(); /// 获取模版内容 public string GetContent(MessageContext messageContext) { string messageContent = ""; string TypeCode = string.IsNullOrEmpty(messageContext.serviceCode) ? "001" : messageContext.serviceCode; try { var Module = unitOfWork.MessageModule.Get(c => c.Type == messageContext.channel && c.TypeNo == TypeCode).FirstOrDefault(); //Content的内容:【一应生活】您有一件单号为expressNumbers company, 已到communityName收发室,请打开一应生活APP“收发室”获取取件码进行取件。点击下载http://a.app.qq.com/o/simple.jsp?pkgname=com.ening.life if (!string.IsNullOrEmpty(Module.Content)) { var content = Module.Content; content = content.Replace("company", messageContext.company); content = content.Replace("expressNumbers", messageContext.expressNumbers); content = content.Replace("communityName", messageContext.communityName); content = content.Replace("Id", messageContext.Id); content = content.Replace("receiveTime", messageContext.receiveTime); content = content.Replace("fetchCode", messageContext.fetchCode); messageContent = content; } return messageContent; } catch (Exception ex) {} return ""; } #endregion }MessageContext class, this is an entity object transmitted and called by the client. There are many dynamic tag variables similar to text messages in the object.
public class MessageContext{ /// 手机号码 public string phone { get; set; } /// 发送信息 public string message { get; set; } /// 签名 public string sign { get; set; } /// 渠道 public string channel { get; set; } /// 内容 public string content { get; set; } /// 取件码 public string fetchCode { get; set; } /// 快递公司 public string company { get; set; } /// 快递单号 public string expressNumbers { get; set; } /// 社区名称 public string communityName { get; set; } /// 到件时间 public string receiveTime { get; set; } /// 序号 public string Id { get; set; } /// 业务代码 public string serviceCode { get; set; } }Controller method externalMerchantSendMessage, which is for external calls
/// 外部商户发送信息 public ActionResult externalMerchantSendMessage(MessageContext param) { logger.Info("[externalMerchantSendMessage]param:" + param); bool isAuth = authModelBusiness.isAuth(param.channel, param.phone, param.sign); if (!isAuth) { return Json(new Result<string>() { resultCode = ((int)ResultCode.NoPermission).ToString(), resultMsg = "签名或无权限访问" }, JsonRequestBehavior.AllowGet); } var meaage = messageModuleBusiness.GetContent(param); if (string.IsNullOrEmpty(meaage)) { return Json(new Result<string>() { resultCode = ((int)ResultCode.failure).ToString(), resultMsg = "发送失败" }, JsonRequestBehavior.AllowGet); } SMSHelper helper = new SMSHelper(); helper.SendSMS(meaage, param.phone); return Json(new Result<string>() { resultCode = ((int)ResultCode.success).ToString(), resultMsg = "发送成功" }, JsonRequestBehavior.AllowGet); }The above are the functions that I have implemented before receiving the development task. It seems that my task is quite simple, but many years of development experience tell me that this needs to be refactored. If I don't care about anything now and just make an interface for adding, deleting, modifying and checking SMS templates, the maintenance people in the future will definitely go crazy. .
function sendMsg() { //var appParam ="phone=15914070649&sign=78a7ce797cf757916c2c7675b6865b54&channel=weijiakeji&content=&fetchCode=1 &company=%E9%A1%BA%E4%B8%B0%E5%BF%AB%E9%80%92&expressNumbers=123456&communityName=%E9%95%BF%E5%9F%8E%E4%B8%80%E8%8A%B1%E5%9B%AD&receiveTime=5&Id=1231"; //Get("/Message/externalMerchantSendMessage?" + appParam, {}); var data = { "phone": "15914070649", "sign": "78a7ce797cf757916c2c7675b6865b54", "channel": "weijiakeji", "fetchCode": 1, "company": "%E9%A1%BA%E4%B8%B0%E5%BF%AB%E9%80%92", "Id": "1231" }; Post('/Message/externalMerchantSendMessage', data); } //WebAPI Post方法 function Post(url, data) { $.ajax({ url: url, contentType: "application/json", type: "POST", dataType: "json", async: true, cache: false, data: JSON.stringify(data), success: function (response) { $('#response').text(JSON.stringify(response)); }, error: function (XMLHttpRequest, textStatus, errorThrown) { alert(textStatus); } }); }; //// WebApi Get方法 function Get(url, data) { $.ajax({ url: url, contentType: "application/json", type: "GET", dataType: "json", async: true, cache: false, //data: JSON.stringify(data), success: function (response) { $('#response').text(JSON.stringify(response)); }, error: function (XMLHttpRequest, textStatus, errorThrown) { alert(textStatus); } }); };It can be seen that what the customer passes is a collection of key-value pairs, which is an object in JSON format. According to the previous code bool isAuth = authModelBusiness.isAuth(param.channel, param.phone, param.sign);, it can be analyzed that there are three parameters that all calling customers must pass, that is: channel, phone, sign, The other parameters are the variable parameters and parameter values of the SMS template.
dynamic param = null; string json = Request.QueryString.ToString(); if (Request.QueryString.Count != 0) //ajax get请求 { //兼容旧的客户调用写法,暂时硬编了 if (json.Contains("param.")) { json = json.Replace("param.", ""); } json = "{" + json.Replace("=", ":'").Replace("&", "',") + "'}"; } else //ajax Post请求 { Request.InputStream.Position = 0; //切记这里必须设置流的起始位置为0,否则无法读取到数据 json = new StreamReader(Request.InputStream).ReadToEnd(); } var serializer = new JavaScriptSerializer(); serializer.RegisterConverters(new[] { new DynamicJsonConverter() }); param = serializer.Deserialize(json, typeof(object));
DynamicJsonConverter is used to convert JSON strings into Object objects. The code is as follows:
using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Dynamic; using System.Linq; using System.Text; using System.Web.Script.Serialization; public sealed class DynamicJsonConverter : JavaScriptConverter { public override object Deserialize(IDictionary<string, object> dictionary, Type type, JavaScriptSerializer serializer) { if (dictionary == null) throw new ArgumentNullException("dictionary"); return type == typeof(object) ? new DynamicJsonObject(dictionary) : null; } public override IDictionary<string, object> Serialize(object obj, JavaScriptSerializer serializer) { throw new NotImplementedException(); } public override IEnumerable<Type> SupportedTypes { get { return new ReadOnlyCollection<Type>(new List<Type>(new[] { typeof(object) })); } } #region Nested type: DynamicJsonObject private sealed class DynamicJsonObject : DynamicObject { private readonly IDictionary<string, object> _dictionary; public DynamicJsonObject(IDictionary<string, object> dictionary) { if (dictionary == null) throw new ArgumentNullException("dictionary"); _dictionary = dictionary; } public override string ToString() { var sb = new StringBuilder("{"); ToString(sb); return sb.ToString(); } private void ToString(StringBuilder sb) { var firstInDictionary = true; foreach (var pair in _dictionary) { if (!firstInDictionary) sb.Append(","); firstInDictionary = false; var value = pair.Value; var name = pair.Key; if (value is string) { sb.AppendFormat("{0}:\"{1}\"", name, value); } else if (value is IDictionary<string, object>) { new DynamicJsonObject((IDictionary<string, object>)value).ToString(sb); } else if (value is ArrayList) { sb.Append(name + ":["); var firstInArray = true; foreach (var arrayValue in (ArrayList)value) { if (!firstInArray) sb.Append(","); firstInArray = false; if (arrayValue is IDictionary<string, object>) new DynamicJsonObject((IDictionary<string, object>)arrayValue).ToString(sb); else if (arrayValue is string) sb.AppendFormat("\"{0}\"", arrayValue); else sb.AppendFormat("{0}", arrayValue); } sb.Append("]"); } else { sb.AppendFormat("{0}:{1}", name, value); } } sb.Append("}"); }
The above is the content of a .NET code reconstruction (Part 1). Please pay attention to more related content. PHP Chinese website (www.php.cn)!
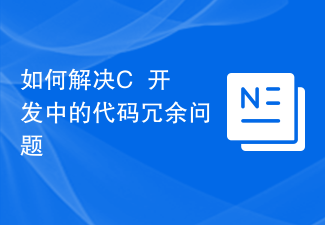
如何解决C++开发中的代码冗余问题代码冗余是指在编写程序时,出现了多个地方有相似或重复的代码。这种问题不仅使得代码难以维护和阅读,还会增加代码量和复杂性。而对于C++开发者来说,解决代码冗余问题尤为重要,因为C++是一种强大的编程语言,但也容易导致代码重复。代码冗余问题的根源在于不合理的设计和编码习惯。要解决这个问题,可以从以下几个方面着手:使用函数和类:C
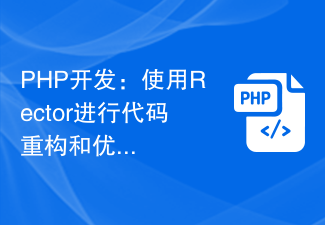
随着时间的推移和需求的变化,一个项目的代码很容易陈旧,难以维护和扩展。在PHP开发中,重构被认为是提高代码质量和开发效率的必备工作之一。而在这个过程中,使用Rector工具可以大大简化代码重构和优化的工作。Rector是一种开源的PHP代码重构工具,可以帮助PHP开发者自动化代码重构和优化,让开发者可以更专注于业务开发和功能的实现上。通过
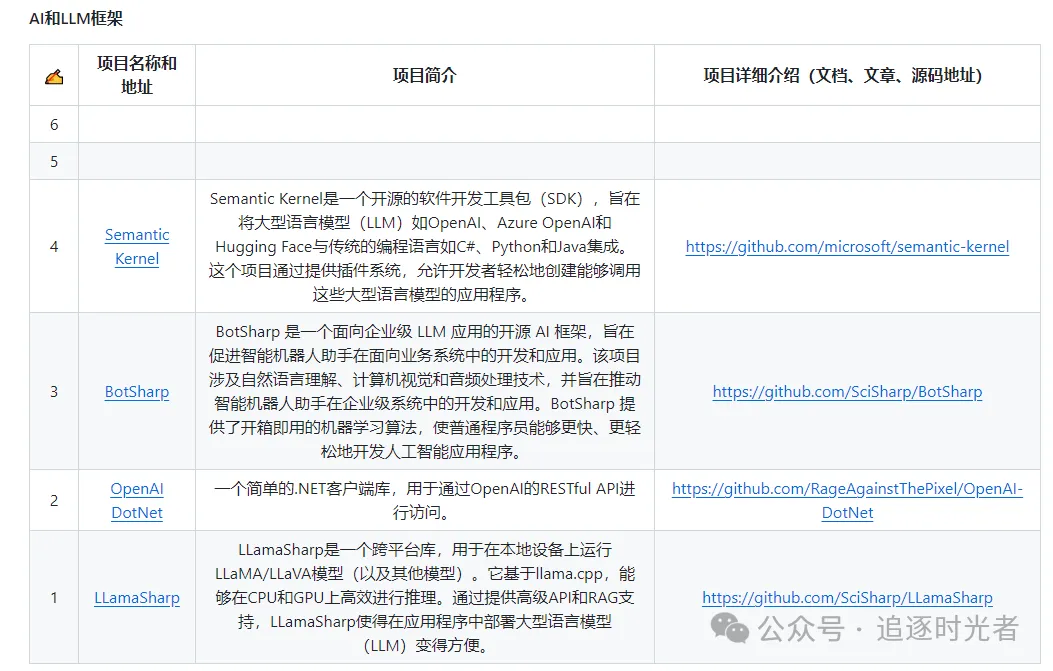
当今人工智能(AI)技术的发展如火如荼,它们在各个领域都展现出了巨大的潜力和影响力。今天大姚给大家分享4个.NET开源的AI模型LLM相关的项目框架,希望能为大家提供一些参考。https://github.com/YSGStudyHards/DotNetGuide/blob/main/docs/DotNet/DotNetProjectPicks.mdSemanticKernelSemanticKernel是一种开源的软件开发工具包(SDK),旨在将大型语言模型(LLM)如OpenAI、Azure
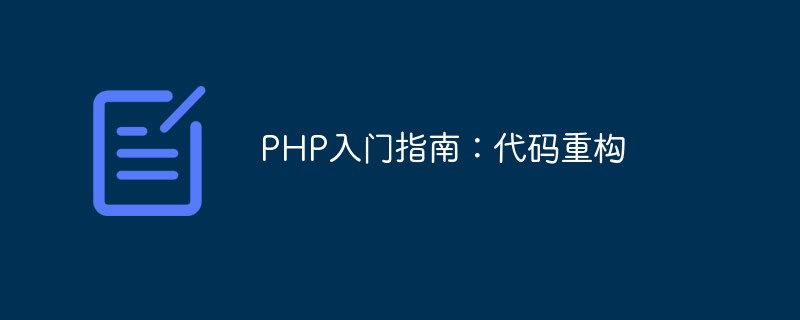
在写PHP代码时,重构是一个非常重要的过程。随着应用程序的增长,代码库会变得越来越庞大,难以阅读和维护。重构是为了解决这个问题,让代码更加模块化,并可以更好地组织和扩展。当我们重构代码时,需要考虑以下几个方面:代码风格代码风格是很重要的一点。保持你的代码风格一致性会让代码更易于阅读和维护。请遵循PHP代码规范,并保持一致。尝试使用代码风格检查工具,例如PHP
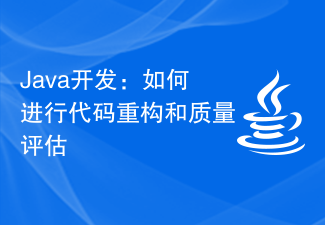
Java开发:代码重构和质量评估引言:在软件开发的过程中,代码重构是提高代码质量和可维护性的重要手段之一。通过对代码进行重构,可以使代码更加优雅、简洁、易于理解和修改。然而,重构并不仅仅是简单地修改一下代码,而是一个需要理性和系统性思考的过程。本文将介绍如何进行代码重构,并结合具体的代码示例进行说明。同时,我们还将讨论如何评估代码质量以及评估的重要性。代码重
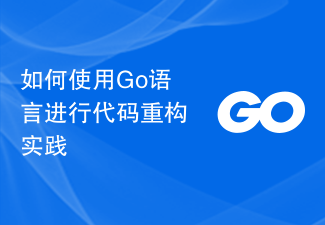
如何使用Go语言进行代码重构实践引言:在软件开发过程中,我们经常会面临代码重构的挑战。代码重构是指对现有的代码进行优化和重组,以提高代码质量和可维护性。本文将介绍如何使用Go语言进行代码重构实践,并附带相应的代码示例。一、代码重构的原则在进行代码重构之前,我们需要明确一些原则,以确保重构的顺利进行。以下是一些重要的代码重构原则:保持代码功能的一致性:在重构过
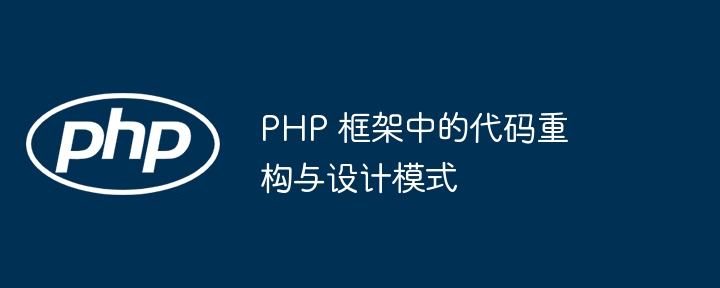
代码重构是一种优化软件结构的过程,涉及重命名、提取方法等技术。设计模式是解决常见软件问题的通用解决方案,例如单例模式和观察者模式。通过重构和使用设计模式,可以提高代码的可维护性、可读性和可扩展性。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
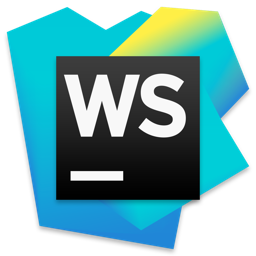
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
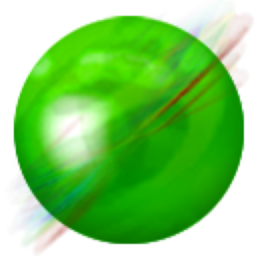
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
