As a C++ programmer, we have already mastered the basic concepts of object-oriented programming, and the syntax of Java is undoubtedly very familiar. In fact, Java is originally derived from C++.
However, there are still some significant differences between C++ and Java. Suffice it to say, these differences represent great advances in technology. Once we understand these differences, we will understand why Java is an excellent programming language. This appendix will guide you through some important characteristics that distinguish Java from C++.
(1). The biggest obstacle is speed: interpreted Java is about 20 times slower than C execution speed. Nothing can prevent the Java language from compiling. As of this writing, some near-real-time compilers have just emerged that can significantly speed things up. Of course, there is every reason to think that there will be pure native compilers for more popular platforms, but without those compilers, there must be some problems that Java cannot solve due to speed limitations.
(2). Like C++, Java also provides two types of comments.
(3) Everything must be placed in a class. There are no global functions or global data. If you want to obtain equivalent functions to global functions, consider placing static methods and static data in a class. Notice there are no such things as structures, enumerations or unions, there are just "classes"!
(4) All methods are defined in the body of the class. So from a C++ perspective, it seems that all functions have been embedded, but this is not the case (embedding issues will be discussed later).
(5) In Java, class definitions take almost the same form as C++. But there is no closing semicolon. There is no class declaration of the form class foo, only a class definition.
class aType() void aMethod() {/* 方法主体*/} }
(6) There is no scope operator "::" in Java. Java uses dot notation for everything, but you don't need to think about it because you can only define elements in a class. Even those method definitions must be inside a class, so there is no need to specify the scope of the scope. One difference we noticed is the call to static methods: using ClassName.methodName(). In addition, the name of the package (package) is established with a period, and the import keyword can be used to implement some functions of C++'s "#include". For example, the following statement:
Import java.awt.*;
(#include is not directly mapped to import, but it has a similar feeling when used.)
(7) Similar to C++, Java contains a series of "primitive types" to achieve more efficient access. In Java, these types include boolean, char, byte, short, int, long, float, and double. The sizes of all major types are intrinsic and machine-independent (to account for porting issues). This will definitely have some impact on performance, depending on the machine. Type checking and requirements have become more stringent in Java. For example:
Conditional expressions can only be of boolean type, and integers cannot be used.
The result of an expression like X+Y must be used; you cannot just use "X+Y" to achieve "side effects".
(8) The char (character) type uses the internationally accepted 16-bit Unicode character set, so it can automatically express characters from most countries.
(9) Static reference strings will be automatically converted into String objects. Unlike C and C++, there are no independent static character array strings available.
(10) Java adds three right shift operators ">>>", which have functions similar to the "logical" right shift operators and can insert zero values at the end. ">>" will insert the sign bit while shifting (i.e., "arithmetic" shift).
(11) Although superficially similar, Java arrays use a quite different structure and have unique behavior compared to C++. There is a read-only length member that lets you know how big the array is. And once the array boundary is exceeded, the runtime check will automatically throw an exception. All arrays are created in the memory "heap", and we can assign one array to another (simply copying the array handle). Array identifiers are first-level objects, and all of their methods generally apply to all other objects.
(12) All objects that do not belong to the main type can only be created through the new command. Unlike C++, Java has no corresponding command to create objects that are not of the main type "on the stack". All major types can only be created on the stack without using the new command. All major classes have their own "wrapper" classes, so equivalent memory "heap"-based objects can be created via new (main type arrays are an exception: they can be initialized via collections like in C++ assign, or use new).
(13) There is no need to declare in advance in Java. If you want to use a class or method before defining it, just use it directly - the compiler will ensure that the appropriate definition is used. So unlike in C++, we don't run into any problems involving early references.
(14) Java does not have a preprocessor. If you want to use a class in another library, just use the import command and specify the library name. There is no preprocessor-like macro.
(15) Java uses packages instead of namespaces. Since everything is put into a class, and thanks to a mechanism called "encapsulation" that does something like namespace decomposition on class names, naming issues are no longer a concern. . The package also collects library components under a single library name. We simply "import" a package and the compiler does the rest.
(16) Object handles defined as class members will be automatically initialized to null. The initialization of basic class data members is reliably guaranteed in Java. If not explicitly initialized, they get a default value (zero or equivalent). They can be explicitly initialized (explicit initialization): either by defining them within the class or in the builder. The syntax used is easier to understand than C++'s syntax, and it is fixed for both static and non-static members. We do not have to define the storage method of static members from the outside, which is different from C++.
(17) In Java, there are no pointers like C and C++. When you create an object with new, you will obtain a reference (this book will always refer to it as a "handle"). For example:
String s = new String("howdy");
However, C++ references must be initialized when they are created and cannot be redefined to a different location. But Java references are not necessarily limited to the location where they are created. They can be defined arbitrarily depending on the situation, which eliminates some of the need for pointers. Another reason for the heavy use of pointers in C and C++ is to be able to point to any memory location (this also makes them unsafe, which is why Java does not provide this support). Pointers are often seen as an efficient means of moving around in an array of primitive variables. Java allows us to achieve the same goal in a more secure form. The ultimate solution to pointer problems is the "inherent method" (discussed in Appendix A). Passing a pointer to a method usually doesn't cause too much of a problem because there are no global functions, just classes. And we can pass a reference to the object. The Java language initially claimed that it "does not use pointers at all!" But as many programmers began to ask, how can it work without pointers? So later it was stated that "use restricted pointers". It is a pointer that you can judge for yourself whether it is "true" or not. But in any case, there is no pointer "arithmetic".
(18) Java provides a "Constructor" similar to C++. If you don't define one yourself, you'll get a default builder. And if a non-default builder is defined, the default builder will not be automatically defined for us. This is the same as C++. Note that there is no copy builder since all arguments are passed by reference.
(19) There is no "Destructor" in Java. There is no "scope" problem with variables. The "life time" of an object is determined by the age of the object, not by the garbage collector. There is a finalize() method that is a member of every class, which is somewhat similar to the C++ "destructor". But finalize() is called by the garbage collector, and is only responsible for releasing "resources" (such as open files, sockets, ports, URLs, etc.). If you want to do something at a specific location, you must create a special method and call it. You cannot rely on finalize(). On the other hand, all objects in C++ will (or "should") be destroyed, but not all objects in Java will be collected as "garbage". Since Java does not support the concept of a destroyer, care must be taken to create a cleanup method when necessary. Moreover, all cleanup methods need to be explicitly called for the base classes and member objects within the class.
(20) Java has a method "overloading" mechanism, which works almost exactly the same as the overloading of C++ functions.
(21) Java does not support default arguments.
(22) There is no goto in Java. The unconditional jump mechanism it adopts is the "break label" or "continue standard", which is used to jump out of the current multiple nested loops.
(23) Java adopts a single-root hierarchical structure, so all objects are uniformly inherited from the root class Object. In C++, we can start a new inheritance tree anywhere, so we often end up seeing a "forest" containing a large number of trees. In Java we only have one hierarchical structure anyway. Although this may seem like a limitation on the surface, more powerful capabilities are often gained because we know that every object must have at least one Object interface. C++ currently seems to be the only OO language that does not enforce a single root structure.
(24) Java does not have templates or other forms of parameterized types. It provides a series of collections: Vector (vector), Stack (stack) and Hashtable (hash table), used to accommodate Object references. Using these collections, a range of our requirements can be met. But these collections are not designed to be called quickly like the C++ "Standard Template Library" (STL). The new collections in Java 1.2 appear more complete, but still do not have the efficient use of authentic templates.
(25) "Garbage collection" means that memory leaks in Java will be much rarer, but not completely impossible (if you call an inherent method for allocating storage space, The garbage collector cannot track and monitor it). However, memory leaks and resource leaks are often caused by improperly written finalize(), or by releasing a resource at the end of an allocated block ("destructors" are particularly convenient at this time). The garbage collector is a great progress based on C++, which makes many programming problems invisible. But it is not suitable for a few problems where the garbage collector is unable to cope with it. But the numerous advantages of the garbage collector make this shortcoming seem trivial.
(26) Java has built-in support for multi-threading. Using a special Thread class, we can create a new thread through inheritance (the run() method is abandoned). If the synchronized keyword is used as a type qualifier for a method, mutual exclusion occurs at the object level. At any given time, only one thread can use an object's synchronized method. On the other hand, after a synchronized method enters, it first "locks" the object, preventing any other synchronized methods from using that object. Only after exiting this method will the object be "unlocked". We are still responsible for implementing more complex synchronization mechanisms between threads by creating our own "monitor" class. Recursive synchronized methods work fine. If threads have the same priority, time "slicing" cannot be guaranteed.
(27) Instead of controlling the declaration code block like C++, we put the access qualifiers (public, private and protected) into the definition of each class member. If an "explicit" (unambiguous) qualifier is not specified, "friendly" will be defaulted. This means that other elements in the same package can also access it (equivalent to them becoming C++ "friends" - friends), but it cannot be accessed by any element outside the package. A class—and every method within a class—has an access qualifier that determines whether it is "visible" outside the file. The private keyword is generally rarely used in Java because "friendly" access is usually more useful than excluding access from other classes in the same package. However, in a multi-threaded environment, the appropriate use of private is very important. Java's protected keyword means "accessible by inheritors and other elements in the package." Note that Java has no equivalent to C++'s protected keyword, which means "can only be accessed by inheritors" (previously "private protected" could be used for this purpose, but this combination of keywords has been cancelled) .
(28) Nested classes. In C++, nesting classes helps hide names and facilitate code organization (but C++'s "namespaces" have made name hiding redundant). Java's concept of "encapsulation" or "packaging" is equivalent to C++'s namespace, so this is no longer an issue. Java 1.1 introduced the concept of "inner classes", which secretly maintain a handle to the outer class - needed when creating inner class objects. This means that the inner class object may be able to access the members of the outer class object without any conditions - as if those members were directly subordinate to the inner class object. This provides a better solution to the callback problem - C++ solves it with pointers to members.
(29) Due to the existence of the inner class introduced earlier, there is no pointer to member in Java.
(30) There is no "embedded" (inline) method in Java. The Java compiler may embed a method at its discretion, but we have no more control over this. In Java, you can use the final keyword for a method to "suggest" embedding. However, embedding functions is only a suggestion for C++ compilers.
(31) Java中的继承具有与C++相同的效果,但采用的语法不同。Java用extends关键字标志从一个基础类的继承,并用super关键字指出准备在基础类中调用的方法,它与我们当前所在的方法具有相同的名字(然而,Java中的super关键字只允许我们访问父类的方法——亦即分级结构的上一级)。通过在C++中设定基础类的作用域,我们可访问位于分级结构较深处的方法。亦可用super关键字调用基础类构建器。正如早先指出的那样,所有类最终都会从Object里自动继承。和C++不同,不存在明确的构建器初始化列表。但编译器会强迫我们在构建器主体的开头进行全部的基础类初始化,而且不允许我们在主体的后面部分进行这一工作。通过组合运用自动初始化以及来自未初始化对象句柄的异常,成员的初始化可得到有效的保证。
public class Foo extends Bar { public Foo(String msg) { super(msg); // Calls base constructor } public baz(int i) { // Override super.baz(i); // Calls base method } }
(32) Java中的继承不会改变基础类成员的保护级别。我们不能在Java中指定public,private或者protected继承,这一点与C++是相同的。此外,在衍生类中的优先方法不能减少对基础类方法的访问。例如,假设一个成员在基础类中属于public,而我们用另一个方法代替了它,那么用于替换的方法也必须属于public(编译器会自动检查)。
(33) Java提供了一个interface关键字,它的作用是创建抽象基础类的一个等价物。在其中填充抽象方法,且没有数据成员。这样一来,对于仅仅设计成一个接口的东西,以及对于用extends关键字在现有功能基础上的扩展,两者之间便产生了一个明显的差异。不值得用abstract关键字产生一种类似的效果,因为我们不能创建属于那个类的一个对象。一个abstract(抽象)类可包含抽象方法(尽管并不要求在它里面包含什么东西),但它也能包含用于具体实现的代码。因此,它被限制成一个单一的继承。通过与接口联合使用,这一方案避免了对类似于C++虚拟基础类那样的一些机制的需要。
为创建可进行“例示”(即创建一个实例)的一个interface(接口)的版本,需使用implements关键字。它的语法类似于继承的语法,如下所示:
public interface Face { public void smile(); } public class Baz extends Bar implements Face { public void smile( ) { System.out.println("a warm smile"); } }
(34) Java中没有virtual关键字,因为所有非static方法都肯定会用到动态绑定。在Java中,程序员不必自行决定是否使用
以上就是java到底和C++有啥区别?的内容,更多相关内容请关注PHP中文网(www.php.cn)!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
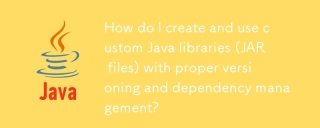
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
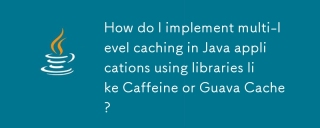
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
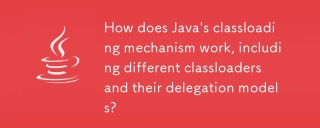
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
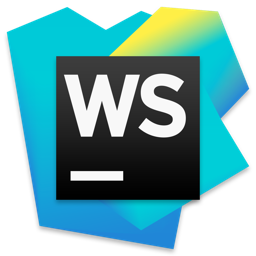
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
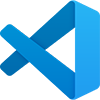
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor