1. Enhanced for overview
Enhanced for loop, also called Foreach loop, is used for traversing arrays and containers (collection classes). When using a foreach loop to traverse array and collection elements, there is no need to obtain the array and collection lengths, and there is no need to access array elements and collection elements based on indexes, which greatly improves efficiency and makes the code much simpler.
2. Explanation from Oracle’s official website
So when should you use a for-each loop? Any time is fine. This really beautifies your code. Unfortunately, you can't use it everywhere. Consider these cases, for example, removing methods. In order to remove the current element, the program needs access to the iterator. The for-each loop hides the iterator, so you can't call the delete function. Therefore, the for-each loop does not work for filtering elements. Loops that require replacing elements when iterating over a collection or array are also not applicable. Finally, it is not suitable for use in parallel loops across multiple collection iterations. Designers should be aware of these pitfalls and consciously design a clean, simple structure to avoid these situations. If you are interested, you can view the API on the official website. If you don’t know how to find the API on the official website, please click to open the official website to view the API method.
3. Enhanced for format
for(集合或者数组元素的类型 变量名 : 集合对象或者数组对象){ 引用变量名的java语句; }
When you see the colon (:), it reads as "come in." The above loop reads as "traverse each TimerTask element in c. "As you can see, the for-each construct combines perfectly with generics. It retains all types of security while removing the remaining clutter. Because you don't have to declare the iterator, you don't have to provide a generic declaration for it. (The compiler has already done it behind your back, you don’t need to care about it.)
Simple experience:
1. Enhance for to traverse the array
package cn.jason01; //增强for遍历数组 public class ForTest01 { public static void main(String[] args) { int[] array={1,2,3}; for(int element: array){ System.out.println(element); } } }
2. Enhance for to traverse the collection
package cn.jason01; import java.util.ArrayList; public class ForTest { public static void main(String[] args) { // 泛型推断,后面可以写可以不写String ArrayList<String> array = new ArrayList(); array.add("a"); array.add("b"); array.add("c"); for (String string : array) { System.out.println(string); } } }
4. Enhance the underlying principle of for
Look at the code first
package cn.jason01; import java.util.ArrayList; import java.util.Iterator; /** * 增强for底层原理 * * @author cassandra * @version 1.1 */ public class ForTest { public static void main(String[] args) { // 泛型推断,后面可以写可以不写String.规范一些是要写上的。 ArrayList<String> array = new ArrayList(); // 添加元素 array.add("a"); array.add("b"); array.add("c"); // 增强for实现 System.out.println("----enhanced for----"); for (String string : array) { System.out.println(string); } // 反编译之后的效果,也就是底层实现原理 System.out.println("---reverse compile---"); String string; for (Iterator iterator = array.iterator(); iterator.hasNext(); System.out.println(string)) { string = (String) iterator.next(); } // 迭代器实现 System.out.println("------Iterator------"); for (Iterator<String> i = array.iterator(); i.hasNext(); System.out.println(i.next())) { } // 普通for实现 System.out.println("-----general for-----"); for (int x = 0; x < array.size(); x++) { System.out.println(array.get(x)); } } }
As can be seen from the above code, the bottom layer is implemented by iterators. Enhanced for actually hides the iterators, so there is no need to create iterators and the code is much simpler. This is also the reason for the introduction of enhanced for, which is to reduce code, facilitate traversal of collections and arrays, and improve efficiency.
Note: Because enhanced for hides the iterator, when using enhanced for to traverse collections and arrays, you must first determine whether it is null, otherwise a null pointer exception will be thrown. The reason is very simple. The bottom layer needs to use an array or collection object to call the iterator() method to create an iterator (Iterator is an interface, so it must be implemented by a subclass). If it is null, an exception will definitely be thrown.
5. Enhance the applicability and limitations of for
1. Applicability
Applicable to traversal of collections and arrays.
2. Limitations:
①The collection cannot be null because the underlying layer is an iterator.
②The iterator is hidden, so the collection cannot be modified (added or deleted) when traversing the collection.
③The corner mark cannot be set.
6. Detailed explanation of enhanced for usage
1. Enhanced for usage in arrays
package cn.jason05; import java.util.ArrayList; import java.util.List; /** * 增强for用法 * * @author cassandra */ public class ForDemo { public static void main(String[] args) { // 遍历数组 int[] arr = { 1, 2, 3, 4, 5 }; for (int x : arr) { System.out.println(x); } } }
2. Enhanced for usage in collections
package cn.jason05; import java.util.ArrayList; import java.util.List; /** * 增强for用法 * * @author cassandra */ public class ForDemo { public static void main(String[] args) { // 遍历集合 ArrayList<String> array = new ArrayList<String>(); array.add("hello"); array.add("world"); array.add("java"); for (String s : array) { System.out.println(s); } // 集合为null,抛出NullPointerException空指针异常 List<String> list = null; if (list != null) { for (String s : list) { System.out.println(s); } } // 增强for中添加或修改元素,抛出ConcurrentModificationException并发修改异常 for (String x : array) { if (array.contains("java")) array.add(1, "love"); } }
3. Perfect combination of generics and enhanced for
Note: It must be perfectly combined with generics, otherwise you have to manually transform downward
1. There is no generic effect and the enhanced for
Student class cannot be used
package cn.jason01; public class Student { private String name1; private String name2; public Student() { super(); } public Student(String name1, String name2) { super(); this.name1 = name1; this.name2 = name2; } public String getName1() { return name1; } public void setName1(String name1) { this.name1 = name1; } public String getName2() { return name2; } public void setName2(String name2) { this.name2 = name2; } }
Test code
package cn.jason01; import java.util.ArrayList; import java.util.Iterator; import java.util.List; public class Test02 { public static void main(String[] args) { // 创建集合1 List list1 = new ArrayList(); list1.add("a"); list1.add("b"); list1.add("c"); // 创建集合2 List list2 = new ArrayList(); list2.add("d"); list2.add("e"); list2.add("f"); // 创建集合三 List list3 = new ArrayList(); // 遍历第一和第二个集合,并添加元素到集合三 for (Iterator i = list1.iterator(); i.hasNext();) { // System.out.println(i.next()); String s = (String) i.next(); for (Iterator j = list2.iterator(); j.hasNext();) { // list2.add(new Student(s,j.next())); String ss = (String) j.next(); list3.add(new Student(s, ss)); } } // 遍历集合三,并输出元素 Student st; for (Iterator k = list3.iterator(); k.hasNext(); System.out .println(new StringBuilder().append(st.getName1()).append(st.getName2()))) { st = (Student) k.next(); } } }
If you remove the two lines of commented code in the above code, the program will report an error, because the collection does not declare the type of the element, and the iterator naturally does not know what type it is. Therefore, if there are no generics, then downward transformation is required. Only iterators can be used, and enhanced for cannot be used.
2. Generics and enhancement for
Generic modification of the above code
package cn.jason01; import java.util.ArrayList; import java.util.Iterator; import java.util.List; /** * 增强for和泛型完美结合 * * @author cassandra */ public class Test03 { public static void main(String[] args) { // 创建集合1 List<String> list1 = new ArrayList<String>(); list1.add("a"); list1.add("b"); list1.add("c"); // 创建集合2 List<String> list2 = new ArrayList<String>(); list2.add("d"); list2.add("e"); list2.add("f"); // 创建集合三 List<Student> list3 = new ArrayList<Student>(); //// 遍历第一和第二个集合,并添加元素到集合三 for (String s1 : list1) { for (String s2 : list2) { list3.add(new Student(s1, s2)); } } // 遍历集合三,并输出元素 for (Student st : list3) { System.out.println(new StringBuilder().append(st.getName1()).append(st.getName2())); } } }
4. Four methods for List collection traversal
## There is an iterator() method in the #Collection interface, which returns an Iterator type. There is an iterator called Iterator. There is a listIterator() method in List, so there is an additional collector ListIterator. Its subclasses LinkedList, ArrayList, and Vector all implement the List and Collection interfaces, so they can be traversed using two iterators. Code testpackage cn.jason05; import java.util.ArrayList; import java.util.Iterator; import java.util.List; import java.util.ListIterator; /** * 这是List集合遍历四种方法 * * @author cassandra */ public class ForDemo01 { public static void main(String[] args) { // 创建集合 List<String> list = new ArrayList<String>(); list.add("hello"); list.add("world"); list.add("java"); // 方法1,Iterator迭代器遍历 Iterator<String> i = list.iterator(); while (i.hasNext()) { String s = i.next(); System.out.println(s); } // 方法2,ListIterator迭代器遍历集合 ListIterator<String> lt = list.listIterator(); while (lt.hasNext()) { String ss = lt.next(); System.out.println(ss); } // 方法3,普通for遍历集合 for (int x = 0; x < list.size(); x++) { String sss = list.get(x); System.out.println(sss); } // 方法4,增强for遍历集合 for (String ssss : list) { System.out.println(ssss); } } }5.Set collection traversal method in 2Since the Set collection does not have get(int index) method, so there is no ordinary for loop, there is no listIterator() method in Set, so there is no ListIterator iterator. So there are only two ways to traverse. Code Test
package cn.jason05; import java.util.HashSet; import java.util.Iterator; import java.util.Set; public class ForTest03 { public static void main(String[] args) { Set<String> set = new HashSet<String>(); set.add("hello"); set.add("world"); set.add("java"); // 方法1,Iterator迭代器遍历集合 Iterator<String> it = set.iterator(); while (it.hasNext()) { System.out.println(it.next()); } // 方法2,增强for遍历集合 for (String s : set) { System.out.println(s); } } }7. Summary1. Enhance the applicability and limitations of for
Applicability: Suitable for traversal of collections and arrays. Limitations: ①The collection cannot be null because the underlying layer is an iterator. ②The corner mark cannot be set. ③The iterator is hidden, so the collection cannot be modified (added or deleted) when traversing the collection. 2. Only by enhancing the combination of for and generics in the collection can new features be brought into play. 3. It is very important to check the new features of the official website. To know what they are, you also need to know why. Only when you understand them in your mind can you use them freely. The above summary of the most complete usage of the for loop, a new feature of Java, is all the content shared by the editor. I hope it can give you a reference, and I hope you will support the PHP Chinese website. For more java new features, the most complete usage summary of the for loop, please pay attention to the PHP Chinese website for related articles!
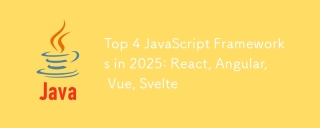
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
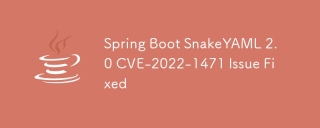
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
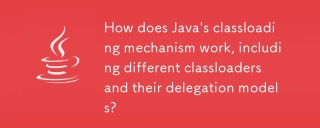
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
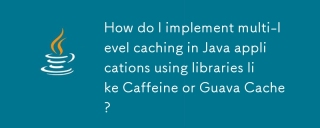
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
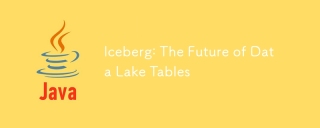
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
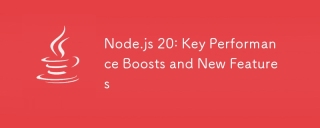
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
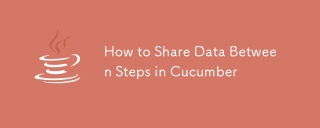
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
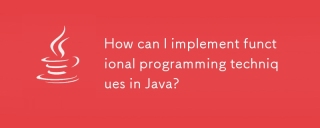
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
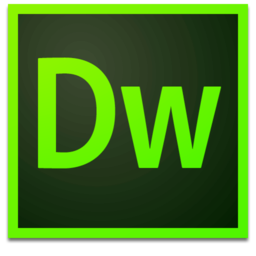
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
