The MySQL client/server protocol provides prepared statements. This function uses the MYSQL_STMT statement handler data structure returned by the mysql_stmt_init() initialization function. For statements that are executed multiple times, preprocessed execution is an effective way. First, the statement is parsed to prepare for execution. Next, execute one or more times later using the statement handle returned by the initialization function.
For statements that are executed multiple times, preprocessing execution is faster than direct execution. The main reason is that only one parsing operation is performed on the query. In the case of direct execution, the query is performed each time the statement is executed. In addition, network traffic is reduced because only the parameter data is sent each time the prepared statement is executed.
Another advantage of prepared statements is that it uses a binary protocol, making data transmission between the client and server more efficient.
Similar to the placeholder concept in Oracle! !
General steps:
Use mysql_stmt_init() to create a prepared statement handle. To prepare a prepared statement on the server, call mysql_stmt_prepare(), passing it a string containing the SQL statement. If the statement generated a result set, call mysql_stmt_result_metadata() to obtain the result set metadata. Although distinct from the result set that contains the columns returned by the query, this metadata itself takes the form of a result set. The metadata result set indicates how many columns are included in the result and contains information about each column. Use mysql_stmt_bind_param() to set the value of any parameter. All parameters must be set. Otherwise, statement execution will return an error or produce unpredictable results. Call mysql_stmt_execute() to execute the statement. If the statement generated a result set, bind the data buffer and retrieve the row values by calling mysql_stmt_bind_result(). Data is fetched into the buffer row by row by repeatedly calling mysql_stmt_fetch() until no more rows are found. Repeat steps 3 through 6 by changing the parameter values and executing the statement again.
Look at the code for details
#include <stdio.h> #include <stdlib.h> #include <string.h> #include <dlfcn.h> #include <mysql/mysql.h> #include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <string.h> #include <errno.h> #include <termios.h> #include <mysql/mysql.h> #include <termios.h> #define STRING_SIZE 50 #define DROP_SAMPLE_TABLE "DROP TABLE IF EXISTS test_table" #define CREATE_SAMPLE_TABLE "CREATE TABLE test_table(col1 INT,\ col2 VARCHAR(40),\ col3 SMALLINT,\ col4 TIMESTAMP)" #define INSERT_SAMPLE "INSERT INTO test_table(col1,col2,col3) VALUES(?,?,?)" int main(int arg, char *args[]) { int ret = 0, i=0; MYSQL *mysql; MYSQL *connect; MYSQL_RES *result; MYSQL_ROW row; MYSQL_FIELD *fields; unsigned int num_fields; //if (arg < 4) //{ // printf("please enter: %s localhost user password dbname\n", args[0]); // return -1; //} mysql = mysql_init(NULL); //连接到mysql server //connect = mysql_real_connect(mysql, args[1], args[2], args[3], args[4],0, 0, 0); //connect = mysql_real_connect(mysql, "localhost", "root", a, args[4],0, 0, 0); connect = mysql_real_connect(mysql, "localhost", "root", "123456", "mydb2", 0, NULL, 0 ); if (connect == NULL) { printf("connect error, %s\n", mysql_error(mysql)); return -1; } ret = mysql_query(connect, "SET NAMES utf8"); //设置字符集为UTF8 if (ret != 0) { printf("设置字符集错误, %s\n", mysql_error(mysql)); return ret; } MYSQL_STMT *stmt; MYSQL_BIND bind[3]; my_ulonglong affected_rows; int param_count; short small_data; int int_data; char str_data[STRING_SIZE]; unsigned long str_length; my_bool is_null; if (mysql_query(mysql, DROP_SAMPLE_TABLE)) { fprintf(stderr, " DROP TABLE failed\n"); fprintf(stderr, " %s\n", mysql_error(mysql)); exit(0); } if (mysql_query(mysql, CREATE_SAMPLE_TABLE)) { fprintf(stderr, " CREATE TABLE failed\n"); fprintf(stderr, " %s\n", mysql_error(mysql)); exit(0); } /* Prepare an INSERT query with 3 parameters */ /* (the TIMESTAMP column is not named; the server */ /* sets it to the current date and time) */ stmt = mysql_stmt_init(mysql); //初始化 预处理环境 生成一个预处理句柄 if (!stmt) { fprintf(stderr, " mysql_stmt_init(), out of memory\n"); exit(0); } if (mysql_stmt_prepare(stmt, INSERT_SAMPLE, strlen(INSERT_SAMPLE))) //预处理环境中 准备sql { fprintf(stderr, " mysql_stmt_prepare(), INSERT failed\n"); fprintf(stderr, " %s\n", mysql_stmt_error(stmt)); exit(0); } fprintf(stdout, " prepare, INSERT successful\n"); /* Get the parameter count from the statement */ param_count= mysql_stmt_param_count(stmt); //预处理环境中 求绑定变量的个数 fprintf(stdout, " total parameters in INSERT: %d\n", param_count); if (param_count != 3) /* validate parameter count */ { fprintf(stderr, " invalid parameter count returned by MySQL\n"); exit(0); } /* Bind the data for all 3 parameters */ memset(bind, 0, sizeof(bind)); /* INTEGER PARAM */ /* This is a number type, so there is no need to specify buffer_length */ bind[0].buffer_type= MYSQL_TYPE_LONG; //为第一个绑定变量设置类型和 输入变量的内存首地址 bind[0].buffer= (char *)&int_data; bind[0].is_null= 0; bind[0].length= 0; /* STRING PARAM */ bind[1].buffer_type= MYSQL_TYPE_STRING; //为第2个绑定变量设置类型和 输入变量的内存首地址 bind[1].buffer= (char *)str_data; bind[1].buffer_length= STRING_SIZE; bind[1].is_null= 0; bind[1].length= &str_length; /* SMALLINT PARAM */ bind[2].buffer_type= MYSQL_TYPE_SHORT; //为第3个绑定变量设置类型和 输入变量的内存首地址 bind[2].buffer= (char *)&small_data; bind[2].is_null= &is_null; bind[2].length= 0; /* Bind the buffers */ if (mysql_stmt_bind_param(stmt, bind)) //把绑定变量设置到 预处理环境中 { fprintf(stderr, " mysql_stmt_bind_param() failed\n"); fprintf(stderr, " %s\n", mysql_stmt_error(stmt)); exit(0); } /* Specify the data values for the first row */ //插入第一条记录 int_data= 10; /* integer */ strncpy(str_data, "MySQL", STRING_SIZE); /* string */ str_length= strlen(str_data); /* INSERT SMALLINT data as NULL */ is_null= 1; /* Execute the INSERT statement - 1*/ if (mysql_stmt_execute(stmt)) { fprintf(stderr, " mysql_stmt_execute(), 1 failed\n"); fprintf(stderr, " %s\n", mysql_stmt_error(stmt)); exit(0); } /* Get the total number of affected rows */ affected_rows= mysql_stmt_affected_rows(stmt); fprintf(stdout, " total affected rows(insert 1): %lu\n", (unsigned long) affected_rows); if (affected_rows != 1) /* validate affected rows */ { fprintf(stderr, " invalid affected rows by MySQL\n"); exit(0); } /* Specify data values for second row, then re-execute the statement */ int_data= 1000; //插入第一条记录 strncpy(str_data, "The most popular Open Source database", STRING_SIZE); str_length= strlen(str_data); small_data= 1000; /* smallint */ is_null= 0; /* reset */ /* Execute the INSERT statement - 2*/ if (mysql_stmt_execute(stmt)) { fprintf(stderr, " mysql_stmt_execute, 2 failed\n"); fprintf(stderr, " %s\n", mysql_stmt_error(stmt)); exit(0); } /* Get the total rows affected */ affected_rows= mysql_stmt_affected_rows(stmt); fprintf(stdout, " total affected rows(insert 2): %lu\n", (unsigned long) affected_rows); if (affected_rows != 1) /* validate affected rows */ { fprintf(stderr, " invalid affected rows by MySQL\n"); exit(0); } /* Close the statement */ if (mysql_stmt_close(stmt)) { fprintf(stderr, " failed while closing the statement\n"); fprintf(stderr, " %s\n", mysql_stmt_error(stmt)); exit(0); } mysql_close(connect); //断开与SQL server的连接 }
The above is the content of the use of prepared statements for getting started with MySQL. For more related content, please pay attention to the PHP Chinese website (www.php.cn)!
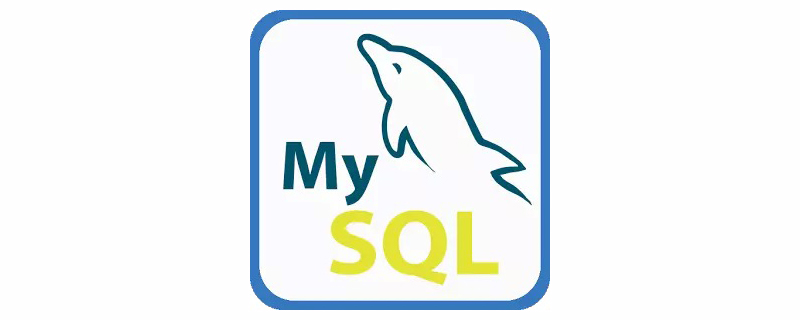
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了关于架构原理的相关内容,MySQL Server架构自顶向下大致可以分网络连接层、服务层、存储引擎层和系统文件层,下面一起来看一下,希望对大家有帮助。
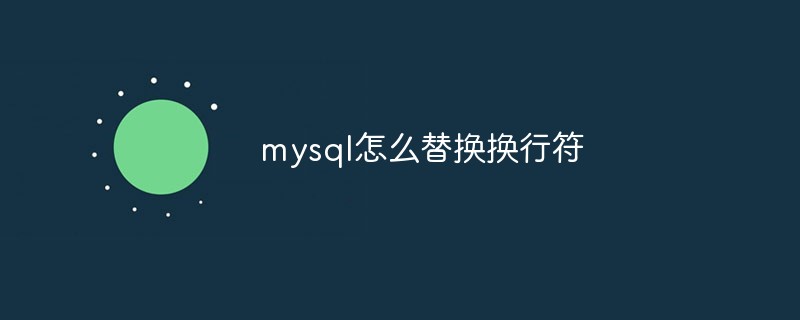
在mysql中,可以利用char()和REPLACE()函数来替换换行符;REPLACE()函数可以用新字符串替换列中的换行符,而换行符可使用“char(13)”来表示,语法为“replace(字段名,char(13),'新字符串') ”。
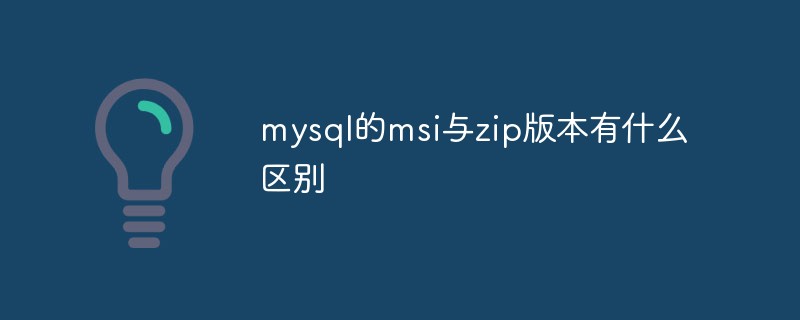
mysql的msi与zip版本的区别:1、zip包含的安装程序是一种主动安装,而msi包含的是被installer所用的安装文件以提交请求的方式安装;2、zip是一种数据压缩和文档存储的文件格式,msi是微软格式的安装包。
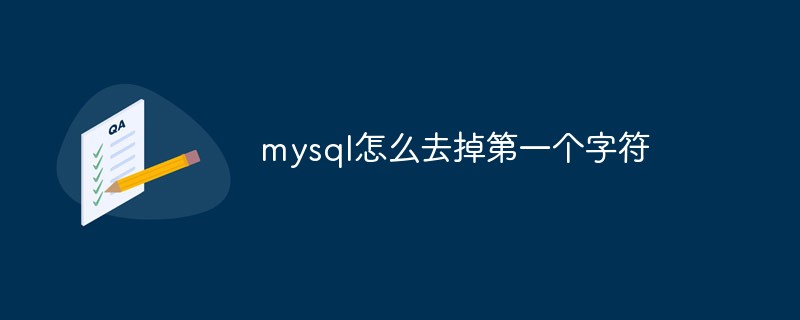
方法:1、利用right函数,语法为“update 表名 set 指定字段 = right(指定字段, length(指定字段)-1)...”;2、利用substring函数,语法为“select substring(指定字段,2)..”。
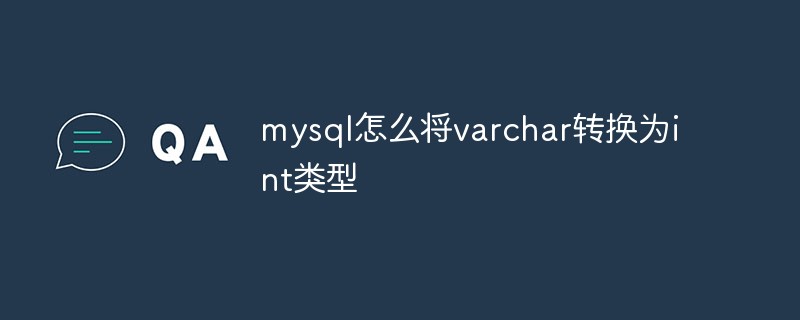
转换方法:1、利用cast函数,语法“select * from 表名 order by cast(字段名 as SIGNED)”;2、利用“select * from 表名 order by CONVERT(字段名,SIGNED)”语句。
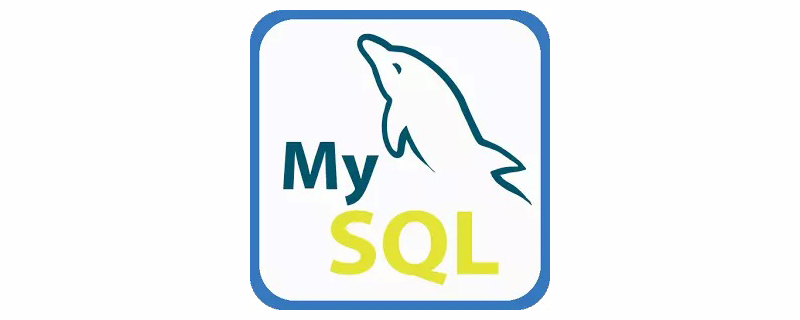
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了关于MySQL复制技术的相关问题,包括了异步复制、半同步复制等等内容,下面一起来看一下,希望对大家有帮助。
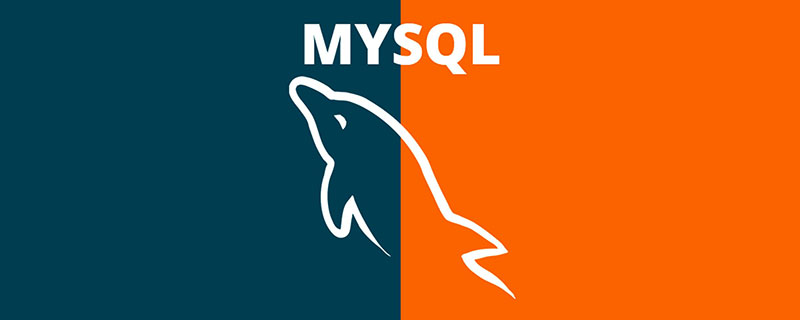
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了mysql高级篇的一些问题,包括了索引是什么、索引底层实现等等问题,下面一起来看一下,希望对大家有帮助。
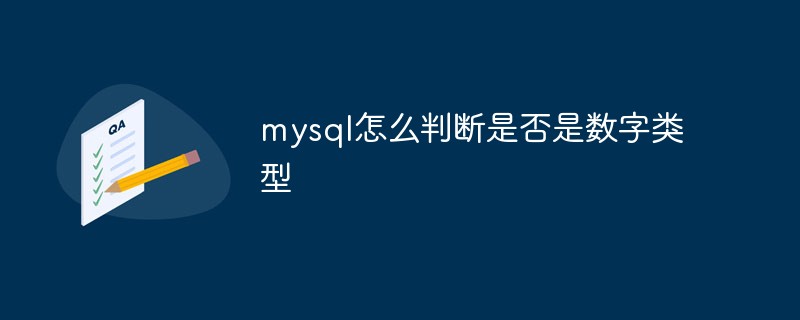
在mysql中,可以利用REGEXP运算符判断数据是否是数字类型,语法为“String REGEXP '[^0-9.]'”;该运算符是正则表达式的缩写,若数据字符中含有数字时,返回的结果是true,反之返回的结果是false。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
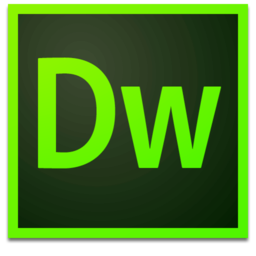
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!
