(1) readonly and const are both used to mark constants.
(2) The initialization assignment is different.
const-modified constants must be assigned a value at the same time they are declared. For example:
public class Class1 { public const int MaxValue = 10; //正确声明 public const MInValue; //错误:常量字段要求提供一个值 public Class1() { MinValue = 10; } }
readonly fields can be assigned during initialization (declaration or constructor). Therefore, readonly fields may have different values depending on the constructor used.
public class Class1 { public readonly int c = 10; //正确声明 public readonly int z; public Class1() { z = 24;//正确 } protected void Load() { z = 24;//错误:无法对只读的字段赋值(构造函数或变量初始值指定项中除外) } }
readonly is an instance member, so different instances can have different constant values, which makes readonly more flexible.
public readonly Color Red = new Color(255, 0, 0); public readonly Color Green = new Color(0, 255, 0); public readonly Color Blue = new Color(0, 0, 255);
(3) const fields are compile-time constants, while readonly fields can be used for run-time constants.
const requires the compiler to be able to calculate a certain value at compile time. At compile time, every place where the constant is called is replaced with the calculated value. Therefore you cannot extract a value from a variable to initialize a constant.
readonly allows a field to be set to a constant, but some operations can be performed and its initial value can be determined. Because readonly is executed at calculation time, it can be initialized with certain variables. This value is determined at runtime.
(4) const is static by default, and readonly must be explicitly declared if it is set to static.
(5) The type of value modified by const is also limited. It can only be one of the following types (or can be converted to the following types): sbyte, byte, short, ushort, int, uint, long, ulong, char, float, double, decimal, bool, string, enum type or reference type. Note that the reference type that can be declared as const can only be string or other reference types whose value is null. readonly can be of any type.
That is to say, when we need a const constant, if its type restricts it from being calculated to a definite value during compilation, then we can solve it by declaring it as static readonly. But there is still a slight difference between the two. Look at the two different documents below.
file1.cs
using System; namespace MyNamespace1 { public class MyClass1 { public static readonly int myField = 10; } }
file2.cs
namespace MyNamespace2 { public class MyClass1 { public static void Main() { Console.WriteLine(MyNamespace1.MyClass1.myField); } } }
The two classes belong to two files file1.cs and file2.cs and are compiled separately. When the domain myField in the file file1.cs is declared as static readonly, if we change the value of myField to 20 due to some need, then we only need to recompile the file file1.cs to file1.dll. When executing file2.exe That is, you will get 20.
But if you change static readonly to const and then change the initialization value of myField, we must recompile all files that reference file1.dll, otherwise the MyNamespace1.MyClass1.myField we reference will not be as we expected. Willing to change. This is especially important to pay attention to during the development of large systems.
(6) object, Array (array) and struct (structure) cannot be declared as const constants.
The above is the difference between const and readonly in c#.net. For more related content, please pay attention to the PHP Chinese website (www.php.cn)!

Vue3中的readonly特性详解readonly是Vue3中提供的一个新特性,用于将一个响应式对象变成只读对象。使用readonly可以确保一个对象只能被读取而不能被修改,从而提高应用的稳定性和安全性。在Vue3中,可以使用readonly函数将一个对象转换为只读对象,例如:import{readonly}from'vue'conststate=readonly({count:0})在上面的代码中,state对象被转换为只读对象,这意味着state.count
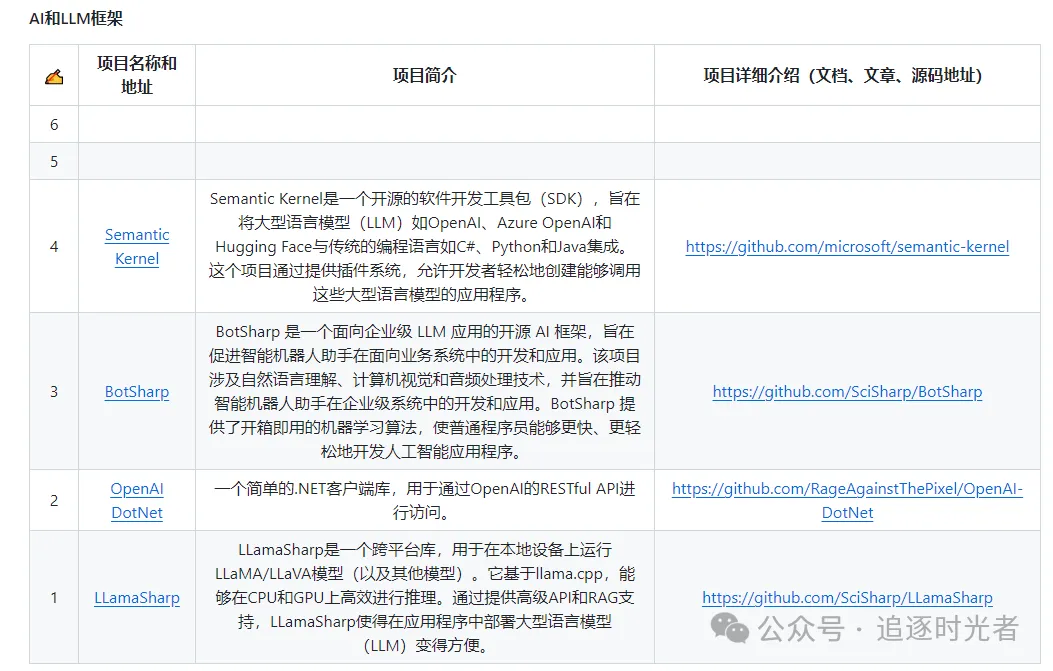
当今人工智能(AI)技术的发展如火如荼,它们在各个领域都展现出了巨大的潜力和影响力。今天大姚给大家分享4个.NET开源的AI模型LLM相关的项目框架,希望能为大家提供一些参考。https://github.com/YSGStudyHards/DotNetGuide/blob/main/docs/DotNet/DotNetProjectPicks.mdSemanticKernelSemanticKernel是一种开源的软件开发工具包(SDK),旨在将大型语言模型(LLM)如OpenAI、Azure
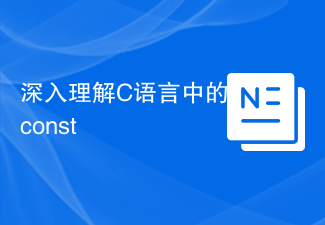
C中const的详解及代码示例在C语言中,const关键字用于定义常量,表示该变量的值在程序执行过程中不能被修改。const关键字可以用于修饰变量、函数参数以及函数返回值。本文将对C语言中const关键字的使用进行详细解析,并提供具体的代码示例。const修饰变量当const用于修饰变量时,表示该变量为只读变量,一旦赋值就不能再修改。例如:constint
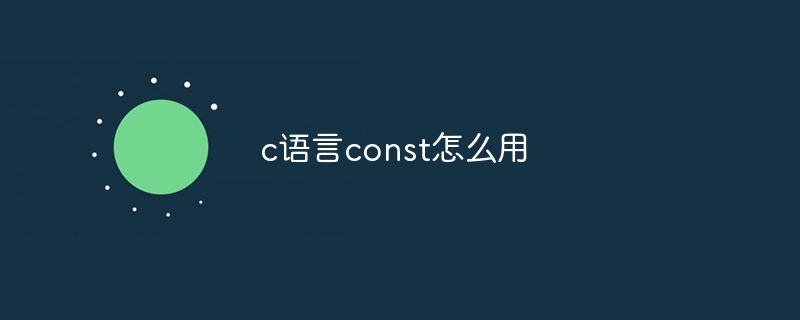
const是关键字,可以用于声明常量、函数参数中的const修饰符、const修饰函数返回值、const修饰指针。详细介绍:1、声明常量,const关键字可用于声明常量,常量的值在程序运行期间不可修改,常量可以是基本数据类型,如整数、浮点数、字符等,也可是自定义的数据类型;2、函数参数中的const修饰符,const关键字可用于函数的参数中,表示该参数在函数内部不可修改等等。
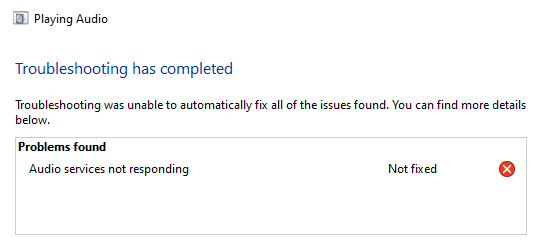
音频输出和输入需要特定的驱动程序和服务才能在Windows11上按预期工作。这些有时最终会在后台遇到错误,从而导致音频问题,如无音频输出、缺少音频设备、音频失真等。如何修复在Windows11上没有响应的音频服务我们建议您从下面提到的修复开始,并逐步完成列表,直到您设法解决您的问题。由于Windows11上的多种原因,音频服务可能无法响应。此列表将帮助您验证和修复阻止音频服务在Windows11上响应的大多数问题。请按照以下相关部分帮助您完成该过程。方法一:重启音频服务您可能会遇
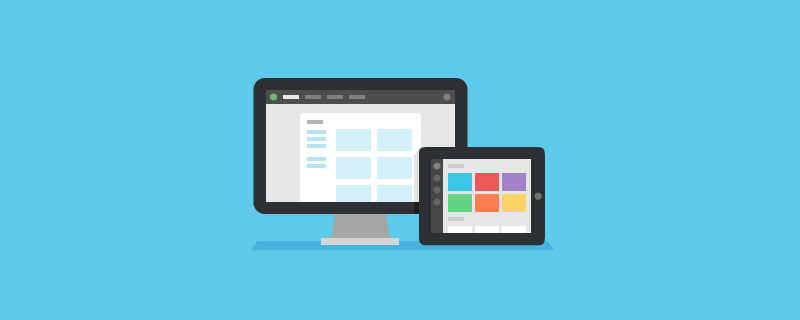
本篇文章给大家带来了关于JavaScript的相关知识,其中主要给大家介绍了var、let以及const的区别有哪些,还有ECMAScript 和 JavaScript的关系介绍,感兴趣的朋友一起来看一下吧,希望对大家有帮助。

C++中const关键字的正确用法:使用const修饰函数,表示函数不会修改传入的参数或类成员。使用const声明函数指针,表示该指针指向常量函数。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
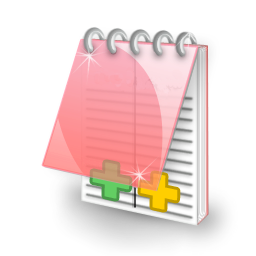
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
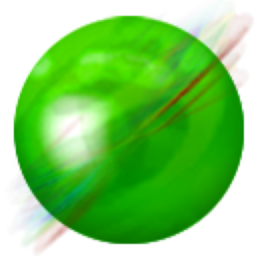
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
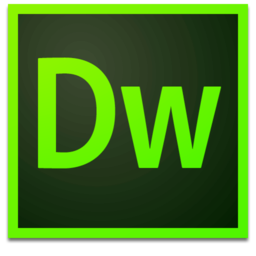
Dreamweaver Mac version
Visual web development tools
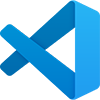
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
