1. Generic class
1.1 Ordinary generic
package test.lujianing; /** * 泛型类 * @param <T> */ class Test<T>{ private T obj; public void setValue(T obj){ this.obj =obj; } public T getValue(){ System.out.println(obj.getClass().getName()); return obj; } } /** * 测试泛型类 */ public class TestOne { public static void main(String[] args) { //测试Integer泛型 Test<Integer> t1 = new Test<Integer>(); t1.setValue(5); Integer i = t1.getValue(); System.out.println(i); //测试Double泛型 Test<Double> t2 = new Test<Double>(); t2.setValue(5.55D); Double d = t2.getValue(); System.out.println(d); //测试String泛型 Test<String> t3 = new Test<String>(); t3.setValue("hello world"); String str =t3.getValue(); System.out.println(str); } }
Output result:
java.lang.Integer 5 java.lang.Double 5.55 java.lang.String hello world
1.2 K/V generic
package test.lujianing; import java.util.HashMap; import java.util.Map; /** * Created by Administrator on 14-3-30. */ class TestKV<K,V>{ private Map<K,V> map=new HashMap<K, V>(); public void put(K k, V v) { map.put(k,v); } public V get(K k) { return map.get(k); } } public class TestFour{ public static void main(String[] args) { TestKV<String,String> t = new TestKV<String, String>(); t.put("name","jianing"); System.out.println(t.get("name")); TestKV<String,Integer> t2 = new TestKV<String, Integer>(); t2.put("age",24); System.out.println(t2.get("age")); } }
Output result :
jianing 24
2. Generic interface
package test.lujianing; /** * 泛型接口 * @param <T> */ public interface TestImpl<T> { public void setValue(T t); public T getValue(); }
Output result:
1 hello word
3. Generic method
package test.lujianing; /** * 泛型方法类 */ class TestMethod{ /** * 泛型方法 */ public <T>T getValue(Object s,Class<T> clazz) { System.out.println(clazz.getName()); T t =null; if(clazz.getName().equals("java.lang.Integer")){ Double d = Double.parseDouble(s.toString()); int i =d.intValue(); t=(T)new Integer(i); } if(clazz.getName().equals("java.lang.Double")){ t=(T)new Double(s.toString()); } return t; } } /** * 泛型方法测试类 */ public class TestThree { public static void main(String[] args) { TestMethod t = new TestMethod(); int i =t.getValue("30.0011",Integer.class); System.out.println(i); double d =t.getValue("40.0022",Double.class); System.out.println(d); } }
Output result:
java.lang.Integer 30 java.lang.Double 40.0022
4. Restrict generics
In the above example, there is no restriction on the scope of class Test
5. Wildcard generics
package test.lujianing; import java.util.HashMap; import java.util.Map; /** * 通配泛型 */ public class TestFive { public static void main(String[] args) { Map<String,Class<? extends Number>> map = new HashMap<String,Class<? extends Number>>(); map.put("Integer",Integer.class); map.put("Double",Double.class); for (Map.Entry<String,Class<? extends Number>> entry : map.entrySet()) { System.out.println("key:" + entry.getKey() + " value:" + entry.getValue()); } } }
Output results:
key:Double value:class java.lang.Double key:Integer value:class java.lang.Integer
Simple example: example for 1.1
public static void main(String[] args) { //测试Integer泛型 Test<Integer> t1 = new Test<Integer>(); t1.setValue(5); fun(t1); //测试Double泛型 Test<Double> t2 = new Test<Double>(); t2.setValue(5.55D); fun(t2); } public static void fun(Test<?> t){ System.out.println("通配泛型"+t.getValue()); }
Output results:
java.lang.Integer 通配泛型5 java.lang.Double 通配泛型5.55
6. Supplement
In generics, you may encounter
For more java generic examples and related articles, please pay attention to the PHP Chinese website!
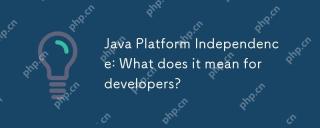
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
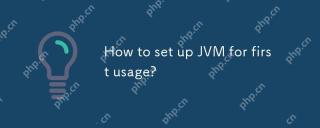
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
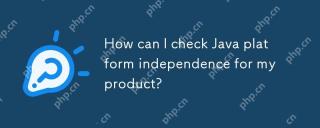
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss
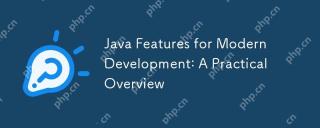
Javastandsoutinmoderndevelopmentduetoitsrobustfeatureslikelambdaexpressions,streams,andenhancedconcurrencysupport.1)Lambdaexpressionssimplifyfunctionalprogramming,makingcodemoreconciseandreadable.2)Streamsenableefficientdataprocessingwithoperationsli
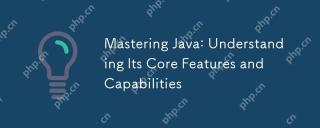
The core features of Java include platform independence, object-oriented design and a rich standard library. 1) Object-oriented design makes the code more flexible and maintainable through polymorphic features. 2) The garbage collection mechanism liberates the memory management burden of developers, but it needs to be optimized to avoid performance problems. 3) The standard library provides powerful tools from collections to networks, but data structures should be selected carefully to keep the code concise.
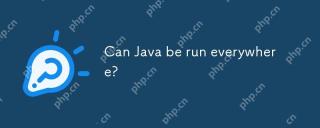
Yes,Javacanruneverywhereduetoits"WriteOnce,RunAnywhere"philosophy.1)Javacodeiscompiledintoplatform-independentbytecode.2)TheJavaVirtualMachine(JVM)interpretsorcompilesthisbytecodeintomachine-specificinstructionsatruntime,allowingthesameJava
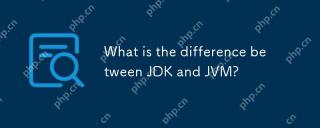
JDKincludestoolsfordevelopingandcompilingJavacode,whileJVMrunsthecompiledbytecode.1)JDKcontainsJRE,compiler,andutilities.2)JVMmanagesbytecodeexecutionandsupports"writeonce,runanywhere."3)UseJDKfordevelopmentandJREforrunningapplications.
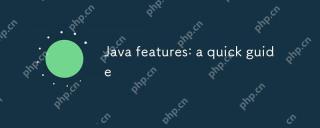
Key features of Java include: 1) object-oriented design, 2) platform independence, 3) garbage collection mechanism, 4) rich libraries and frameworks, 5) concurrency support, 6) exception handling, 7) continuous evolution. These features of Java make it a powerful tool for developing efficient and maintainable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
