The Graphics class provides basic drawing methods, and the Graphics2D class provides more powerful drawing capabilities. This section explains the Graphics class, and the next section explains Graphics2D.
The Graphics class provides basic geometric drawing methods, mainly including: drawing line segments, drawing rectangles, drawing circles, drawing colored graphics, drawing ellipses, drawing arcs, drawing polygons, etc.
1. Draw a line
To draw a line segment in the window, you can use the drawLine() method of the Graphics class:
drawLine(int x1,int y1,int x2,int y2)
For example, the following code is at point (3,3) Draw a line segment from the point (50,50) and draw a point at the point (100,100).
g.drawLine(3,3,50,50);//画一条线段 g.drawLine(100,100,100,100);//画一个点。
2. Draw a rectangle
There are two kinds of rectangles: ordinary type and rounded type.
(1) There are two methods for drawing ordinary rectangles:
drawRect(int x, int y, int width, int height): Draw a rectangle surrounded by a wireframe. The parameters x and y specify the position of the upper left corner, and the parameters width and height are the width and height of the rectangle.
fillRect(int x, int y, int width, int height): fills a rectangle with a predetermined color to obtain a colored rectangular block.
The following code is an example of drawing a rectangle:
g.drawRect(80,100,40,25);//画线框 g.setColor(Color.yellow);g.fillRect(20,70,20,30);//画着色块
(2) There are also two methods for drawing a rounded rectangle:
drawRoundRect(int x,int y,int width, int height, int arcWidth, int arcHeight): It is a rounded rectangle surrounded by lines. The parameters x and y specify the position of the upper left corner of the rectangle; the parameters width and height are the width and height of the rectangle; arcWidth and arcHeight are the transverse diameter and longitudinal diameter of the fillet arc respectively.
fillRoundRect(int x, int y, int width, int height, int arcWidth, int archeight): It is a rounded rectangle filled with a predetermined color. The meaning of each parameter is the same as the previous method.
The following code is an example of drawing a rectangle:
g.drawRoundRect(10,10,150,70,40,25);//画一个圆角矩形 g.setColor(Color.blue); g.fillRoundRect(80,100,100,100,60,40);//涂一个圆角矩形块 g.drawRoundRect(10,150,40,40,40,40);//画圆 g.setColor(Color.red); g.fillRoundRect(80,100,100,100,100,100);//画圆块
You can use the method of drawing a rounded rectangle to draw a circle. When the width and height of the rectangle are equal, the horizontal diameter of the rounded arc and the longitudinal diameter of the rounded arc Also equal and equal to the width and height of the rectangle, a circle is drawn. See the comments in the above example. The former is to draw a circle, and the latter is to paint a circle block.
3. Draw a three-dimensional rectangle
There are two methods for drawing a three-dimensional rectangle:
draw3DRect(int x, int y, int width, int height, boolean raised): Draw a highlighted rectangle. Among them, x and y specify the position of the upper left corner of the rectangle, the parameters width and height are the width and height of the rectangle, and the parameter raised is whether it is highlighted or not.
fill3DRect(int x,int y,int width,int height,boolean raised): Fill a highlighted rectangle with a predetermined color.
The following code is an example of drawing a protruding rectangle:
g.draw3DRect(80,100,40,25,true);//画一个线框 g.setColor(Color.yellow); g.fill3DRect(20,70,20,30,true);//画一个着色块
4. Draw an ellipse
The ellipse is determined by the horizontal and vertical axes of the ellipse. There are two methods for drawing an ellipse:
drawOval(int x, int y, int width, int height): draws an ellipse surrounded by lines. The parameters x and y specify the position of the upper left corner of the ellipse, and the parameters width and height are the horizontal and vertical axes.
fillOval(int x, int y, int width, int height): It is an oval filled with a predetermined color and is a coloring block. You can also use the method of drawing an ellipse to draw a circle. When the horizontal axis and the vertical axis are equal, the ellipse drawn is a circle.
The following code is an example of drawing an ellipse:
g.drawOval(10,10,60,120);//画椭圆 g.setColor(Color.cyan);g.fillOval(100,30,60,60);//涂圆块 g.setColor(Color.magenta);g.fillOval(15,140,100,50);//涂椭圆
5. Draw an arc
There are two methods for drawing an arc:
drawArc(int x,int y,int width, int height, int startAngle, int arcAngle): Draw an arc line as part of the ellipse. The center of the ellipse is the center of its enclosing rectangle, where the parameters are the coordinates (x, y) of the upper left corner of the enclosing rectangle, the width is width, and the height is height. The unit of parameter startAngle is "degree", and the starting angle of 0 degrees refers to the 3 o'clock position. The parameters startAngle and arcAngle indicate that starting from the startAngle angle, an arc of arcAngle degrees is drawn in the counterclockwise direction. By convention, positive degrees are in the counterclockwise direction. Negative degrees are clockwise, for example -90 degrees is 6 o'clock.
fillArc(int x, int y, int width, int height, int startAngle, int arcAngle): Use the color set by the setColor() method to draw a part of the colored ellipse.
The following code is an example of drawing an arc:
g.drawArc(10,40,90,50,0,180);//画圆弧线 g.drawArc(100,40,90,50,180,180);//画圆弧线 g.setColor(Color.yellow); g.fillArc(10,100,40,40,0,-270);//填充缺右上角的四分之三的椭圆 g.setColor(Color.green); g.fillArc(60,110,110,60,-90,-270);//填充缺左下角的四分之三的椭圆
6. Drawing a polygon
A polygon is a closed plane diagram formed by connecting multiple line segments end to end. The x-coordinates and y-coordinates of the endpoints of the polygon line segments are stored in two arrays respectively. To draw a polygon is to connect them with straight line segments in the order of the given coordinate points. The following are two commonly used methods for drawing polygons:
drawPolygon(int xpoints[],int yPoints[],int nPoints): Draw a polygon
fillPolygon(int xPoints[],int yPoints[],int nPoints) : Color the polygon with the color set by method setColor(). The array xPoints[] stores x coordinate points, yPoints[] stores y coordinate points, and nPoints is the number of coordinate points.
Note that the above method does not automatically close the polygon. To draw a closed polygon, the last point of the given coordinate point must be the same as the first point. The following code implements filling a triangle and drawing an octagon .
int px1[]={50,90,10,50};//首末点相重,才能画多边形 int py1[]={10,50,50,10}; int px2[]={140,180,170,180,140,100,110,140}; int py2[]={5,25,35,45,65,35,25,5}; g.setColor(Color.blue); g.fillPolygon(px1,py1,4); g.setColor(Color.red); g.drawPolygon(px2,py2,9)
You can also use polygon objects to draw polygons. Create a polygon object using the polygon class Polygon, and then use this object to draw polygons. The main method of the Polygon class:
Polygon(): Creates a polygon object with no coordinate points for the time being.
Polygon(int xPoints[],int yPoints[],int nPoints): Create a polygon object using the specified coordinate points.
addPoint(): Add a coordinate point to the Polygon object.
drawPolygon(Polygon p): Draw a polygon.
fillPolygon(Polygon p): Fills the polygon with the specified color.
例如,以下代码,画一个三角形和填充一个黄色的三角形。注意,用多边形对象画封闭多边形不要求首末点重合。
int x[]={140,180,170,180,140,100,110,100}; int y[]={5,25,35,45,65,45,35,25}; Polygon ponlygon1=new Polygon(); polygon1.addPoint(50,10); polygon1.addPoint(90,50); polygon1.addPoint(10,50); g.drawPolygon(polygon1); g.setColor(Color.yellow); Polygon polygon2 = new Polygon(x,y,8); g.fillPolygon(polygon2);
7. 擦除矩形块
当需要在一个着色图形的中间有一个空缺的矩形的情况,可用背景色填充一矩形块实现,相当于在该矩形块上使用了 “橡皮擦”.实现的方法是:
clearRect(int x,int y, int width,int height):擦除一个由参数指定的矩形块的着色。
例如,以下代码实现在一个圆中擦除一个矩形块的着色:
g.setColor(Color.blue); g.fillOval(50,50,100,100);g.clearRect(70,70,40,55);
8. 限定作图显示区域
用一个矩形表示图形的显示区域,要求图形在指定的范围内有效,不重新计算新的坐标值,自动实现超出部分不显示。方法是clipRect(int x,int y,int width,int height),限制图形在指定区域内的显示,超出部分不显示。多个限制区有覆盖时,得到限制区域的交集区域。例如,代码:
g.clipRect(0,0,100,50);g.clipRect(50,25,100,50);
相当于
g.clipRect(50,25,50,25);
9. 复制图形
利用Graphics类的方法copyArea()可以实现图形的复制,其使用格式是:
copyArea(int x,int y,int width,int height, int dx, int dy),dx和dy分别表示将图形粘贴到原位置偏移的像素点数,正值为往右或往下偏移是,负值为往左或往上偏移量。位移的参考点是要复制矩形的左上角坐标。
例如,以下代码示意图形的复制,将一个矩形的一部分、另一个矩形的全部分别自制。
g.drawRect(10,10,60,90); g.fillRect(90,10,60,90); g.copyArea(40,50,60,70,-20,80); g.copyArea(110,50,60,60,10,80);
【例】小应用程序重写update()方法,只清除圆块,不清除文字,窗口显示一个不断移动的红色方块。
import java.applet.*;
import java.awt.*;
public class Example7_3 extends Applet{
int i=1;
public void init(){
setBackground(Color.yellow);
}
public void paint(Graphics g){
i = i+8; if(i>160)i=1;
g.setColor(Color.red);g.fillRect(i,10,20,20);
g.drawString("我正学习update()方法",100,100);
try{
Thread.sleep(100);
}
catch(InterruptedException e){}
repaint();
}
public void update(Graphics g){
g.clearRect(i,10,200,100);//不清除"我正在学习update()方法"
paint(g);
}
}
一般的绘图程序要继承JFrame,定义一个JFrame窗口子类,还要继承JPanel,定义一个JPanel子类。在JPanel子类 中重定义方法paintComponent(),在这个方法中调用绘图方法,绘制各种图形。
【例】使用XOR绘图模式的应用程序。
import javax.swing.*; import java.awt.*; public class Example7_4 extends JFrame{ public static void main(String args[]){ GraphicsDemo myGraphicsFrame = new GraphicsDemo(); } } class ShapesPanel extends JPanel{ SharpesPanel(){ setBackground(Color.white); } public void paintComponent(Graphics g){ super.paintComponent(g); setBackground(Color.yellow); //背景色为黄色 g.setXORMode(Color.red); //设置XOR绘图模式,颜色为红色 g.setColor(Color.green); g.fillRect(20, 20, 80, 40); //实际颜色是green + yellow的混合色=灰色 g.setColor(Color.yellow); g.fillRect(60, 20, 80, 40); //后一半是yellow+yellow=read,前一半是yellow+灰色 g.setColor(Color.green); g.fillRect(20, 70, 80, 40); //实际颜色是green+yellow的混合色=灰色. g.fillRect(60, 70, 80, 40); //前一半是(green+yellow)+gray =背景色,后一半是green+yellow = gray g.setColor(Color.green); g.drawLine(80, 100, 180, 200); //该直线是green+yellow = gray g.drawLine(100, 100, 200, 200); //同上 /*再绘制部分重叠的直线.原直线中间段是灰色+灰色=背景色,延长部分是green+yellow=gray.*/ g.drawLine(140, 140, 220, 220); g.setColor(Color.yellow); //分析下列直线颜色变化,与早先的力有重叠 g.drawLine(20, 30, 160, 30); g.drawLine(20, 75, 160, 75); } } class GraphicsDemod extends JFrame{ public GraphicsDemo(){ this.getContentPane().add(new ShapesPanel()); setTile("基本绘图方法演示"); setSize(300, 300); setVisible(true); } }
更多使用Java的Graphics类进行绘图的方法详解相关文章请关注PHP中文网!
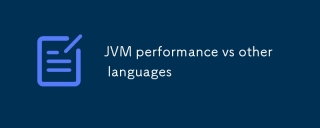
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
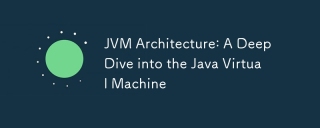
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
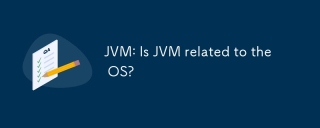
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
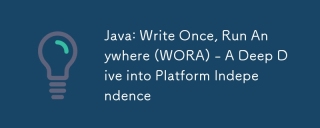
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
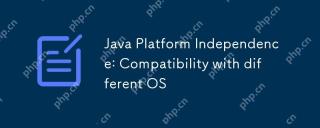
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
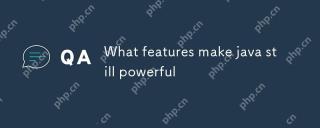
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
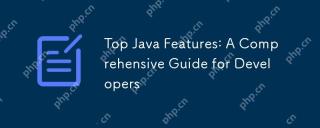
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
