The examples in this article describe the life cycle of Android activity. Share it with everyone for your reference, the details are as follows:
The activity class is in the android.app package, and the inheritance system is as follows:
1.Java.lang.Object
2. android.content.Context
3.android.app.ApplicationContext
4.android.app.Activity
activity is separate and used to handle user operations. Almost all activities have to deal with users, so the activity class creates a window. Developers can put the UI on the window created by the activity through the setContentView(View) interface. When the activity points to the full-screen window, it can also be implemented in other ways. : As a floating window (through the theme collection of windowIsFloating), or embedded in other activities (using ActivityGroup). Most Activity subclasses need to implement the following two interfaces:
① The onCreate(Bundle) interface is where the activity is initialized. Here you can usually call setContentView(int) to set the UI defined in the resource file. Use findViewById(int) to get the window defined in the UI.
② The onPause() interface is where the user is ready to leave the activity. Here, any modifications should be submitted (usually used by ContentProvider to save data ).
In order to use Context.startActivity(), all activity classes must have related "activity" items defined in the AndroidManifest.xml file.
The activity class is an important part of the Android application life cycle.
Activity life cycle
Activities in the system are managed by an Activity stack. When a new Activity is started, it will be placed on the top of the stack and become a running Activity. The previous Activity will remain in the stack and will no longer be placed in the foreground until the new Activity exits.
Activity has four essentially different states:
1. In the foreground of the screen (top of the Activity stack), it is called the active state or running state (active or running)
2. If an Activity loses focus but is still visible (a new non-full-screen Activity or a transparent Activity is placed on the top of the stack), it is called Paused. A suspended Activity remains alive (keeps all state, member information, and connection to the window manager), but will be killed if the system memory is extremely low.
3. If an Activity is completely covered by another Activity, it is called Stopped. It still retains all state and member information, but it is no longer visible, so its window is hidden, and the Stopped Activity will be killed when system memory needs to be used elsewhere.
4. If an Activity is in the Paused or Stopped state, the system can delete the Activity from the memory. The Android system uses two methods to delete it, either requiring the Activity to end or directly killing its process. When the activity is shown to the user again, it must restart and reset the previous state.
The following figure shows the important state transitions of Activity. The rectangular box indicates the callback interface of Activity between state transitions. Developers can overload the implementation to execute relevant code. The colored ovals indicate the activity's callback interface. state.
In the above figure, Activity has three key cycles:
1. The entire life cycle, starting from onCreate(Bundle) to onDestroy() Finish. Activity sets all "global" state in onCreate() and releases all resources in onDestory(). For example, if an Activity has a thread running in the background for downloading data from the network, the Activity can create the thread in onCreate() and stop the thread in onDestory().
2. The visible life cycle starts from onStart() and ends with onStop(). During this time, the Activity can be seen on the screen, although it may not be in the foreground and cannot interact with the user. Between these two interfaces, it is necessary to maintain the UI data and resources displayed to the user. For example, you can register an IntentReceiver in onStart to listen for changes in the UI caused by data changes. When the display is no longer needed, you can register it in onStop() Unregister it in . Both onStart() and onStop() can be called multiple times because the Activity can switch between visible and hidden at any time.
3. The life cycle of the foreground starts from onResume() and ends with onPause(). During this time, the activity is at the front of all activities and interacts with the user. Activity can frequently switch between resumed and paused states, for example: when the device is preparing to sleep, when an Activity processing result is dispatched, and when a new Intent is dispatched. So the code in these interface methods should be very lightweight.
The entire life cycle of Activity is defined in the following interface methods, and all methods can be overloaded. All Activities need to implement onCreate(Bundle) to initialize settings. Most Activities need to implement onPause() to submit changed data. Currently, most Activities also need to implement the onFreeze() interface to restore in onCreate(Bundle). The status of the setting.
public class Activity extends ApplicationContext { protected void onCreate(Bundle icicle); protected void onStart(); protected void onRestart(); protected void onResume(); protected void onFreeze(Bundle outIcicle); protected void onPause(); protected void onStop(); protected void onDestroy(); }
I hope this article will be helpful to everyone in Android programming.
For more detailed articles on the life cycle of activities in Android development, please pay attention to the PHP Chinese website!
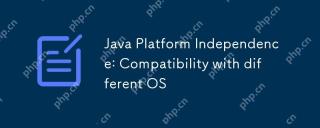
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
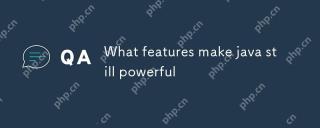
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
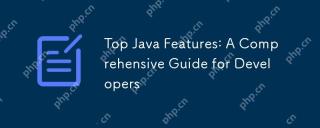
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.
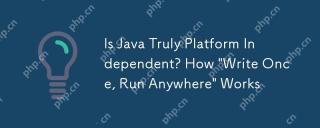
JavaisnotentirelyplatformindependentduetoJVMvariationsandnativecodeintegration,butitlargelyupholdsitsWORApromise.1)JavacompilestobytecoderunbytheJVM,allowingcross-platformexecution.2)However,eachplatformrequiresaspecificJVM,anddifferencesinJVMimpleme
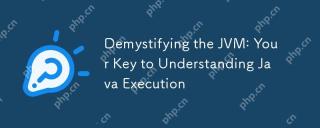
TheJavaVirtualMachine(JVM)isanabstractcomputingmachinecrucialforJavaexecutionasitrunsJavabytecode,enablingthe"writeonce,runanywhere"capability.TheJVM'skeycomponentsinclude:1)ClassLoader,whichloads,links,andinitializesclasses;2)RuntimeDataAr
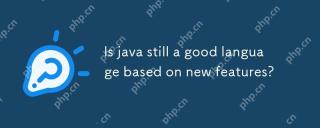
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
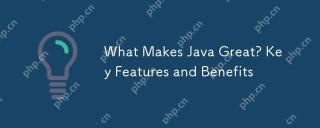
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
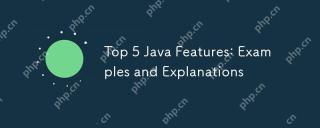
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
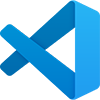
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
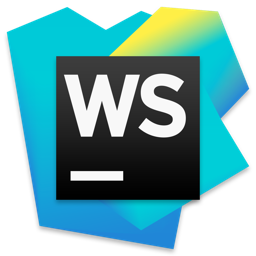
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Notepad++7.3.1
Easy-to-use and free code editor
