Business Requirements
Recently, the company requires the development of a web version of the app. Since the app is content-oriented and has a chat module, general multi-page development is not very suitable, and it is mainly for mobile browsing. It is more demanding in terms of loading speed or user experience. After researching many frameworks and models, I finally pieced together something like this.
Server
There is no doubt that using node, using typescript can effectively check errors while coding, and there is no pressure to write the server in a strongly typed language.
#app.ts 只贴重要代码 var webpack = require('webpack') var webpackDevMiddleware = require('webpack-dev-middleware') var WebpackConfig = require('./webpack.config') import * as index from "./routes/index"; import * as foo from "./routes/foo"; import * as bar from "./routes/bar"; var app = express(); //启动服务的时候 打包并监听客户端用到的文件,webpackDevMiddleware是开发模式,他会打包js在内存里面,你改了文件,它也会重新打包 app.use(webpackDevMiddleware(webpack(WebpackConfig), { publicPath: '/__build__/', stats: { colors: true } })); //一般的配置项 app.set('views', __dirname + '/views'); app.set('view engine', 'ejs'); app.set('view options', { layout: false }); app.use(bodyParser.urlencoded({ extended: true })); app.use(bodyParser.json()); app.use(methodOverride()); app.use(express.static(__dirname + '/public')); var env = process.env.NODE_ENV || 'development'; if (env === 'development') { app.use(errorHandler()); } //路由配置 app.get('/', index.index); app.get('/foo', foo.index); app.get('/bar', bar.index); app.listen(3000, function(){ console.log("Demo Express server listening on port %d in %s mode", 3000, app.settings.env); }); export var App = app;
Server-side rendering page
#index.ts import express = require("express") import vueServer = require("vue-server") //服务端渲染vue的插件 var Vue = new vueServer.renderer(); //创建一个服务端的vue export function index(req: express.Request, res: express.Response) { //创建一个组件 var vm = new Vue({ template: ` <p>This is index!</p> ` }); //等待html渲染完成,再返回给浏览器 vueServer.htmlReady是vue-server的自带事件 vm.$on('vueServer.htmlReady', function(html:string) { //这里用的是ejs模板 可以把需要用到的数据设置成window下的全局变量,方便客户端的js访问。 res.render('layout',{server_html:html,server_data:'window.cm_data = {name:"张三"}'}) }); };
#layout.ejs 访问这个SPA的所有url返回的都是这个页面 <meta>标签都可以动态设置,只要传参数进来就可以 <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>Vue Router Example</title> <style> .v-link-active { color: red; } </style> <script> //定义一些前端需要用到的全局属性,文章ID或用户信息什么的 //index.ts中传过来的是 window.cm_data = {name:"张三"} //前端就能访问到了 <%-server_data%> </script> </head> <body> //这里的id是前端需要用到的一个标识 <div id="app"> <h1 id="Hello-nbsp-App">Hello App!</h1> <p> <a v-link="{ path: '/foo' }">Go to Foo</a> <a v-link="{ path: '/bar' }">Go to Bar</a> </p> //router-view是客户端vue-router需要解析的dom //server_html是根据访问url地址生成的html,是做SEO的重点,不加载下面的app.js也可以看到内容 <router-view> <%-server_html%> </router-view> </div> //webpack打包好的js,主要是路由配置 <script src="/__build__/app.js"></script> </body> </html>
Client
#app.js 这个是/__build__/app.js,可以用es6编写,webpack会转换的 import Vue from './vue.min' //客户端的vue.js import VueRouter from './vue-router.min' //vue的路由插件,配合webpack可以很简单实现懒加载 //懒加载路由 只有访问这个路由才会加载js import Foo from 'bundle?lazy!../../components/foo' //配合webpack的bundle-loader,轻松实现懒加载 import Bar from 'bundle?lazy!../../components/bar' import Index from 'bundle?lazy!../../components/index' var App = Vue.extend({}) Vue.use(VueRouter) var router = new VueRouter({ //这里要好好说一下,一定要设置html5模式,不然前后端URL不统一会发生问题 //比如访问 http://localhost:3000/ 服务端定义是访问index.ts这个路由文件 //如果不是html5模式的话,经过客户端js运行之后会变成http://localhost:3000/#!/ //在比如直接浏览器输入 http://localhost:3000/foo 服务端定义是访问.ts这个路由文件 //如果不是html5模式的话,经过客户端js运行之后会变成 http://localhost:3000/foo/#!/ //设置了html5模式后,加载完js后不会加上#!这2个类似锚点的字符,实现前后端路由统一如果用户刷新浏览器的话,服务端也能渲染出相应的页面。 history: true, //html5模式 去掉锚点 saveScrollPosition: true //记住页面的滚动位置 html5模式适用 }) //定义路由,要和服务端路由路径定义的一样 router.map({ '/' : { component: Index //前端路由定义, }, '/foo': { component: Foo }, '/bar': { component: Bar } }) //启动APP router.start(App, '#app')
What needs to be improved
Unify the front-end and back-end templates, and have found a way to separate the html , the node side uses the fs.readFileSync method to obtain it, and the client side uses webpack's raw-loader to obtain the html content
For more articles related to using node+vue.js to implement SPA applications, please pay attention to the PHP Chinese website!
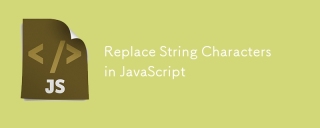
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
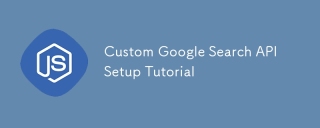
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
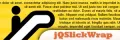
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
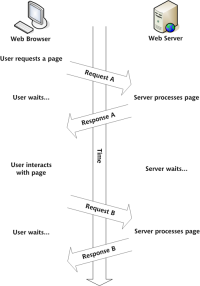
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
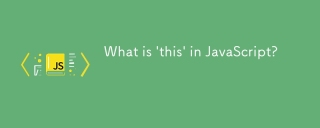
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was
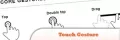
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
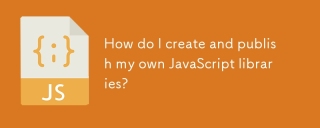
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
