If you are familiar with web development on .NET or other similar platforms, you may be like, what is the point of setting up a web server? Create a web project in Visual Studio and click to run. This is indeed the case, but please don't forget that the cost is that, for example, if you use .NET to develop a Web application, you use a complete IIS as your Web server foundation, so that when your application is released, it will I can only use IIS. And if you use a stand-alone server (built by yourself using System.Web.Hosting), you have to deal with various HttpListeners and corresponding threads, which will be more troublesome. After all, .NET is not focused on the Web. Node.js provides a convenient and customizable approach in this regard, on which you can build a sophisticated service platform that is fully oriented to your application.
1. Establishing a simple web server involves some basic knowledge points of Node.js:
1. Request module
In Node.js, the system provides many Useful modules (of course you can also write your own modules in JavaScript, we will explain them in detail in future chapters), such as http, url, etc. Modules encapsulate specific functions and provide corresponding methods or properties. To use these modules, you need to first request the module to obtain its operation object.
For example, if you want to use the system's http module, you can write:
var libHttp = require('http'); //请求HTTP协议模块
In this way, future programs will be able to access the functions of the http module through the variable libHttp. The following system modules are used in the routines in this chapter:
http: encapsulates the server and client implementation of the http protocol;
url: encapsulates the parsing and processing of urls;
fs: encapsulates the function of file system operations ;
path: Encapsulates the path parsing function.
With these modules, we can build our own applications standing on the shoulders of giants.
2. Console
In order to better observe the running of the program and to check errors when exceptions occur, you can use the console function by passing the variable console.
console.log('这是一段日志信息'); 计时并在控制台上输出计时信息: //开始计时 console.timeEnd('计时器1'); //开始名称为“计时器1”的计时器 ... ... ... //结束计时,并输出到控制台 console.timeEnd('计时器1'); //结束称为“计时器1”的计时器并输出
3. Define functions
The way to define functions in Node.js is exactly the same as in ordinary JavaScript, but our recommended writing method is as follows, that is, using a variable to name the function, which can be more convenient and clear Pass functions as parameters to other functions:
//定义一个名为showErr的函数 var showErr=function(msg){ var inf="错误!"+msg; console.log(inf+msg); return msg; }
4. Create a Web server and listen for access requests
The most important thing about creating a Web server is to provide a response function for Web requests, which has two parameters, The first represents the information requested by the client, and the other represents the information that will be returned to the client. In the response function, the request information should be parsed, and the returned content should be assembled according to the request.
//请求模块 var libHttp = require('http'); //HTTP协议模块 //Web服务器主函数,解析请求,返回Web内容 var funWebSvr = function (req, res){ res.writeHead(200, {'Content-Type': 'text/html'}); res.write('<html><body>'); res.write('<h1 id="nbsp-Node-js-nbsp">*** Node.js ***</h1>'); res.write('<h2 id="Hello">Hello!</h2>'); res.end('</body></html>'); } //创建一个http服务器 var webSvr=libHttp.createServer(funWebSvr); //开始侦听8124端口 webSvr.listen(8124);
5. Parse Web requests
For simple Web page access requests, important information is contained in the URL of the request information parameters. We can use the URL parsing module to parse the access path in the URL and use The path module assembles the access path into the actual file path to be accessed for return.
var reqUrl=req.url; //获取请求的url //向控制台输出请求的路径 console.log(reqUrl); //使用url解析模块获取url中的路径名 var pathName = libUrl.parse(reqUrl).pathname; //使用path模块获取路径名中的扩展名 if (libPath.extname(pathName)=="") { //如果路径没有扩展名 pathName+="/"; //指定访问目录 } if (pathName.charAt(pathName.length-1)=="/"){ //如果访问目录 pathName+="index.html"; //指定为默认网页 } //使用路径解析模块,组装实际文件路径 var filePath = libPath.join("./WebRoot",pathName);
6. Set the return header
Since it is a Web request, the http return header needs to be included in the return content. The focus here is to set the content of the http return header according to the file extension of the file path to be accessed. type.
var contentType=""; //使用路径解析模块获取文件扩展名 var ext=libPath.extname(filePath); switch(ext){ case ".html": contentType= "text/html"; break; case ".js": contentType="text/javascript"; break; ... ... default: contentType="application/octet-stream"; } //在返回头中写入内容类型 res.writeHead(200, {"Content-Type": contentType });
7. Write the accessed file content into the returned object
With the actual path of the file that needs to be accessed and the content type corresponding to the file, you can use the fs file system module to read the file stream and returned to the client.
//判断文件是否存在 libPath.exists(filePath,function(exists){ if(exists){//文件存在 //在返回头中写入内容类型 res.writeHead(200, {"Content-Type": funGetContentType(filePath) }); //创建只读流用于返回 var stream = libFs.createReadStream(filePath, {flags : "r", encoding : null}); //指定如果流读取错误,返回404错误 stream.on("error", function() { res.writeHead(404); res.end("<h1 id="nbsp-Read-nbsp-Error">404 Read Error</h1>"); }); //连接文件流和http返回流的管道,用于返回实际Web内容 stream.pipe(res); } else { //文件不存在 //返回404错误 res.writeHead(404, {"Content-Type": "text/html"}); res.end("<h1 id="nbsp-Not-nbsp-Found">404 Not Found</h1>"); } });
2. Testing and operation
1. Complete source code
The following 100 lines of JavaScript are all the source code to create such a simple web server:
//------------------------------------------------ //WebSvr.js // 一个演示Web服务器 //------------------------------------------------ //开始服务启动计时器 console.time('[WebSvr][Start]'); //请求模块 var libHttp = require('http'); //HTTP协议模块 var libUrl=require('url'); //URL解析模块 var libFs = require("fs"); //文件系统模块 var libPath = require("path"); //路径解析模块 //依据路径获取返回内容类型字符串,用于http返回头 var funGetContentType=function(filePath){ var contentType=""; //使用路径解析模块获取文件扩展名 var ext=libPath.extname(filePath); switch(ext){ case ".html": contentType= "text/html"; break; case ".js": contentType="text/javascript"; break; case ".css": contentType="text/css"; break; case ".gif": contentType="image/gif"; break; case ".jpg": contentType="image/jpeg"; break; case ".png": contentType="image/png"; break; case ".ico": contentType="image/icon"; break; default: contentType="application/octet-stream"; } return contentType; //返回内容类型字符串 } //Web服务器主函数,解析请求,返回Web内容 var funWebSvr = function (req, res){ var reqUrl=req.url; //获取请求的url //向控制台输出请求的路径 console.log(reqUrl); //使用url解析模块获取url中的路径名 var pathName = libUrl.parse(reqUrl).pathname; if (libPath.extname(pathName)=="") { //如果路径没有扩展名 pathName+="/"; //指定访问目录 } if (pathName.charAt(pathName.length-1)=="/"){ //如果访问目录 pathName+="index.html"; //指定为默认网页 } //使用路径解析模块,组装实际文件路径 var filePath = libPath.join("./WebRoot",pathName); //判断文件是否存在 libPath.exists(filePath,function(exists){ if(exists){//文件存在 //在返回头中写入内容类型 res.writeHead(200, {"Content-Type": funGetContentType(filePath) }); //创建只读流用于返回 var stream = libFs.createReadStream(filePath, {flags : "r", encoding : null}); //指定如果流读取错误,返回404错误 stream.on("error", function() { res.writeHead(404); res.end("<h1 id="nbsp-Read-nbsp-Error">404 Read Error</h1>"); }); //连接文件流和http返回流的管道,用于返回实际Web内容 stream.pipe(res); } else { //文件不存在 //返回404错误 res.writeHead(404, {"Content-Type": "text/html"}); res.end("<h1 id="nbsp-Not-nbsp-Found">404 Not Found</h1>"); } }); } //创建一个http服务器 var webSvr=libHttp.createServer(funWebSvr); //指定服务器错误事件响应 webSvr.on("error", function(error) { console.log(error); //在控制台中输出错误信息 }); //开始侦听8124端口 webSvr.listen(8124,function(){ //向控制台输出服务启动的信息 console.log('[WebSvr][Start] running at http://127.0.0.1:8124/'); //结束服务启动计时器并输出 console.timeEnd('[WebSvr][Start]'); });
2. Resource directory
Since we want to establish a Web server, we need to create a WebRoot directory to store actual web pages and image resources. The directory name of "WebRoot" is used in the above source code to assemble the actual file path.
3. Run and test
Enter in the command line:
node.exe WebSvr.js
Our Web server is now running. At this time, it can be accessed through the browser. The operation effect is as follows:
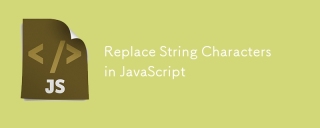
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
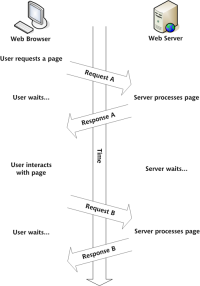
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
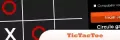
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
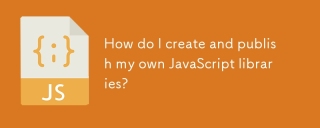
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
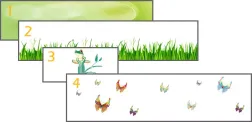
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
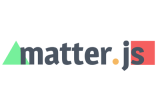
Matter.js is a 2D rigid body physics engine written in JavaScript. This library can help you easily simulate 2D physics in your browser. It provides many features, such as the ability to create rigid bodies and assign physical properties such as mass, area, or density. You can also simulate different types of collisions and forces, such as gravity friction. Matter.js supports all mainstream browsers. Additionally, it is suitable for mobile devices as it detects touches and is responsive. All of these features make it worth your time to learn how to use the engine, as this makes it easy to create a physics-based 2D game or simulation. In this tutorial, I will cover the basics of this library, including its installation and usage, and provide a
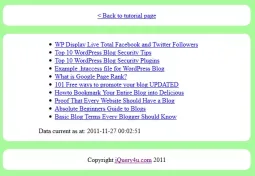
This article demonstrates how to automatically refresh a div's content every 5 seconds using jQuery and AJAX. The example fetches and displays the latest blog posts from an RSS feed, along with the last refresh timestamp. A loading image is optiona

The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
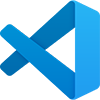
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.