Java exceptions are divided into two types, checked exceptions and unchecked exceptions. Another name is exceptions and errors.
Simply put, checked can be recovered during execution, while unchecked exceptions are errors that cannot be handled.
checked exception:
means invalid and cannot be predicted in the program. For example, invalid user input, file does not exist, network or database link error. These are all external reasons and cannot be controlled within the program.
Must be handled explicitly in the code. For example, try-catch block processing, or add a throws description to the method to throw the exception to the upper layer of the call stack.
Inherited from java.lang.Exception (except java.lang.RuntimeException).
unchecked exception:
indicates an error, a logical error in the program. Is a subclass of RuntimeException, such as IllegalArgumentException, NullPointerException and IllegalStateException.
There is no need to explicitly catch unchecked exceptions in the code for processing.
Inherits from java.lang.RuntimeException (and java.lang.RuntimeException inherits from java.lang.Exception).
The checked exception in Java needs to be explicitly caught or re-thrown by try-catch in the code. If you do not need to handle this exception, you can simply throw the exception again. This exception exists One drawback is that many people are accustomed to writing an empty catch block directly in the code. This not only makes the code redundant and "ugly", but also brings trouble to debugging and increases the difficulty of code maintenance. So some people say that checked makes the code verbose, and empty catch blocks are meaningless, so checked exceptions should be removed from the Java standard. For example, there is no concept of checked exceptions in C#, and C# does not force explicit catching of exceptions.
The reason why Java exceptions are divided into these two types should be due to the following considerations:
Checked exceptions can help developers realize which lines may cause exceptions, because Java's API It has been explained which methods may be called that may throw exceptions. If it is not processed, the compilation will not pass. To a certain extent, this approach can avoid some errors in the program.
Two simple examples
checked exception
import java.io.BufferedReader; import java.io.File; import java.io.FileReader; public class Main { public static void main(String[] args) { File f = new File("C:\test.txt"); FileReader r = new FileReader(f); //A BufferedReader br = new BufferedReader(r); br.readLine(); //B br.close(); //C } }
This code cannot be compiled because of A and B and C lines will throw IOException. You must put this code into a try-catch block, or add throws IOException to the main method to compile.
2. Unchecked exception
public class Main { public static void main(String[] args) { int a = 0; int b = 100; int c = b/a; } }
Can be compiled, but an error will be reported during execution
Exception in thread “main” java.lang.ArithmeticException: / by zero at Main.main(Main.java:13)
ArithmeticException is an unchecked exception.
Custom exception
1. checked exception
Custom exception class InvalidUrlException
public class InvalidUrlException extends Exception { public InvalidUrlException(String s){ super(s); } }
public class Main { public static void getRemoteData(String url) throws InvalidUrlException{ if(isValidUrl(url)){ //获取远程数据 } else throw new InvalidUrlException("Invalid URL: " + url); } public static boolean isValidUrl(String url){ .... //验证URL是否有效 } public static void main(String[] args) { getRemoteData(args[0]); } }
If you call getRemoteData in the main method, there are two methods, One is try-catch, and the other is to directly add throws InvalidUrlException to main.
2. unchecked exception
If you change InvalidUrlException to extends RuntimeException
public class InvalidUrlException extends Exception { public InvalidUrlException(String s){ super(s); } }
, then main does not need to add throws or try-catch.
Select checked or unchecked exception?
Some Java books recommend using checked exception handlers for all recoverable exceptions and unchecked exceptions for unrecoverable errors. But in fact, most of the Java exceptions inherited from RuntimeException can also be recovered in the program. For example, NullPointerException, IllegalArgumentExceptions, divide-by-0 exceptions, etc. can be captured and processed to allow the program to continue running. Only some special circumstances will disrupt the execution of the program, such as reading the configuration file during startup. If the configuration file does not exist or there is a serious error, the program has to exit.
The following are some arguments for and against checked exceptions:
The compiler forces the catching or throwing of unchecked exceptions so that developers always remember to handle exceptions.
Methods that throw checked exceptions must declare throws. Throws becomes part of the method or interface, which brings inconvenience to subsequent versions when adding or modifying method exceptions.
Unchecked exceptions do not need to be handled explicitly but make exception handling difficult.
When calling a checked exception method, you must handle the exception of this method, which confuses the upper caller code.
It is up to you to decide whether to choose checked or unchecked. It is difficult to say which one is definitely correct, and vice versa. Among the more popular languages at present, Java seems to be the only one that supports checked exceptions, while other languages only have unchecked exceptions.
The above is the content of Java’s checked and unchecked exceptions. For more related content, please pay attention to the PHP Chinese website (www.php.cn)!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
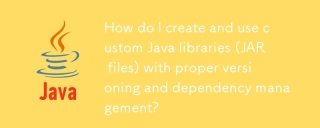
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
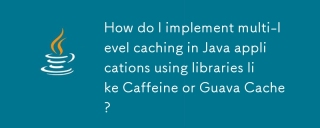
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
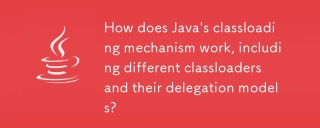
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
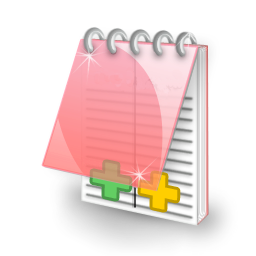
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
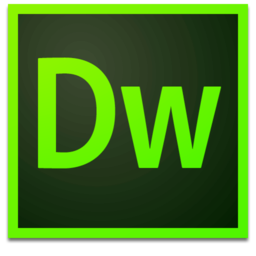
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor