


1. Object-oriented feature two: inheritance
Definition:
means that one object directly uses the properties and methods of another object.
Benefits of inheritance:
1. Provide code reusability.
2. Let classes have a direct relationship with each other, providing a prerequisite for the third characteristic polymorphism.
Single inheritance is supported in java. It does not directly support multiple inheritance, but improves the multiple inheritance mechanism in C++.
Single inheritance: A subclass can only have one parent class.
Multiple inheritance: A subclass can have multiple direct parent classes (not allowed in java, improved, not directly supported, because multiple parent classes have the same members, which will cause calling uncertainty, in java it is It is reflected by the "multi-implementation" method.
Java supports multi-level (multiple) inheritance (for example, if C inherits B and B inherits A, an inheritance system will appear.)
(No nonsense: when you want to use an inheritance. When building a system, 1 look at the top-level classes in the class to understand the basic functions of the system. 2. Create the subclass object in the system to complete the function use)
When is inheritance defined? When there is an ownership relationship between them, it is defined as inheritance (xxx is a type of yyy; xxx extends yyy)
When members of this class have the same name as local variables, use this to distinguish them.
When the member variables in the child and parent classes have the same name. The usage of super is very similar to the same name.
this: represents a reference to an object of this class.
2. Function. Override
Concept: When the member function is exactly the same in the subclass, the function of the subclass will be run. This phenomenon is called an override operation.
Two characteristics of functions:
1. In the same class. Overload 2. Override. Override
1. Overriding in subclasses. When using a parent class method, the subclass permissions must be greater than or equal to the parent class's permissions. (When the parent class method is private, it is not called an override.)
2. Static can only override static, or be overridden by static.
When extending a class into a subclass, the subclass needs to retain the function declaration of the parent class, but when you want to define the unique content of the function in the subclass, use the override operation to complete.
Sub-parent class Characteristics of the constructor in
When the subclass constructs the object, it is found that the parent class also runs when accessing the subclass constructor. Why?
Why do we need to access the constructor when the subclass is instantiated? What about the constructor in the parent class?
That’s because the subclass inherits the parent class and obtains the content (properties) of the parent class, so before using the parent class’s content, you must first see how the parent class initializes its own content. . So when constructing an object, the subclass must access the constructor in the parent class. In order to complete this necessary action, the super() statement is added to the constructor of the subclass.
If there is no empty parameter constructor defined in the parent class, then the constructor of the subclass must use super to specify which constructor in the parent class is to be called. At the same time, if this is used to call the constructor of this class in the subclass constructor, then super will be gone, because both super and this can only be defined in the first line. So there can only be one. But what is guaranteed is that there will definitely be other constructors in the subclass that access the constructor of the parent class.
Note: The supre statement must be defined in the first line of the subclass constructor. Because the initialization action of the parent class must be completed first.
An object instantiation process:
For example, Person p = new Person();
1, the JVM will read the Person.class file in the specified path and load it into the memory, and will load the parent class of Person first (If there is a direct parent class).
2. Create space in the heap memory and assign an address.
3, and in the object space, initialize the properties in the object by default.
4. Call the corresponding constructor for initialization.
5. In the constructor, the first line will first call the constructor in the parent class for initialization.
6. After the parent class is initialized, the attributes of the subclass are displayed and initialized.
7, then perform specific initialization of the subclass constructor.
8. After initialization, assign the address value to the reference variable.
Picture (steps are as follows):
3.final keyword
1.final is a modifier that can modify the class, methods, variables.
2. Final modified classes cannot be inherited.
3.Final modified methods cannot be overridden. (Note here the difference with static. If the subclass is static, it can still be overridden)
4. The variable modified by final is a constant and can only be assigned once.
Why should we use final to modify variables? In fact, if a piece of data is fixed in the program, then it can be used directly. However, this is not readable, so the data is given a name. Moreover, the value of this variable name cannot change, so it is fixed by adding final.
Standard writing: all letters of constants should be in capital letters, multiple words, and linked with _ in the middle.
Disadvantages of inheritance:
The disadvantages of inheritance break encapsulation (the solution can be to use the modifier final for final declaration)
4. Abstract class
Abstract: vague, general, incomprehensible, not specific.
Features:
1. When a method is only declared but not implemented, the method is an abstract method and needs to be modified by abstract. Abstract methods must be defined in an abstract class, and the class must also be modified by abstract.
2. Abstract classes cannot be instantiated. (Reason: Because it is meaningless to call abstract methods)
3. An abstract class must have all abstract methods covered by its subclasses before the subclass can be instantiated. Otherwise, this subclass is still an abstract class.
Question understanding:
1. Is there a constructor in an abstract class? Some are used to initialize subclass objects.
2. Can an abstract class not define abstract methods? It's possible, but it's rare. The purpose is to prevent this class from creating objects. AWT's adapter object is this class. Usually the methods in this class have a method body, but no content. eq: void show(){}This type has no method body
3. Which keywords cannot the abstract keyword coexist with?
private does not work, because private is only known to the parent class, and abstraction needs to be overridden, but subclasses cannot override it, so it cannot coexist with private.
static No, because if the member is static, it can be called directly with the class name, but the method is not a specific method body in the abstract, so it cannot coexist.
final No. Because final sets members and classes cannot be overridden or inherited, they cannot coexist.
4. What are the similarities and differences between abstract classes and general classes?
Similar points: abstract classes and general classes are both used to describe things, and both have internal members.
Differences: 1. General classes have enough information to describe things, while abstract classes may not have enough information to describe things.能 2. The abstraction method cannot be defined in the general class, and only non -abstract methods can be defined; abstract categories can be defined as abstract methods, and non -abstract methods can be defined.
3. General classes can be instantiated, but abstract classes cannot be instantiated.
5. Does an abstract class have to be a parent class? Yes, because the subclass needs to override its method before the subclass can be instantiated.
The above is the basic introductory essay on Java (11) JavaSE version - the content of inheritance, overwriting, and abstract classes. For more related content, please pay attention to the PHP Chinese website (www.php.cn)!
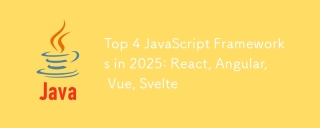
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
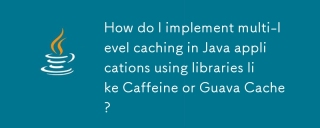
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
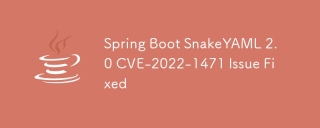
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
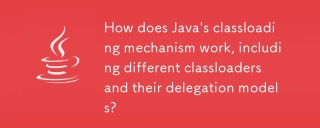
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
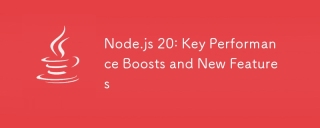
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
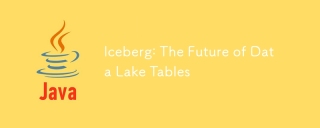
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
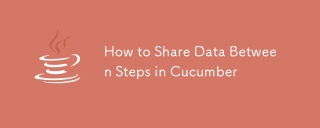
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
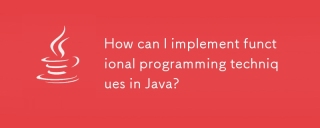
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
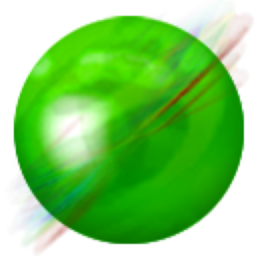
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
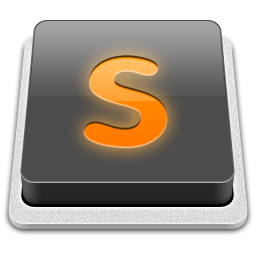
SublimeText3 Mac version
God-level code editing software (SublimeText3)
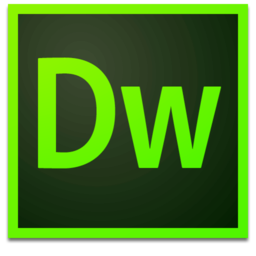
Dreamweaver Mac version
Visual web development tools
