Let’s look at some important features related to scope chains and function [[scope]] attributes.
Closures
In ECMAScript, closures are directly related to the [[scope]] of a function. As we mentioned, [[scope]] is stored when the function is created and will live and die with the function. In fact, a closure is the combination of the function code and its [[scope]]. Therefore, [[Scope]] includes as one of its objects the lexical scope (parent variable object) created within the function. When the function is further activated, within this lexical chain of variable objects (which are stored statically at creation time), variables from higher scopes will be searched. For example:
var x = 10; function foo() { alert(x); } (function () { var x = 20; foo(); // 10, but not 20 })();
We see again that during identifier resolution, using the lexical scope defined when the function was created - the variable resolves to 10 instead of 30. Furthermore, this example also clearly shows that the [[scope]] of a function (in this case an anonymous function returned from function "foo") persists even after the scope created by the function has been completed.
More details about the theory of closures in ECMAScript and its execution mechanism will be explained later.
The [[scope]] of the function created through the constructor
In the above example, we see that the [[scope]] attribute of the function is obtained when the function is created, and all the variables of the parent context can be accessed through this attribute . However, there is an important exception to this rule, and it involves functions created through function constructors.
var x = 10; function foo() { var y = 20; function barFD() { // 函数声明 alert(x); alert(y); } var barFE = function () { // 函数表达式 alert(x); alert(y); }; var barFn = Function('alert(x); alert(y);'); barFD(); // 10, 20 barFE(); // 10, 20 barFn(); // 10, "y" is not defined } foo();
We see that the function "bar" created through the function constructor cannot access the variable "y". But this does not mean that the function "barFn" does not have the [[scope]] attribute (otherwise it cannot access the variable "x"). The problem is that the [[scope]] attribute of a function created via the function constructor is always the only global object. With this in mind, it is not possible to create a top-level context closure other than the global one through such a function.
Two-dimensional scope chain search
The most important point in searching in the scope chain is that the properties of the variable object (if any) must be taken into account - derived from the prototype feature of ECMAScript. If a property is not found directly in the object, the query continues in the prototype chain. That is often called two-dimensional chain search. (1) Scope chain link; (2) Each scope chain - going deep into the prototype chain link. We can see this effect if the properties are defined in Object.prototype.
function foo() { alert(x); } Object.prototype.x = 10; foo(); // 10
Active objects do not have prototypes, we can see in the example below:
function foo() { var x = 20; function bar() { alert(x); } bar(); } Object.prototype.x = 10; foo(); // 20
If the activation object of the function "bar" context has a prototype, then "x" will be resolved in Object.prototype because it is in It is not directly parsed in AO. But in the first example above, during identifier resolution, we reach the global object (not always the case in some implementations), which inherits from Object.prototype, and accordingly, "x" resolves to 10.
The same situation occurs with named function expressions (abbreviated as NFE) in some versions of SpiderMokey, where a specific object stores the optional name of a function expression inherited from Object.prototype, and in some versions of Blackberry version, the execution-time activation object inherits from Object.prototype.
Scope chains in global and eval contexts
Not necessarily interesting here, but a reminder is necessary. The global context's scope chain contains only global objects. The context of code eval has the same scope chain as the current calling context.
globalContext.Scope = [ Global ]; evalContext.Scope === callingContext.Scope;
The impact on the scope chain during code execution
In ECMAScript, there are two statements that can modify the scope chain during the code execution phase. This is the with statement and catch statement. They are added to the front of the scope chain, and objects must be looked up among the identifiers that appear in these declarations. If one of these occurs, the scope chain is briefly modified as follows:
Scope = withObject|catchObject + AO|VO + [[Scope]]
In this example, add the object as its parameter (so that, without the prefix, the object's properties become accessible).
var foo = {x: 10, y: 20}; with (foo) { alert(x); // 10 alert(y); // 20 }
The scope chain is modified like this:
Scope = foo + AO|VO + [[Scope]]
We see again that through the with statement, the resolution of the identifier in the object is added to the front end of the scope chain:
var x = 10, y = 10; with ({x: 20}) { var x = 30, y = 30; alert(x); // 30 alert(y); // 30 } alert(x); // 10 alert(y); // 30
在进入上下文时发生了什么?标识符“x”和“y”已被添加到变量对象中。此外,在代码运行阶段作如下修改:
x = 10, y = 10;
对象{x:20}添加到作用域的前端;
在with内部,遇到了var声明,当然什么也没创建,因为在进入上下文时,所有变量已被解析添加;
在第二步中,仅修改变量“x”,实际上对象中的“x”现在被解析,并添加到作用域链的最前端,“x”为20,变为30;
同样也有变量对象“y”的修改,被解析后其值也相应的由10变为30;
此外,在with声明完成后,它的特定对象从作用域链中移除(已改变的变量“x”--30也从那个对象中移除),即作用域链的结构恢复到with得到加强以前的状态。
在最后两个alert中,当前变量对象的“x”保持同一,“y”的值现在等于30,在with声明运行中已发生改变。
同样,catch语句的异常参数变得可以访问,它创建了只有一个属性的新对象--异常参数名。图示看起来像这样:
try { ... } catch (ex) { alert(ex); }
作用域链修改为:
var catchObject = { ex: <exception object> }; Scope = catchObject + AO|VO + [[Scope]]
在catch语句完成运行之后,作用域链恢复到以前的状态。
结论
在这个阶段,我们几乎考虑了与执行上下文相关的所有常用概念,以及与它们相关的细节。按照计划--函数对象的详细分析:函数类型(函数声明,函数表达式)和闭包。顺便说一下,在这篇文章中,闭包直接与[[scope]]属性相关,但是,关于它将在合适的篇章中讨论。
以上就是JavaScript作用域链其三:作用域链特征的内容,更多相关内容请关注PHP中文网(www.php.cn)!
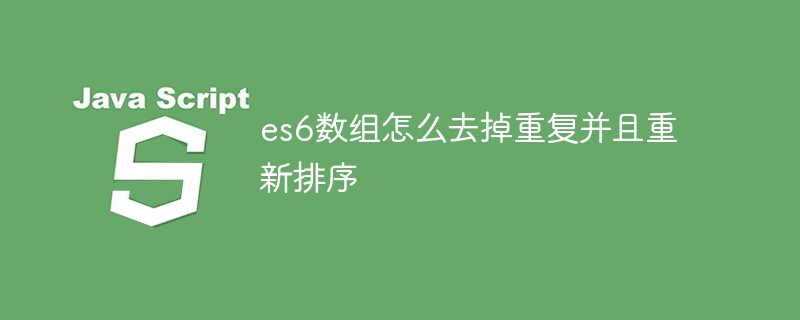
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
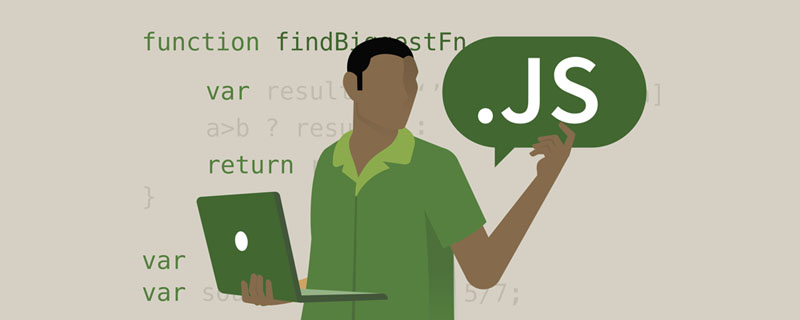
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
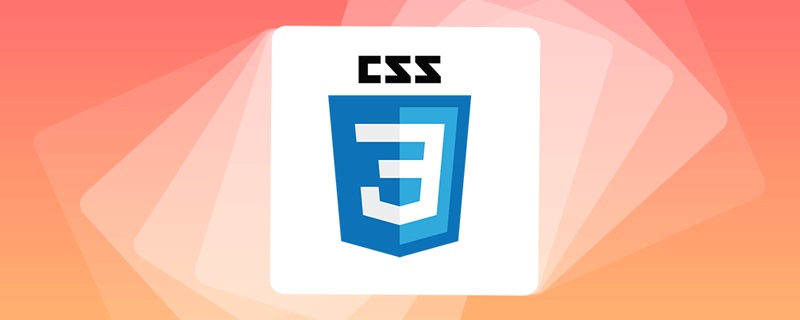
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
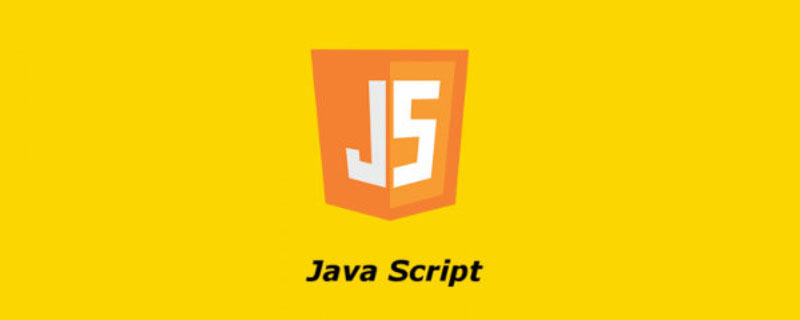
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
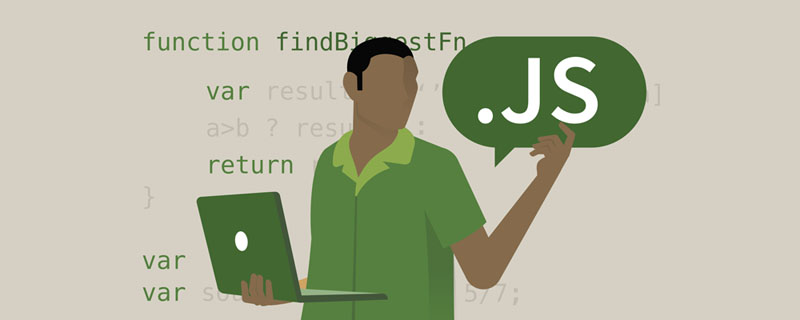
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
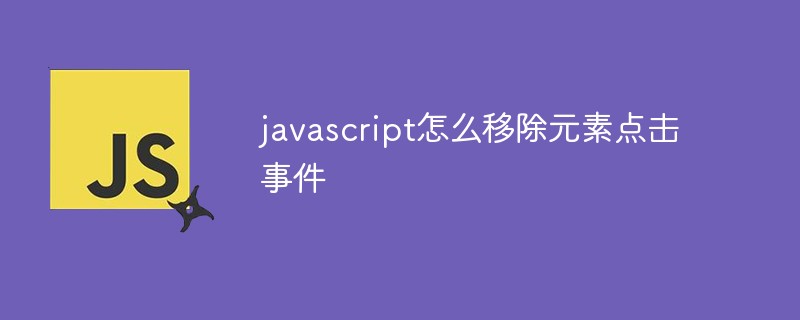
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
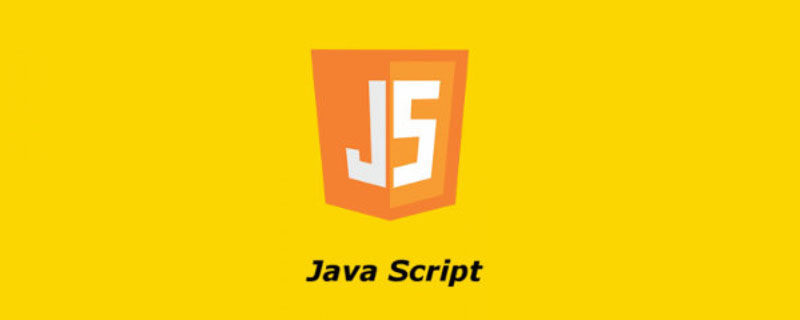
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
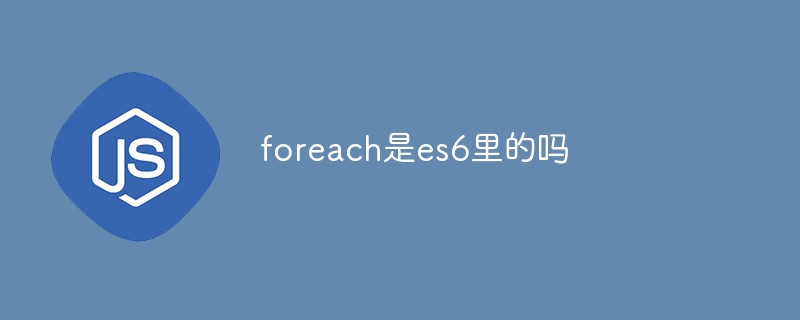
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
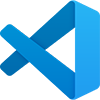
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
