Hello everyone, I am SUNWEN from Wuhan Chinese Normal University. Haha, I finally had time to go out and play in the afternoon. I arrived at Guiyuan Temple. The ticket is ten yuan, which is not expensive. SUNWEN took a lot of photos. It won’t take long for everyone to see. Take a look.
Without further ado, let’s get to the point. What I want to tell you this time is the attributes in C#. What are attributes? For example, I am a male, and male is my attribute. I am A freshman student, freshman is also an attribute of mine. An attribute is the property of an object. It’s very simple, haha! Below, I will give two examples. The first example shows how to declare an attribute that can be modified. Another example creates an abstract property (Abstract) and shows how to discard it in a subclass. OK, let’s get started.
Example 1:
000: // PRopertiesperson.cs
001 : using System;
002: class Person
003: {
004: private string myName ="N/A";
005: private int myAge = 0;
006:
007: // Declare a character attribute Name
008: public string Name
009: {
010: get
011: {
012: return myName;
013: }
014: set
015: {
016: myName = value;
017: }
018: }
019:
020: // Declare an Age property of type int
021: public int Age
022: {
023: get
024: {
025: return myAge;
026: }
027: set
028 : {
029: myAge = value;
030: }
031: }
032:
033: public override string ToString()
034: {
035: return "Name = " + Name + ", Age = " + Age;
036: }
037:
038: public static void Main()
039: {
040: Console.WriteLine("Simple Properties");
041:
042: // Create a Person instance
043 : Person person = new Person();
044:
045: //Print out its properties
046: Console.WriteLine("Person details - {0}", person);
047:
048: // Right Make some settings for the properties
049: person.Name = "Joe";
050: person.Age = 99;
051: Console.WriteLine("Person details - {0}", person);
052:
053: / / Add age
054: person.Age += 1;
055: Console.WriteLine("Person details - {0}", person);
056: }
057: }
The output of this example is:
Simple Properties
Person details - Name = N/A, Age = 0
Person details - Name = Joe, Age = 99
Person details - Name = Joe, Age = 100
Okay, I got up again. I wrote this yesterday, I ran to bed and fell asleep, haha. It’s the second day of May Day. Let’s see how many articles I can write today. I wrote two articles yesterday.
From the above program, we can see that the setting of attributes of a class , borrowing the concept of VB, it is different from Java. (This is M$, TMD!) Some friends may be wondering why we can use Console.WriteLine() to print an object person. In fact, it makes sense Simple, just like in JAVA, when the regulator uses a print method, the object automatically calls its ToString() (toString in JAVA, TMD, almost wrong again!) method. On line 33, we can see There is a shadow of this method. The override keyword is probably a method that overrides the parent class. Isn’t this a bit redundant? We can see that the setting of an object’s attributes is completed through a combination of get() and set(). , of course, there is also something called value. In addition, you can also control the read/write permissions of an attribute, just simply remove get() and set(). For example, if you don't want to write attributes, just Just don’t set(), if you don’t want to read, don’t get(). I always feel that C# is not as flexible as JAVA in this regard (it’s over, I’m going to get beat up again!).
Second Example:
This example illustrates how to create abstract attributes (Abstract). What are abstract attributes? The so-called abstract attributes are... (Oh, there is so much nonsense every time! FT) A Abstract classes do not provide execution attribute access procedures, and they can be ignored in subclasses. The following example has three files. You have to compile them separately to get the results. They are:
abstractshape.cs: Shape class, Contains an Area abstract property
shapes.cs: a subclass of Shape
shapetest.cs: display program.
To compile these programs, run: csc abstractshape.cs shapes.cs shapetest.cs. After running, it will Generate the executable program shapetest.exe.
000: // Propertiesabstractshape.cs
001: using System;
002:
003: public abstract class Shape
004: {
005: private string myId;
006:
007: public Shape(string s)
008: {
009: Id = s; // This sentence calls the set builder of the Id attribute
010: }
011:
012: public string Id
013: {
014: get
015: {
016: return myId ;
017: }
018:
019: set
020: {
021: myId = value;
022: }
023: }
024:
025: public abstract double Area
026: {
027: get;
028: }
029:
030: public override string ToString()
031: {
032: return Id + " Area = " + double.Format(Area, "F");
033: }
034: }
Looking at this program, it is actually very simple. When the object of this class pair is created, the initialization part is 007-010. It gives the parameter s of the object to the Id attribute. Then the operation of the previous example is performed. In fact, we can compare abstract attributes with interfaces in JAVA. They only mention the name of a method without providing the content of this method. Just like the abstract attribute Area, there is a get, but it does not The content in the get method (maybe it cannot be called a method) is not specified, that is, what is required to be done by get. This task is done by its subclasses.
Second file: In this file, a class overrides Override the Area property.
000: // Propertiesshapes.cs
001: public class Square : Shape
002: {
003: private int mySide;
004:
005: public Square(int side, string id ) : base(id)
006: {
007: mySide = side;
008: }
009:
010: public override double Area
011: {
012: get
013: {
014: return mySide * mySide ;
015: }
016: }
017: }
018:
019: public class Circle : Shape
020: {
021: private int myRadius;
022:
023: public Circle(int radius, string id) : base(id)
024: {
025: myRadius = radius;
026: }
027:
028: public override double Area
029: {
030: get
031: {
032: return myRadius * myRadius * System.Math.PI;
033: }
034: }
035: }
036:
037: public class Rectangle : Shape
038: {
039: private int myWidth;
040: private int myHeight;
041:
042: public Rectangle(int width, int height, string id) : base(id)
043: {
044: myWidth = width;
045: myHeight = height;
046: }
047:
048: public override double Area
049: {
050: get
051: {
052: return myWidth * myHeight;
053: }
054: }
055: }
This example makes us a little confused, what does it do? , it seems to be inheritance, equivalent to extends in JAVA. I think so. Let’s take a look first.
The third file below is a test file. It’s very simple. Take a look.
000: // Propertiesshapetest.cs
001: public class TestClass
002: {
003: public static void Main()
004: {
005: Shape[] shapes =
006: {
007: new Square(5, "Square #1"),
008: new Circle(3, "Circle #1"),
009: new Rectangle(4, 5, "Rectangle #1")
010: };
011:
012: System .Console.WriteLine("Shapes Collection");
013: foreach(Shape s in shapes)
014: {
015: System.Console.WriteLine(s);
016: }
017:
018: }
019 : }
From this example, the : symbol does mean extends, which is inheritance. What does inheritance mean? To put it bluntly, it means having children. For example, in the following sentence sunwenson extends sunwen, the name means that the class sunwenson inherits In addition to sunwen, the sunwenson class has everything in the sunwen class, and at the same time, you can add and delete some things in sunwen. It is as simple as that, but this is an important technology in the development of modern software because it can greatly improve the reusability of software. .Alas, only juniors and seniors can say this, so I am not qualified. Haha.
The output of this program is:
Shapes Collection
Square #1 Area = 25.00
Circle #1 Area = 28.27
Rectangle #1 Area = 20.00
That’s it, this section is over again. It’s a bit difficult to understand this section, especially for those who have no experience in JAVA or C++ programming. But don’t be afraid, have the courage to learn it, and you will definitely learn it. I have learned something. I have to take a rest. Hey, I haven’t eaten breakfast yet!
The above is the content of SUNWEN tutorial - C# Advanced (4). For more related content, please pay attention to the PHP Chinese website ( www.php.cn)!
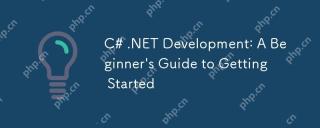
To start C#.NET development, you need to: 1. Understand the basic knowledge of C# and the core concepts of the .NET framework; 2. Master the basic concepts of variables, data types, control structures, functions and classes; 3. Learn advanced features of C#, such as LINQ and asynchronous programming; 4. Be familiar with debugging techniques and performance optimization methods for common errors. With these steps, you can gradually penetrate the world of C#.NET and write efficient applications.
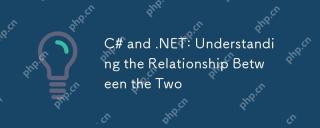
The relationship between C# and .NET is inseparable, but they are not the same thing. C# is a programming language, while .NET is a development platform. C# is used to write code, compile into .NET's intermediate language (IL), and executed by the .NET runtime (CLR).
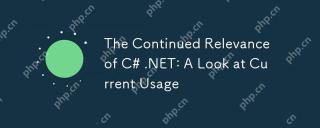
C#.NET is still important because it provides powerful tools and libraries that support multiple application development. 1) C# combines .NET framework to make development efficient and convenient. 2) C#'s type safety and garbage collection mechanism enhance its advantages. 3) .NET provides a cross-platform running environment and rich APIs, improving development flexibility.
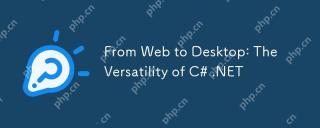
C#.NETisversatileforbothwebanddesktopdevelopment.1)Forweb,useASP.NETfordynamicapplications.2)Fordesktop,employWindowsFormsorWPFforrichinterfaces.3)UseXamarinforcross-platformdevelopment,enablingcodesharingacrossWindows,macOS,Linux,andmobiledevices.
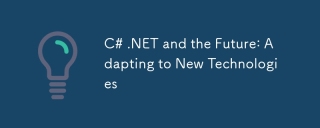
C# and .NET adapt to the needs of emerging technologies through continuous updates and optimizations. 1) C# 9.0 and .NET5 introduce record type and performance optimization. 2) .NETCore enhances cloud native and containerized support. 3) ASP.NETCore integrates with modern web technologies. 4) ML.NET supports machine learning and artificial intelligence. 5) Asynchronous programming and best practices improve performance.
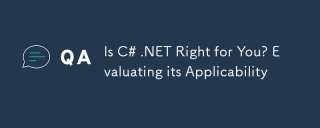
C#.NETissuitableforenterprise-levelapplicationswithintheMicrosoftecosystemduetoitsstrongtyping,richlibraries,androbustperformance.However,itmaynotbeidealforcross-platformdevelopmentorwhenrawspeediscritical,wherelanguageslikeRustorGomightbepreferable.
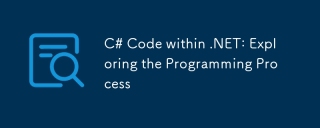
The programming process of C# in .NET includes the following steps: 1) writing C# code, 2) compiling into an intermediate language (IL), and 3) executing by the .NET runtime (CLR). The advantages of C# in .NET are its modern syntax, powerful type system and tight integration with the .NET framework, suitable for various development scenarios from desktop applications to web services.
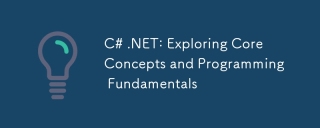
C# is a modern, object-oriented programming language developed by Microsoft and as part of the .NET framework. 1.C# supports object-oriented programming (OOP), including encapsulation, inheritance and polymorphism. 2. Asynchronous programming in C# is implemented through async and await keywords to improve application responsiveness. 3. Use LINQ to process data collections concisely. 4. Common errors include null reference exceptions and index out-of-range exceptions. Debugging skills include using a debugger and exception handling. 5. Performance optimization includes using StringBuilder and avoiding unnecessary packing and unboxing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
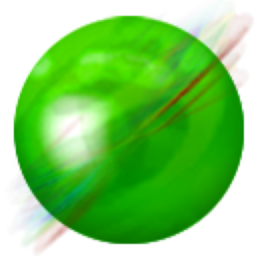
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
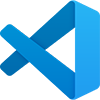
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.