Foreword
I believe everyone is used to common array-related operations in jquery or underscore and other libraries, such as $.isArray, _.some, _.find and other methods. This is nothing more than some additional packaging for array operations in native js.
Here we mainly summarize common APIs for JavaScript array operations. I believe it will be helpful for everyone to solve program problems.
1. Properties
An array in JavaScript is a special object. The index used to represent the offset is a property of the object, and the index may be an integer. However, these numeric indices are converted to string types internally because property names in JavaScript objects must be strings.
2. Operations
1 Determine the array type
var array0 = []; // 字面量 var array1 = new Array(); // 构造器 // 注意:在IE6/7/8下是不支持Array.isArray方法的 alert(Array.isArray(array0)); // 考虑兼容性,可使用 alert(array1 instanceof Array); // 或者 alert(Object.prototype.toString.call(array1) === '[object Array]');
2 Arrays and strings
are very simple: to convert from an array to a string, use join; to convert from a string to an array, use split.
// join - 由数组转换为字符串,使用join console.log(['Hello', 'World'].join(',')); // Hello,World // split - 由字符串转换为数组,使用split console.log('Hello World'.split(' ')); // ["Hello", "World"]
3 Find elements
I believe everyone commonly uses the string type indexOf, but few know that the indexOf of an array can also be used to find elements.
// indexOf - 查找元素 console.log(['abc', 'bcd', 'cde'].indexOf('bcd')); // 1 // var objInArray = [ { name: 'king', pass: '123' }, { name: 'king1', pass: '234' } ]; console.log(objInArray.indexOf({ name: 'king', pass: '123' })); // -1 var elementOfArray = objInArray[0]; console.log(objInArray.indexOf(elementOfArray)); // 0
As can be seen from the above, for arrays containing objects, the indexOf method does not obtain the corresponding search results through in-depth comparison, but only compares the references of the corresponding elements.
4 Array connection
Use concat. Please note that a new array will be generated after using concat.
var array1 = [1, 2, 3]; var array2 = [4, 5, 6]; var array3 = array1.concat(array2); // 实现数组连接之后,会创建出新的数组 console.log(array3);
5 List-like operations
are used to add elements, push and unshift can be used respectively, and pop and shift can be used to remove elements.
// push/pop/shift/unshift var array = [2, 3, 4, 5]; // 添加到数组尾部 array.push(6); console.log(array); // [2, 3, 4, 5, 6] // 添加到数组头部 array.unshift(1); console.log(array); // [1, 2, 3, 4, 5, 6] // 移除最后一个元素 var elementOfPop = array.pop(); console.log(elementOfPop); // 6 console.log(array); // [1, 2, 3, 4, 5] // 移除第一个元素 var elementOfShift = array.shift(); console.log(elementOfShift); // 1 console.log(array); // [2, 3, 4, 5]
6 The splice method
has two main uses:
① Add and delete elements from the middle of the array
② Obtain a new array from the original array
Of course, the two uses are combined in one go, in some scenarios Focus on purpose one, and some focus on purpose two.
Add and delete elements from the middle of the array. The splice method adds elements to the array. You need to provide the following parameters
① Starting index (that is, where you want to start adding elements)
②
The number of elements to be deleted or the number of elements to be extracted (this parameter is set to 0 when adding elements)
③ The elements you want to add to the array
var nums = [1, 2, 3, 7, 8, 9]; nums.splice(3, 0, 4, 5, 6); console.log(nums); // [1, 2, 3, 4, 5, 6, 7, 8, 9] // 紧接着做删除操作或者提取新的数组 var newnums = nums.splice(3, 4); console.log(nums); // [1, 2, 3, 8, 9] console.log(newnums); // [4, 5, 6, 7]
7 Sorting
Mainly introduces the two methods of reverse and sort . Array reversal uses reverse, and the sort method can be used not only for simple sorting, but also for complex sorting.
// 反转数组 var array = [1, 2, 3, 4, 5]; array.reverse(); console.log(array); // [5, 4, 3, 2, 1] 我们先对字符串元素的数组进行排序 var arrayOfNames = ["David", "Mike", "Cynthia", "Clayton", "Bryan", "Raymond"]; arrayOfNames.sort(); console.log(arrayOfNames); // ["Bryan", "Clayton", "Cynthia", "David", "Mike", "Raymond"]
We sort an array of numeric elements
// 如果数组元素时数字类型,sort()方法的排序结果就不能让人满意了 var nums = [3, 1, 2, 100, 4, 200]; nums.sort(); console.log(nums); // [1, 100, 2, 200, 3, 4]
The sort method sorts the elements in lexicographic order, so it assumes that the elements are all of string type, so even if the elements are of numeric type, they are considered characters String type. At this time, you can pass in a size comparison function when calling the method. When sorting, the sort() method will compare the sizes of the two elements in the array based on this function to determine the order of the entire array.
var compare = function(num1, num2) { return num1 > num2; }; nums.sort(compare); console.log(nums); // [1, 2, 3, 4, 100, 200] var objInArray = [ { name: 'king', pass: '123', index: 2 }, { name: 'king1', pass: '234', index: 1 } ]; // 对数组中的对象元素,根据index进行升序 var compare = function(o1, o2) { return o1.index > o2.index; }; objInArray.sort(compare); console.log(objInArray[0].index < objInArray[1].index); // true
8 The iterator method
mainly includes forEach and every, some and map, and filter
forEach. I believe everyone knows it. Let’s mainly introduce the other four methods.
Every method accepts a function with a return value of Boolean type and uses this function for each element in the array. This method returns true if the function returns true for all elements.
var nums = [2, 4, 6, 8]; // 不生成新数组的迭代器方法 var isEven = function(num) { return num % 2 === 0; }; // 如果都是偶数,才返回true console.log(nums.every(isEven)); // true some方法也接受一个返回值为布尔类型的函数,只要有一个元素使得该函数返回true,该方法就返回true。 var isEven = function(num) { return num % 2 === 0; }; var nums1 = [1, 2, 3, 4]; console.log(nums1.some(isEven)); // true
Both methods map and filter can generate new arrays. The new array returned by map is the result of applying a certain function to the original elements. For example:
var up = function(grade) { return grade += 5; } var grades = [72, 65, 81, 92, 85]; var newGrades = grades.ma
The filter method is very similar to the every method, passing in a function whose return value is a Boolean type. Different from the every() method, when the function is applied to all elements in the array and the result is true, this method does not return true, but returns a new array containing the result of applying the function. Elements.
var isEven = function(num) { return num % 2 === 0; }; var isOdd = function(num) { return num % 2 !== 0; }; var nums = []; for (var i = 0; i < 20; i++) { nums[i] = i + 1; } var evens = nums.filter(isEven); console.log(evens); // [2, 4, 6, 8, 10, 12, 14, 16, 18, 20] var odds = nums.filter(isOdd); console.log(odds); // [1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
3. Summary
There are still some problems in the above methods that are not supported by low-level browsers, and other methods need to be used for compatible implementation.
These are common methods that may not be easy for everyone to think of. You may wish to pay more attention to it.
I hope this article will be helpful to everyone’s JavaScript programming design.
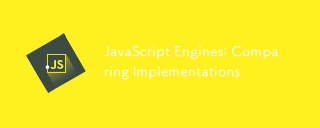
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
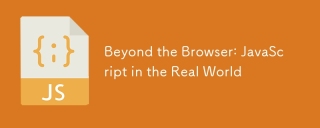
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
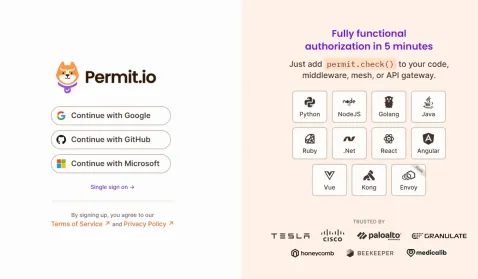
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
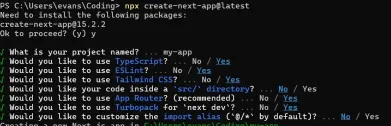
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
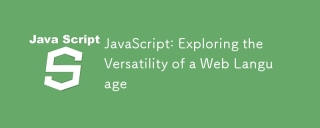
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
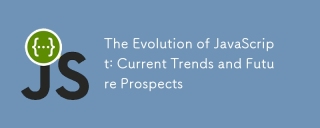
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
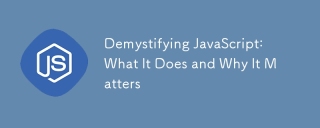
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
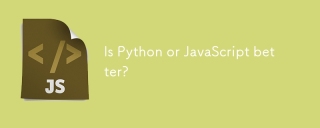
Python is more suitable for data science and machine learning, while JavaScript is more suitable for front-end and full-stack development. 1. Python is known for its concise syntax and rich library ecosystem, and is suitable for data analysis and web development. 2. JavaScript is the core of front-end development. Node.js supports server-side programming and is suitable for full-stack development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
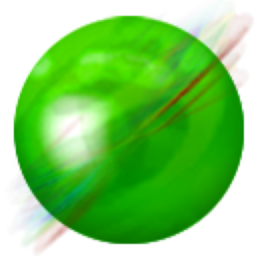
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
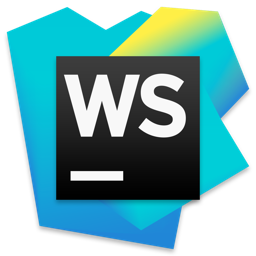
WebStorm Mac version
Useful JavaScript development tools
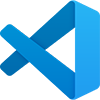
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft