Error levels of PHP
First of all, you need to understand what errors there are in php. As of php5.5, there are a total of 16 error levels
Note: When trying the following code, please make sure to open the error_log:
error_reporting(E_ALL); ini_set('display_errors', 'On');
E_ERROR
This kind of error is a fatal error and will display Fatal Error on the page. When this kind of error occurs, the program cannot continue to execute. Error example:
// Fatal error: Call to undefined function hpinfo() in /tmp/php/index.php on line 5 hpinfo(); //E_ERRORNote that if there is an uncaught exception, this level will also be triggered.
// Fatal error: Uncaught exception 'Exception' with message 'test exception' in /tmp/php/index.php:5 Stack trace: #0 {main} thrown in /tmp/php/index.php on line 5 throw new \Exception("test exception");This error is just a warning, it will not terminate the script, the program will continue, and the error message displayed is Warning. For example, include a file that does not exist.
//Warning: include(a.php): failed to open stream: No such file or directory in /tmp/php/index.php on line 7 //Warning: include(): Failed opening 'a.php' for inclusion (include_path='.:/usr/share/pear:/usr/share/php') in /tmp/php/index.php on line 7 include("a.php"); //E_WARNING
E_NOTICE
This kind of error is more minor, reminding you that this place should not be written like this. This is also a runtime error. The wrong code may not have problems elsewhere, but only in the current context.
For example, the $b variable does not exist and we assign it to another variable//Notice: Undefined variable: b in /tmp/php/index.php on line 9 $a = $b; //E_NOTICE
E_PARSE
This error occurs during compilation. A syntax error is found during compilation and syntax analysis cannot be performed.
For example, z below is not set as a variable.// Parse error: syntax error, unexpected '=' in /tmp/php/index.php on line 20 z=1; // E_PARSE
E_STRICT
This error was introduced after PHP5. Your code can run, but it is not written in the way recommended by PHP.
For example, passing the ++ symbol in the function parameter// Strict Standards: Only variables should be passed by reference in /tmp/php/index.php on line 17 function change (&$var) { $var += 10; } $var = 1; change(++$var); // E_STRICT
E_RECOVERABLE_ERROR
This level is actually the ERROR level, but it is expected to be captured. If it is not captured by error processing, the performance is the same as E_ERROR.
It often occurs when the formal parameter defines a type, but the wrong type is passed in when calling. Its error reminder also has the word Catachable in front of E_ERROR's fatal error.//Catchable fatal error: Argument 1 passed to testCall() must be an instance of A, instance of B given, called in /tmp/php/index.php on line 37 and defined in /tmp/php/index.php on line 33 class A { } class B { } function testCall(A $a) { } $b = new B(); testCall($b);
E_DEPRECATED
This error means that you are using an old version of the function, and later versions of this function may be disabled or not maintained.
For example, curl's CURLOPT_POSTFIELDS uses @FILENAME to upload files// Deprecated: curl_setopt(): The usage of the @filename API for file uploading is deprecated. Please use the CURLFile class instead in /tmp/php/index.php on line 42 $ch = curl_init("http://www.php.cn/upload.php"); curl_setopt($ch, CURLOPT_POSTFIELDS, array('fileupload' => '@'. "test"));
E_CORE_ERROR, E_CORE_WARNING
These two errors are generated by the PHP engine and occur during the PHP initialization process.
E_COMPILE_ERROR, E_COMPILE_WARNING
These two errors are generated by the PHP engine and occur during the compilation process.
E_USER_ERROR, E_USER_WARNING, E_USER_NOTICE, E_USER_DEPRECATED,
These errors are all caused by users. Using trigger_error is equivalent to a hole that triggers various error types for users. This is a good way to escape try catch exceptions.
trigger_error("Cannot divide by zero", E_USER_ERROR); // E_USER_ERROR // E_USER_WARING // E_USER_NOTICE // E_USER_DEPRECATED
E_ALL
E_STRICT all error and warning messages out.
Error control
There are many configurations and parameters in php that can control errors and error log display. The first step, what we need to know is what are the incorrect configurations in PHP?
We follow the php+php-fpm model. There are actually two configuration files that will affect the display of php errors. One is the configuration file php.ini of php itself, and the other is the configuration file of php-fpm. php -fpm.conf.Configuration in php.ini
error_reporting = E_ALL // 报告错误级别,什么级别的 error_log = /tmp/php_errors.log // php中的错误显示的日志位置 display_errors = On // 是否把错误展示在输出上,这个输出可能是页面,也可能是stdout display_startup_errors = On // 是否把启动过程的错误信息显示在页面上,记得上面说的有几个Core类型的错误是启动时候发生的,这个就是控制这些错误是否显示页面的。 log_errors = On // 是否要记录错误日志 log_errors_max_len = 1024 // 错误日志的最大长度 ignore_repeated_errors = Off // 是否忽略重复的错误 track_errors = Off // 是否使用全局变量$php_errormsg来记录最后一个错误 xmlrpc_errors = 0 //是否使用XML-RPC的错误信息格式记录错误 xmlrpc_error_number = 0 // 用作 XML-RPC faultCode 元素的值。 html_errors = On // 是否把输出中的函数等信息变为HTML链接 docref_root = http://manual/en/ // 如果html_errors开启了,这个链接的根路径是什么 fastcgi.logging = 0 // 是否把php错误抛出到fastcgi中We are often asked, what is the difference between error_reporting and display_errors? These two functions are completely different. PHP will log and standard output by default (if it is fpm mode, the standard output is the page) The parameter of error_reporting is the error level. Indicates what level should trigger an error. If we tell PHP that all error levels do not need to trigger errors, then neither the log nor the page will display this error, which is equivalent to nothing happening. display_errors controls whether error messages should be displayed on the standard output. log_errors controls whether error messages should be recorded in the log. error_log is the location where the error log is displayed. This is often rewritten in php-fpm, so it is often found that the error logs of cli and fpm are not in the same file. ignore_repeated_errors This flag controls that if there are duplicate logs, then only one will be recorded, such as the following program:
error_reporting(E_ALL); ini_set('ignore_repeated_errors', 1); ini_set('ignore_repeated_source', 1); $a = $c; $a = $c; //E_NOTICE //Notice: Undefined variable: c in /tmp/php/index.php on line 20NOTICE would have appeared twice, but now, it will only appear once...track_errors is turned on The last error information will be stored in the variable. This may be of some use when recording logs. But I think it is really useless... html_errors and docref_root are two very user-friendly configurations. After configuring these two parameters, if the error message we return contains some information in the document, it will change into link form.
error_reporting(E_ALL); ini_set('html_errors', 1); ini_set('docref_root', "https://secure.php.net/manual/zh/"); include("a2.php"); //E_WARNINGallows you to quickly locate where we made errors. Isn’t it very human~
The configuration in php-fpm
error_log = /var/log/php-fpm/error.log // php-fpm自身的日志 log_level = notice // php-fpm自身的日志记录级别 php_flag[display_errors] = off // 覆盖php.ini中的某个配置变量,可被程序中的ini_set覆盖 php_value[display_errors] = off // 同php_flag php_admin_value[error_log] = /tmp/www-error.log // 覆盖php.ini中的某个配置变量,不可被程序中的ini_set覆盖 php_admin_flag[log_errors] = on // 同php_admin_value catch_workers_output = yes // 是否抓取fpmworker的输出 request_slowlog_timeout = 0 // 慢日志时长 slowlog = /var/log/php-fpm/www-slow.log // 慢日志记录php-fpm also has an error_log configuration, which is often confused with the error_log configuration in php.ini. But what they record is different. The error_log of php-fpm only records the logs of php-fpm itself, such as fpm startup and shutdown. The error_log in php.ini is the error log that records the php program itself.
So to override the error_log configuration in php.ini in php-fpm, you need to use the following functions:
php_flag
php_value
php_admin_flag
php_admin_value
The two functions of these four admin functions indicate that after the variable is set, the variable cannot be reassigned using ini_set in the code. The php_flag/value is still based on the ini_set in the php code.
Slowlog is recorded by fpm. You can use the request_slowlog_timeout setting to determine the length of the slow log.
Summary
What we often confuse is the log issue, and why certain levels of logs are not recorded in the log. The most important thing is to look at the three configurations of error_log, display_errors, and log_errors. But when looking at the configuration, we also need to pay attention to distinguishing what is the configuration in php.ini and what is the configuration in php-fpm.ini.
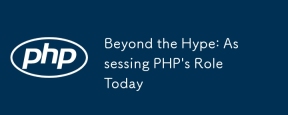
PHP remains a powerful and widely used tool in modern programming, especially in the field of web development. 1) PHP is easy to use and seamlessly integrated with databases, and is the first choice for many developers. 2) It supports dynamic content generation and object-oriented programming, suitable for quickly creating and maintaining websites. 3) PHP's performance can be improved by caching and optimizing database queries, and its extensive community and rich ecosystem make it still important in today's technology stack.
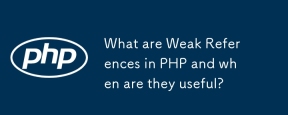
In PHP, weak references are implemented through the WeakReference class and will not prevent the garbage collector from reclaiming objects. Weak references are suitable for scenarios such as caching systems and event listeners. It should be noted that it cannot guarantee the survival of objects and that garbage collection may be delayed.
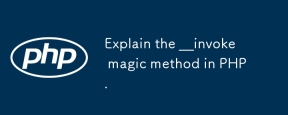
The \_\_invoke method allows objects to be called like functions. 1. Define the \_\_invoke method so that the object can be called. 2. When using the $obj(...) syntax, PHP will execute the \_\_invoke method. 3. Suitable for scenarios such as logging and calculator, improving code flexibility and readability.
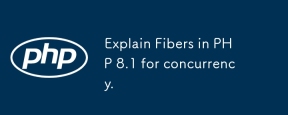
Fibers was introduced in PHP8.1, improving concurrent processing capabilities. 1) Fibers is a lightweight concurrency model similar to coroutines. 2) They allow developers to manually control the execution flow of tasks and are suitable for handling I/O-intensive tasks. 3) Using Fibers can write more efficient and responsive code.
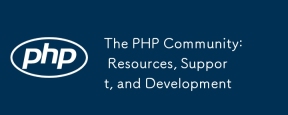
The PHP community provides rich resources and support to help developers grow. 1) Resources include official documentation, tutorials, blogs and open source projects such as Laravel and Symfony. 2) Support can be obtained through StackOverflow, Reddit and Slack channels. 3) Development trends can be learned by following RFC. 4) Integration into the community can be achieved through active participation, contribution to code and learning sharing.
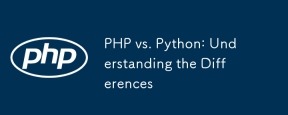
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
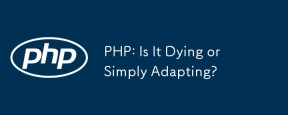
PHP is not dying, but constantly adapting and evolving. 1) PHP has undergone multiple version iterations since 1994 to adapt to new technology trends. 2) It is currently widely used in e-commerce, content management systems and other fields. 3) PHP8 introduces JIT compiler and other functions to improve performance and modernization. 4) Use OPcache and follow PSR-12 standards to optimize performance and code quality.
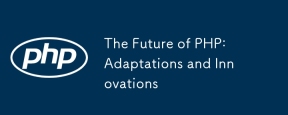
The future of PHP will be achieved by adapting to new technology trends and introducing innovative features: 1) Adapting to cloud computing, containerization and microservice architectures, supporting Docker and Kubernetes; 2) introducing JIT compilers and enumeration types to improve performance and data processing efficiency; 3) Continuously optimize performance and promote best practices.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
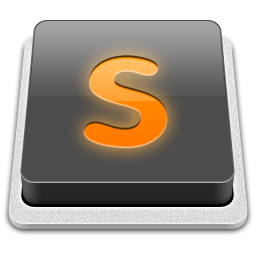
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
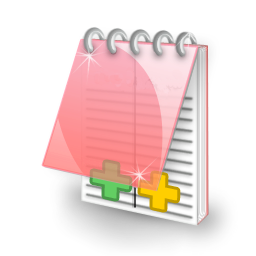
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function