Introduction to Vue.js
vue means view in French. Vue.js is a lightweight, high-performance, componentable MVVM library, and has a very easy-to-use API.
1. Dynamic parameter display
After asynchronous ajax request, the returned data parameters are received and displayed on the front end
1.1 introduced js and also added jQuery
<script type="text/javascript" src="/js/vue.min.js"></script> <script type="text/javascript" src="/js/jquery-2.1.3.js"></script>
1.2 html
<div id="app"> <p>{{ message }}</p> <button v-on:click="showData">显示数据</button> </div>
1.3 JS
Note: The JS here must be placed inside $(function() {}), or written into the body
new Vue({ el: '#app', data: { message: '' }, methods: { showData: function () { var _self = this; $.ajax({ type: 'GET', url: '...', success:function(data) { _self.message = JSON.stringify(data); } }); } } })
2. Dynamic list display
Start to display a blank list, ajax asynchronous After the request, the returned data list information is received and displayed
2.1 introduced js, also added jquery
<script type="text/javascript" src="/js/vue.min.js"></script> <script type="text/javascript" src="/js/jquery-2.1.3.js"></script>
2.2 html
<div id="app"> <table> <thead> <tr> <th style='width:3%; text-align: left'>ID</th> <th style='width:5%; text-align: left'>名称</th> <th style='width:10%; text-align: left'>条形码</th> <th style='width:10%; text-align: left'>简称</th> </tr> </thead> <tbody> <tr v-for="goods in goodsList"> <td>{{goods.id}}</td> <td>{{goods.name}}</td> <td>{{goods.barcode}}</td> <td>{{goods.shortName}}</td> </tr> </tbody> </table> <button v-on:click="nameSearch()">查询</button><br><br> </div>
2.3 JS
var goodsVue = new Vue({ el: '#app', data: { goodsList : '' }, methods: { nameSearch: function () { var _self = this; $.ajax({ type: 'GET', url: '...', success:function(data) { _self.goodsList = data; } }); } } })

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
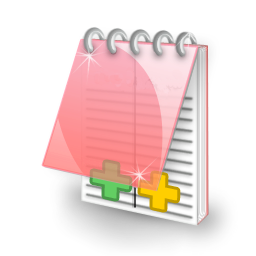
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
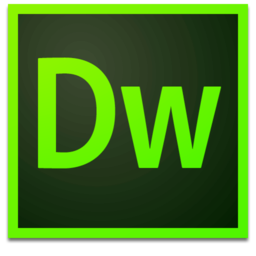
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor
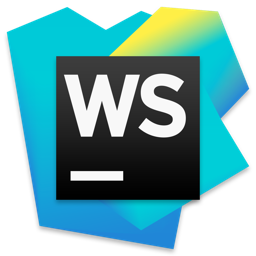
WebStorm Mac version
Useful JavaScript development tools
