"""" There are two ways to use threads in Python: functions or classes to wrap thread objects.
1. Functional: Call the start_new_thread() function in the thread module to generate a new thread. The thread can end by waiting for the thread to end naturally, or by calling the thread.exit() or thread.exit_thread() method in the thread function.
import time
import thread
def timer(no,interval):
cnt=0
while cnt print 'thread(%d)'%(cnt)
time.sleep(interval)
cnt= cnt+1
def test():
thread.start_new_thread(timer,(1,1))
thread.start_new_thread(timer,(2,2))
if __name__=='__main__':
print ' thread starting'
test()
time.sleep(20)
thread.exit_thread()
print 'exit...'
2. Create a subclass of threading.Thread to wrap a thread object,
threading.Thread class Usage:
1, call threading.Thread.__init__(self, name = threadname) in the __init__ of your own thread class
Threadname is the name of the thread
2, run(), usually needs to be rewritten and written The code implements the required functionality.
3, getName(), get the thread object name
4, setName(), set the thread object name
5, start(), start the thread
6, jion([timeout]), wait for another thread to end and then run again.
7, setDaemon(bool), sets whether the sub-thread ends with the main thread, and must be called before start(). Default is False.
8, isDaemon(), determines whether the thread ends with the main thread.
9, isAlive(), check whether the thread is running.
import threading
import time
class timer(threading.Thread):
def __init__(self,num,interval):
threading.Thread.__init__(self)
self.thread_num=num
self.interval=interval
self .thread_stop=False
def run(self):
while not self.thread_stop:
print 'thread object (%d), time %sn' %(self.thread_num,time.ctime())
time.sleep( self.interval)
def stop(self):
self.thread_stop=True
def test():
thread1=timer(1,1)
thread2=timer(2,2)
thread1.start()
thread2.start()
time.sleep(10)
thread1.stop()
thread2.stop()
return
if __name__=='__main__':
test()
"""
"""
Question The reason is that the access of multiple threads to the same resource is not controlled, causing data damage and making the results of thread operation unpredictable. This phenomenon is called "thread unsafe".
import threading
import time
class MyThread(threading.Thread):
def run(self):
for i in range(3):
time.sleep(1)
msg='I am '+ self.getName ()+'@'+str(i)
print msg
def test():
for i in range(5):
t=MyThread()
t.start()
if __name__=='__main__ ':
test()
The above example leads to the most common problem of multi-threaded programming: data sharing. When multiple threads modify a certain shared data, synchronization control is required.
Thread synchronization can ensure that multiple threads safely access competing resources. The simplest synchronization mechanism is to introduce a mutex lock. A mutex introduces a state to a resource: locked/unlocked. When a thread wants to change shared data, it must first lock it. At this time, the status of the resource is "locked" and other threads cannot change it; until the thread releases the resource and changes the status of the resource to "unlocked", other threads can Lock the resource again. The mutex lock ensures that only one thread performs writing operations at a time, thereby ensuring the correctness of data in multi-threaded situations. Among them, the lock method acquire can have an optional parameter timeout for the timeout period. If timeout is set, the return value can be used to determine whether the lock has been obtained after the timeout, so that some other processing can be performed
The Lock class is defined in the threading module
import threading
import time
class MyThread(threading.Thread ):
def run(self):
global num
time.sleep(1)
if mutex.acquire(): num=num+1
print self.name+' set num to '+str(num)+'n'
mutex.release()
num=0
mutex=threading.Lock()
def test():
for i in range(5):
t=MyThread()
t.start()
if __name__=='__main__':
test()
"""
"""A simpler deadlock situation is when a thread "iteratively" requests the same resource, which will directly cause a deadlock:
import threading
import time
class MyThread(threading.Thread) :
def run(self):
using using ’ using ’s ’ s ’ using ’s ’ using ’ s ‐ ‐ ‐ ‐ name+' set num to '+str(num) G Print MSG in Mutex.acquire ()
mutex.release ()
mutex.release ()
num = 0
mutex = Threading.lock ()
for I in Range (5):
T = MyThread()
t.start()
if __name__ == '__main__':
test()
In order to support multiple requests for the same resource in the same thread, python provides a "reentrant lock": threading.RLock. RLock maintains a Lock and a counter variable internally. The counter records the number of acquires, so that the resource can be required multiple times. Until all acquires of a thread are released, other threads can obtain resources. In the above example, if RLock is used instead of Lock, no deadlock will occur:
import threading
import time
class MyThread(threading.Thread):
def run(self):
global num
time.sleep(1)
if mutex. re()
use using use using use using using using through through ‐ ‐ off ‐ ‐ ‐ ‐ ‐ ‐ ()
num = 0
mutex = threading.RLock()
def test():
for i in range(5):
t = MyThread()
t.start()
if __name__ == '__main__':
Test()
"""
"""
Python multi-threaded programming (5): Condition variable synchronization
Mutex lock is the simplest thread synchronization mechanism. The Condition object provided by Python provides a solution to complex thread synchronization issues support. Condition is called a condition variable. In addition to providing acquire and release methods similar to Lock, it also provides wait and notify methods. The thread first acquires a condition variable and then determines some conditions. If the condition is not met, wait; if the condition is met, perform some processing to change the condition, and notify other threads through the notify method. Other threads in the wait state will re-judge the condition after receiving the notification. This process is repeated continuously to solve complex synchronization problems.
It can be thought that the Condition object maintains a lock (Lock/RLock) and a waiting pool. The thread obtains the Condition object through acquire. When the wait method is called, the thread releases the lock inside the Condition and enters the blocked state. At the same time, the thread is recorded in the waiting pool. When the notify method is called, the Condition object will select a thread from the waiting pool and notify it to call the acquire method to try to acquire the lock.
The constructor of the Condition object can accept a Lock/RLock object as a parameter. If not specified, the Condition object will create an RLock internally.
In addition to the notify method, the Condition object also provides the notifyAll method, which can notify all threads in the waiting pool to try to acquire the internal lock. Due to the above mechanism, threads in the waiting state can only be awakened through the notify method, so the function of notifyAll is to prevent threads from being in a silent state forever.
The classic problem that demonstrates condition variable synchronization is the producer and consumer problem: Suppose there is a group of producers (Producer) and a group of consumers (Consumer) interacting with products through a market. The producer's "strategy" is to produce 100 products and put them on the market if the remaining products on the market are less than 1,000; while the consumer's "strategy" is to produce more than 100 products on the market. Consume 3 products. The code to use Condition to solve the problem of producers and consumers is as follows:
import threading
import time
class Producer(threading.Thread):
def run(self):
global count
while True:
if con.acquire() :
if count & gt; 1000:
con.wait ()
else:
Count = Count+100
Print Self.name+'Produce 100, Count ='+Str (Count)
TIME. Sleep (1)
class Customer(threading.Thread):
def run(self):
global count
while True:
if con.acquire():
if count>100:
count=count-100
print self .name+ 'consume 100, count='+str(count)
else:
con.wait()
con.release() time.sleep(1)
count=500
con=threading.Condition()
def test():
for i in range(5):
p=Producer()
p.start()
c=Customer()
c.start()
print i
if __name__==' __main__':
test()
The default global variables in python can be read in the function, but cannot be written. However,
are only readable for con, so there is no need to introduce global """
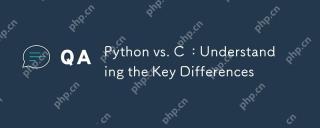
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
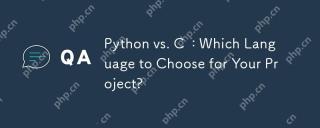
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
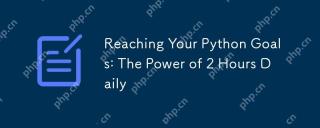
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
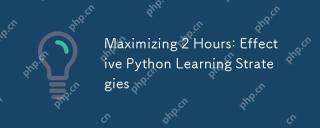
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
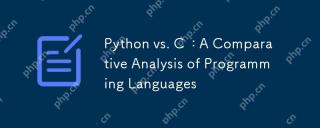
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
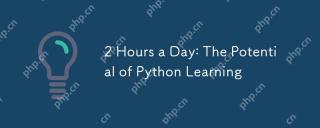
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
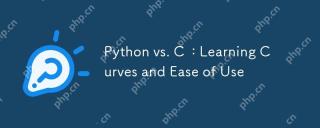
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
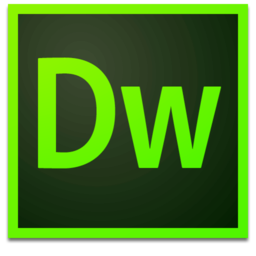
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
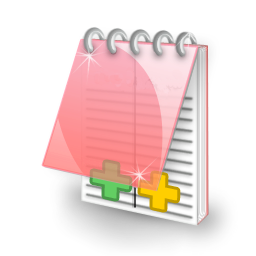
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function