1. Write a simple Angular App
Before starting to write the test, we first write a simple calculation App, which will calculate the sum of two numbers.
The code is as follows:
<html> <head> <script type="text/javascript" src="https://code.angularjs.org/1.4.0-rc.2/angular.min.js"></script> </head> <body> <!-- This div element corresponds to the CalculatorController we created via the JavaScript--> <div ng-controller="CalculatorController"> <input ng-model="x" type="number"> <input ng-model="y" type="number"> <strong>{{z}}</strong> <!-- the value for ngClick maps to the sum function within the controller body --> <input type="button" ng-click="sum()" value="+"> </div> </body> <script type="text/javascript"> // Creates a new module called 'calculatorApp' angular.module('calculatorApp', []); // Registers a controller to our module 'calculatorApp'. angular.module('calculatorApp').controller('CalculatorController', function CalculatorController($scope) { $scope.z = 0; $scope.sum = function() { $scope.z = $scope.x + $scope.y; }; }); // load the app angular.element(document).ready(function() { angular.bootstrap(document, ['calculatorApp']); }); </script> </html>
Second, briefly talk about some basic concepts involved:
Create a module
What is angular.module? It is a place for creating and recycling modules. We create a new module called calculatorApp and we add the component to this module.
angular.module('calculatorApp', []);
About the second parameter? The second parameter is required and indicates that we are creating a new module. If our application needs other dependencies, we can pass them ['ngResource', 'ngCookies']. The presence of the second parameter indicates that this is an instance of the module returned by the request.
Conceptually, it is meant to mean something like the following:
* angular.module.createInstance(name, requires); * angular.module.getInstance(name)
However, actually we write it like this:
* angular.module('calculatorApp', []); // i.e. createInstance * angular.module('calculatorApp'); // i.e. getInstance
More information about module https://docs.angularjs .org/api/ng/function/angular.module
2. Add a controller to the module
Then we add a controller to the angular module example
angular.module('calculatorApp').controller('CalculatorController', function CalculatorController($scope) { $scope.z = 0; $scope.sum = function() { $scope.z = $scope.x + $scope.y; }; });
The controller is mainly responsible for business logic and view binding , $scope is the direct messenger to the view's controller.
3. Connect elements in the view
In the HTML below, we need to calculate the value in the input, and these are contained in the div of this controller.
<div ng-controller="CalculatorController"> <input ng-model="x" type="number"> <input ng-model="y" type="number"> <strong>{{z}}</strong> <!-- the value for ngClick maps to the sum function within the controller body --> <input type="button" ng-click="sum()" value="+"> </div>
Input The value bound to ng-model is the same as that defined on $scope, such as $scope.x. We also bound the $scope.sum method to the button element using ng-click.
3. Add tests
Next we finally get to our topic, add some unit tests to the controller. We ignore the html part of the code and focus mainly on the controller code.
angular.module('calculatorApp').controller('CalculatorController', function CalculatorController($scope) { $scope.z = 0; $scope.sum = function() { $scope.z = $scope.x + $scope.y; }; });
In order to test the controller, we need to mention the following points? + How to create a controller instance + How to get/set the properties of an object + How to call functions in $scope
describe('calculator', function () { beforeEach(angular.mock.module('calculatorApp')); var $controller; beforeEach(angular.mock.inject(function(_$controller_){ $controller = _$controller_; })); describe('sum', function () { it('1 + 1 should equal 2', function () { var $scope = {}; var controller = $controller('CalculatorController', { $scope: $scope }); $scope.x = 1; $scope.y = 2; $scope.sum(); expect($scope.z).toBe(3); }); }); });
Before we start, we need to introduce ngMock. We add angular.mock
, ngMock to the test code Modules provide a mechanism for unit testing of virtual services.
4. How to get the controller instance
Using ngMock we can register a calculator app instance.
beforeEach(angular.mock.module('calculatorApp'));
Once the calculatorApp is initialized, we can use the inject function, which can solve the controller reference problem.
beforeEach(angular.mock.inject(function(_$controller_) { $controller = _$controller_; }));
Once the app is loaded, we use the inject function, and the $controller service can obtain an instance of CalculatorController.
var controller = $controller('CalculatorController', { $scope: $scope });
5. How to get/set the attributes of an object
In the previous code, we can already get an instance of a controller. The second parameter in the brackets is actually the controller itself. Our controller only has A parameter $scope object
function CalculatorController($scope) { ... }
In our test $scope represents a simple JavaScript object.
var $scope = {}; var controller = $controller('CalculatorController', { $scope: $scope }); // set some properties on the scope object $scope.x = 1; $scope.y = 2;
We set the values of x and y to simulate what is shown in the gif just now. We agree that we can also read the properties in the object, just like the assertion in the following test:
expect($scope.z).toBe(3);
6. How to call the functions in $scope
The last thing is how we simulate the user’s click , just like what we use in most JS, it is actually just a simple call to the function,
$scope.sum();
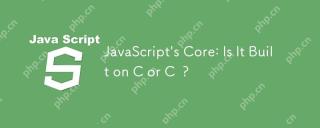
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
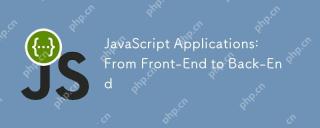
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
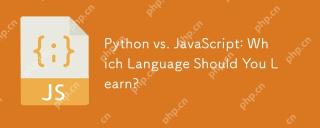
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
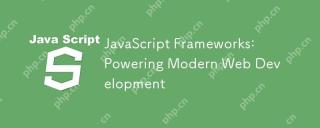
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
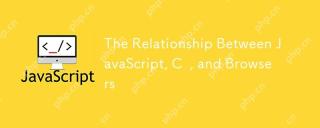
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
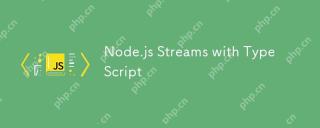
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
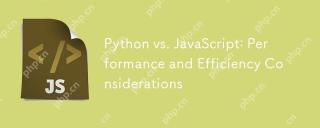
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
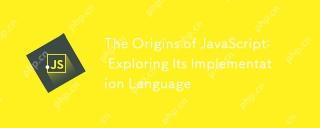
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
