


jquery cooperates with .NET to realize click-to-specify binding data and can download it with one click
You need to click on the bound data to download the specified attachments and download them in batches (the bound data is not datagrid, background splicing binding).
The rendering is as follows:
The general idea:
1.jquery gets the id of the selected bound data, assigns this id to an array, and finally assigns the value of this array to the hidden object created on the page Variable
2. Get the value of the hidden variable in the background, loop it through the array, get the download address of the bound value, and finally package and download it
Firstly, the div in the html is bound according to the background
<div id="downloadInfo" runat="server"></div>
Secondly It is the option to download attachments. It is implemented using jquery and assigns the value to the hidden variable in the page. The code is as follows:
// 下载附件的选择 $attach = $("#download-list"); var arr = [] $attach.on('click', '.no', function () { $(this).toggleClass('checked');//设置和移除,选中与不选中 if ($(this).hasClass('checked')) { var guid = $(this).children("#hidAttachGuid").val(); arr.push(guid);//将guid添加到arr数组中 } else {//取消选中时 var guid = $(this).children("#hidAttachGuid").val(); var n = arr.indexOf(guid); if (n != -1) arr.splice(n, 1);//将指定不选中的guid移除arr数组 } $("[id$='arrayGuid']").val(arr); });
Because it is spliced in the background, the button is also spliced in the background, and the background button calls js
<button type='button' class='one-download' onclick='download()'>一键下载</button> function download() { $("#btnDownload").click(); }
js triggers hidden button event
<span style="display: none"> <asp:Button ID="btnDownload" OnClick="btnDownload_Click" Text="确定" runat="server" /> <input type="text" id="arrayGuid" runat="server" /> </span>
Backend one-click package download code:
protected void btnDownload_Click(object sender, EventArgs e) { //ZipFileByCode(); string attachGuid = arrayGuid.Value; string[] sArray = attachGuid.Split(','); List<string> list = new List<string>(); foreach (string i in sArray) { //这里是循环得到指定需要下载的所有id } Download(list, ""+lblCourseName.Text+"相关附件材料.rar"); }
public void ZipFileByCode() { MemoryStream ms = new MemoryStream(); byte[] buffer = null; using (ZipFile file = ZipFile.Create(ms)) { file.BeginUpdate(); file.NameTransform = new MyNameTransfom();//通过这个名称格式化器,可以将里面的文件名进行一些处理。默认情况下,会自动根据文件的路径在zip中创建有关的文件夹。 file.Add(Server.MapPath("/Content/images/img01.jpg")); file.CommitUpdate(); buffer = new byte[ms.Length]; ms.Position = 0; ms.Read(buffer, 0, buffer.Length); } Response.AddHeader("content-disposition", "attachment;filename=test.zip"); Response.BinaryWrite(buffer); Response.Flush(); Response.End(); }
Same layer code as pageload
private void Download(IEnumerable<string> files, string zipFileName) { //根据所选文件打包下载 MemoryStream ms = new MemoryStream(); byte[] buffer = null; using (ZipFile file = ZipFile.Create(ms)) { file.BeginUpdate(); file.NameTransform = new MyNameTransfom();//通过这个名称格式化器,可以将里面的文件名进行一些处理。默认情况下,会自动根据文件的路径在zip中创建有关的文件夹。 foreach (var item in files) { file.Add(item); } //file.Add(Server.MapPath("../../BigFileUpLoadStorage/1.png")); file.CommitUpdate(); buffer = new byte[ms.Length]; ms.Position = 0; ms.Read(buffer, 0, buffer.Length); } Response.AddHeader("content-disposition", "attachment;filename=" + zipFileName); Response.BinaryWrite(buffer); Response.Flush(); Response.End(); }

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
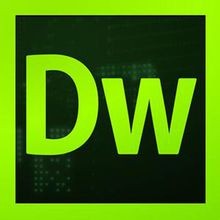
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

Zend Studio 13.0.1
Powerful PHP integrated development environment
