1.List sorting
This is different from the sorting of arrays.
In fact, Java has implementations for sorting arrays and Lists. For arrays, you can use Arrays.sort directly. For Lists and Vectors, you can use the Collections.sort method.
Java API provides 2 methods for sorting collection types:
java.util.Collections.sort(java.util.List)
java.util.Collections.sort(java.util.List, java.util .Comparator)
If the elements in the collection are all of the same type and implement the Comparable interface, you can call the first method directly.
If you have other sorting ideas, for example, if you don’t want to follow the natural sorting, you can also pass a Comparator, such as reverse.
The case of different elements is more complicated and can be ignored for now.
In general, if you have a new class, such as Player, and you want to sort it, let it implement the Comparable interface and implement the compareTo method. For example, if you want to sort by age, starting from the smallest, then you can implement it as follows:
public class Player implements Comparable<Player>{ private String name; private int age; public Player(String name, int age){ this.name=name; this.age=age; } public int getAge(){ return age; } public void setAge(int age){ this.age = age; } //实现接口方法,将来排序的时候sort看正负数还是零来进行判断大小 @Override public int compareTo(Player player){ return this.getAge() - player.getAge(); } }
The Comparable interface defaults to sorting in natural order. Of course, you can unexpectedly directly implement the compareTo method in reverse, or you can , just don’t do this according to the agreement. If you don’t confuse it, others may confuse it. If everything is done according to the agreement, it will be less likely to get confused. So Comparator is actually used when you are not satisfied with the natural sorting, or when simple natural sorting cannot achieve the sorting you want. For example, if you want to sort according to the absolute value of the values, obviously you cannot use Comparable. You have to write a Comparator implementation class yourself, implement the compare method, and return positive, negative, or zero numbers according to the way you want.
There are some Comparators that come with the system, such as Collections.reverseOrder(), String.CASE_INSENSITIVE_ORDER.
2.Set sorting
Java has an implementation class for Set that is arranged in natural order, TreeSet. Just operate the reference of this TreeSet object, and it will be sorted by itself. Of course, TreeSet also provides multiple constructors, especially the constructor that receives Comparator type parameters, allowing developers to sort according to their own ideas, not just limited to natural sorting.
Another way is to directly load the set into a list object, and then use sorting.
3.Map sorting
This is a little more troublesome.
Map is a key-value pair, so it can be sorted by key or value. Usually, because the keys cannot be the same, but the values can be the same, many values are used for sorting. Let’s give an example first.
Principle In fact, it is more convenient to convert to List in the end.
When traversing Map, you need to use something called Map.Entry. If you have a Map object map, then you can use map.entrySet() to get a set object, which is filled with Map.Entry. If you want Traversing, it is very simple to use iterator to get all the elements inside. If you want to sort, it is best to put this set into a list, then define a Comparator object, implement the compare method in it, return a difference, such as the difference in scores of athletes, and then use the Collections.sort method , pass in the list object and comparator object, and the sorting is completed.
Let’s take an example
public class MapSort{ public static void main(String[] args){ Map<String, Player> map = new HashMap<String, Player>(); Player p1 = new Player("John", 1000); Player p2 = new Player("Ben", 3000); Player p3 = new Player("Jack", 2000); map.put(p1); map.put(p2); map.put(p3); //将Map里面的所以元素取出来先变成一个set,然后将这个set装到一个list里面 List<Map.Entry<String, Player>> list = new ArrayList<Map.Entry<String, Player>>(map.entrySet()); //定义一个comparator Comparator<Map.Entry<String, Player>> comparator = new Comparator<Map.Entry<String, Player>>(){ @Override public int compare(Entry<String, Player> p1, Entry<String, Player> p2){ //之所以使用减号,是想要按照分数从高到低来排列 return -(p1.getValue().score - p2.getValue().score); } }; Collections.sort(list, comparator); for(Map.Entry<String, Player> entry:list){ System.out.println(entry.getValue().name + ":" + entry.getValue().score); } } } class Player{ String name; int score; public Player(String name, int score){ this.name == name; this.score == score; } }
After sorting it like this, the following content will be output in the end
Ben:3000
Jack:2000
John:1000
Speaking of the final summary, in fact, all Collection sorting can finally be converted to List sorting, because Collections itself provides support for List sorting.
Map can be turned into a set, and set can be turned into a list, so both can be turned into a list.
1. For the simplest case, the class to be sorted implements a Comparable interface, then implements the compare method, performs subtraction in a natural way, returns the result of the subtraction, and then directly uses Collections.sort(List list) The method will do. This kind is called natural sorting, which is only suitable for original List and Set.
2. If you don’t want to use natural sorting, no problem, then define a Comparator object, implement the logic there, and then use Collections.sort(List list, Comparator comparator).
3. For Map, it is a little more complicated, but the principle is the second case.
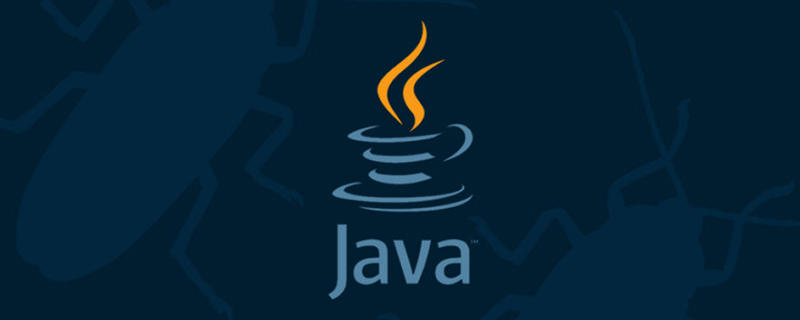
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
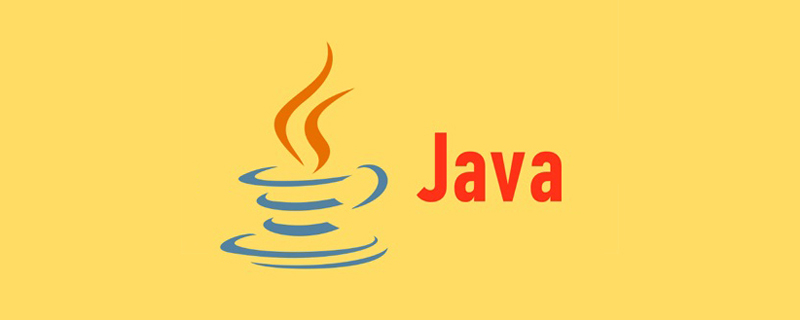
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
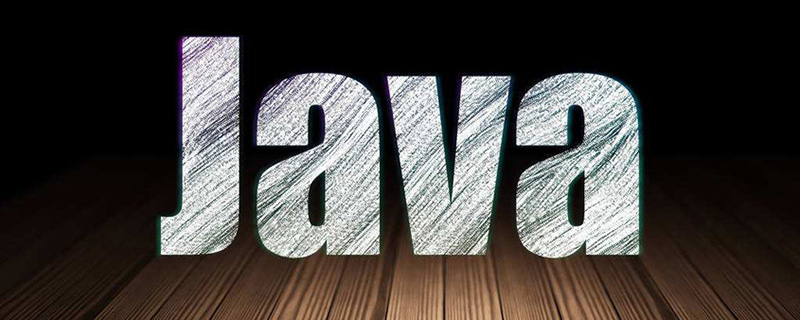
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
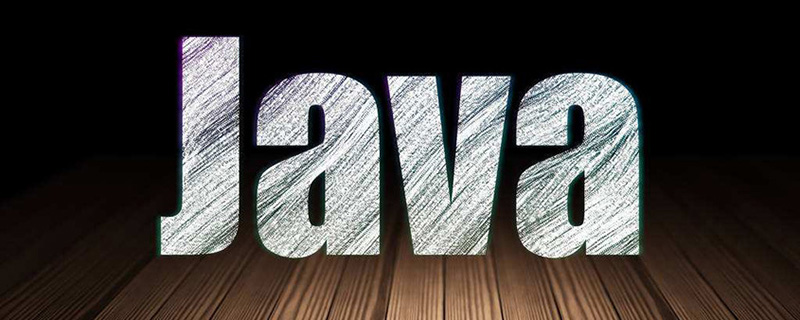
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
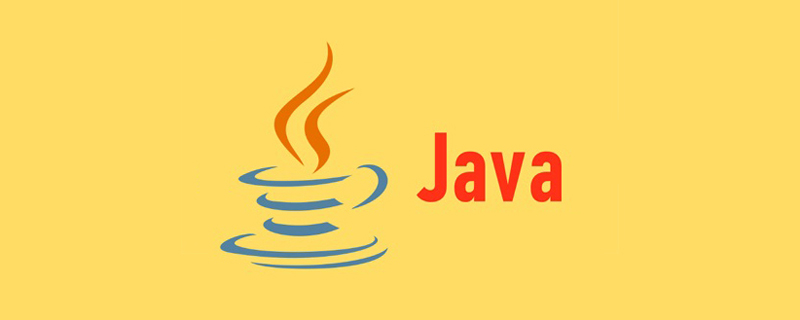
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
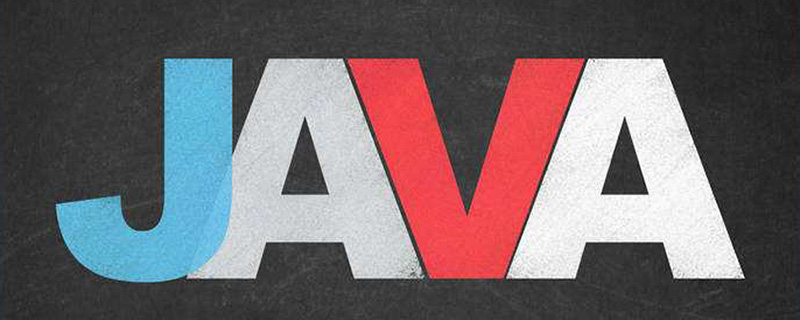
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
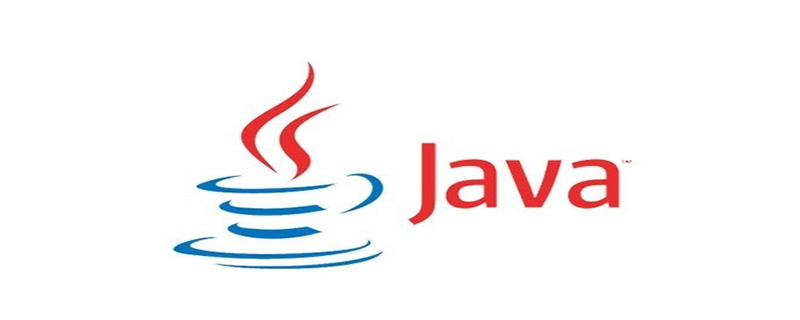
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。
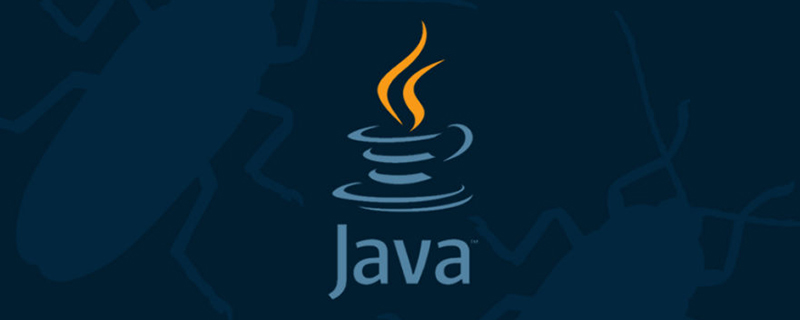
本篇文章给大家带来了关于Java的相关知识,其中主要整理了Stream流的概念和使用的相关问题,包括了Stream流的概念、Stream流的获取、Stream流的常用方法等等内容,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
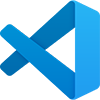
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft