This is the last new version before version 2.0. It has many new features, one of which is support for Source Map.
, open the compressed version, scroll to the bottom, you can see the last line is like this:
//@ sourceMappingURL=jquery.min.map
This is the Source Map. It is an independent map file in the same directory as the source code. You can click in to see what it looks like.
This is a very useful function. This article will explain this function in detail.
1. Start with source code conversion
JavaScript scripts are becoming more and more complex. Most source code (especially various function libraries and frameworks) must be converted before it can be put into the production environment.
Common source code conversion mainly involves the following three situations:
(1) Compression and reduction in size. For example, the source code of jQuery 1.9 is 252KB before compression and 32KB after compression.
(2) Merge multiple files to reduce the number of HTTP requests.
(3) Other languages are compiled into JavaScript. The most common example is CoffeeScript.
These three situations all make the actual running code different from the development code, making debugging difficult.
Usually, the JavaScript interpreter will tell you which line and column of code has an error. However, this does nothing for the transformed code. For example, jQuery 1.9 has only 3 lines after compression, each line has 30,000 characters, and all internal variables have been renamed. You look at the error message and feel clueless, not knowing the original location it corresponds to.
This is the problem that Source map wants to solve.
2. What is Source map
Simply put, Source map is an information file that stores location information. In other words, each position of the converted code corresponds to the position before conversion.
With it, when an error occurs, the debugging tool will directly display the original code instead of the converted code. This undoubtedly brings great convenience to developers.
Currently, only Chrome browser supports this feature. In the Settings of Developer Tools, make sure "Enable source maps" is selected.
3. How to enable Source map
As mentioned before, just add a line at the end of the converted code.
//@ sourceMappingURL=/path/to/file.js.map
map files can be placed on the network or in the local file system.
4. How to generate Source map
The most common method is to use Google's Closure compiler.
The format of the generated command is as follows:
java -jar compiler.jar
--js script.js
--create_source_map ./script-min.js.map
--source_ map_format=V3
U- JS_OUTPUT_FILE SCRIPT-min.js The meaning of each parameter is as follows: -JS: Create_source_map before conversion: generated Source Map files , currently all V3 is used. - js_output_file: Converted code file. For other generation methods, please refer to this article. 5. Source map format Open the Source map file, it looks like this: sourceRoot: "", sources: ["foo.js", "bar.js"], names: ["src", "maps", "are", "fun"], mappings: "AAgBC, SAAQ,CAAEA" } The entire file is a JavaScript object that can be read by the interpreter. It mainly has the following attributes: - version: the version of the Source map, currently 3. - file: converted file name. - sourceRoot: The directory where the file before conversion is located. If it is in the same directory as the file before conversion, this item will be empty. - sources: files before conversion. This item is an array, indicating that there may be multiple file merges. - names: All variable names and attribute names before conversion. - Mappings: Strings that record location information, detailed below. 6. Mappings attribute The following is the really interesting part: how the positions of the two files correspond one to one.
The key is the mappings attribute of the map file. This is a long string that is divided into three layers.
The first level is line correspondence, represented by a semicolon (;). Each semicolon corresponds to a line of converted source code. Therefore, the content before the first semicolon corresponds to the first line of the source code, and so on.
The second layer is position correspondence, represented by commas (,). Each comma corresponds to a position of the converted source code. Therefore, the content before the first comma corresponds to the first position of the source code of the line, and so on.
The third layer is position conversion, represented by VLQ encoding, which represents the source code position before conversion corresponding to the position.
For example, assume that the content of the mappings attribute is as follows:
Mappings: "AAAAA,BBBBB;CCCCC"
means that the converted source code is divided into two lines, and the first line has two positions , there is a position in the second row.
7. Principle of position correspondence
Each position uses five bits to represent five fields.
Counting from the left,
- the first digit, indicating which column (of the converted code) this position is in.
- The second digit indicates which file in the sources attribute this location belongs to.
- The third digit indicates which line of code this position belongs to before conversion.
- The fourth digit indicates which column of the code before conversion this position belongs to.
- The fifth digit indicates which variable in the names attribute this position belongs to.
There are a few points that need to be explained. First, all values are base 0. Secondly, the fifth position is not required. If the position does not correspond to the variable in the names attribute, the fifth position can be omitted. Again, each bit is represented by VLQ encoding; since VLQ encoding is variable length, each bit can be composed of multiple characters.
If a certain position is AAAAA, since A represents 0 in VLQ encoding, the five bits in this position are actually 0. It means that the position is in the 0th column of the converted code, corresponding to the 0th file in the sources attribute, and belongs to the 0th row and 0th column of the pre-converted code, corresponding to the 0th variable in the names attribute.
8. VLQ encoding
Finally, let’s talk about how to use VLQ encoding to represent values.
This encoding was first used for MIDI files and was later adopted by many formats. Its characteristic is that it can express large values very concisely.
VLQ encoding is variable length. If the (integer) value is between -15 and +15 (inclusive), it is represented by one character; if it exceeds this range, it needs to be represented by multiple characters. It stipulates that each character uses 6 binary bits, which can be borrowed from the Base 64 encoded character table.
Among these 6 bits, the first bit on the left (the highest bit) indicates whether it is "continuation". If it is 1, it means that the 6 bits after these 6 bits also belong to the same number; if it is 0, it means that the value ends with these 6 bits.
Continuation
| bit), depends on whether these 6 bits are certain The first character of the VLQ encoding of a numeric value. If yes, this bit represents the "sign" (sign), 0 is positive, 1 is negative (the sign of the Source map is fixed to 0); if not, this bit has no special meaning and is counted as part of the value.
9. VLQ encoding: example
Let’s look at an example of how to VLQ encode the value 16.
The first step is to rewrite 16 into binary form 10000.
The second step is to add the sign bit on the far right. Because 16 is greater than 0, the sign bit is 0, and the entire number becomes 100000.
The third step, starting from the lowest bit on the right, segment the entire number every 5 digits, that is, into two segments: 1 and 00000. If the segment where the highest bit is located is less than 5 digits, 0 is added in front, so the two segments become 00001 and 00000.
The fourth step is to reverse the order of the two paragraphs, namely 00000 and 00001.
The fifth step is to add a "continuous bit" at the front of each segment. Except for the last segment which is 0, the others are all 1, which becomes 100000 and 000001.
The sixth step is to convert each paragraph into Base 64 encoding.
Looking up the table shows that 100000 is g and 000001 is B. Therefore, the VLQ code for the value 16 is gB. The above process may seem complicated, but it is actually very simple to do. For the specific implementation, please see the official base64-vlq.js file, which has detailed comments.
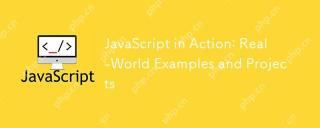
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
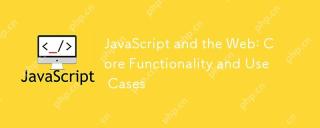
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
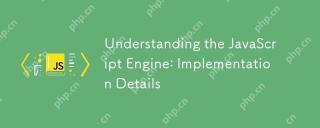
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
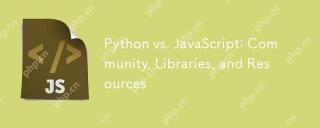
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
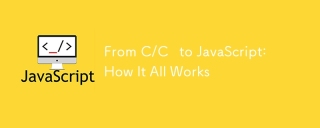
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
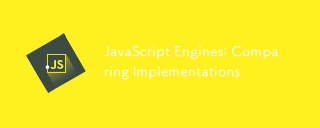
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
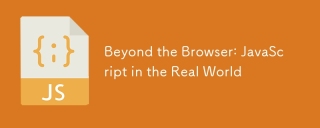
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
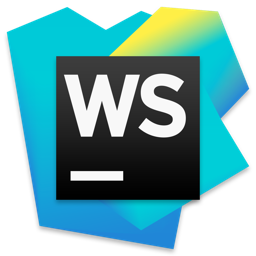
WebStorm Mac version
Useful JavaScript development tools
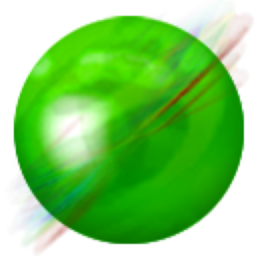
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.