


The following are the top 10 algorithm-related concepts in programming interviews. I will illustrate these concepts through some simple examples. Since fully mastering these concepts requires more effort, this list is intended only as an introduction. This article will look at the problem from a Java perspective, including the following concepts:
1. String
If the IDE does not have the code auto-completion function, you should remember the following methods.
toCharArray() // 获得字符串对应的char数组 Arrays.sort() // 数组排序Arrays.toString(char[] a) // 数组转成字符串 charAt(int x) // 获得某个索引处的字符 length() // 字符串长度 length // 数组大小
2. Linked list
In Java, the implementation of linked list is very simple. Each node Node has a value val and a link next pointing to the next node.
class Node { int val; Node next; Node(int x) { val = x; next = null; } }
Two famous applications of linked lists are Stack and Queue.
Stack:
class Stack{ Node top; public Node peek(){ if(top != null){ return top; } return null; } public Node pop(){ if(top == null){ return null; }else{ Node temp = new Node(top.val); top = top.next; return temp; } } public void push(Node n){ if(n != null){ n.next = top; top = n; } } }
Queue:
class Queue{ Node first, last; public void enqueue(Node n){ if(first == null){ first = n; last = first; }else{ last.next = n; last = n; } } public Node dequeue(){ if(first == null){ return null; }else{ Node temp = new Node(first.val); first = first.next; return temp; } } }
3. Tree
The tree here usually refers to a binary tree. Each node contains a left child node and a right child node, like the following:
class TreeNode{ int value; TreeNode left; TreeNode right; }
The following is the tree with Some related concepts:
Balanced vs. Unbalanced: In a balanced binary tree, the depth difference between the left and right subtrees of each node is at most 1 (1 or 0).
Full Binary Tree: Every node except the leaf nodes has two children.
Perfect Binary Tree: It is a full binary tree with the following properties: all leaf nodes have the same depth or are at the same level, and each parent node must have two children.
Complete Binary Tree: In a binary tree, every level except maybe the last one is completely filled, and all nodes must be as close to the left as possible.
Translator’s Note: A perfect binary tree is also vaguely called a complete binary tree. An example of a perfect binary tree is the ancestry graph of a person at a given depth, since every person must have two biological parents. A complete binary tree can be thought of as a perfect binary tree that can have a number of additional leaf nodes leaning to the left. Question: What is the difference between a perfect binary tree and a full binary tree? (Reference: http://xlinux.nist.gov/dads/HTML/perfectBinaryTree.html)
4. Graph
Graph-related issues are mainly focused on depth first search (depth first search) and breadth first search (breath first) search).
The following is a simple implementation of graph breadth first search.
1) Define GraphNode
class GraphNode{ int val; GraphNode next; GraphNode[] neighbors; boolean visited; GraphNode(int x) { val = x; } GraphNode(int x, GraphNode[] n){ val = x; neighbors = n; } public String toString(){ return "value: "+ this.val; } }
2) Define a queue Queue
class Queue{ GraphNode first, last; public void enqueue(GraphNode n){ if(first == null){ first = n; last = first; }else{ last.next = n; last = n; } } public GraphNode dequeue(){ if(first == null){ return null; }else{ GraphNode temp = new GraphNode(first.val, first.neighbors); first = first.next; return temp; } } }
3) Use queue Queue to implement breadth-first search
public class GraphTest { public static void main(String[] args) { GraphNode n1 = new GraphNode(1); GraphNode n2 = new GraphNode(2); GraphNode n3 = new GraphNode(3); GraphNode n4 = new GraphNode(4); GraphNode n5 = new GraphNode(5); n1.neighbors = new GraphNode[]{n2,n3,n5}; n2.neighbors = new GraphNode[]{n1,n4}; n3.neighbors = new GraphNode[]{n1,n4,n5}; n4.neighbors = new GraphNode[]{n2,n3,n5}; n5.neighbors = new GraphNode[]{n1,n3,n4}; breathFirstSearch(n1, 5); } public static void breathFirstSearch(GraphNode root, int x){ if(root.val == x) System.out.println("find in root"); Queue queue = new Queue(); root.visited = true; queue.enqueue(root); while(queue.first != null){ GraphNode c = (GraphNode) queue.dequeue(); for(GraphNode n: c.neighbors){ if(!n.visited){ System.out.print(n + " "); n.visited = true; if(n.val == x) System.out.println("Find "+n); queue.enqueue(n); } } } } }
Output:
1 value: 2 value: 3 value: 5 Find value: 5
2 value: 4
5. Sorting
The following is the time complexity of different sorting algorithms. You can go to the wiki to take a look at the basic ideas of these algorithms.
Also, here are some implementations/demos: Counting sort, Mergesort, Quicksort, InsertionSort.
"Visually intuitive experience of 7 commonly used sorting algorithms"
"Video: 6-minute demonstration of 15 sorting algorithms"
6. Recursion vs. Iteration
For programmers, recursion should be an innate A built-in thought can be illustrated with a simple example.
Question: There are n steps, and you can only go up 1 or 2 steps at a time. How many ways are there?
Step 1: Find the relationship between the first n steps and the first n-1 steps.
In order to walk up n steps, there are only two ways: climb 1 step from n-1 steps to get there or climb 2 steps from n-2 steps to get there. If f(n) is the number of ways to climb to the nth step, then f(n) = f(n-1) + f(n-2).
Step 2: Make sure the start conditions are correct.
f(0) = 0;
f(1) = 1;
public static int f(int n){ if(n <= 2) return n; int x = f(n-1) + f(n-2); return x; }
The time complexity of the recursive method is exponential in n, because there are many redundant calculations, as follows:
f(5) f(4) + f(3) f(3) + f(2) + f(2) + f(1) f(2) + f(1) + f(1) + f(0) + f(1) + f(0) + f(1) f(1) + f(0) + f(1) + f(1) + f(0) + f(1) + f(0) + f(1)
The direct idea is to Convert recursion to iteration:
public static int f(int n) { if (n <= 2){ return n; } int first = 1, second = 2; int third = 0; for (int i = 3; i <= n; i++) { third = first + second; first = second; second = third; } return third; }
For this example, iteration takes less time, you may also want to take a look at Recursion vs Iteration.
7. Dynamic Programming
Dynamic programming is a technique for solving problems of the following nature:
A problem can be solved by solving smaller sub-problems (Translator’s Note: The optimal solution to the problem contains its sub-problems The optimal solution, that is, the optimal substructure properties).
The solutions to some sub-problems may need to be calculated multiple times (Translator’s Note: This is the overlapping nature of sub-problems).
The solutions to the subproblems are stored in a table, so that each subproblem only needs to be calculated once.
Requires extra space to save time.
The step climbing problem completely conforms to the above four properties, so it can be solved by dynamic programming method.
public static int[] A = new int[100]; public static int f3(int n) { if (n <= 2) A[n]= n; if(A[n] > 0) return A[n]; else A[n] = f3(n-1) + f3(n-2);//store results so only calculate once! return A[n]; }
8. Bit operations
Bit operators:
获得给定数字n的第i位:(i从0计数并从右边开始)
public static boolean getBit(int num, int i){ int result = num & (1<<i); if(result == 0){ return false; }else{ return true; }
例如,获得数字10的第2位:
i=1, n=10
11010&10=10
10 is not 0, so return true;
9. 概率问题
解决概率相关的问题通常需要很好的规划了解问题(formatting the problem),这里刚好有一个这类问题的简单例子:
一个房间里有50个人,那么至少有两个人生日相同的概率是多少?(忽略闰年的事实,也就是一年365天)
计算某些事情的概率很多时候都可以转换成先计算其相对面。在这个例子里,我们可以计算所有人生日都互不相同的概率,也就是:365/365 * 364/365 * 363/365 * … * (365-49)/365,这样至少两个人生日相同的概率就是1 – 这个值。
public static double caculateProbability(int n){ double x = 1; for(int i=0; i<n; i++){ x *= (365.0-i)/365.0; } double pro = Math.round((1-x) * 100); return pro/100;
calculateProbability(50) = 0.97
10. 排列组合
组合和排列的区别在于次序是否关键。
如果你有任何问题请在下面评论。
参考/推荐资料:
1. Binary tree
2. Introduction to Dynamic Programming
3. UTSA Dynamic Programming slides
4. Birthday paradox
5. Cracking the Coding Interview: 150 Programming Interview Questions and Solutions, Gayle Laakmann McDowell
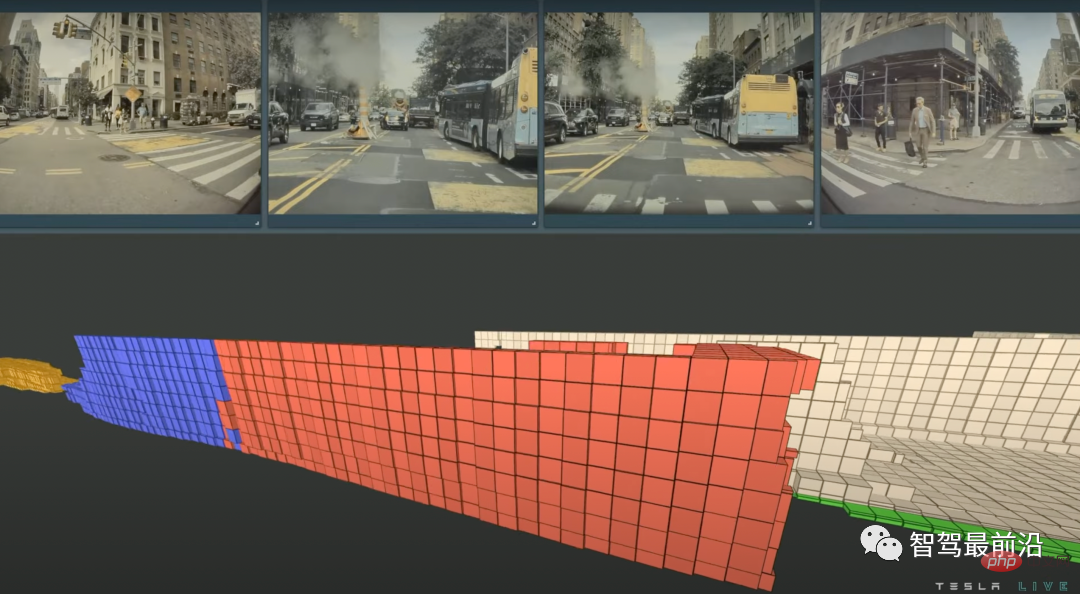
特斯拉是一个典型的AI公司,过去一年训练了75000个神经网络,意味着每8分钟就要出一个新的模型,共有281个模型用到了特斯拉的车上。接下来我们分几个方面来解读特斯拉FSD的算法和模型进展。01 感知 Occupancy Network特斯拉今年在感知方面的一个重点技术是Occupancy Network (占据网络)。研究机器人技术的同学肯定对occupancy grid不会陌生,occupancy表示空间中每个3D体素(voxel)是否被占据,可以是0/1二元表示,也可以是[0, 1]之间的
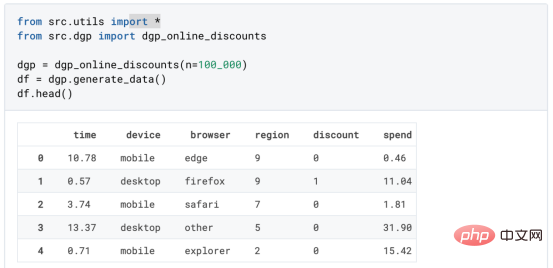
译者 | 朱先忠审校 | 孙淑娟在我之前的博客中,我们已经了解了如何使用因果树来评估政策的异质处理效应。如果你还没有阅读过,我建议你在阅读本文前先读一遍,因为我们在本文中认为你已经了解了此文中的部分与本文相关的内容。为什么是异质处理效应(HTE:heterogenous treatment effects)呢?首先,对异质处理效应的估计允许我们根据它们的预期结果(疾病、公司收入、客户满意度等)选择提供处理(药物、广告、产品等)的用户(患者、用户、客户等)。换句话说,估计HTE有助于我
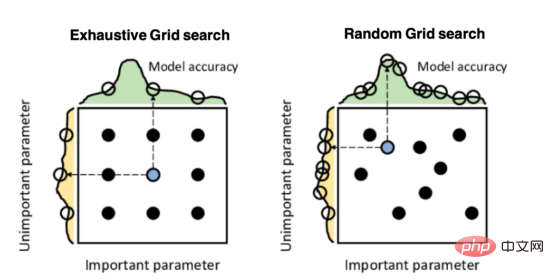
译者 | 朱先忠审校 | 孙淑娟引言模型超参数(或模型设置)的优化可能是训练机器学习算法中最重要的一步,因为它可以找到最小化模型损失函数的最佳参数。这一步对于构建不易过拟合的泛化模型也是必不可少的。优化模型超参数的最著名技术是穷举网格搜索和随机网格搜索。在第一种方法中,搜索空间被定义为跨越每个模型超参数的域的网格。通过在网格的每个点上训练模型来获得最优超参数。尽管网格搜索非常容易实现,但它在计算上变得昂贵,尤其是当要优化的变量数量很大时。另一方面,随机网格搜索是一种更快的优化方法,可以提供更好的
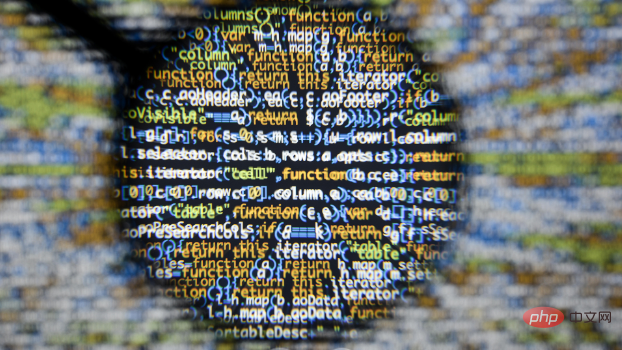
导读:因果推断是数据科学的一个重要分支,在互联网和工业界的产品迭代、算法和激励策略的评估中都扮演者重要的角色,结合数据、实验或者统计计量模型来计算新的改变带来的收益,是决策制定的基础。然而,因果推断并不是一件简单的事情。首先,在日常生活中,人们常常把相关和因果混为一谈。相关往往代表着两个变量具有同时增长或者降低的趋势,但是因果意味着我们想要知道对一个变量施加改变的时候会发生什么样的结果,或者说我们期望得到反事实的结果,如果过去做了不一样的动作,未来是否会发生改变?然而难点在于,反事实的数据往往是
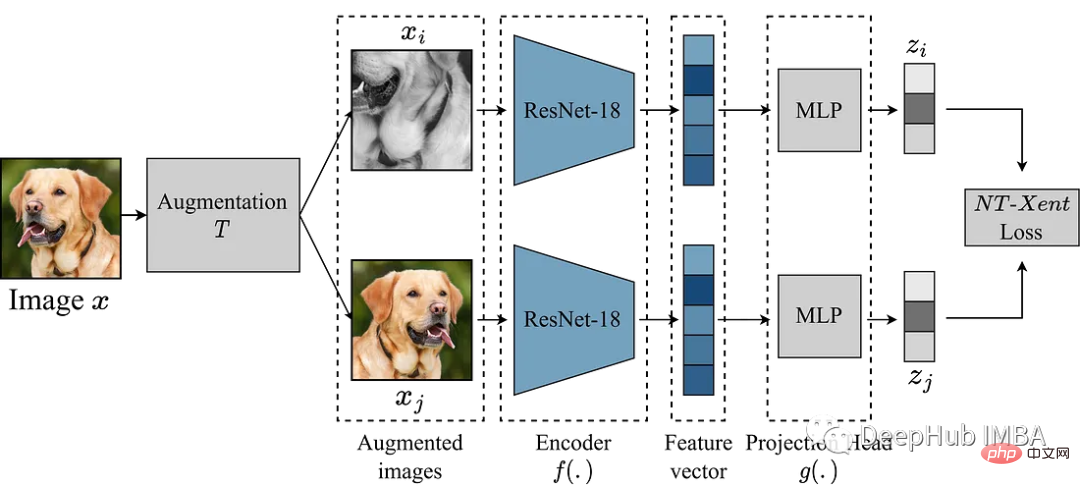
SimCLR(Simple Framework for Contrastive Learning of Representations)是一种学习图像表示的自监督技术。 与传统的监督学习方法不同,SimCLR 不依赖标记数据来学习有用的表示。 它利用对比学习框架来学习一组有用的特征,这些特征可以从未标记的图像中捕获高级语义信息。SimCLR 已被证明在各种图像分类基准上优于最先进的无监督学习方法。 并且它学习到的表示可以很容易地转移到下游任务,例如对象检测、语义分割和小样本学习,只需在较小的标记
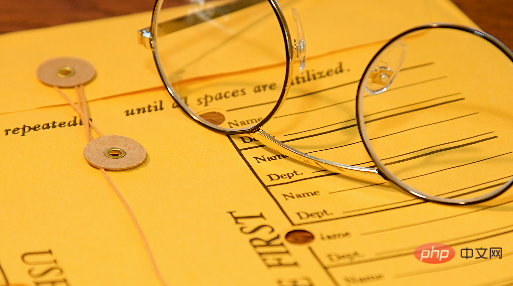
一、盒马供应链介绍1、盒马商业模式盒马是一个技术创新的公司,更是一个消费驱动的公司,回归消费者价值:买的到、买的好、买的方便、买的放心、买的开心。盒马包含盒马鲜生、X 会员店、盒马超云、盒马邻里等多种业务模式,其中最核心的商业模式是线上线下一体化,最快 30 分钟到家的 O2O(即盒马鲜生)模式。2、盒马经营品类介绍盒马精选全球品质商品,追求极致新鲜;结合品类特点和消费者购物体验预期,为不同品类选择最为高效的经营模式。盒马生鲜的销售占比达 60%~70%,是最核心的品类,该品类的特点是用户预期时
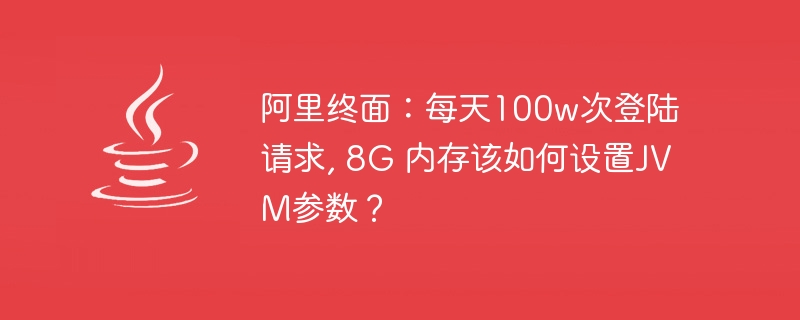
就在上周,一个同学在阿里云技术面终面的时候被问到这么一个问题:假设一个每天100w次登陆请求的平台,一个服务节点 8G 内存,该如何设置JVM参数? 觉得回答的不太理想,过来找我复盘。
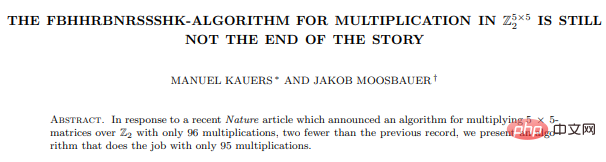
10 月 5 日,AlphaTensor 横空出世,DeepMind 宣布其解决了数学领域 50 年来一个悬而未决的数学算法问题,即矩阵乘法。AlphaTensor 成为首个用于为矩阵乘法等数学问题发现新颖、高效且可证明正确的算法的 AI 系统。论文《Discovering faster matrix multiplication algorithms with reinforcement learning》也登上了 Nature 封面。然而,AlphaTensor 的记录仅保持了一周,便被人类


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
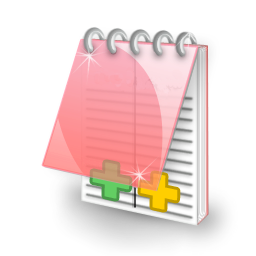
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 English version
Recommended: Win version, supports code prompts!
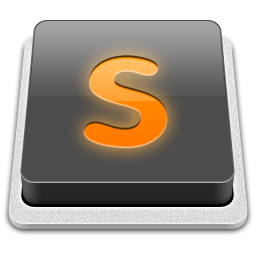
SublimeText3 Mac version
God-level code editing software (SublimeText3)
