In our cloud usage analysis API, formatted analysis data is returned (here refers to generating analysis graphs). Recently, we added a feature that allows users to select a time period (initially only by day). The problem is that the time period of each day in the code is highly coupled...
For example, the following code:
private static List<DataPoint> createListWithZerosForTimeInterval(DateTime from, DateTime to, ImmutableSet<Metric<? extends Number>> metrics) { List<DataPoint> points = new ArrayList<>(); for (int i = 0; i <= Days.daysBetween(from, to).getDays(); i++) { points.add(new DataPoint().withDatas(createDatasWithZeroValues(metrics)) .withDayOfYear(from.withZone(DateTimeZone.UTC) .plusDays(i) .withTimeAtStartOfDay())); } return points; }
Note: Days, Minutes, Hours, Weeks and Months appear at the back of the code part. This code comes from the Joda-Time Java time and date API. Even the names of the methods don't reflect (their respective functionality). These names are firmly tied to the concept of days.
I have also tried using different time periods (such as months, weeks, hours). But I've seen bad switch/cases sneaking around in code.
You need to know that switch/case=sin has penetrated deeply into my heart. I already thought so during my two internship experiences during college. Therefore, I would avoid switch/case at all costs. This is mainly because they violate the open-closed principle. I deeply believe that following this principle is the best practice for writing object-oriented code. I'm not the only one who thinks this way, Robert C. Martin once said:
In many ways, the open-closed principle is the core of object-oriented design. Following this principle will reap tremendous benefits from object-oriented techniques, such as reusability and maintainability1.
I told myself: "We may be able to discover some new features using Java8 to avoid dangerous switch/case situations." Use Java 8's new functions (not that new, but you know what I mean). I decided to use an enum to represent the different available time periods.
public enum TimePeriod { MINUTE(Dimension.MINUTE, (from, to) -> Minutes.minutesBetween(from, to).getMinutes() + 1, Minutes::minutes, from -> from.withZone(DateTimeZone.UTC) .withSecondOfMinute(0) .withMillisOfSecond(0)), HOUR(Dimension.HOUR, (from, to) -> Hours.hoursBetween(from, to).getHours() + 1, Hours::hours, from -> from.withZone(DateTimeZone.UTC) .withMinuteOfHour(0) .withSecondOfMinute(0) .withMillisOfSecond(0)), DAY(Dimension.DAY, (from, to) -> Days.daysBetween(from, to).getDays() + 1, Days::days, from -> from.withZone(DateTimeZone.UTC) .withTimeAtStartOfDay()), WEEK(Dimension.WEEK, (from, to) -> Weeks.weeksBetween(from, to).getWeeks() + 1, Weeks::weeks, from -> from.withZone(DateTimeZone.UTC) .withDayOfWeek(1) .withTimeAtStartOfDay()), MONTH(Dimension.MONTH, (from, to) -> Months.monthsBetween(from, to).getMonths() + 1, Months::months, from -> from.withZone(DateTimeZone.UTC) .withDayOfMonth(1) .withTimeAtStartOfDay()); private Dimension<Timestamp> dimension; private BiFunction<DateTime, DateTime, Integer> getNumberOfPoints; private Function<Integer, ReadablePeriod> getPeriodFromNbOfInterval; private Function<DateTime, DateTime> getStartOfInterval; private TimePeriod(Dimension<Timestamp> dimension, BiFunction<DateTime, DateTime, Integer> getNumberOfPoints, Function<Integer, ReadablePeriod> getPeriodFromNbOfInterval, Function<DateTime, DateTime> getStartOfInterval) { this.dimension = dimension; this.getNumberOfPoints = getNumberOfPoints; this.getPeriodFromNbOfInterval = getPeriodFromNbOfInterval; this.getStartOfInterval = getStartOfInterval; } public Dimension<Timestamp> getDimension() { return dimension; } public int getNumberOfPoints(DateTime from, DateTime to) { return getNumberOfPoints.apply(from, to); } public ReadablePeriod getPeriodFromNbOfInterval(int nbOfInterval) { return getPeriodFromNbOfInterval.apply(nbOfInterval); } public DateTime getStartOfInterval(DateTime from) { return getStartOfInterval.apply(from); } }
With the enumeration, I was able to easily modify the code to allow the user to specify a time period for the chart data points.
The original call was like this:
for (int i = 0; i <= Days.daysBetween(from, to).getDays(); i++)
became the call like this:
for (int i = 0; i < timePeriod.getNumberOfPoints(from, to); i++)
The Usage Analytics service code that supports the getGraphDataPoints call has been completed and supports time periods. It is worth mentioning that it takes into account the open-closed principle I said before.
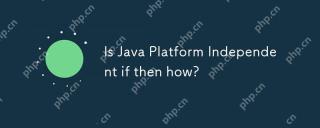
Java is platform-independent because of its "write once, run everywhere" design philosophy, which relies on Java virtual machines (JVMs) and bytecode. 1) Java code is compiled into bytecode, interpreted by the JVM or compiled on the fly locally. 2) Pay attention to library dependencies, performance differences and environment configuration. 3) Using standard libraries, cross-platform testing and version management is the best practice to ensure platform independence.
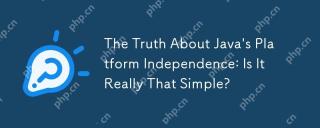
Java'splatformindependenceisnotsimple;itinvolvescomplexities.1)JVMcompatibilitymustbeensuredacrossplatforms.2)Nativelibrariesandsystemcallsneedcarefulhandling.3)Dependenciesandlibrariesrequirecross-platformcompatibility.4)Performanceoptimizationacros
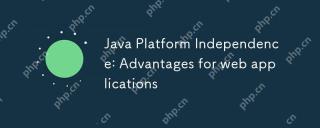
Java'splatformindependencebenefitswebapplicationsbyallowingcodetorunonanysystemwithaJVM,simplifyingdeploymentandscaling.Itenables:1)easydeploymentacrossdifferentservers,2)seamlessscalingacrosscloudplatforms,and3)consistentdevelopmenttodeploymentproce
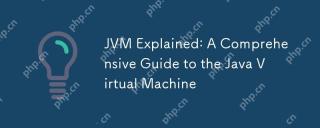
TheJVMistheruntimeenvironmentforexecutingJavabytecode,crucialforJava's"writeonce,runanywhere"capability.Itmanagesmemory,executesthreads,andensuressecurity,makingitessentialforJavadeveloperstounderstandforefficientandrobustapplicationdevelop
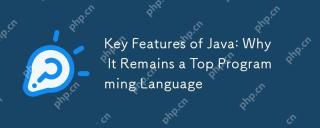
Javaremainsatopchoicefordevelopersduetoitsplatformindependence,object-orienteddesign,strongtyping,automaticmemorymanagement,andcomprehensivestandardlibrary.ThesefeaturesmakeJavaversatileandpowerful,suitableforawiderangeofapplications,despitesomechall
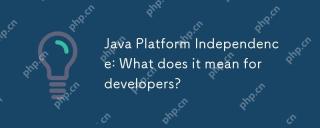
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
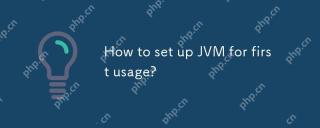
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
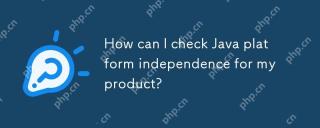
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
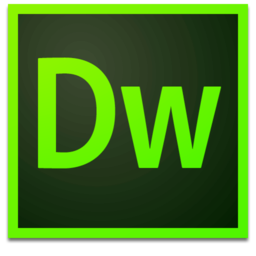
Dreamweaver Mac version
Visual web development tools
