String operations in JavaScript
1. Overview
Strings are almost everywhere in JavaScript. When you process user input data, when you read or set the attributes of DOM objects, when you operate cookies, Of course there is more…. The core part of JavaScript provides a set of properties and methods for common string operations, such as splitting strings, changing the case of strings, operating substrings, etc.
Most current browsers can also benefit from the power of regular expressions, as it greatly simplifies a large number of string manipulation tasks, but it also requires you to overcome a somewhat steep learning curve. Here, we mainly introduce some operations on the string itself. Regular expressions will be covered in future essays.
2. Creation of String
There are several ways to create a string. The simplest is to enclose a set of characters in quotes, which can be assigned to a string variable.
var myStr = "Hello, String!";
You can use double quotes or single quotes to include a string, but be aware that the pair of quotes that delimit the string must be the same and cannot be mixed.
A statement like var myString = "Fluffy is a pretty cat.'; is illegal.
Allows the use of two types of quotes, making certain operations simple, such as embedding one into the other:
document.write("<img src='img/logo.jpg' height='30' width='100' alt='Logo'>");
We created several strings in the above script, but in essence, they are not real string objects. To be precise, they are string type values. To create a string object, you can. Use the following statement: var strObj = new String("Hello, String!");
Use the typeof operator to see that the myStr type above is string, and the strObj type is object
If you want to know the string. The length, use its length attribute: string.length.
Method to get the character at the specified position of the string: string.charAt(index); Concatenate one or more strings into one large string
Solution:
Very simple, just use a "+" to "add" the two strings:
var longString = "One piece " + "plus one more piece.";
To concatenate multiple characters The string is accumulated into a string, you can also use the "+=" operator:
var result = ""; result += "My name is Anders" result += " and my age is 25";To add a newline character to the string, you need to use the escape character "n":
var confirmString = "You did not enter a response to the last " + "question.\n\nSubmit form anyway?"; var confirmValue = confirm(confirmString);
But this method only Can be used in situations like warnings and confirmation dialog boxes. If this text is presented as HTML content, it will be invalid. In this case, use "
" to replace it:
var htmlString = "First line of string.<br>Second line of string."; document.write(htmlString);
String object The method concat() is also provided, which performs the same function as "+":
string.concat(value1, value2, ...)
But the concat() method is obviously not as intuitive and concise as "+"
4. The problem of accessing substrings of strings
. :
Get a copy of a part of a string.
Solution:
Use the substring() or slice() method (NN4+, IE4+). Their specific usage is explained below.
The prototype of substring() is: string.substring(from, to)
The first parameter from specifies the starting position of the substring in the original string (0-based index); the second parameter to is optional and specifies the subcharacter The string is at the end of the original string (0-based index). Generally, it should be larger than from. If it is omitted, the substring will go to the end of the original string.
If the parameter from is not careful. What will happen if it is larger than the parameter to? JavaScript will automatically adjust the starting and ending positions of the substring, that is, substring() always starts from the smaller of the two parameters and ends with the larger one. Note, however, that it includes the character at the starting position, but not the character at the ending position.
var fullString = "Every dog has his day."; var section = fullString.substring(0, 4); // section is "Ever". section = fullString.substring(4, 0); // section is also "Ever". section = fullString.substring(1, 1); // section is an empty string. section = fullString.substring(-2, 4); // section is "Ever", same as fullString.substring(0, 4);
The prototype of slice() is: string.slice(start, end)
The parameter start represents the starting position of the substring. If it is a negative number, it can be understood as the starting position from the bottom, for example -3 means Start from the third to last; the parameter end represents the end position. Like start, it can also be a negative number, and its meaning also indicates the end of the penultimate position. The parameters of slice() can be negative, so it is more flexible than substring(), but less tolerant. If start is larger than end, it will return an empty string (example omitted).
Another method is substr(), whose prototype is: string.substr(start, length)
From the prototype, we can see the meaning of its parameters. start represents the starting position, and length represents the length of the substring. . The JavaScript standard discourages the use of this method.
5. Case conversion of strings
Question:
在你的页面上有文本框接收用户的输入信息,比如城市,然后你会根据他的城市的不同做不同的处理,这时自然会用到字符串比较,那么在比较前,最好进行大小写转换,这样只要考虑转换后的情形即可;或者要在页面上收集数据,然后将这些数据存储在数据库,而数据库恰好只接收大写字符;在这些情况下,我们都要考虑对字符串进行大小写转换。
解决方案:
使用toLowerCase()和toUpperCase()方法:
var city = "ShanGHai"; city = city.toLowerCase(); // city is "shanghai" now.
六、判断两个字符串是否相等
问题:
比如,你想拿用户的输入值与已知的字符串比较
解决方案:
先将用户的输入值全部转换为大写(或小写),然后再行比较:
var name = document.form1.txtUserName.value.toLowerCase(); if(name == "urname") { // statements go here. }
JavaScript有两种相等运算符。一种是完全向后兼容的,标准的"==",如果两个操作数类型不一致,它会在某些时候自动对操作数进行类型转换,考虑下面的赋值语句:
var strA = "i love you!"; var strB = new String("i love you!");
这两个变量含有相同的字符序列,但数据类型却不同,前者为string,后者为object,在使用"=="操作符时,JavaScript会尝试各种求值,以检测两者是否会在某种情况下相等。所以下面的表达式结果为true: strA == strB。
第二种操作符是"严格"的"===",它在求值时不会这么宽容,不会进行类型转换。所以表达式strA === strB的值为false,虽然两个变量持有的值相同。
有时代码的逻辑要求你判断两个值是否不相等,这里也有两个选择:"!="和严格的"!==",它们的关系就类似于"=="和"==="。
讨论:
"=="和"!="在求值时会尽可能地寻找值的匹配性,但你可能还是想在比较前进行显式的类型转换,以"帮助"它们完成工作。比如,如果想判断一个用户的输入值(字符串)是否等于一个数字,你可以让"=="帮你完成类型转换:
if(document.form1.txtAge.value == someNumericVar) { ... }
也可以提前转换:
if(parseInt(document.form1.txtAge.value) == someNumericVar) { ... }
如果你比较习惯于强类型的编程语言(比如C#,Java等),那么这里你可以延续你的习惯(类型转换),这样也会增强程序的可读性。
有一种情况需要注意,就是计算机的区域设置。如果用""来比较字符串,那么JavaScript把它们作为Unicode来比较,但显然,人们在浏览网页时不会把文本当作Unicode来阅读:)比如在西班牙语中,按照传统的排序,"ch"将作为一个字符排在"c"和"d"之间。localeCompare()提供了一种方式,可以帮助你使用默认区域设置下的字符排序规则。
var strings; // 要排序的字符串数组,假设已经得到初始化
strings.sort(function(a,b) { return a.localeCompare(b) }); // 调用sort()方法进行排序
七、字符串的查找
问题:
判断一个字符串是否包含另一个字符串。
解决方案:
使用string的indexOf()方法:
strObj.indexOf(subString[, startIndex])
strObj为要进行判断的字符串,subString为要在strObj查找的子字符串,startIndex是可选的,表示查找的开始位置(基于0的索引),如果startIndex省略,则从strObj开始处查找,如果startIndex小于0,则从0开始,如果startIndex大于最大索引,则从最大索引处开始。
indexOf()返回strObj中subString的开始位置,如果没有找到,则返回-1。在脚本中,可以这么使用:
if(largeString.indexOf(shortString) != -1) { // 如果包含,进行相应处理; }
也许一个字符串会包含另一字符串不止一次,这时第二个参数startIndex也许会派上用场,下面这个函数演示如何求得一个字符串包含另外一个字符串的次数:
function countInstances(mainStr, subStr) { var count = 0; var offset = 0; do { offset = mainStr.indexOf(subStr, offset); if(offset != -1) { count++; offset += subStr.length; } }while(offset != -1) return count; }
String对象有一个与indexOf()对应的方法,lastIndexOf():
strObj.lastIndexOf(substring[, startindex])
strObj为要进行判断的字符串,subString为要在strObj查找的子字符串,startIndex是可选的,表示查找的开始位置(基于0的索引),如果startIndex省略,则从strObj末尾处查找,如果startIndex小于0,则从0开始,如果startIndex大于最大索引,则从最大索引处开始。该方法自右向左查找,返回subString在strObj中最后出现的位置,如果没有找到,返回-1。
八、在Unicode值和字符串中的字符间转换
问题:
获得一个字符的Unicode编码值,反之亦然。
解决方案:
要获得字符的Unicode编码,可以使用string.charCodeAt(index)方法,其定义为:
strObj.charCodeAt(index)
index为指定字符在strObj对象中的位置(基于0的索引),返回值为0与65535之间的16位整数。例如:
var strObj = "ABCDEFG"; var code = strObj.charCodeAt(2); // Unicode value of character 'C' is 67
如果index指定的索引处没有字符,则返回值为NaN。
要将Unicode编码转换为一个字符,使用String.fromCharCode()方法,注意它是String对象的一个"静态方法",也就是说在使用前不需要创建字符串实例:
String.fromCharCode(c1, c2, ...)
它接受0个或多个整数,返回一个字符串,该字符串包含了各参数指定的字符,例如:
var str = String.fromCharCode(72, 101, 108, 108, 111); // str == "Hello"
讨论:
Unicode包含了这个世界上很多书写语言的字符集,但别因为Unicode包含一个字符就期望这个字符能够在警告对话框、文本框或页面呈现时正常显示。如果字符集不可用,在页面将显示为问号或其它符号。一台典型的北美的计算机将不能在屏幕上显示中文字符,除非中文的字符集及其字体已经安装。
以上就是JavaScript中的字符串操作的内容,更多相关内容请关注PHP中文网(www.php.cn)!
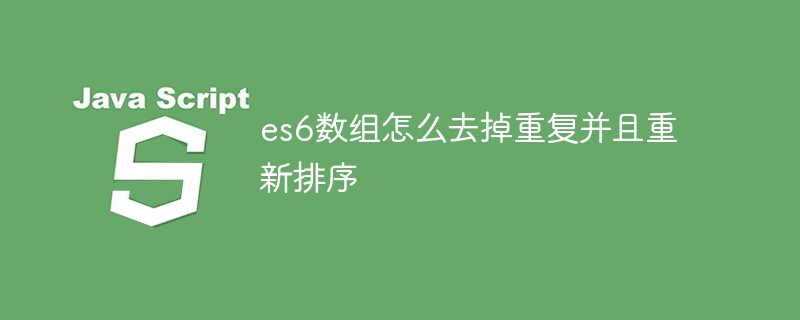
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
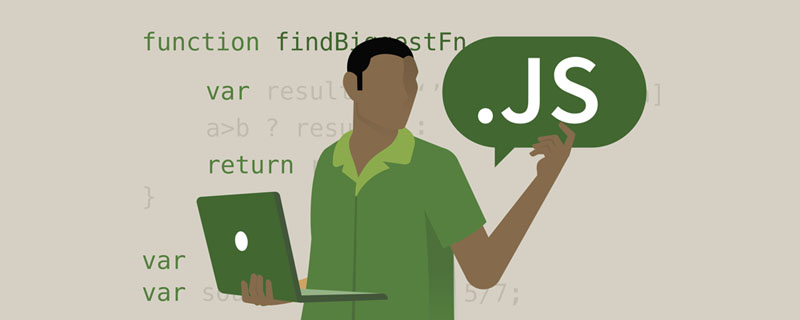
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
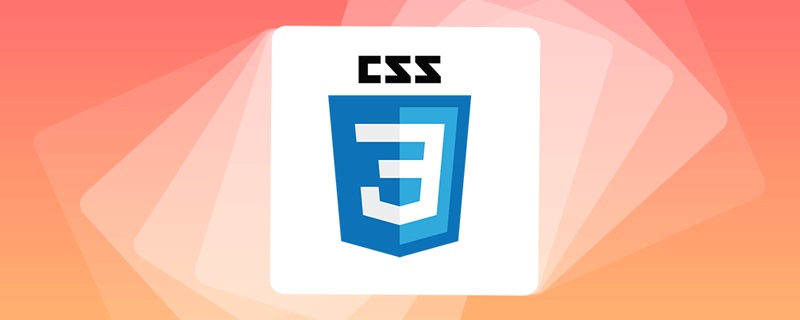
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
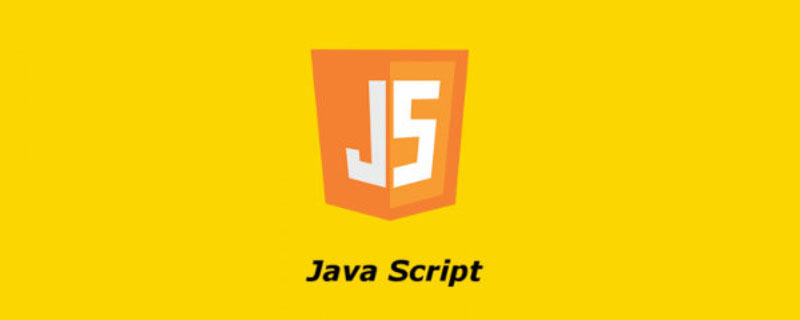
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
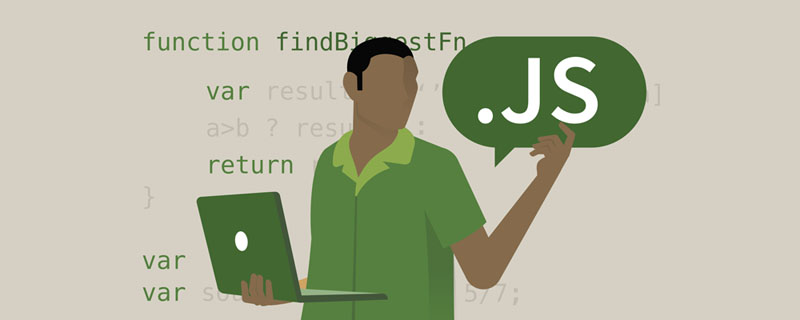
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
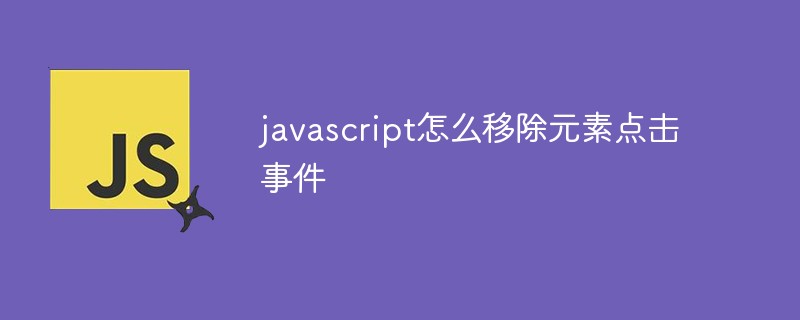
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
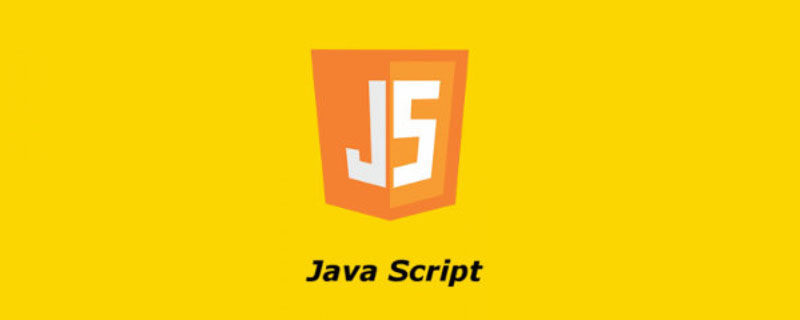
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
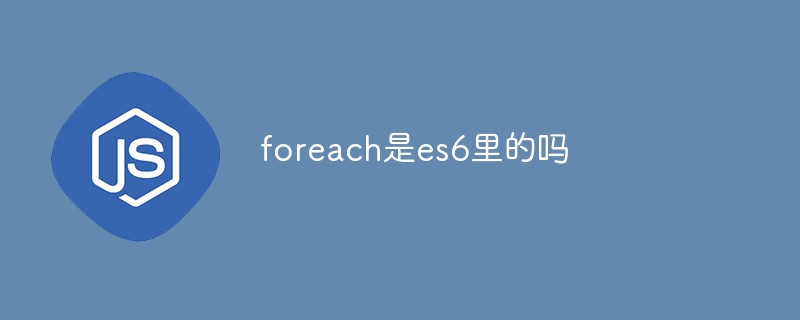
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
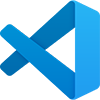
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
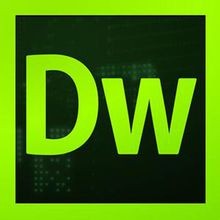
Dreamweaver CS6
Visual web development tools
