What is CGI
CGI is currently maintained by NCSA. NCSA defines CGI as follows:
CGI (Common Gateway Interface), common gateway interface, which is a program that runs on the server such as: HTTP server, providing the same client HTML page Interface.
Web browsing
To better understand how CGI works, we can start with the process of clicking a link or URL on a web page:
1. Use your browser to access the URL and connect to the HTTP web server.
2. After receiving the request information, the web server will parse the URL and check whether the accessed file exists on the server. If the file exists, return the content of the file, otherwise an error message will be returned.
3. The browser receives information from the server and displays the received file or error message.
CGI programs can be Python scripts, PERL scripts, SHELL scripts, C or C++ programs, etc.
CGI Architecture Diagram
Web Server Support and Configuration
Before you perform CGI programming, make sure that your Web server supports CGI and has configured a CGI handler.
All HTTP server execution CGI programs are saved in a pre-configured directory. This directory is called the CGI directory, and by convention, it is named /var/www/cgi-bin.
The extension of CGI files is .cgi, and python can also use the .py extension.
By default, the cgi-bin directory where the Linux server is configured to run is /var/www.
If you want to specify other directories for running CGI scripts, you can modify the httpd.conf configuration file as follows:
AllowOverride None
Options ExecCGI
Order allow,deny
Allow from all
Options All
Directory>
The first CGI program
We use Python to create the first CGI program. The file name is hellp.py. The file is located in the /var/www/cgi-bin directory. The content is as follows. Modify the file The permissions are 755:
#!/usr/bin/python
print "Content-type: text/htmlrnrn"
print ''
print '
'print '
print ''
print '
'print '
Hello Word! This is my first CGI program
'CONTENT_TYPE The value of this environment variable indicates the MIME type of the information passed. Currently, the environment variable CONTENT_TYPE is generally: application/x-www-form-urlencoded, which indicates that the data comes from HTML forms.
CONTENT_LENGTH If the information transmission method between the server and the CGI program is POST, this environment variable is the number of bytes of valid data that can be read from the standard input STDIN. This environment variable must be used when reading the entered data.
HTTP_COOKIE The COOKIE content in the client.
HTTP_USER_AGENT Provides client browser information including version number or other proprietary data.
PATH_INFO The value of this environment variable represents other path information immediately after the CGI program name. It often appears as a parameter to CGI programs.
QUERY_STRING If the information transfer method between the server and the CGI program is GET, the value of this environment variable is the information transferred. This information follows the CGI program name, separated by a question mark '?'.
REMOTE_ADDR The value of this environment variable is the IP address of the client sending the request, such as 192.168.1.67 above. This value is always present. And it is the unique identifier that the Web client needs to provide to the Web server, which can be used in CGI programs to distinguish different Web clients.
REMOTE_HOST The value of this environment variable contains the host name of the client that sent the CGI request. If the query you want to query is not supported, there is no need to define this environment variable.
REQUEST_METHOD Provides the method by which the script is called. For scripts using the HTTP/1.0 protocol, only GET and POST are meaningful.
SCRIPT_FILENAME The full path of the CGI script
SCRIPT_NAME The name of the CGI script
SERVER_NAME This is the host name, alias or IP address of your WEB server.
SERVER_SOFTWARE The value of this environment variable contains the name and version number of the HTTP server that calls the CGI program. For example, the above value is Apache/2.2.14 (Unix)
The following is a simple CGI script that outputs CGI environment variables:
#!/usr/bin/python
import os
print "Content-type: text/htmlrnrn";
print "Environment
";
for param in os.environ.keys():
print "%20s: %s
" % (param, os.environ[param])
GET and POST methods
The browser client passes two There are two ways to transmit information to the server, the two methods are the GET method and the POST method.
Use the GET method to transmit data
The GET method sends the encoded user information to the server. The data information is included in the URL of the request page, separated by "?", as shown below:
http: //www.test.com/cgi-bin/hello.py?key1=value1&key2=value2
Some other notes about GET requests:
GET requests can be cached
GET requests remain in the browser
GET request can be bookmarked in the history
GET request should not be used when handling sensitive data
GET request has a length limit
GET request should only be used to retrieve data
Simple url example: GET Method
The following is a simple URL that uses the GET method to send two parameters to the hello_get.py program:
/cgi-bin/hello_get.py?first_name=ZARA&last_name=ALI
The following Code for hello_get.py file:
#!/usr/bin/python
# CGI processing module
import cgi, cgitb
# Create an instantiation of FieldStorage
form = cgi.FieldStorage()
# Get data
first_name = form.getvalue('first_name')
last_name = form.getvalue('last_name')
print "Content-type: text/htmlrnrn"
print ""
print "
"print "
print ""
print "
"print "
Hello %s %s
" % (first_name, last_name)print ""
print ""
Browser request output result:
Hello ZARA ALI
Simple form example: GET method
The following is an HTML form that uses the GET method to send two data to the server. The submitted server script is also the hello_get.py file. The code is as follows:
Use POST method to pass data
Use POST method Transmitting data to the server is more secure and reliable. Some sensitive information such as user passwords need to be transmitted using POST.
The following is also hello_get.py, which can also process POST form data submitted by the browser:
#!/usr/bin/python
# Introducing the CGI module
import cgi, cgitb
# Create FieldStorage instance
form = cgi.FieldStorage()
# Get form data
first_name = form.getvalue('first_name')
last_name = form.getvalue('last_name')
print "Content-type:text/htmlrnrn"
print ""
print "
"print "
print ""
print "
"print "
Hello %s %s
" % (first_name, last_name)print " "
print ""
The following form submits data to the server script hello_get.py through the POST method:
The following is the code of the checkbox.cgi file:
#!/usr/bin/python
# Introduce CGI processing module
import cgi, cgitb
# Create an instance of FieldStorage
form = cgi.FieldStorage()
# Receive field data
if form.getvalue('maths' ):
math_flag = "ON"
else:
math_flag = "OFF"
if form.getvalue('physics'):
physics_flag = "ON"
else:
Physics_flag = " OFF"
print "Content-type:text/htmlrnrn"
print ""
print "
"print "
print ""
print "
"print "
CheckBox Maths is : %s
" % math_flagprint "
CheckBox Physics is : %s
" % physics_flagprint ""
print ""
Transfer Radio data through CGI program
Radio only to The server passes a data, the HTML code is as follows:
radiobutton.py 脚本代码如下:
#!/usr/bin/python
# Import modules for CGI handling
import cgi, cgitb
# Create instance of FieldStorage
form = cgi.FieldStorage()
# Get data from fields
if form.getvalue('subject'):
subject = form.getvalue('subject')
else:
subject = "Not set"
print "Content-type:text/htmlrnrn"
print ""
print "
"print "
print ""
print "
"print "
Selected Subject is %s
" % subjectprint ""
print ""
通过CGI程序传递 Textarea 数据
Textarea向服务器传递多行数据,HTML代码如下:
textarea.cgi脚本代码如下:
#!/usr/bin/python
# Import modules for CGI handling
import cgi, cgitb
# Create instance of FieldStorage
form = cgi.FieldStorage()
# Get data from fields
if form.getvalue('textcontent'):
text_content = form.getvalue('textcontent')
else:
text_content = "Not entered"
print "Content-type:text/htmlrnrn"
print ""
print "
";print "
print ""
print "
"print "
Entered Text Content is %s
" % text_contentprint ""
通过CGI程序传递下拉数据
HTML下拉框代码如下:
dropdown.py 脚本代码如下所示:
#!/usr/bin/python
# Import modules for CGI handling
import cgi, cgitb
# Create instance of FieldStorage
form = cgi.FieldStorage()
# Get data from fields
if form.getvalue('dropdown'):
subject = form.getvalue('dropdown')
else:
subject = "Not entered"
print "Content-type:text/htmlrnrn"
print ""
print "
"print "
print ""
print " & lt; body & gt; "
Print" & lt; h2 & gt; select subject is%s & lt;/h2 & gt; gt; "" ""
Cookies used in CGIA big shortcoming of the http protocol is that it does not judge the user's identity, which brings great inconvenience to programmers. The emergence of the cookie function makes up for this shortcoming. All cookies are to write record data on the customer's hard drive through the customer's browser when the customer accesses the script. The data information will be retrieved the next time the customer accesses the script, thereby achieving the function of identity discrimination. Cookies are commonly used in Password is being determined. Cookie syntaxhttp cookie is sent through the http header, which is earlier than the file transfer. The syntax of set-cookie in the header is as follows: Set-cookie:name= name;expires=date;path=path;domain=domain;secure name=name: You need to set the cookie value (name cannot use ";" and ","), when there are multiple name values Separate with ";" For example: name1=name1;name2=name2;name3=name3. expires=date: The validity period of cookie, format: expires="Wdy,DD-Mon-YYYY HH:MM:SS" path=path: Set the path supported by cookie, if path is a path, then Cookies are effective for all files and subdirectories in this directory, for example: path="/cgi-bin/". If path is a file, the cookie is effective for this file, for example: path="/cgi-bin/cookie" .cgi". domain=domain: The domain name that is valid for the cookie, for example: domain="www.chinalb.com" secure: If this flag is given, it means that the cookie can only be delivered through the https server of the SSL protocol. The reception of cookies is achieved by setting the environment variable HTTP_COOKIE. CGI programs can obtain cookie information by retrieving this variable. Cookie SettingsCookie setting is very simple, the cookie will be sent separately in the http header. The following example sets UserID and Password in cookies:<p></p>#!/usr/bin/python<p></p> <p></p>print "Set-Cookie:UserID=XYZ;rn"<p></p>print "Set-Cookie:Password=XYZ123;rn"<p></p>print "Set-Cookie:Expires=Tuesday, 31-Dec-2007 23:12:40 GMT";rn"<p></p>print "Set-Cookie:Domain=www. ziqiangxuetang.com;rn"<p></p>print "Set-Cookie:Path=/perl;n"<p></p>print "Content-type:text/htmlrnrn"<p></p>.....Rest of the HTML Content....<p></p> <p></p><p></p>The above example uses Set-Cookie header information to set Cookie information. Other attributes of Cookie are optionally set, such as expiration time Expires, domain name, and path. This information is set in. "Content-type: text/htmlrnrn". <p></p>Retrieve Cookie information<p></p>Cookie information retrieval page is very simple. Cookie information is stored in the CGI environment variable HTTP_COOKIE, and the storage format is as follows: <p></p><p></p><p></p>key1=value1; key2=value2;key3=value3....<p></p> <p></p><p></p>The following is a simple CGI program to retrieve cookie information: <p></p><p></p><p></p>#!/usr/bin/python<p></p> <p></p>#Import modules for CGI handling <p></p>from os import environ<p></p>import cgi, cgitb<p></p> <p></p>if environ.has_key('HTTP_COOKIE'):<p></p> for cookie in map(strip, split(environ['HTTP_COOKIE'], ';' )):<p></p> (key, value) = split(cookie, '=');<p></p> if key == "UserID":<p></p> user_id = value<p></p><p></p> if key == "Password" :<p></p> password = value<p></p><p></p>print "User ID = %s" % user_id<p></p>print "Password = %s" % password<p></p> <p></p><p></p>The output of the above script is as follows: <p></p><p></p><p></p>U ser ID = XYZ<p> DPassword = xyz123</p><p> File Upload example: </p><p>Html Set the form of upload file to set the enableype property as Multipart/Form-Data, the code is as shown below: </p><p></p><p></p><p> & lt; html & gt; </p><p> </p><p> </p><p> </p><p><input type="submit" value="Upload"></p><p> </p><p></p> <p></p><p> </p><p></p><p>save_file.py script file code is as follows: </p><p></p><p></p><p>#!/usr/bin/python</p><p> </p><p>import cgi, os</p><p>import cgit b; cgitb.enable ()</p><p></p><p>form = cgi.FieldStorage()</p><p></p><p># Get the file name</p><p>fileitem = form['filename']</p><p></p><p># Check whether the file is uploaded</p><p>if fileitem.filename:</p> <p> # Set file path </p><p> fn = os.path.basename(fileitem.filename) </p><p> open('/tmp/' + fn, 'wb').write(fileitem.file.read())</p><p> </p><p> message = 'The file "' + fn + '" was uploaded successfully'</p><p> </p><p>else:</p><p> message = 'No file was uploaded'</p><p>print """</p><p>Content-Type: text/htmln </p><p>< ;html></p><p></p><p> </p><p>%s</p><p></p><p></p><p>""" % (message,)</p><p> </p> <p></p> <p>If the system you are using is Unix/Linux, you must replace the file separator. Under window, you only need to use the open() statement: </p><p></p><p></p><p>fn = os.path.basename(fileitem.filename.replace ("\", "/" ))</p><p> </p><p></p><p>File download dialog box</p><p>If we need to provide the user with a file download link and pop up the file download dialog box after the user clicks the link, we set the HTTP header information To implement these functions, the function code is as follows: </p><p></p><p></p><p>#!/usr/bin/python</p><p></p><p># HTTP Header</p><p>print "Content-Type: application/octet-stream; name="FileName"rn ";</p><p>print "Content-Disposition: attachment; filename="FileName"rnn";</p><p></p><p># Actual File Content will go hear.</p><p>fo = open("foo.txt", "rb")</p><p> </p><p>str = fo.read();</p><p>print str</p><p></p><p>#Close opend file</p><p>fo.close()</p><p> </p><p></p><p></p><p></p>
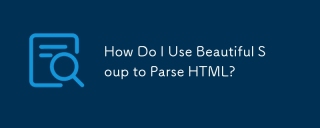
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
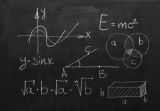
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
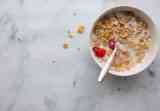
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
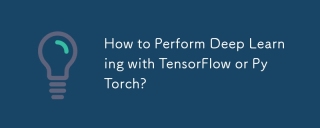
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
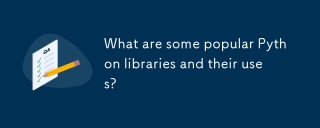
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
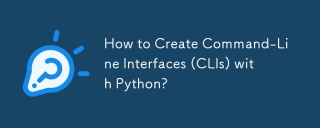
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
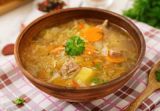
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
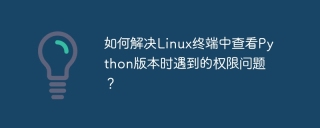
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
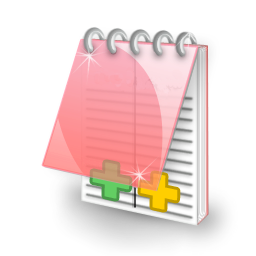
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
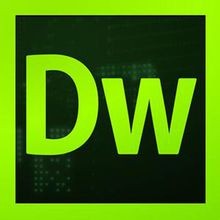
Dreamweaver CS6
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
