Basic Principle
In js, variables include 5 basic types and a complex data type Object. Of course, commonly used functions and arrays are all objects. For basic types and complex types, there are two different storage methods - stack storage and heap storage. The reason why two storage methods are implemented is very simple, that is, once the basic type is initialized, the memory size is fixed, and accessing the variable means accessing the actual data in the memory of the variable, which is called access by value. The object type may increase its size at some point, and the memory size is not fixed. For example, dynamically adding attributes of objects, dynamically increasing the size of arrays, etc. will increase the size of variables and cannot be maintained on the stack. Therefore, js puts the object type variables into the heap, allowing the interpreter to allocate memory for it as needed, and access it through the reference pointer of the object. Because the memory address size of the object in the heap is fixed, it can be The memory address is stored in a reference to stack memory. This method is called access by reference. Well, it is important to understand this and you can avoid a lot of problems in future programming. Let’s take a look at the following code:
var a = 'I am a string.'; //a,b,c的变量中保存的都是实际的值,因为他们是基本类型的变量 var b = 1010; var c = false; var d = a; //d中保存着和“a值一样的副本,它们互不影响” a = 'I am different from d'; alert(d); //输出'I am a string'
The above code is easy to understand, that is to say, the copy of variables accessed by value “What’s yours is yours, what’s mine is mine, we all have copies and do not affect each other.” And for variables accessed by value, Access by reference is slightly different:
var e = { name : 'I am an object', setName : function(name){ this.name = name; } }; var f = e; //赋值操作,实际上的结果是e,f都是指向那个对象的引用指针 f.setName('I am different from e,I am object f.'); alert(e.name); //对f进行操作,e的值也改变了!
For reference type assignment, to put it bluntly, the pointer of the object is copied. Both pointers point to the same entity object. There is no copy, and the original object remains only one! good. The above is the biggest and most fundamental difference between basic types and reference types! I use a picture to express it vividly:
* Basic type variables and object pointers are stored in the stack memory; object entities are stored in the heap
*Copy the front and rear stacks and heaps The situation in
Problems caused by reference types
1. The problem of using the prototype model to create objects
We all know that in JavaScript OO (Object Oriented), the biggest benefit of using the prototype model to create objects is that it can make object instances Share the properties and methods contained in the prototype. This avoids the shortcomings of the constructor pattern, that is, each object will have a copy of each method, and each method will be recreated on each instance, making method reuse meaningless.
Well, using the prototype pattern shares methods for all instances, but when there are properties with reference type values in the prototype, the problem arises:
var Person = function(){ }; Person.prototype = { constructor : Person, name : 'Hanzongze', hobby : ['basketable', 'swiming', 'running'], //注意,这里包含着一个引用类型的属性 sayName : function(){ alert(this.name); } }; var person1 = new Person(); var person2 = new Person(); person1.hobby.push('music'); alert(person2.hobby); //输出为'basketable', 'swiming', 'running','music' alert(person1.hobby === person2.hobby); //true
Since the hobby property is a reference type value, it is used by the Person constructor The hobby attributes of the created instances will all point to this reference entity, and the attributes between instance objects interfere with each other. This is not the result we want. To avoid this type of problem, the solution is to use a combination of constructor model and prototype model:
var Person = function(){ this.name = 'Hanzongze'; this.hobby = ['basketable', 'swiming', 'running']; //对引用类型的值使用构造函数模式 }; Person.prototype = { constructor : Person, sayName : function(){ alert(this.name); } }; var person1 = new Person(); var person2 = new Person(); person1.hobby.push('music'); alert(person2.hobby); //输出 'basketable', 'swiming', 'running',说明对person1的修改没有影响到person2 alert(person1.hobby === person2.hobby); //false
2. Problems in prototypal inheritance
The essence of this problem is actually the same as the previous one. It just happens in the context of prototypal inheritance. Look at a prototype chain inheritance problem:
var Person = function(){ this.name = 'Hanzongze'; this.hobby = ['basketable', 'swiming', 'running']; }; Person.prototype = { constructor : Person, sayName : function(){ alert(this.name); } }; //子类型Student function Student(){ } Student.prototype = new Person(); //Student继承了Person var student1 = new Student(); var student2 = new Student(); student1.hobby.push('music'); //对子类实例student1的引用属性做了改动 var student3 = new Student(); alert(student2.hobby); //输出'basketable', 'swiming', 'running', 'music' alert(student3.hobby); //输出'basketable', 'swiming', 'running', 'music'
In this code, you can see that the subclass Student inherits from the parent class Person. But since prototypal inheritance is used, that is to say, the instance of the parent class serves as the prototype of the subclass, so the reference type attributes in the instance are also inherited in the prototype prototype of the subclass. Instances of the subclass share the reference attribute and influence each other.
The solution is to use the borrowed constructor solution (but it is not an ideal solution. The ideal solution is to use a combination of prototype chain and borrowed constructor. It involves a lot of inheritance patterns. I will briefly describe it here. I will write an article in the future. Detailed article):
var Person = function(){ this.name = 'Hanzongze'; this.hobby = ['basketable', 'swiming', 'running']; }; Person.prototype = { constructor : Person, sayName : function(){ alert(this.name); } }; function Student(){ //借用构造函数,继承了Person Person.call(this); } var student1 = new Student(); var student2 = new Student(); student1.hobby.push('music'); alert(student2.hobby); //输出'basketable', 'swiming', 'running', 'music'
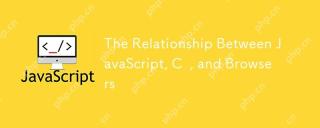
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
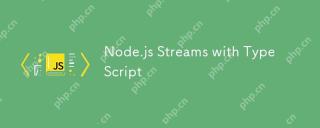
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
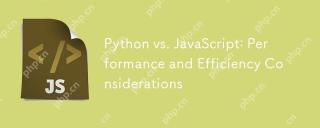
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
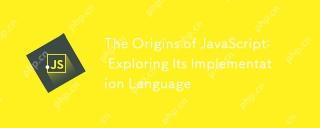
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
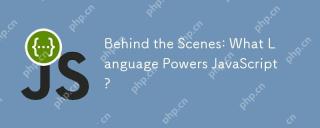
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
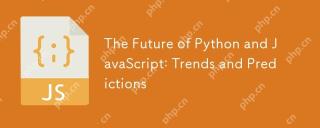
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
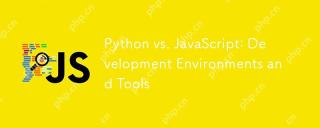
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
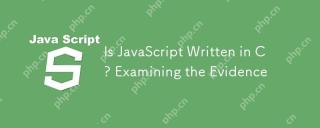
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
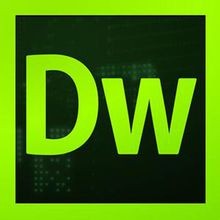
Dreamweaver CS6
Visual web development tools
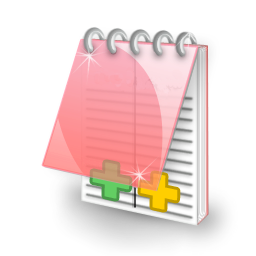
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
